Home » JavaScript Object Methods » JavaScript Object.assign()

JavaScript Object.assign()
Summary : in this tutorial, you will learn how to use the JavaScript Object.assign() method in ES6.
The following shows the syntax of the Object.assign() method:
The Object.assign() copies all enumerable and own properties from the source objects to the target object. It returns the target object.
The Object.assign() invokes the getters on the source objects and setters on the target. It assigns properties only, not copying or defining new properties.
Using JavaScript Object.assign() to clone an object
The following example uses the Object.assign() method to clone an object .
Note that the Object.assign() only carries a shallow clone, not a deep clone.
Using JavaScript Object.assign() to merge objects
The Object.assign() can merge source objects into a target object which has properties consisting of all the properties of the source objects. For example:
If the source objects have the same property, the property of the later object overwrites the earlier one:
- Object.assign() assigns enumerable and own properties from a source object to a target object.
- Object.assign() can be used to clone an object or merge objects .
Popular Tutorials
Popular examples, reference materials, learn python interactively, javascript object methods.
- JavaScript assign()
- JavaScript create()
- JavaScript defineProperties()
- JavaScript defineProperty()
- JavaScript entries()
- JavaScript freeze()
- JavaScript fromEntries()
- JavaScript getOwnPropertyDescriptor()
- JavaScript getOwnPropertyDescriptors()
- JavaScript getOwnPropertyNames()
- JavaScript getOwnPropertySymbols()
- JavaScript getPrototypeOf()
- JavaScript hasOwnProperty()
- JavaScript is()
- JavaScript isExtensible()
- JavaScript isFrozen()
- JavaScript isPrototypeOf()
- JavaScript isSealed()
- JavaScript keys()
- JavaScript preventExtensions()
- JavaScript propertyIsEnumerable()
- JavaScript seal()
- JavaScript setPrototypeOf()
- JavaScript toLocaleString()
- JavaScript toString()
- JavaScript valueOf()
- JavaScript values()
JavaScript Tutorials
JavaScript Object.keys()
JavaScript Object.values()
JavaScript Object.getOwnPropertyNames()
JavaScript Object.is()
- Javascript Object.defineProperties()
- JavaScript Object.entries()
JavaScript Object.assign()
The Object.assign() method copies all the enumerable properties of the given objects to a single object and returns it.
assign() Syntax
The syntax of the assign() method is:
Here, assign() is a static method. Hence, we need to access the method using the class name, Object .
assign() Parameters
The assign() method takes in:
- target - the target object to which we will copy all the properties of the sources .
- sources - the source object(s) whose properties we want to copy.
assign() Return Value
The assign() method returns the target object.
Note: Properties in the target object are overwritten by properties in the sources if they have the same key.
Example 1: Javascript Object.assign() to Clone Objects
In the above example, we have used the assign() method to assign the contents of obj to newObject .
Since assign() modifies the target object and returns the same object, both copy and newObject are clones of one another. As a result, we get identical outputs when we print them both.
Example 2: assign() to Merge Objects
In the above example, we have used assign() to merge the objects o1 , o2 , and o3 into a new object o4 .
Using the empty object {} as a target object ensures that the properties of the other objects are copied to a new object without modifying any of the source objects.
As can be seen from the output, properties of later objects overwrite that of earlier ones. For example,
- the b key from o1 is overwritten by its counterpart in o2 .
- the c keys from o1 and o2 are overwritten by their counterpart in o3 .
Note: If the source value is a reference to an object, it only copies the reference value.
Sorry about that.
Related Functions
JavaScript Library
- Skip to main content
- Select language
- Skip to search
- Object.assign()
Return value
Copying accessors.
The Object.assign() method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object.
The target object.
Description
Properties in the target object will be overwritten by properties in the sources if they have the same key. Later sources' properties will similarly overwrite earlier ones.
The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties versus just copying or defining new properties. This may make it unsuitable for merging new properties into a prototype if the merge sources contain getters. For copying property definitions, including their enumerability, into prototypes Object.getOwnPropertyDescriptor() and Object.defineProperty() should be used instead.
Both String and Symbol properties are copied.
In case of an error, for example if a property is non-writable, a TypeError will be raised, and the target object can be changed if any properties are added before error is raised.
Note that Object.assign() does not throw on null or undefined source values.
Cloning an object
Warning for deep clone.
For deep cloning, we need to use other alternatives because Object.assign() copies property values. If the source value is a reference to an object, it only copies that reference value.
Merging objects
Merging objects with same properties.
The properties are overwritten by other objects that have the same properties later in the parameters order.
Copying symbol-typed properties
Properties on the prototype chain and non-enumerable properties cannot be copied, primitives will be wrapped to objects, exceptions will interrupt the ongoing copying task.
This polyfill doesn't support symbol properties, since ES5 doesn't have symbols anyway:
Specifications
Browser compatibility.
- Object.defineProperties()
- Enumerability and ownership of properties
Document Tags and Contributors
- ECMAScript 2015
- Standard built-in objects
- Object.prototype
- Object.prototype.__count__
- Object.prototype.__noSuchMethod__
- Object.prototype.__parent__
- Object.prototype.__proto__
- Object.prototype.constructor
- Object.create()
- Object.defineProperty()
- Object.entries()
- Object.freeze()
- Object.getNotifier()
- Object.getOwnPropertyDescriptor()
- Object.getOwnPropertyDescriptors()
- Object.getOwnPropertyNames()
- Object.getOwnPropertySymbols()
- Object.getPrototypeOf()
- Object.is()
- Object.isExtensible()
- Object.isFrozen()
- Object.isSealed()
- Object.keys()
- Object.observe()
- Object.preventExtensions()
- Object.prototype.__defineGetter__()
- Object.prototype.__defineSetter__()
- Object.prototype.__lookupGetter__()
- Object.prototype.__lookupSetter__()
- Object.prototype.eval()
- Object.prototype.hasOwnProperty()
- Object.prototype.isPrototypeOf()
- Object.prototype.propertyIsEnumerable()
- Object.prototype.toLocaleString()
- Object.prototype.toSource()
- Object.prototype.toString()
- Object.prototype.unwatch()
- Object.prototype.valueOf()
- Object.prototype.watch()
- Object.seal()
- Object.setPrototypeOf()
- Object.unobserve()
- Object.values()
- Inheritance:
- Function.arguments
- Function.arity
- Function.caller
- Function.displayName
- Function.length
- Function.name
- Function.prototype
- Function.prototype.apply()
- Function.prototype.bind()
- Function.prototype.call()
- Function.prototype.isGenerator()
- Function.prototype.toSource()
- Function.prototype.toString()
Contact Mentor
Projects + Tutorials on React JS & JavaScript
5 ways to Add a Property to object in JavaScript
To demonstrate adding new properties to JavaScript object, we will consider the following example of an employee object:
There are multiple instances to consider, which are as follows:
- Static property names : Addition of “id” property to an employee object.
- Dynamic property names : Include property based on the value of “customProp” variable.
- Adding properties from another object : Add location property from a person object to an employee object.
1. “object.property_name” syntax
The dot notation is the simplest way to access/modify the properties of a JavaScript object. A new property can be initialized by the following syntax:
In the below example, we are creating an “id” property with the value 130 for the employee object.
Further, adding properties for nested objects follows a similar syntax:
Below, the “country” property is added to a nested object which is the value of the “location” parent property in the employee object.
This approach is most ideal for the following instances:
- The property name is a static value and needs to be initialized manually.
- Property names don’t include special characters like extra space, etc.
2. Access property though “object[‘property_name’]”
The syntax based on the square brackets is an alternative approach with similar capabilities and avoids most of the disadvantages of the dot operator. The syntax for adding new property using square brackets is as follows:
Square bracket syntax offers the following advantages over traditional dot operator:
- When the property name is based on a dynamic variable . For example, the property name is retrieved from API calls, user inputs, etc.
- The property name string value contains special characters like extra space, etc.
In the below example, the “Last name” property cannot be directly added using dot operator syntax due to the presence of space characters . Hence, we are able to accomplish the creation of “Last name” property using square bracket syntax.
The nested objects are accessed using a series of multiple square bracket syntax or alternatively, a combination of dot operator and square brackets can also be used.
3. Create new property using Object.defineProperty()
JavaScript Object class also provides native “ defineProperty() ” method to define new properties for an object. In addition to specifying the value of the new property, “ defineProperty() ” also allow configuring the behavior for the property .
The generic syntax for “defineProperty()” is as follows:
In the below example, we define “id” property for the employee object with a value of 130 and writable as false . Hence, subsequent changes in the value of the id property are discarded. Read more about Object.defineProperty from developer.mozilla.org/Object/defineProperty .
4. Spread operator syntax “{ …object, property_name: property_value } “
The spread operator allows the creation of a soft copy of an object with existing properties. The Spread operator followed by an inline property definition allows the addition of new properties. Further, the properties of another object can be added by using the spread operator multiple times.
In the below example, we create a copy of the employee object combined with location and id properties. Next line, “id” object is added to employee object using the spread operator .
5. Assign properties using Object.assign()
The “Object.assign()” method allows properties of the source object to be added to the target object . By defining all the new properties which need to be added to the source object, we achieve the addition of properties to a target object.
In the below example, we add the “id” property with value 670 to the employee object using Object.assign.
Finally, to conclude the summary of the methods to add properties to objects is as follows:
- Dot method syntax is ideal for static property values.
- Square bracket syntax works best for dynamic values from external API, user input, etc.
- Object.defineProperty() is used when the property’s writability, getter, setters, etc needs to be configured.
- In object/array formatter functions and when properties from another object are included, the spread operator and Object.assign() are more ideal.
Related Articles
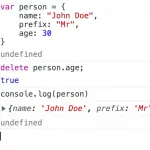
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript object properties.
Properties are the most important part of any JavaScript object.
JavaScript Properties
Properties are the values associated with a JavaScript object.
A JavaScript object is a collection of unordered properties.
Properties can usually be changed, added, and deleted, but some are read only.
Accessing JavaScript Properties
The syntax for accessing the property of an object is:
The expression must evaluate to a property name.
Advertisement
JavaScript for...in Loop
The JavaScript for...in statement loops through the properties of an object.
The block of code inside of the for...in loop will be executed once for each property.
Looping through the properties of an object:
Adding New Properties
You can add new properties to an existing object by simply giving it a value.
Assume that the person object already exists - you can then give it new properties:
Deleting Properties
The delete keyword deletes a property from an object:
or delete person["age"];
The delete keyword deletes both the value of the property and the property itself.
After deletion, the property cannot be used before it is added back again.
The delete operator is designed to be used on object properties. It has no effect on variables or functions.
Nested Objects
Values in an object can be another object:
You can access nested objects using the dot notation or the bracket notation:
Nested Arrays and Objects
Values in objects can be arrays, and values in arrays can be objects:
To access arrays inside arrays, use a for-in loop for each array:
Property Attributes
All properties have a name. In addition they also have a value.
The value is one of the property's attributes.
Other attributes are: enumerable, configurable, and writable.
These attributes define how the property can be accessed (is it readable?, is it writable?)
In JavaScript, all attributes can be read, but only the value attribute can be changed (and only if the property is writable).
( ECMAScript 5 has methods for both getting and setting all property attributes)
Prototype Properties
JavaScript objects inherit the properties of their prototype.
The delete keyword does not delete inherited properties, but if you delete a prototype property, it will affect all objects inherited from the prototype.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

11 Properties: assignment vs. definition
- 11.1.1 Assignment
- 11.1.2 Definition
- 11.2.1 Assigning to a property
- 11.2.2 Defining a property
- 11.3.1 Only definition allows us to create a property with arbitrary attributes
- 11.3.2 The assignment operator does not change properties in prototypes
- 11.3.3 Assignment calls setters, definition doesn’t
- 11.3.4 Inherited read-only properties prevent creating own properties via assignment
- 11.4.1 The properties of an object literal are added via definition
- 11.4.2 The assignment operator = always uses assignment
- 11.4.3 Public class fields are added via definition
- 11.5 Further reading and sources of this chapter
There are two ways of creating or changing a property prop of an object obj :
- Assigning : obj.prop = true
- Defining : Object.defineProperty(obj, '', {value: true})
This chapter explains how they work.
For this chapter, you should be familiar with property attributes and property descriptors. If you aren’t, check out §9 “Property attributes: an introduction” .
11.1 Assignment vs. definition
11.1.1 assignment.
We use the assignment operator = to assign a value value to a property .prop of an object obj :
This operator works differently depending on what .prop looks like:
Changing properties: If there is an own data property .prop , assignment changes its value to value .
Invoking setters: If there is an own or inherited setter for .prop , assignment invokes that setter.
Creating properties: If there is no own data property .prop and no own or inherited setter for it, assignment creates a new own data property.
That is, the main purpose of assignment is making changes. That’s why it supports setters.
11.1.2 Definition
To define a property with the key propKey of an object obj , we use an operation such as the following method:
This method works differently depending on what the property looks like:
- Changing properties: If an own property with key propKey exists, defining changes its property attributes as specified by the property descriptor propDesc (if possible).
- Creating properties: Otherwise, defining creates an own property with the attributes specified by propDesc (if possible).
That is, the main purpose of definition is to create an own property (even if there is an inherited setter, which it ignores) and to change property attributes.
11.2 Assignment and definition in theory (optional)
In specification operations, property descriptors are not JavaScript objects but Records , a spec-internal data structure that has fields . The keys of fields are written in double brackets. For example, Desc.[[Configurable]] accesses the field .[[Configurable]] of Desc . These records are translated to and from JavaScript objects when interacting with the outside world.
11.2.1 Assigning to a property
The actual work of assigning to a property is handled via the following operation in the ECMAScript specification:
These are the parameters:
- O is the object that is currently being visited.
- P is the key of the property that we are assigning to.
- V is the value we are assigning.
- Receiver is the object where the assignment started.
- ownDesc is the descriptor of O[P] or null if that property doesn’t exist.
The return value is a boolean that indicates whether or not the operation succeeded. As explained later in this chapter , strict-mode assignment throws a TypeError if OrdinarySetWithOwnDescriptor() fails.
This is a high-level summary of the algorithm:
- It traverses the prototype chain of Receiver until it finds a property whose key is P . The traversal is done by calling OrdinarySetWithOwnDescriptor() recursively. During recursion, O changes and points to the object that is currently being visited, but Receiver stays the same.
- Depending on what the traversal finds, an own property is created in Receiver (where recursion started) or something else happens.
In more detail, this algorithm works as follows:
If O has a prototype parent , then we return parent.[[Set]](P, V, Receiver) . This continues our search. The method call usually ends up invoking OrdinarySetWithOwnDescriptor() recursively.
Otherwise, our search for P has failed and we set ownDesc as follows:
With this ownDesc , the next if statement will create an own property in Receiver .
- If ownDesc.[[Writable]] is false , return false . This means that any non-writable property P (own or inherited!) prevents assignment.
- The current object O and the current property descriptor ownDesc on one hand.
- The original object Receiver and the original property descriptor existingDescriptor on the other hand.
- (If we get here, then we are still at the beginning of the prototype chain – we only recurse if Receiver does not have a property P .)
- If existingDescriptor specifies an accessor, return false .
- If existingDescriptor.[[Writable]] is false , return false .
- Return Receiver.[[DefineOwnProperty]](P, { [[Value]]: V }) . This internal method performs definition, which we use to change the value of property Receiver[P] . The definition algorithm is described in the next subsection.
- (If we get here, then Receiver does not have an own property with key P .)
- Return CreateDataProperty(Receiver, P, V) . ( This operation creates an own data property in its first argument.)
- (If we get here, then ownDesc describes an accessor property that is own or inherited.)
- Let setter be ownDesc.[[Set]] .
- If setter is undefined , return false .
- Perform Call(setter, Receiver, «V») . Call() invokes the function object setter with this set to Receiver and the single parameter V (French quotes «» are used for lists in the specification).
Return true .
11.2.1.1 How do we get from an assignment to OrdinarySetWithOwnDescriptor() ?
Evaluating an assignment without destructuring involves the following steps:
- In the spec, evaluation starts in the section on the runtime semantics of AssignmentExpression . This section handles providing names for anonymous functions, destructuring, and more.
- If there is no destructuring pattern, then PutValue() is used to make the assignment.
- For property assignments, PutValue() invokes the internal method .[[Set]]() .
- For ordinary objects, .[[Set]]() calls OrdinarySet() (which calls OrdinarySetWithOwnDescriptor() ) and returns the result.
Notably, PutValue() throws a TypeError in strict mode if the result of .[[Set]]() is false .
11.2.2 Defining a property
The actual work of defining a property is handled via the following operation in the ECMAScript specification:
The parameters are:
- The object O where we want to define a property. There is a special validation-only mode where O is undefined . We are ignoring this mode here.
- The property key P of the property we want to define.
- extensible indicates if O is extensible.
- Desc is a property descriptor specifying the attributes we want the property to have.
- current contains the property descriptor of an own property O[P] if it exists. Otherwise, current is undefined .
The result of the operation is a boolean that indicates if it succeeded. Failure can have different consequences. Some callers ignore the result. Others, such as Object.defineProperty() , throw an exception if the result is false .
This is a summary of the algorithm:
If current is undefined , then property P does not currently exist and must be created.
- If extensible is false , return false indicating that the property could not be added.
- Otherwise, check Desc and create either a data property or an accessor property.
If Desc doesn’t have any fields, return true indicating that the operation succeeded (because no changes had to be made).
If current.[[Configurable]] is false :
- ( Desc is not allowed to change attributes other than value .)
- If Desc.[[Configurable]] exists, it must have the same value as current.[[Configurable]] . If not, return false .
- Same check: Desc.[[Enumerable]]
Next, we validate the property descriptor Desc : Can the attributes described by current be changed to the values specified by Desc ? If not, return false . If yes, go on.
- If the descriptor is generic (with no attributes specific to data properties or accessor properties), then validation is successful and we can move on.
- The current property must be configurable (otherwise its attributes can’t be changed as necessary). If not, false is returned.
- Change the current property from a data property to an accessor property or vice versa. When doing so, the values of .[[Configurable]] and .[[Enumerable]] are preserved, all other attributes get default values ( undefined for object-valued attributes, false for boolean-valued attributes).
- (Due to current.[[Configurable]] being false , Desc.[[Configurable]] and Desc.[[Enumerable]] were already checked previously and have the correct values.)
- If Desc.[[Writable]] exists and is true , then return false .
- If Desc.[[Value]] exists and does not have the same value as current.[[Value]] , then return false .
- There is nothing more to do. Return true indicating that the algorithm succeeded.
- (Note that normally, we can’t change any attributes of a non-configurable property other than its value. The one exception to this rule is that we can always go from writable to non-writable. This algorithm handles this exception correctly.)
- If Desc.[[Set]] exists, it must have the same value as current.[[Set]] . If not, return false .
- Same check: Desc.[[Get]]
Set the attributes of the property with key P to the values specified by Desc . Due to validation, we can be sure that all of the changes are allowed.
11.3 Definition and assignment in practice
This section describes some consequences of how property definition and assignment work.
11.3.1 Only definition allows us to create a property with arbitrary attributes
If we create an own property via assignment, it always creates properties whose attributes writable , enumerable , and configurable are all true .
Therefore, if we want to specify arbitrary attributes, we must use definition.
And while we can create getters and setters inside object literals, we can’t add them later via assignment. Here, too, we need definition.
11.3.2 The assignment operator does not change properties in prototypes
Let us consider the following setup, where obj inherits the property prop from proto .
We can’t (destructively) change proto.prop by assigning to obj.prop . Doing so creates a new own property:
The rationale for this behavior is as follows: Prototypes can have properties whose values are shared by all of their descendants. If we want to change such a property in only one descendant, we must do so non-destructively, via overriding. Then the change does not affect the other descendants.
11.3.3 Assignment calls setters, definition doesn’t
What is the difference between defining the property .prop of obj versus assigning to it?
If we define, then our intention is to either create or change an own (non-inherited) property of obj . Therefore, definition ignores the inherited setter for .prop in the following example:
If, instead, we assign to .prop , then our intention is often to change something that already exists and that change should be handled by the setter:
11.3.4 Inherited read-only properties prevent creating own properties via assignment
What happens if .prop is read-only in a prototype?
In any object that inherits the read-only .prop from proto , we can’t use assignment to create an own property with the same key – for example:
Why can’t we assign? The rationale is that overriding an inherited property by creating an own property can be seen as non-destructively changing the inherited property. Arguably, if a property is non-writable, we shouldn’t be able to do that.
However, defining .prop still works and lets us override:
Accessor properties that don’t have a setter are also considered to be read-only:
The fact that read-only properties prevent assignment earlier in the prototype chain, has been given the name override mistake :
- It was introduced in ECMAScript 5.1.
- On one hand, this behavior is consistent with how prototypal inheritance and setters work. (So, arguably, it is not a mistake.)
- On the other hand, with the behavior, deep-freezing the global object causes unwanted side-effects.
- There was an attempt to change the behavior, but that broke the library Lodash and was abandoned ( pull request on GitHub ).
- Pull request on GitHub
- Wiki page on ECMAScript.org ( archived )
11.4 Which language constructs use definition, which assignment?
In this section, we examine where the language uses definition and where it uses assignment. We detect which operation is used by tracking whether or not inherited setters are called. See §11.3.3 “Assignment calls setters, definition doesn’t” for more information.
11.4.1 The properties of an object literal are added via definition
When we create properties via an object literal, JavaScript always uses definition (and therefore never calls inherited setters):
11.4.2 The assignment operator = always uses assignment
The assignment operator = always uses assignment to create or change properties.
11.4.3 Public class fields are added via definition
Alas, even though public class fields have the same syntax as assignment, they do not use assignment to create properties, they use definition (like properties in object literals):
Destructuring assignment
The two most used data structures in JavaScript are Object and Array .
- Objects allow us to create a single entity that stores data items by key.
- Arrays allow us to gather data items into an ordered list.
However, when we pass these to a function, we may not need all of it. The function might only require certain elements or properties.
Destructuring assignment is a special syntax that allows us to “unpack” arrays or objects into a bunch of variables, as sometimes that’s more convenient.
Destructuring also works well with complex functions that have a lot of parameters, default values, and so on. Soon we’ll see that.
Array destructuring
Here’s an example of how an array is destructured into variables:
Now we can work with variables instead of array members.
It looks great when combined with split or other array-returning methods:
As you can see, the syntax is simple. There are several peculiar details though. Let’s see more examples to understand it better.
It’s called “destructuring assignment,” because it “destructurizes” by copying items into variables. However, the array itself is not modified.
It’s just a shorter way to write:
Unwanted elements of the array can also be thrown away via an extra comma:
In the code above, the second element of the array is skipped, the third one is assigned to title , and the rest of the array items are also skipped (as there are no variables for them).
…Actually, we can use it with any iterable, not only arrays:
That works, because internally a destructuring assignment works by iterating over the right value. It’s a kind of syntax sugar for calling for..of over the value to the right of = and assigning the values.
We can use any “assignables” on the left side.
For instance, an object property:
In the previous chapter, we saw the Object.entries(obj) method.
We can use it with destructuring to loop over the keys-and-values of an object:
The similar code for a Map is simpler, as it’s iterable:
There’s a well-known trick for swapping values of two variables using a destructuring assignment:
Here we create a temporary array of two variables and immediately destructure it in swapped order.
We can swap more than two variables this way.
The rest ‘…’
Usually, if the array is longer than the list at the left, the “extra” items are omitted.
For example, here only two items are taken, and the rest is just ignored:
If we’d like also to gather all that follows – we can add one more parameter that gets “the rest” using three dots "..." :
The value of rest is the array of the remaining array elements.
We can use any other variable name in place of rest , just make sure it has three dots before it and goes last in the destructuring assignment.
Default values
If the array is shorter than the list of variables on the left, there will be no errors. Absent values are considered undefined:
If we want a “default” value to replace the missing one, we can provide it using = :
Default values can be more complex expressions or even function calls. They are evaluated only if the value is not provided.
For instance, here we use the prompt function for two defaults:
Please note: the prompt will run only for the missing value ( surname ).
Object destructuring
The destructuring assignment also works with objects.
The basic syntax is:
We should have an existing object on the right side, that we want to split into variables. The left side contains an object-like “pattern” for corresponding properties. In the simplest case, that’s a list of variable names in {...} .
For instance:
Properties options.title , options.width and options.height are assigned to the corresponding variables.
The order does not matter. This works too:
The pattern on the left side may be more complex and specify the mapping between properties and variables.
If we want to assign a property to a variable with another name, for instance, make options.width go into the variable named w , then we can set the variable name using a colon:
The colon shows “what : goes where”. In the example above the property width goes to w , property height goes to h , and title is assigned to the same name.
For potentially missing properties we can set default values using "=" , like this:
Just like with arrays or function parameters, default values can be any expressions or even function calls. They will be evaluated if the value is not provided.
In the code below prompt asks for width , but not for title :
We also can combine both the colon and equality:
If we have a complex object with many properties, we can extract only what we need:
The rest pattern “…”
What if the object has more properties than we have variables? Can we take some and then assign the “rest” somewhere?
We can use the rest pattern, just like we did with arrays. It’s not supported by some older browsers (IE, use Babel to polyfill it), but works in modern ones.
It looks like this:
In the examples above variables were declared right in the assignment: let {…} = {…} . Of course, we could use existing variables too, without let . But there’s a catch.
This won’t work:
The problem is that JavaScript treats {...} in the main code flow (not inside another expression) as a code block. Such code blocks can be used to group statements, like this:
So here JavaScript assumes that we have a code block, that’s why there’s an error. We want destructuring instead.
To show JavaScript that it’s not a code block, we can wrap the expression in parentheses (...) :
Nested destructuring
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions.
In the code below options has another object in the property size and an array in the property items . The pattern on the left side of the assignment has the same structure to extract values from them:
All properties of options object except extra that is absent in the left part, are assigned to corresponding variables:
Finally, we have width , height , item1 , item2 and title from the default value.
Note that there are no variables for size and items , as we take their content instead.
Smart function parameters
There are times when a function has many parameters, most of which are optional. That’s especially true for user interfaces. Imagine a function that creates a menu. It may have a width, a height, a title, items list and so on.
Here’s a bad way to write such a function:
In real-life, the problem is how to remember the order of arguments. Usually IDEs try to help us, especially if the code is well-documented, but still… Another problem is how to call a function when most parameters are ok by default.
That’s ugly. And becomes unreadable when we deal with more parameters.
Destructuring comes to the rescue!
We can pass parameters as an object, and the function immediately destructurizes them into variables:
We can also use more complex destructuring with nested objects and colon mappings:
The full syntax is the same as for a destructuring assignment:
Then, for an object of parameters, there will be a variable varName for property incomingProperty , with defaultValue by default.
Please note that such destructuring assumes that showMenu() does have an argument. If we want all values by default, then we should specify an empty object:
We can fix this by making {} the default value for the whole object of parameters:
In the code above, the whole arguments object is {} by default, so there’s always something to destructurize.
Destructuring assignment allows for instantly mapping an object or array onto many variables.
The full object syntax:
This means that property prop should go into the variable varName and, if no such property exists, then the default value should be used.
Object properties that have no mapping are copied to the rest object.
The full array syntax:
The first item goes to item1 ; the second goes into item2 , all the rest makes the array rest .
It’s possible to extract data from nested arrays/objects, for that the left side must have the same structure as the right one.
We have an object:
Write the destructuring assignment that reads:
- name property into the variable name .
- years property into the variable age .
- isAdmin property into the variable isAdmin (false, if no such property)
Here’s an example of the values after your assignment:
The maximal salary
There is a salaries object:
Create the function topSalary(salaries) that returns the name of the top-paid person.
- If salaries is empty, it should return null .
- If there are multiple top-paid persons, return any of them.
P.S. Use Object.entries and destructuring to iterate over key/value pairs.
Open a sandbox with tests.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- Method Chaining in JavaScript
- Functional Programming in JavaScript
- PugJS Basic Tags
- Phases of JavaScript Event
- How to remove CSS style of <style> tag using JavaScript/jQuery ?
- How to validate email address using RegExp in JavaScript ?
- How to zoom-in and zoom-out image using JavaScript ?
- How to replace plain URL with link using JavaScript ?
- How to determine which element the mouse pointer move over using JavaScript ?
- How to convert list of elements in an array using jQuery ?
- Service Workers in Javascript
- How to remove portion of a string after certain character in JavaScript ?
- How to check if string contains only digits in JavaScript ?
- How to sort a list alphabetically using jQuery ?
- How to print the content of an object in JavaScript ?
- Introduction to KnockoutJS
- Sound generation on clicking the button using JavaScript
- JavaScript | WebAPI | File | File.size Property
- JavaScript | WebAPI | File | File.type Property
JavaScript Program to Implement Own Object Assign Method
Let’s create a custom function called assign that does exactly what Object.assign() does. It will take one or more objects as sources and a target object. Then, it will copy all the properties we can loop over (called enumerable properties) from the source objects to the target object. If a property already exists in the target with the same name, its value will be overwritten.
These are the following methods to Implement Own Object Assign Method:
Table of Content
Using Basic Looping
Using object.keys and foreach.
This method iterates through the source objects using a for loop and uses a conditional statement to determine whether the property is already present in the target object before assigning it.
Example: The keyword is assigned. The assign function is used to merge properties from multiple source objects into a single target object.
This method retrieves all of the keys from the source objects using Object.keys, then loops through them using forEach. After that, bracket notation is used to access dynamic properties during assignment.
Example: JavaScript’s `assign` function merges properties from multiple source objects into a target object, exemplified by the code snippet merging `obj1` and `obj2`.
Please Login to comment...
Similar reads.
- JavaScript Programs
- Web Technologies
- Otter.ai vs. Fireflies.ai: Which AI Transcribes Meetings More Accurately?
- Google Chrome Will Soon Let You Talk to Gemini In The Address Bar
- AI Interior Designer vs. Virtual Home Decorator: Which AI Can Transform Your Home Into a Pinterest Dream Faster?
- Top 10 Free Webclipper on Chrome Browser in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
How to Check If an Object Has a Property in JavaScript
There are several methods to check if an object has a property in JavaScript, including the in operator, object.hasOwn() method, checking for undefined value and more.

When we need to check if an object has a property in JavaScript , we have several methods at our disposal. This includes JavaScript operators, specific static methods from the object class, object instance method, array instance method and a custom JavaScript function.
JavaScript: Check if an Object Has a Property Methods
- In operator
- Object.prototype.hasOwnProperty() method
- Object.hasOwn() method
- Check for undefined value
- Object.keys() and Array.prototype.some method
- Custom JavaScript util function.
Let’s take a look at each one with examples below.
6 Ways to Check If an Object Has a Property in JavaScript
1. in operator.
The in operator returns true if the specified property is present inside the object or inside its prototype chain. The operator also works for the objects created with Object.create() static method.
More on JavaScript 5 Things to Know About the JavaScript Delete Operator
2. Object.prototype.hasOwnProperty() method
We can also use the object instance method hasOwnProperty() to check if an object contains a specific property .
Although Object.prototype.hasOwnProperty() has been in JavaScript for quite a time, it has some drawbacks. hasOwnProperty() doesn’t work for objects created using Object.create(null) because it doesn’t inherit from Object.prototype , which makes the hasOwnProperty() unreachable for the created object. Using hasOwnProperty() , the object will throw an exception.
3. Object.hasOwn() method
As part of the ECMAScript 2022 version, we can use a static hasOwn() method inside the Object class. Object.hasOwn() is recommended over hasOwnProperty() in browsers where it is supported. Object.hasOwn() is the intended alternative for the Object.prototype.hasOwnProperty() method.
4. Check for Undefined Value
When we try to access the non-existent property of an object, then we have undefined value as the result. So, we can use this approach and do something only when property value is not undefined.
5. Object.keys() and Array.prototype.some() methods
This approach involves using Object.keys() and Array.prototype.some() methods.
We’re converting the object to the array of properties, and then we have a method with a predicate function where we are checking the presence of the target property name. This method has the same drawback as the Object.prototype.hasOwnProperty() because we can’t find the object property if the object is created with Object.create() method.
6. Custom JavaScript util function
This hasKey() function accepts the object and target property name arguments. If both arguments are defined, then we have a for-in loop through the object, and inside each iteration, we have a check if the current property key is equal to the target one (input parameter).
More on JavaScript How to Find Values in a JavaScript Array
Best Method to Check If a Property Exists in JavaScript
In general, being proficient with JavaScript objects is essential in web development.
As you can see, we have a lot of options we can use to check the presence of the property inside the specific JavaScript object. From my experience, the “in” operator and Object.hasOwn() method are the best ones to use in everyday work. Also, if you don’t have ECMAScript 2022 version, you can also use the “in” operator or check for undefined value.
Recent Expert Contributors Articles
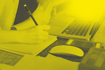

IMAGES
VIDEO
COMMENTS
The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties.
Here's what I came up with: var obj = (obj = {}, obj[field] = 123, obj) It looks a little bit complex at first, but it's really simple. We use the Comma Operator to run three commands in a row: obj = {}: creates a new object and assigns it to the variable obj. obj[field] = 123: adds a computed property name to obj.
The Object.assign() copies all enumerable and own properties from the source objects to the target object. It returns the target object. The Object.assign() invokes the getters on the source objects and setters on the target. It assigns properties only, not copying or defining new properties. Using JavaScript Object.assign() to clone an object
In the above example, we have used assign() to merge the objects o1, o2, and o3 into a new object o4. const o4 = Object.assign({}, o1, o2, o3); Using the empty object {} as a target object ensures that the properties of the other objects are copied to a new object without modifying any of the source objects. As can be seen from the output ...
Note that only an object's enumerable properties will be copied over with Object.assign. The first argument is the source object, and the subsequent arguments are source objects. You can pass-in multiple source objects, and duplicate properties in sources passed last will win:
A property has a key (also known as "name" or "identifier") before the colon ":" and a value to the right of it.. In the user object, there are two properties:. The first property has the name "name" and the value "John".; The second one has the name "age" and the value 30.; The resulting user object can be imagined as a cabinet with two signed files labeled "name" and "age".
Description. Properties in the target object will be overwritten by properties in the sources if they have the same key. Later sources' properties will similarly overwrite earlier ones. The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the ...
Property Assignment. Once we've defined an object, we're not stuck with all the properties we wrote. Objects are mutable meaning we can update them after we create them! We can use either dot notation, ., or bracket notation, [], and the assignment operator, = to add new key-value pairs to an object or change an existing property. One of ...
1. "object.property_name" syntax. The dot notation is the simplest way to access/modify the properties of a JavaScript object. A new property can be initialized by the following syntax: object.new_property = new_value. In the below example, we are creating an "id" property with the value 130 for the employee object. // Add new property.
JavaScript Properties. Properties are the values associated with a JavaScript object. A JavaScript object is a collection of unordered properties. Properties can usually be changed, added, and deleted, but some are read only.
11.1.1 Assignment #. We use the assignment operator = to assign a value value to a property .prop of an object obj: obj.prop = value. This operator works differently depending on what .prop looks like: Changing properties: If there is an own data property .prop, assignment changes its value to value. Invoking setters: If there is an own or ...
Introduction: In the dynamic world of JavaScript, the ability to precisely control object properties is a crucial skill for developers. The Object.defineProperty() method provides a powerful ...
Property assignment is frequently used to add new properties to an object. My post explained that that can cause problems. Hence, it is best to follow the simple rules: If you want to create a new property, use definition. If you want to change the value of a property, use assignment. - Maizere Pathak.Nepal.
If an object or an array contains other nested objects and arrays, we can use more complex left-side patterns to extract deeper portions. In the code below options has another object in the property size and an array in the property items. The pattern on the left side of the assignment has the same structure to extract values from them:
The Object.assign() method is used to copy the values of all enumerable own properties from one or more source objects to a target object. It will return the target object. You could assign the property value to a new object and return it. list.map(item => Object.assign({}, item, { href: customFunction(item.name) })); Notice that this might not ...
After that, bracket notation is used to access dynamic properties during assignment. Example: JavaScript's `assign` function merges properties from multiple source objects into a target object, exemplified by the code snippet merging `obj1` and `obj2`. JavaScript
We're converting the object to the array of properties, and then we have a method with a predicate function where we are checking the presence of the target property name. This method has the same drawback as the Object.prototype.hasOwnProperty() because we can't find the object property if the object is created with Object.create() method.
Explanations: Short-circuit evaluation ( true && {}, false && {}) would return an Object or a Boolean false value. In the case an Object is returned, its properties get spread and assigned to the parent object. In the case false value is returned, the parent object isn't polluted, because ES6 treats false, undefined, null and etc values as {}.
Here, "global objects" refer to objects in the global scope. The global object itself can be accessed using the this operator in the global scope. In fact, the global scope consists of the properties of the global object, including inherited properties, if any. Other objects in the global scope are either created by the user script or provided ...
Problem: In fact you are assigning the content of second with undefined, because at the time you are trying to refer the first property, at the assignement time, it doesn't exist yet in your container object.. Solution: You need either to store the content of first property in an object before the assignement or create your container object with only first property and then define container ...