Browse Course Material
Course info, instructors.
- Prof. Eric Grimson
- Prof. John Guttag

Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
Introduction to Computer Science and Programming
Assignments.

You are leaving MIT OpenCourseWare
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
CSE341: Homework and Study Note for Programming Languages on Coursera
Weiting-Zhang/Programming-Languages
Folders and files, repository files navigation, programming languages on coursera.
This course is an introduction to the basic concepts of programming languages, with a strong emphasis on functional programming . The course uses the languages ML (in Part A ), Racket (in Part B ), and Ruby (in Part C ) as vehicles for teaching the concepts, but the real intent is to teach enough about how any language “fits together” to make you more effective programming in any language -- and in learning new ones.
Introduction
This repo contains all my work for this course. All the code base, quiz questions, screenshot, and images, are taken from, unless specified, Programming Languages on Coursera . The course is also avilable on washington.edu .
What I want to say
VERBOSE CONTENT WARNING: YOU CAN JUMP TO THE NEXT SECTION IF YOU WANT
Here I released these solutions, which are only for your reference purpose . It may help you to save some time. And I hope you don't copy any part of the code (the programming assignments are fairly easy if you read the instructions carefully), see the quiz solutions before you start your own adventure.
Currently, this repo has 3 major parts you may be interested in and I will give a list here.
Homework Assignments and Exams
Part A: ML and Functional Pragramming
- Week 2 - Homework 1 - ML Functions, Tuples, Lists, and More : 104/100
- Week 3 - Homework 2 - Datatypes, Pattern Matching, Tail Recursion, and More : 104/100
- Week 4 - Homework 3 - First-Class Functions and Closures : 100/100
- Week 5 - Part A - Exam
Part B: Racket and Dynamically Typed Language
- Week 1 - Homework 4 - Racket, Delaying Evaluation, Memoization, Macros
- Week 2 - Homework 5 - Structs, Implementing Languages, Static vs. Dynamic Typing
- Week 3 - Section Quiz
Part C: Ruby and Object-Oriented Language
- Week 1 - Homework 6 - Ruby, Object-Oriented Programming, Subclassing
- Week 2 - Homework 7 - Program Decomposition, Mixins, Subtyping, and More
- Week 3 - Final Exam
- Standard ML 100.0%

Learning programming languages
Strategies for programming assignments, citing code.
Coding assignments can range from simple programs to full-blown applications. It is important to know how to approach such assignments, so that you can complete them to the best of your ability. Some platforms you may be asked to develop for are:
- Web — websites and web apps for browsers like Chrome or Firefox
- Mobile — mobile apps for iOS and Android
- Desktop — desktop applications or programs for Windows, macOS and Linux.
If you are starting a coding assignment in a programming language that you are not familiar with, there are tutorials you can take to understand the basics in a few hours:
- Learn a range of programming language training and tutorials from LinkedIn Learning (UQ login is required)
- Codecademy has courses on web development, data science and computer science
- w3schools has tutorials on web development languages — HTML, CSS, JavaScript, Python etc.
Get more information on tools for web, software and mobile application development .
Universal strategies that can be applied to make your programming assignments easier:
- Start early — this gives you more time to think about the task and how you might approach it, but also more time to get help, if needed
- Plan your program using pseudocode — pseudocode is a great method for planning what you want to code in way that is easy for people to understand
- Create the HTML, CSS and JavaScript files
- Link the CSS and JavaScript files using the appropriate tags in your HTML file.
- Use comments — all programming languages allow you to write comments that are lines which are ignored by the program. A great way to use comments is to type out the steps required for coding a piece of functionality. You can then follow these steps to code it line by line
- Take a break — if you ever get stuck, it’s probably a good time to take a break. Breaks as short as 5 minutes can be enough to clear your mind
- Explain your problems to a rubber duck — Rubber duck debugging is actually a legitimate way of solving programming related problems. It works simply by explaining your problems aloud, which can help you find what’s causing bugs in your code.
Students should confirm what is permitted for a specific assignment prior to commencing. Some assignments do not permit the use of external support and all code must be written by the individual. However, if you are allowed to use externally sourced code it must be referenced if it is not your own original work. Failure to reference externally sourced, non-original work can result in misconduct proceedings. An external source is code, including from anywhere on the internet or from a tutorial, taken or used to write your own code.
References should provide clear and accurate information for each source and should identify where they have been used in your work. A single URL is not a complete or accurate reference. URLs should link directly to the work cited, not just to the website it is hosted on. An example format for referencing online sources:
[where used] : [title of asset], [creator of asset]; retrieved from [website title] ([URL]), Last accessed [DD/MM/YYYY}
For code references, the [where used] should map to the position in the code e.g. in the code itself, create a comment to identify where in your code you have used an external source:
For code with no changes or adaptations
(How to comment) retrieved from Unity Answers : how to comment the lines? (http://answers.unity3d.com/answers/221574/view.html target=”_blank” rel=”noopener noreferrer”) Last accessed 11/9/2017
Ensure you are using syntax specifically for the programming language being used.
For code that you have changed or adapted
You should describe how you adapted it.
(Output Loop) retrieved from Unity Answers : Writing a Loop (http://answers.unity3d.com/answers/221574/view.html target=”_blank” rel=”noopener noreferrer”) Last accessed 9/9/2017
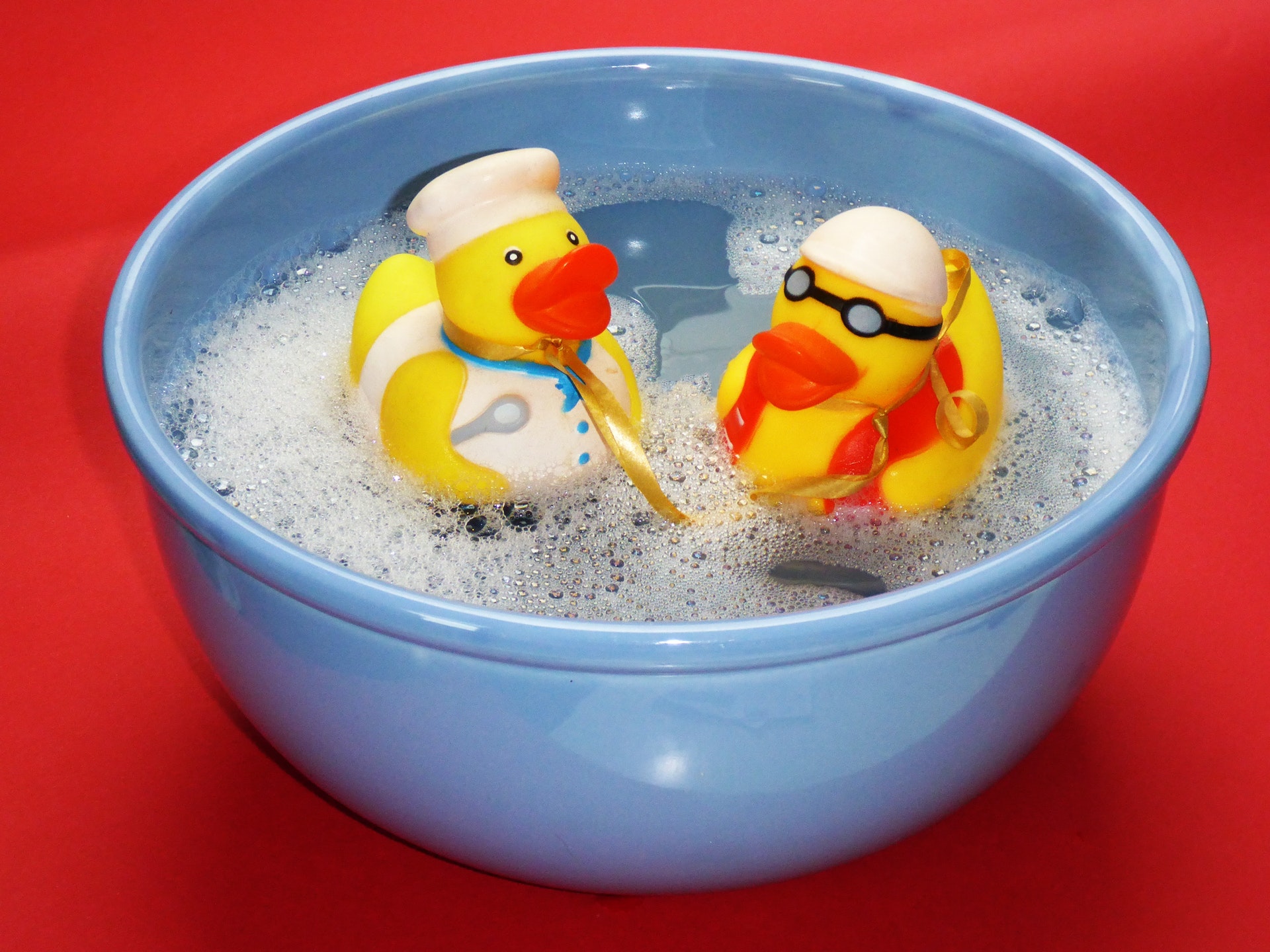
Types of Assignments Copyright © 2023 by The University of Queensland is licensed under a Creative Commons Attribution-NonCommercial 4.0 International License , except where otherwise noted.
Share This Book

Want to create or adapt books like this? Learn more about how Pressbooks supports open publishing practices.
Kenneth Leroy Busbee
An assignment statement sets and/or re-sets the value stored in the storage location(s) denoted by a variable name; in other words, it copies a value into the variable. [1]
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within most programming languages the symbol used for assignment is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
Simple Assignment
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
Assignment with an Expression
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would be assigned to the variable named: total_cousins.
Assignment with Identifier Names in the Expression
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
- cnx.org: Programming Fundamentals – A Modular Structured Approach using C++
- Wikipedia: Assignment (computer science) ↵
Programming Fundamentals Copyright © 2018 by Kenneth Leroy Busbee is licensed under a Creative Commons Attribution-ShareAlike 4.0 International License , except where otherwise noted.
Share This Book
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
Bitwise Operators in C Programming
C Precedence And Associativity Of Operators
- Compute Quotient and Remainder
- Find the Size of int, float, double and char
C if...else Statement
- Make a Simple Calculator Using switch...case
C Programming Operators
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition.
C has a wide range of operators to perform various operations.
C Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
Example 1: Arithmetic Operators
The operators + , - and * computes addition, subtraction, and multiplication respectively as you might have expected.
In normal calculation, 9/4 = 2.25 . However, the output is 2 in the program.
It is because both the variables a and b are integers. Hence, the output is also an integer. The compiler neglects the term after the decimal point and shows answer 2 instead of 2.25 .
The modulo operator % computes the remainder. When a=9 is divided by b=4 , the remainder is 1 . The % operator can only be used with integers.
Suppose a = 5.0 , b = 2.0 , c = 5 and d = 2 . Then in C programming,
C Increment and Decrement Operators
C programming has two operators increment ++ and decrement -- to change the value of an operand (constant or variable) by 1.
Increment ++ increases the value by 1 whereas decrement -- decreases the value by 1. These two operators are unary operators, meaning they only operate on a single operand.
Example 2: Increment and Decrement Operators
Here, the operators ++ and -- are used as prefixes. These two operators can also be used as postfixes like a++ and a-- . Visit this page to learn more about how increment and decrement operators work when used as postfix .
C Assignment Operators
An assignment operator is used for assigning a value to a variable. The most common assignment operator is =
Example 3: Assignment Operators
C relational operators.
A relational operator checks the relationship between two operands. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Relational operators are used in decision making and loops .
Example 4: Relational Operators
C logical operators.
An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming .
Example 5: Logical Operators
Explanation of logical operator program
- (a == b) && (c > 5) evaluates to 1 because both operands (a == b) and (c > b) is 1 (true).
- (a == b) && (c < b) evaluates to 0 because operand (c < b) is 0 (false).
- (a == b) || (c < b) evaluates to 1 because (a = b) is 1 (true).
- (a != b) || (c < b) evaluates to 0 because both operand (a != b) and (c < b) are 0 (false).
- !(a != b) evaluates to 1 because operand (a != b) is 0 (false). Hence, !(a != b) is 1 (true).
- !(a == b) evaluates to 0 because (a == b) is 1 (true). Hence, !(a == b) is 0 (false).
C Bitwise Operators
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
Bitwise operators are used in C programming to perform bit-level operations.
Visit bitwise operator in C to learn more.
Other Operators
Comma operator.
Comma operators are used to link related expressions together. For example:
The sizeof operator
The sizeof is a unary operator that returns the size of data (constants, variables, array, structure, etc).
Example 6: sizeof Operator
Other operators such as ternary operator ?: , reference operator & , dereference operator * and member selection operator -> will be discussed in later tutorials.
Table of Contents
- Arithmetic Operators
- Increment and Decrement Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- sizeof Operator
Video: Arithmetic Operators in C
Sorry about that.
Related Tutorials

Chelsea Troy
How to implement variable assignment in a programming language.
I’m working my way through Crafting Interpreters . The project guides programmers through building their own interpreters for the Lox programming language. I started writing a blog series about my progress. You can see all the posts so far right here .
In the last post covering part of Chapter 7, we talked about error handling, at that time largely in the context of parsing and interpreting expressions.
Now in Chapter 8 we get into some of the fun stuff—state.

I have talked about state a couple of times in the past on this blog—in particular, here , during the series about the book Structure and Interpretation of Computer Programs. If you like this series, you’ll probably like that one 😉.

The short version:
Procedural code without state—for example, a stateless function—amounts to a series of substitution operations in which an argument name serves as a placeholder that gets substituted at runtime with the passed-in inputs. The same inputs always result in the same output, and this makes the function “pure.” (In Crafting Interpreters , Bob Nystrom points out what we’re all thinking: that the programmers who wax poetic about their devotion to dispassionate logic also label said functions with such weighty language as “pure.”)
Add state, though, and this changes . Bob equates the presence of state to the Lox programming language’s “memory.” And just like a human memory, it influences statements’ behavior. The result from print a depends on what we assigned to a .
It also means that the programming language has to recognize a , which we can accomplish by:
- Parsing some assignment syntax like var a = "Hallo!"
- Interpreting that assignment syntax by assigning a key a in a key-value store to point to the value "Hallo!"
The key-value store doesn’t have to be all that fancy.
In the 80 line Scheme interpreter that we talked about in this previous post , we use a plain Python dictionary for the task. In fact, Python itself used dicts to delineate scope in early versions ( here’s the PEP where Python solidified the thrust of their current approach to state scoping, back in version 2.1). In Crafting Interpreters , Bob does similar:
The Environment class wraps a plain Java HashMap . Two methods wrap the HashMap ‘s put and get functions with semantically relevant names. That’s it.
This implementation of Environment supports only global scope: there’s one environment, all its variables are accessible everywhere, and either they’re in there or they’re not. Later, when we endeavor to include nested scopes, Environment gets a new attribute—a reference to another Environment , set to nil for the global scope—and when a variable is referenced, the environments check themselves and then, recursively, their enclosing environments, until they reach the global one.
I’ll bring up that SICP post one more time here because we do something similar in that implementation, although we copy the parent environment rather than referencing it:
For nested expressions, our nested environments are recursively passed through, first to seval (which evaluates expressions), then back into a new environment if evaluation reveals another procedure. …we’re slapping our new local env on top of a copy of the existing environment, and for a huge environment that will be really space inefficient. We’ve talked on this blog before about a similar situation: tracking nested transactions in an implementation of an in-memory database . If this were a real interpreter and not a toy for learning purposes, I might turn to a solution like that one. Note that the nested scopes wouldn’t face that annoying deletion snafu we faced with the database: local scopes can overwrite the values of variables from broader scopes, but they don’t delete them.
So the implementation isn’t super clever.
P.S. If you’re interested in trying out implementing a nested scope yourself, I gotchu! Here’s an interactive activity that I wrote for my Python Programming students to practice implementing database transactions for a pet shop database (complete with fun, dancing parrots!). It’s level-appropriate for someone who is comfortable using dictionaries/hashmaps/associative arrays in at least one modern (last updated after 2012) object-oriented programming language. To get this running locally, go to your command line and do:
- git clone https://github.com/MPCS-51042/materials.git
- cd materials
- pip install jupyter (there’s also a pipfile if you’re a pipenv user, but I don’t want folks who aren’t regular Pythonistas to have to screw around with virtual environments just to try this exercise)
- jupyter notebook (this is going to open a tab in your browser with a list of folders)
- Navigate to materials > database_efficiency_exercise > 2_Transactions_(Space_Efficiency).ipynb
- The file is a REPL: you can run any of the code cells with SHIFT + ENTER. I recommend starting by going to the top bar and clicking Kernel > Restart and Run All. This will run the code I have provided so you have the Table implementation available in scope, for example.
- Try out the activity! I have tried to make the instructions as clear as possible and would welcome feedback on whether you were able to complete the exercise on your own 😊
A simple implementation does not mean an absence of decisions, by the way.
From this chapter of Crafting Interpreters about variable declaration and assignment, one of the things I most appreciated was the time that Bob took to consider the decisions that programming language authors make about how variable referencing and assignment work. Let’s go over some of those decisions:
1. Will the language use lexical or dynamic scope for variables?
That is, will the are over which the variable is defined by visible in the structure of the code and predictable before runtime ( lexical scope )? In most modern programming languages, it is. But we can see an example of dynamic scope with methods on classes. Suppose we have two classes that each possess a method with the same signature, and a function takes in either of those two classes as an argument. Which of the twin methods is in scope at runtime depends on which class the argument passed to the function is an instance of. That class’s method is in scope. The other class’s method is not in scope.
Lexical scope allows us to use specific tokens to identify when to create a new child environment. In Lox, we use curly braces for this {} , as do Swift and Java. Ruby also functions with curly braces, although using do..end is more idiomatic. Python determines scope with level of indentation.
2. Will the language allow variable reassignment?
If we want immutable data that has no chance of changing out from under a programmer, then we can insist that the value of a variable remain the same after it is created until it is destroyed. Haskell does its best to force this, and constants in many languages aim to provide this option.
Most programming languages allow for mutable variables, though, that programmers can define once and then reassign later. Many of them distinguish these from constants with a specific keyword. For example, JavaScript provides const for immutable constant declaration and var for mutable variable declaration. Swift does the same with let and var . Kotlin does it with val and var (“var” is a very popular choice for this).
3. Will the language allow referencing undeclared variables?
What happens if a programmer says print a; and no a has been declared? Lox raises an error at compile time. Ruby, by contrast, allows it. In Ruby, puts a where a is not declared will print nothing, but it won’t error out. puts a.inspect will print nil . Bob calls this strategy “too permissive for me” in the chapter. I…tend to agree, but I also don’t relish being all the way at the other end, say, at Java 6, where we had to null check everything in perpetual fear of the dreaded Null Pointer Exception.
I’m a fan of the Optional paradigm that we’ve seen in some more modern programming language implementations like Java 8, Swift, and Kotlin. I want to know if a variable could be null so I can handle it appropriately.
4. Will the language allow implicit variable declaration?
Lox distinguishes between declaring a new variable ( var a = "What's"; ) and assigning it a new value ( a = "Up?" ;). Without that var keyword, it will assume the intent is to reassign, and it will raise an error at compile time because the variable is not yet declared.
Ruby and Python, by contrast, assume that an assignment to a variable that does not yet exist means declare it . So a = 1 in Ruby sets 1 to a if a is nil, and reassigns a to point to 1 if it was previously pointing to something else.
Convenient, for sure. But in my experience, this does open programmers up to more scope-related insidious bugs because an initialization of a local variable and a reassignment of a global one look the same.
Languages might always differ on some of these questions.
And that’s okay: different languages serve different purposes and different communities. A programming language author can establish and communicate a perspective on a language’s purpose and use cases, then allow those decisions to drive implementation choices.
If you liked this piece, you might also like:
This talk about what counts as a programming language , explained with a raccoon stealing catfood from a cat…somehow
The Raft series, of course! Learn to implement a distributed system from the comfort of your home, complete with gifs and smack talk!
Lessons from Space: Edge Free Programming —about what launchpads have in common with software, and what that means for you and your work!
Share this:
Leave a reply cancel reply.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Discover more from Chelsea Troy
Subscribe now to keep reading and get access to the full archive.
Type your email…
Continue reading
- Assignment Statement
An Assignment statement is a statement that is used to set a value to the variable name in a program .
Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted by a variable name.

The symbol used in an assignment statement is called as an operator . The symbol is ‘=’ .
Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’.
The Basic Syntax of Assignment Statement in a programming language is :
variable = expression ;
variable = variable name
expression = it could be either a direct value or a math expression/formula or a function call
Few programming languages such as Java, C, C++ require data type to be specified for the variable, so that it is easy to allocate memory space and store those values during program execution.
data_type variable_name = value ;
In the above-given examples, Variable ‘a’ is assigned a value in the same statement as per its defined data type. A data type is only declared for Variable ‘b’. In the 3 rd line of code, Variable ‘a’ is reassigned the value 25. The 4 th line of code assigns the value for Variable ‘b’.
Assignment Statement Forms
This is one of the most common forms of Assignment Statements. Here the Variable name is defined, initialized, and assigned a value in the same statement. This form is generally used when we want to use the Variable quite a few times and we do not want to change its value very frequently.
Tuple Assignment
Generally, we use this form when we want to define and assign values for more than 1 variable at the same time. This saves time and is an easy method. Note that here every individual variable has a different value assigned to it.
(Code In Python)
Sequence Assignment
(Code in Python)
Multiple-target Assignment or Chain Assignment
In this format, a single value is assigned to two or more variables.
Augmented Assignment
In this format, we use the combination of mathematical expressions and values for the Variable. Other augmented Assignment forms are: &=, -=, **=, etc.
Browse more Topics Under Data Types, Variables and Constants
- Concept of Data types
- Built-in Data Types
- Constants in Programing Language
- Access Modifier
- Variables of Built-in-Datatypes
- Declaration/Initialization of Variables
- Type Modifier
Few Rules for Assignment Statement
Few Rules to be followed while writing the Assignment Statements are:
- Variable names must begin with a letter, underscore, non-number character. Each language has its own conventions.
- The Data type defined and the variable value must match.
- A variable name once defined can only be used once in the program. You cannot define it again to store other types of value.
- If you assign a new value to an existing variable, it will overwrite the previous value and assign the new value.
FAQs on Assignment Statement
Q1. Which of the following shows the syntax of an assignment statement ?
- variablename = expression ;
- expression = variable ;
- datatype = variablename ;
- expression = datatype variable ;
Answer – Option A.
Q2. What is an expression ?
- Same as statement
- List of statements that make up a program
- Combination of literals, operators, variables, math formulas used to calculate a value
- Numbers expressed in digits
Answer – Option C.
Q3. What are the two steps that take place when an assignment statement is executed?
- Evaluate the expression, store the value in the variable
- Reserve memory, fill it with value
- Evaluate variable, store the result
- Store the value in the variable, evaluate the expression.
Customize your course in 30 seconds
Which class are you in.

Data Types, Variables and Constants
- Variables in Programming Language
- Concept of Data Types
- Declaration of Variables
- Type Modifiers
- Access Modifiers
- Constants in Programming Language
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

C Data Types
C operators.
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
C File Handling
- C Cheatsheet
C Interview Questions
- C Programming Language Tutorial
- C Language Introduction
- Features of C Programming Language
- C Programming Language Standard
- C Hello World Program
- Compiling a C Program: Behind the Scenes
- Tokens in C
- Keywords in C
C Variables and Constants
- C Variables
- Constants in C
- Const Qualifier in C
- Different ways to declare variable as constant in C
- Scope rules in C
- Internal Linkage and External Linkage in C
- Global Variables in C
- Data Types in C
- Literals in C
- Escape Sequence in C
- Integer Promotions in C
- Character Arithmetic in C
- Type Conversion in C
C Input/Output
- Basic Input and Output in C
- Format Specifiers in C
- printf in C
- Scansets in C
- Formatted and Unformatted Input/Output functions in C with Examples
- Operators in C
- Arithmetic Operators in C
- Unary operators in C
- Relational Operators in C
- Bitwise Operators in C
- C Logical Operators
Assignment Operators in C
- Increment and Decrement Operators in C
- Conditional or Ternary Operator (?:) in C
- sizeof operator in C
- Operator Precedence and Associativity in C
C Control Statements Decision-Making
- Decision Making in C (if , if..else, Nested if, if-else-if )
- C - if Statement
- C if...else Statement
- C if else if ladder
- Switch Statement in C
- Using Range in switch Case in C
- while loop in C
- do...while Loop in C
- For Versus While
- Continue Statement in C
- Break Statement in C
- goto Statement in C
- User-Defined Function in C
- Parameter Passing Techniques in C
- Function Prototype in C
- How can I return multiple values from a function?
- main Function in C
- Implicit return type int in C
- Callbacks in C
- Nested functions in C
- Variadic functions in C
- _Noreturn function specifier in C
- Predefined Identifier __func__ in C
- C Library math.h Functions
C Arrays & Strings
- Properties of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- What are the data types for which it is not possible to create an array?
- How to pass an array by value in C ?
- Strings in C
- Array of Strings in C
- What is the difference between single quoted and double quoted declaration of char array?
- C String Functions
- Pointer Arithmetics in C with Examples
- C - Pointer to Pointer (Double Pointer)
- Function Pointer in C
- How to declare a pointer to a function?
- Pointer to an Array | Array Pointer
- Difference between constant pointer, pointers to constant, and constant pointers to constants
- Pointer vs Array in C
- Dangling, Void , Null and Wild Pointers in C
- Near, Far and Huge Pointers in C
- restrict keyword in C
C User-Defined Data Types
- C Structures
- dot (.) Operator in C
- Structure Member Alignment, Padding and Data Packing
- Flexible Array Members in a structure in C
- Bit Fields in C
- Difference Between Structure and Union in C
- Anonymous Union and Structure in C
- Enumeration (or enum) in C
C Storage Classes
- Storage Classes in C
- extern Keyword in C
- Static Variables in C
- Initialization of static variables in C
- Static functions in C
- Understanding "volatile" qualifier in C | Set 2 (Examples)
- Understanding "register" keyword in C
C Memory Management
- Memory Layout of C Programs
- Dynamic Memory Allocation in C using malloc(), calloc(), free() and realloc()
- Difference Between malloc() and calloc() with Examples
- What is Memory Leak? How can we avoid?
- Dynamic Array in C
- How to dynamically allocate a 2D array in C?
- Dynamically Growing Array in C
C Preprocessor
- C Preprocessor Directives
- How a Preprocessor works in C?
- Header Files in C
- What’s difference between header files "stdio.h" and "stdlib.h" ?
- How to write your own header file in C?
- Macros and its types in C
- Interesting Facts about Macros and Preprocessors in C
- # and ## Operators in C
- How to print a variable name in C?
- Multiline macros in C
- Variable length arguments for Macros
- Branch prediction macros in GCC
- typedef versus #define in C
- Difference between #define and const in C?
- Basics of File Handling in C
- C fopen() function with Examples
- EOF, getc() and feof() in C
- fgets() and gets() in C language
- fseek() vs rewind() in C
- What is return type of getchar(), fgetc() and getc() ?
- Read/Write Structure From/to a File in C
- C Program to print contents of file
- C program to delete a file
- C Program to merge contents of two files into a third file
- What is the difference between printf, sprintf and fprintf?
- Difference between getc(), getchar(), getch() and getche()
Miscellaneous
- time.h header file in C with Examples
- Input-output system calls in C | Create, Open, Close, Read, Write
- Signals in C language
- Program error signals
- Socket Programming in C
- _Generics Keyword in C
- Multithreading in C
- C Programming Interview Questions (2024)
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
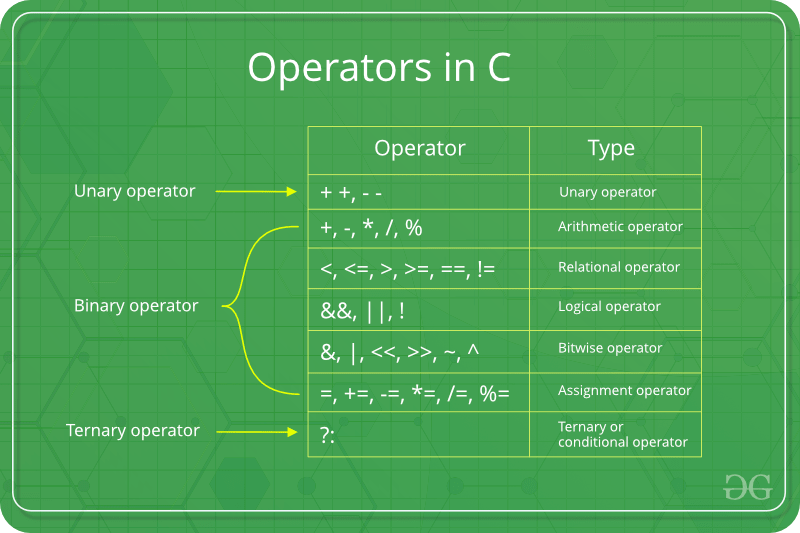
Assignment operators are used for assigning value to a variable. The left side operand of the assignment operator is a variable and right side operand of the assignment operator is a value. The value on the right side must be of the same data-type of the variable on the left side otherwise the compiler will raise an error.
Different types of assignment operators are shown below:
1. “=”: This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example:
2. “+=” : This operator is combination of ‘+’ and ‘=’ operators. This operator first adds the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a += 6) = 11.
3. “-=” This operator is combination of ‘-‘ and ‘=’ operators. This operator first subtracts the value on the right from the current value of the variable on left and then assigns the result to the variable on the left. Example:
If initially value stored in a is 8. Then (a -= 6) = 2.
4. “*=” This operator is combination of ‘*’ and ‘=’ operators. This operator first multiplies the current value of the variable on left to the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 5. Then (a *= 6) = 30.
5. “/=” This operator is combination of ‘/’ and ‘=’ operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result to the variable on the left. Example:
If initially value stored in a is 6. Then (a /= 2) = 3.
Below example illustrates the various Assignment Operators:
Please Login to comment...
Similar reads.
- C-Operators
- cpp-operator
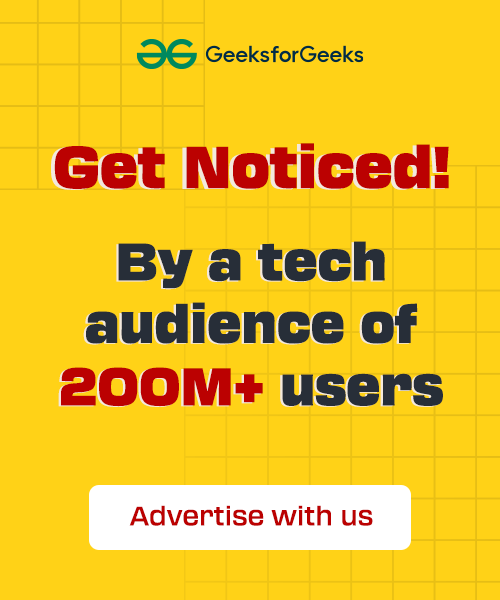
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
Assignments. pdf. 98 kB Getting Started: Python and IDLE. file. 193 B shapes. file. 3 kB subjects. file. 634 kB words. pdf. 52 kB ... Programming Languages; Download Course. Over 2,500 courses & materials Freely sharing knowledge with learners and educators around the world.
Module 2 • 1 hour to complete. This module contains two things: (1) The information for the [unusual] software you need to install for Programming Languages Part A. (2) An optional "fake" homework that you can turn in for auto-grading and peer assessment to get used to the mechanics of assignment turn-in that we will use throughout the course.
This course is an introduction to the basic concepts of programming languages, with a strong emphasis on functional programming. The course uses the languages ML (in Part A ), Racket (in Part B ), and Ruby (in Part C ) as vehicles for teaching the concepts, but the real intent is to teach enough about how any language "fits together" to ...
Programming Language Design and Usage Main Themes Programming Language as a Tool for Thought Idioms ... - programs have mutable storage (state) modified by assignments - the most common and familiar paradigm • object-oriented (Simula 67, Smalltalk, Eiffel, Ada95, Java, C#)
Programming Languages; Software Design and Engineering; Learning Resource Types notes Lecture Notes. group_work Projects. assignment_turned_in Programming Assignments with Examples. Download Course. Over 2,500 courses & materials Freely sharing knowledge with learners and educators around the world.
Some popular functional programming languages include: Scala. Erlang. Haskell. Elixir. F#. 3. Object-oriented programming languages (OOP) This type of language treats a program as a group of objects composed of data and program elements, known as attributes and methods.
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Coding - Types of Assignments. 8. Coding. Learning programming languages. Strategies for programming assignments. Citing code. Coding assignments can range from simple programs to full-blown applications. It is important to know how to approach such assignments, so that you can complete them to the best of your ability.
Discussion. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within most programming languages the symbol used for assignment is the equal symbol.
Assignment: An assignment is a statement in computer programming that is used to set a value to a variable name. The operator used to do assignment is denoted with an equal sign (=). This operand works by assigning the value on the right-hand side of the operand to the operand on the left-hand side. It is possible for the same variable to hold ...
Here are 10 basic coding projects for beginners: 1. Build a chess game. Building a chess game is a great way to hone your ability to think like a developer. It'll also allow you to practice using algorithms, as you'll have to create not only the board and game pieces but also the specific moves that each piece can make. 2.
To submit a programming assignment: Open the assignment page for the assignment you want to submit. Read the assignment instructions and download any starter files. Finish the coding tasks in your local coding environment. Check the starter files and instructions when you need to. If the assignment uses script submission, submit your assignment ...
C programming has two operators increment ++ and decrement -- to change the value of an operand (constant or variable) by 1. Increment ++ increases the value by 1 whereas decrement -- decreases the value by 1. These two operators are unary operators, meaning they only operate on a single operand.
Because Python is a dynamically typed language, successive assignments to a given variable can change the variable's data type. Changing the data type of a variable during a program's execution is considered bad practice and highly discouraged. ... Note that in a programming language that doesn't support this kind of assignment, you'd ...
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
The result from print a depends on what we assigned to a. It also means that the programming language has to recognize a, which we can accomplish by: Parsing some assignment syntax like var a = "Hallo!" Interpreting that assignment syntax by assigning a key a in a key-value store to point to the value "Hallo!"
Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms :-. 1. Basic form: This form is the most common form. p, *q = 'Hello'. print('p = ', p)
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other ...
The symbol used in an assignment statement is called as an operator. The symbol is '='. Note: The Assignment Operator should never be used for Equality purpose which is double equal sign '=='. The Basic Syntax of Assignment Statement in a programming language is : variable = expression ; where, variable = variable name
Object-oriented programming: C++ supports object-oriented programming paradigms, enabling developers to write modular and reusable code. Platform independence: C++ programs can be compiled and executed on different operating systems, making it a versatile language.
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
2. BASIC Programming language : BASIC programming language is one of the earliest and simplest high-level programming language which was a popular choice among many persons in 1970s, because of its simplicity and easy to learn capability.BASIC stands for Beginner's All- purpose Symbolic Instruction Code. The popular language was developed by John Kemeny and Thomas Kurtz in 1960s at Dartmouth ...