10 Python Practice Exercises for Beginners With Detailed Solutions

- python basics
- get started with python
- online practice
A great way to improve quickly at programming with Python is to practice with a wide range of exercises and programming challenges. In this article, we give you 10 Python practice exercises to boost your skills.
Practice exercises are a great way to learn Python. Well-designed exercises expose you to new concepts, such as writing different types of loops, working with different data structures like lists, arrays, and tuples, and reading in different file types. Good exercises should be at a level that is approachable for beginners but also hard enough to challenge you, pushing your knowledge and skills to the next level.
If you’re new to Python and looking for a structured way to improve your programming, consider taking the Python Basics Practice course. It includes 17 interactive exercises designed to improve all aspects of your programming and get you into good programming habits early. Read about the course in the March 2023 episode of our series Python Course of the Month .
Take the course Python Practice: Word Games , and you gain experience working with string functions and text files through its 27 interactive exercises. Its release announcement gives you more information and a feel for how it works.
Each course has enough material to keep you busy for about 10 hours. To give you a little taste of what these courses teach you, we have selected 10 Python practice exercises straight from these courses. We’ll give you the exercises and solutions with detailed explanations about how they work.
To get the most out of this article, have a go at solving the problems before reading the solutions. Some of these practice exercises have a few possible solutions, so also try to come up with an alternative solution after you’ve gone through each exercise.
Let’s get started!

Exercise 1: User Input and Conditional Statements
Write a program that asks the user for a number then prints the following sentence that number of times: ‘I am back to check on my skills!’ If the number is greater than 10, print this sentence instead: ‘Python conditions and loops are a piece of cake.’ Assume you can only pass positive integers.
Here, we start by using the built-in function input() , which accepts user input from the keyboard. The first argument is the prompt displayed on the screen; the input is converted into an integer with int() and saved as the variable number. If the variable number is greater than 10, the first message is printed once on the screen. If not, the second message is printed in a loop number times.
Exercise 2: Lowercase and Uppercase Characters
Below is a string, text . It contains a long string of characters. Your task is to iterate over the characters of the string, count uppercase letters and lowercase letters, and print the result:
We start this one by initializing the two counters for uppercase and lowercase characters. Then, we loop through every letter in text and check if it is lowercase. If so, we increment the lowercase counter by one. If not, we check if it is uppercase and if so, we increment the uppercase counter by one. Finally, we print the results in the required format.
Exercise 3: Building Triangles
Create a function named is_triangle_possible() that accepts three positive numbers. It should return True if it is possible to create a triangle from line segments of given lengths and False otherwise. With 3 numbers, it is sometimes, but not always, possible to create a triangle: You cannot create a triangle from a = 13, b = 2, and c = 3, but you can from a = 13, b = 9, and c = 10.
The key to solving this problem is to determine when three lines make a triangle regardless of the type of triangle. It may be helpful to start drawing triangles before you start coding anything.

Notice that the sum of any two sides must be larger than the third side to form a triangle. That means we need a + b > c, c + b > a, and a + c > b. All three conditions must be met to form a triangle; hence we need the and condition in the solution. Once you have this insight, the solution is easy!
Exercise 4: Call a Function From Another Function
Create two functions: print_five_times() and speak() . The function print_five_times() should accept one parameter (called sentence) and print it five times. The function speak(sentence, repeat) should have two parameters: sentence (a string of letters), and repeat (a Boolean with a default value set to False ). If the repeat parameter is set to False , the function should just print a sentence once. If the repeat parameter is set to True, the function should call the print_five_times() function.
This is a good example of calling a function in another function. It is something you’ll do often in your programming career. It is also a nice demonstration of how to use a Boolean flag to control the flow of your program.
If the repeat parameter is True, the print_five_times() function is called, which prints the sentence parameter 5 times in a loop. Otherwise, the sentence parameter is just printed once. Note that in Python, writing if repeat is equivalent to if repeat == True .
Exercise 5: Looping and Conditional Statements
Write a function called find_greater_than() that takes two parameters: a list of numbers and an integer threshold. The function should create a new list containing all numbers in the input list greater than the given threshold. The order of numbers in the result list should be the same as in the input list. For example:
Here, we start by defining an empty list to store our results. Then, we loop through all elements in the input list and test if the element is greater than the threshold. If so, we append the element to the new list.
Notice that we do not explicitly need an else and pass to do nothing when integer is not greater than threshold . You may include this if you like.
Exercise 6: Nested Loops and Conditional Statements
Write a function called find_censored_words() that accepts a list of strings and a list of special characters as its arguments, and prints all censored words from it one by one in separate lines. A word is considered censored if it has at least one character from the special_chars list. Use the word_list variable to test your function. We've prepared the two lists for you:
This is another nice example of looping through a list and testing a condition. We start by looping through every word in word_list . Then, we loop through every character in the current word and check if the current character is in the special_chars list.
This time, however, we have a break statement. This exits the inner loop as soon as we detect one special character since it does not matter if we have one or several special characters in the word.
Exercise 7: Lists and Tuples
Create a function find_short_long_word(words_list) . The function should return a tuple of the shortest word in the list and the longest word in the list (in that order). If there are multiple words that qualify as the shortest word, return the first shortest word in the list. And if there are multiple words that qualify as the longest word, return the last longest word in the list. For example, for the following list:
the function should return
Assume the input list is non-empty.
The key to this problem is to start with a “guess” for the shortest and longest words. We do this by creating variables shortest_word and longest_word and setting both to be the first word in the input list.
We loop through the words in the input list and check if the current word is shorter than our initial “guess.” If so, we update the shortest_word variable. If not, we check to see if it is longer than or equal to our initial “guess” for the longest word, and if so, we update the longest_word variable. Having the >= condition ensures the longest word is the last longest word. Finally, we return the shortest and longest words in a tuple.
Exercise 8: Dictionaries
As you see, we've prepared the test_results variable for you. Your task is to iterate over the values of the dictionary and print all names of people who received less than 45 points.
Here, we have an example of how to iterate through a dictionary. Dictionaries are useful data structures that allow you to create a key (the names of the students) and attach a value to it (their test results). Dictionaries have the dictionary.items() method, which returns an object with each key:value pair in a tuple.
The solution shows how to loop through this object and assign a key and a value to two variables. Then, we test whether the value variable is greater than 45. If so, we print the key variable.
Exercise 9: More Dictionaries
Write a function called consonant_vowels_count(frequencies_dictionary, vowels) that takes a dictionary and a list of vowels as arguments. The keys of the dictionary are letters and the values are their frequencies. The function should print the total number of consonants and the total number of vowels in the following format:
For example, for input:
the output should be:
Working with dictionaries is an important skill. So, here’s another exercise that requires you to iterate through dictionary items.
We start by defining a list of vowels. Next, we need to define two counters, one for vowels and one for consonants, both set to zero. Then, we iterate through the input dictionary items and test whether the key is in the vowels list. If so, we increase the vowels counter by one, if not, we increase the consonants counter by one. Finally, we print out the results in the required format.
Exercise 10: String Encryption
Implement the Caesar cipher . This is a simple encryption technique that substitutes every letter in a word with another letter from some fixed number of positions down the alphabet.
For example, consider the string 'word' . If we shift every letter down one position in the alphabet, we have 'xpse' . Shifting by 2 positions gives the string 'yqtf' . Start by defining a string with every letter in the alphabet:
Name your function cipher(word, shift) , which accepts a string to encrypt, and an integer number of positions in the alphabet by which to shift every letter.
This exercise is taken from the Word Games course. We have our string containing all lowercase letters, from which we create a shifted alphabet using a clever little string-slicing technique. Next, we create an empty string to store our encrypted word. Then, we loop through every letter in the word and find its index, or position, in the alphabet. Using this index, we get the corresponding shifted letter from the shifted alphabet string. This letter is added to the end of the new_word string.
This is just one approach to solving this problem, and it only works for lowercase words. Try inputting a word with an uppercase letter; you’ll get a ValueError . When you take the Word Games course, you slowly work up to a better solution step-by-step. This better solution takes advantage of two built-in functions chr() and ord() to make it simpler and more robust. The course contains three similar games, with each game comprising several practice exercises to build up your knowledge.
Do You Want More Python Practice Exercises?
We have given you a taste of the Python practice exercises available in two of our courses, Python Basics Practice and Python Practice: Word Games . These courses are designed to develop skills important to a successful Python programmer, and the exercises above were taken directly from the courses. Sign up for our platform (it’s free!) to find more exercises like these.
We’ve discussed Different Ways to Practice Python in the past, and doing interactive exercises is just one way. Our other tips include reading books, watching videos, and taking on projects. For tips on good books for Python, check out “ The 5 Best Python Books for Beginners .” It’s important to get the basics down first and make sure your practice exercises are fun, as we discuss in “ What’s the Best Way to Practice Python? ” If you keep up with your practice exercises, you’ll become a Python master in no time!
You may also like
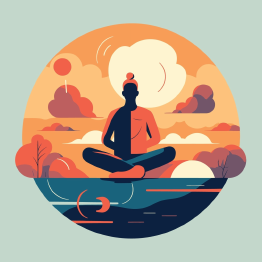
How Do You Write a SELECT Statement in SQL?
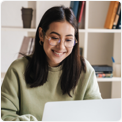
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively.
Python Examples
The best way to learn Python is by practicing examples. This page contains examples on basic concepts of Python. We encourage you to try these examples on your own before looking at the solution.
All the programs on this page are tested and should work on all platforms.
Want to learn Python by writing code yourself? Enroll in our Interactive Python Course for FREE.
- Python Program to Check Prime Number
- Python Program to Add Two Numbers
- Python Program to Find the Factorial of a Number
- Python Program to Make a Simple Calculator
- Python Program to Print Hello world!
- Python Program to Find the Square Root
- Python Program to Calculate the Area of a Triangle
- Python Program to Solve Quadratic Equation
- Python Program to Swap Two Variables
- Python Program to Generate a Random Number
- Python Program to Convert Kilometers to Miles
- Python Program to Convert Celsius To Fahrenheit
- Python Program to Check if a Number is Positive, Negative or 0
- Python Program to Check if a Number is Odd or Even
- Python Program to Check Leap Year
- Python Program to Find the Largest Among Three Numbers
- Python Program to Print all Prime Numbers in an Interval
- Python Program to Display the multiplication Table
- Python Program to Print the Fibonacci sequence
- Python Program to Check Armstrong Number
- Python Program to Find Armstrong Number in an Interval
- Python Program to Find the Sum of Natural Numbers
- Python Program to Display Powers of 2 Using Anonymous Function
- Python Program to Find Numbers Divisible by Another Number
- Python Program to Convert Decimal to Binary, Octal and Hexadecimal
- Python Program to Find ASCII Value of Character
- Python Program to Find HCF or GCD
- Python Program to Find LCM
- Python Program to Find the Factors of a Number
- Python Program to Shuffle Deck of Cards
- Python Program to Display Calendar
- Python Program to Display Fibonacci Sequence Using Recursion
- Python Program to Find Sum of Natural Numbers Using Recursion
- Python Program to Find Factorial of Number Using Recursion
- Python Program to Convert Decimal to Binary Using Recursion
- Python Program to Add Two Matrices
- Python Program to Transpose a Matrix
- Python Program to Multiply Two Matrices
- Python Program to Check Whether a String is Palindrome or Not
- Python Program to Remove Punctuations From a String
- Python Program to Sort Words in Alphabetic Order
- Python Program to Illustrate Different Set Operations
- Python Program to Count the Number of Each Vowel
- Python Program to Merge Mails
- Python Program to Find the Size (Resolution) of an Image
- Python Program to Find Hash of File
- Python Program to Create Pyramid Patterns
- Python Program to Merge Two Dictionaries
- Python Program to Safely Create a Nested Directory
- Python Program to Access Index of a List Using for Loop
- Python Program to Flatten a Nested List
- Python Program to Slice Lists
- Python Program to Iterate Over Dictionaries Using for Loop
- Python Program to Sort a Dictionary by Value
- Python Program to Check If a List is Empty
- Python Program to Catch Multiple Exceptions in One Line
- Python Program to Copy a File
- Python Program to Concatenate Two Lists
- Python Program to Check if a Key is Already Present in a Dictionary
- Python Program to Split a List Into Evenly Sized Chunks
- Python Program to Parse a String to a Float or Int
- Python Program to Print Colored Text to the Terminal
- Python Program to Convert String to Datetime
- Python Program to Get the Last Element of the List
- Python Program to Get a Substring of a String
- Python Program to Print Output Without a Newline
- Python Program Read a File Line by Line Into a List
- Python Program to Randomly Select an Element From the List
- Python Program to Check If a String Is a Number (Float)
- Python Program to Count the Occurrence of an Item in a List
- Python Program to Append to a File
- Python Program to Delete an Element From a Dictionary
- Python Program to Create a Long Multiline String
- Python Program to Extract Extension From the File Name
- Python Program to Measure the Elapsed Time in Python
- Python Program to Get the Class Name of an Instance
- Python Program to Convert Two Lists Into a Dictionary
- Python Program to Differentiate Between type() and isinstance()
- Python Program to Trim Whitespace From a String
- Python Program to Get the File Name From the File Path
- Python Program to Represent enum
- Python Program to Return Multiple Values From a Function
- Python Program to Get Line Count of a File
- Python Program to Find All File with .txt Extension Present Inside a Directory
- Python Program to Get File Creation and Modification Date
- Python Program to Get the Full Path of the Current Working Directory
- Python Program to Iterate Through Two Lists in Parallel
- Python Program to Check the File Size
- Python Program to Reverse a Number
- Python Program to Compute the Power of a Number
- Python Program to Count the Number of Digits Present In a Number
- Python Program to Check If Two Strings are Anagram
- Python Program to Capitalize the First Character of a String
- Python Program to Compute all the Permutation of the String
- Python Program to Create a Countdown Timer
- Python Program to Count the Number of Occurrence of a Character in String
- Python Program to Remove Duplicate Element From a List
- Python Program to Convert Bytes to a String
Holy Python
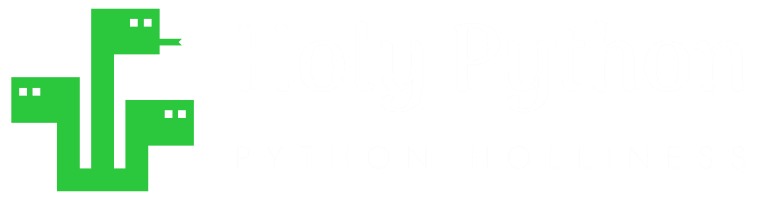
Beginner Python Exercises
Here are some enjoyable Python Exercises for you to solve! We strive to offer a huge selection of Python Exercises so you can internalize the Python Concepts through these exercises.
Among these Python Exercises you can find the most basic Python Concepts about Python Syntax and built-in functions and methods .
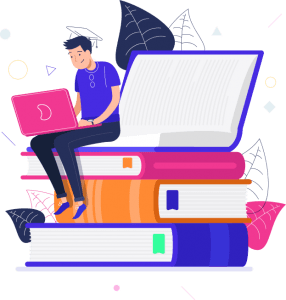
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
Choose the topics you'd like to practice from our extensive exercise list.
Python Beginner Exercises consist of some 125+ exercises that can be solved by beginner coders and newcomers to the Python world.
Majority of these exercises are online and interactive which offers an easier and convenient entry point for beginners.
First batch of the exercises starts with print function as a warm up but quickly, it becomes all about data.
You can start exercising with making variable assignments and move on with the most fundamental data types in Python:
Exercise 1: print() function | (3) : Get your feet wet and have some fun with the universal first step of programming.
Exercise 2: Variables | (2) : Practice assigning data to variables with these Python exercises.
Exercise 3: Data Types | (4) : Integer (int), string (str) and float are the most basic and fundamental building blocks of data in Python.
Exercise 4: Type Conversion | (8) : Practice converting between basic data types of Python when applicable.
Exercise 5: Data Structures | (6) : Next stop is exercises of most commonly used Python Data Structures. Namely;
- Dictionaries and
- Strings are placed under the microscope.
Exercise 6: Lists | (14) : It’s hard to overdo Python list exercises. They are fun and very fundamental so we prepared lots of them. You will also have the opportunity to practice various Python list methods.
Exercise 7: Tuples | (8) : Python Tuples Exercises with basic applications as well as common tuples methods
Exercise 8: Dictionaries | (11) : Practice with Python Dictionaries and some common dictionary methods.
Exercise 9: Strings | (14) : Basic string operations as well as many string methods can be practiced through 10+ Python String Exercises we have prepared for you.
Next batch consist of some builtin Python functions and Python methods. The difference between function and method in Python is that functions are more like standalone blocks of codes that take arguments while methods apply directly on a class of objects.
That’s why we can talk about list methods, string methods, dictionary methods in Python but functions can be used alone as long as appropriate arguments are passed in them.
Exercise 10: len() function | (5) : Python’s len function tells the length of an object. It’s definitely a must know and very useful in endless scenarios. Whether it’s manipulating strings or counting elements in a list, len function is constantly used in computer programming.
Exercise 11: .sort() method | (7) : Practice sort method in Beginner Python Exercises and later on you’ll have the opportunity practice sorted function in Intermediate Python Exercises.
Exercise 12: .pop() method | (3) : A list method pop can be applied to Python list objects. It’s pretty straightforward but actually easy to confuse. These exercises will help you understand pop method better.
Exercise 13: input() function | (6) : Input function is a fun and useful Python function that can be used to acquire input values from the user.
Exercise 14: range() function | (5) : You might want to take a real close look at range function because it can be very useful in numerous scenarios.
These range exercises offer an opportunity to get a good practice and become proficient with the usage of Python’s range function.
Exercise 15: Error Handling | (7) : Error Handling is a must know coding skill and Python has some very explanatory Error Codes that will make a coder’s life easier if he/she knows them!
Exercise 16: Defining Functions | (9) : Practicing user defined Python functions will take your programming skills to the next step. Writing functions are super useful and fun. It makes code reusable too.
Exercise 17: Python Slicing Notation | (8) : Python’s slicing notations are very interesting but can be confusing for beginner coders. These exercises will help you master them.
Exercise 18: Python Operators | (6) : Operators are another fundamental concept. We have prepared multiple exercises so they can be confident in using Python Operators.
If you struggle with any of the exercises, you can always refer to the Beginner Python Lessons .
If you’d like to challenge yourself with the next level of Python exercises check out the Intermediate Python Exercises we have prepared for you.
FREE ONLINE PYTHON COURSES
Choose from over 100+ free online Python courses.
Python Lessons
Beginner lessons.
Simple builtin Python functions and fundamental concepts.
Intermediate Lessons
More builtin Python functions and slightly heavier fundamental coding concepts.
Advanced Lessons
Python concepts that let you apply coding in the real world generally implementing multiple methods.
Python Exercises
Beginner exercises.
Basic Python exercises that are simple and straightforward.
Intermediate Exercises
Slightly more complex Python exercises and more Python functions to practice.
Advanced Exercises
Project-like Python exercises to connect the dots and prepare for real world tasks.
Thank you for checking out our Python programming content. All of the Python lessons and exercises we provide are free of charge. Also, majority of the Python exercises have an online and interactive interface which can be helpful in the early stages of learning computer programming.
However, we do recommend you to have a local Python setup on your computer as soon as possible. Anaconda is a free distribution and it comes as a complete package with lots of libraries and useful software (such as Spyder IDE and Jupyter Notebook) You can check out this: simple Anaconda installation tutorial .
Home » Python Basics
Python Basics
In this section, you’ll learn basic Python. If you’re completely new to Python programming, this Python basics section is perfect for you.
After completing the tutorials, you’ll be confident in Python programming and be able to create simple programs in Python.
Section 1. Fundamentals
- Syntax – introduce you to the basic Python programming syntax.
- Variables – explain to you what variables are and how to create concise and meaningful variables.
- Strings – learn about string data and some basic string operations.
- Numbers – introduce to you the commonly-used number types including integers and floating-point numbers.
- Booleans – explain the Boolean data type, falsy and truthy values in Python.
- Constants – show you how to define constants in Python.
- Comments – learn how to make notes in your code.
- Type conversion – learn how to convert a value of one type to another e.g., converting a string to a number.
Section 2. Operators
- Comparison operators – introduce you to the comparison operators and how to use them to compare two values.
- Logical operators – show you how to use logical operators to combine multiple conditions.
Section 3. Control flow
- if…else statement – learn how to execute a code block based on a condition.
- Ternary operator – introduce you to the Python ternary operator that makes your code more concise.
- for loop with range() – show you how to execute a code block for a fixed number of times by using the for loop with range() function.
- while – show you how to execute a code block as long as a condition is True.
- break – learn how to exit a loop prematurely.
- continue – show you how to skip the current loop iteration and start the next one.
- pass – show you how to use the pass statement as a placeholder.
Section 4. Functions
- Python functions – introduce you to functions in Python, and how to define functions, and reuse them in the program.
- Default parameters – show you how to specify the default values for function parameters.
- Keyword arguments – learn how to use the keyword arguments to make the function call more obvious.
- Recursive functions – learn how to define recursive functions in Python.
- Lambda Expressions – show you how to define anonymous functions in Python using lambda expressions.
- Docstrings – show you how to use docstrings to document a function.
Section 5. Lists
- List – introduce you to the list type and how to manipulate list elements effectively.
- Tuple – introduce you to the tuple which is a list that doesn’t change throughout the program.
- Sort a list in place – show you how to use the sort() method to sort a list in place.
- Sort a List – learn how to use the sorted() function to return a new sorted list from the original list.
- Slice a List – show you how to use the list slicing technique to manipulate lists effectively.
- Unpack a list – show you how to assign list elements to multiple variables using list unpacking.
- Iterate over a List – learn how to use a for loop to iterate over a list.
- Find the index of an element – show you how to find the index of the first occurrence of an element in a list.
- Iterables – explain to you iterables, and the difference between an iterable and an iterator.
- Transform list elements with map() – show you how to use the map() function to transform list elements.
- Filter list elements with filter() – use the filter() function to filter list elements.
- Reduce list elements into a value with reduce() – use the reduce() function to reduce list elements into a single value.
- List comprehensions – show you how to create a new list based on an existing list.
Section 6. Dictionaries
- Dictionary – introduce you to the dictionary type.
- Dictionary comprehension – show you how to use dictionary comprehension to create a new dictionary from an existing one.
Section 7. Sets
- Set – explain to you the Set type and show you how to manipulate set elements effectively.
- Set comprehension – explain to you the set comprehension so that you can create a new set based on an existing set with a more concise and elegant syntax.
- Union of Sets – show you how to union two or more sets using the union() method or set union operator ( | ).
- Intersection of Sets – show you how to intersect two or more sets using the intersection() method or set intersection operator ( & ).
- Difference of sets – learn how to find the difference between sets using the set difference() method or set difference operator ( - )
- Symmetric Difference of sets – guide you on how to find the symmetric difference of sets using the symmetric_difference() method or the symmetric difference operator ( ^ ).
- Subset – check if a set is a subset of another set.
- Superset – check if a set is a superset of another set.
- Disjoint sets – check if two sets are disjoint.
Section 8. Exception handling
- try…except – show you how to handle exceptions more gracefully using the try…except statement.
- try…except…finally – learn how to execute a code block whether an exception occurs or not.
- try…except…else – explain to you how to use the try…except…else statement to control the follow of the program in case of exceptions.
Section 9. More on Python Loops
- for…else – explain to you the for else statement.
- while…else – discuss the while else statement.
- do…while loop emulation – show you how to emulate the do…while loop in Python by using the while loop statement.
Section 10. More on Python functions
- Unpacking tuples – show you how to unpack a tuple that assigns individual elements of a tuple to multiple variables.
- *args Parameters – learn how to pass a variable number of arguments to a function.
- **kwargs Parameters – show you how to pass a variable number of keyword arguments to a function.
- Partial functions – learn how to define partial functions.
- Type hints – show you how to add type hints to the parameters of a function and how to use the static type checker (mypy) to check the type statically.
Section 11. Modules & Packages
- Modules – introduce you to the Python modules and show you how to write your own modules.
- Module search path – explain to you how the Python module search path works when you import a module.
- __name__ variable – show you how to use the __name__ variable to control the execution of a Python file as a script or as a module.
- Packages – learn how to use packages to organize modules in more structured ways.
Section 12. Working with files
- Read from a text file – learn how to read from a text file.
- Write to a text file – show you how to write to a text file.
- Create a new text file – walk you through the steps of creating a new text file.
- Check if a file exists – show you how to check if a file exists.
- Read CSV files – show you how to read data from a CSV file using the csv module.
- Write CSV files – learn how to write data to a CSV file using the csv module.
- Rename a file – guide you on how to rename a file.
- Delete a file – show you how to delete a file.
Section 13. Working Directories
- Working with directories – show you commonly used functions to work with directories.
- List files in a Directory – list files in a directory.
Section 14. Third-party Packages, PIP, and Virtual Environments
- Python Package Index (PyPI) and pip – introduce you to the Python package index and how to install third-party packages using pip.
- Virtual Environments – understand Python virtual environments and more importantly, why you need them.
- Install pipenv on Windows – show you how to install the pipenv tool on Windows.
Section 15. Strings
- F-strings – learn how to use the f-strings to format text strings in a clear syntax.
- Raw strings – use raw strings to handle strings that contain the backslashes.
- Backslash – explain how Python uses the backslashes ( \ ) in string literals.
25 Python Projects for Beginners – Easy Ideas to Get Started Coding Python
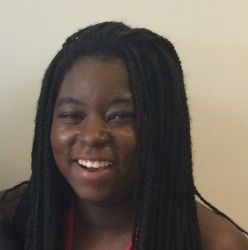
The best way to learn a new programming language is to build projects with it.
I have created a list of 25 beginner friendly project tutorials in Python.
My advice for tutorials would be to watch the video, build the project, break it apart and rebuild it your own way. Experiment with adding new features or using different methods.
That will test if you have really learned the concepts or not.
You can click on any of the projects listed below to jump to that section of the article.
If you are not familiar with the basics of Python, then I would suggest watching this beginner freeCodeCamp Python tutorial .
Python Projects You Can Build
- Guess the Number Game (computer)
- Guess the Number Game (user)
- Rock, paper, scissors
- Countdown Timer
- Password Generator
- QR code encoder / decoder
- Tic-Tac-Toe
- Tic-Tac-Toe AI
- Binary Search
- Minesweeper
- Sudoku Solver
- Photo manipulation in Python
- Markov Chain Text Composer
- Connect Four
- Online Multiplayer Game
- Web Scraping Program
- Bulk file renamer
- Weather Program
Code a Discord Bot with Python - Host for Free in the Cloud
- Space invaders game
Mad libs Python Project
In this Kylie Ying tutorial, you will learn how to get input from the user, work with f-strings, and see your results printed to the console.
This is a great starter project to get comfortable doing string concatenation in Python.
Guess the Number Game Python Project (computer)
In this Kylie Ying tutorial, you will learn how to work with Python's random module , build functions, work with while loops and conditionals, and get user input.
Guess the Number Game Python Project (user)
In this Kylie Ying tutorial, you will build a guessing game where the computer has to guess the correct number. You will work with Python's random module , build functions, work with while loops and conditionals, and get user input.
Rock, paper, scissors Python Project
In this Kylie Ying tutorial , you will work with random.choice() , if statements, and getting user input. This is a great project to help you build on the fundamentals like conditionals and functions.
Hangman Python Project
In this Kylie Ying tutorial, you will learn how to work with dictionaries, lists, and nested if statements. You will also learn how to work with the string and random Python modules.
Countdown Timer Python Project
In this Code With Tomi tutorial , you will learn how to build a countdown timer using the time Python module. This is a great beginner project to get you used to working with while loops in Python.
Password Generator Python Project
In this Code With Tomi tutorial , you will learn how to build a random password generator. You will collect data from the user on the number of passwords and their lengths and output a collection of passwords with random characters.
This project will give you more practice working with for loops and the random Python module.
QR code encoder / decoder Python Project
In this Code With Tomi tutorial , you will learn how to create your own QR codes and encode/decode information from them. This project uses the qrcode library.
This is a great project for beginners to get comfortable working with and installing different Python modules.
Tic-Tac-Toe Python Project
In this Kylie Ying tutorial, you will learn how to build a tic-tac-toe game with various players in the command line. You will learn how to work with Python's time and math modules as well as get continual practice with nested if statements.
Tic-Tac-Toe AI Python Project
In this Kylie Ying tutorial, you will learn how to build a tic-tac-toe game where the computer never loses. This project utilizes the minimax algorithm which is a recursive algorithm used for decision making.
Binary Search Python Project
In this Kylie Ying tutorial, you will learn how to implement the divide and conquer algorithm called binary search. This is a common searching algorithm which comes up in job interviews, which is why it is important to know how to implement it in code.
Minesweeper Python Project
In this Kylie Ying tutorial, you will build the classic minesweeper game in the command line. This project focuses on recursion and classes.
Sudoku Solver Python Project
In this Kylie Ying tutorial, you will learn how to build a sudoku solver which utilizes the backtracking technique. Backtracking is a recursive technique that searches for every possible combination to help solve the problem.
Photo Manipulation in Python Project
In this Kylie Ying tutorial, you will learn how to create an image filter and change the contrast, brightness, and blur of images. Before starting the project, you will need to download the starter files .
Markov Chain Text Composer Python Project
In this Kylie Ying tutorial, you will learn about the Markov chain graph model and how it can be applied the relationship of song lyrics. This project is a great introduction into artificial intelligence in Python.
Pong Python Project
In this Christian Thompson tutorial , you will learn how to recreate the classic pong game in Python. You will be working with the os and turtle Python modules which are great for creating graphics for games.

Snake Python Project
In this Tech with Tim tutorial, you will learn how to recreate the classic snake game in Python. This project uses Object-oriented programming and Pygame which is a popular Python module for creating games.
Connect Four Python Project
In this Keith Galli tutorial, you will learn how to build the classic connect four game. This project utilizes the numpy , math , pygame and sys Python modules.
This project is great if you have already built some smaller beginner Python projects. But if you haven't built any Python projects, then I would highly suggest starting with one of the earlier projects on the list and working your way up to this one.
Tetris Python Project
In this Tech with Tim tutorial, you will learn how to recreate the classic Tetris game. This project utilizes Pygame and is great for beginner developers to take their skills to the next level.
Online Multiplayer Game Python Project
In this Tech with Tim tutorial, you will learn how to build an online multiplayer game where you can play with anyone around the world. This project is a great introduction to working with sockets, networking, and Pygame.
Web Scraping Program Python Project
In this Code With Tomi tutorial , you will learn how to ask for user input for a GitHub user link and output the profile image link through web scraping. Web scraping is a technique that collects data from a web page.
Bulk File Re-namer Python Project
In this Code With Tomi tutorial , you will learn how to build a program that can go into any folder on your computer and rename all of the files based on the conditions set in your Python code.
Weather Program Python Project
In this Code With Tomi tutorial , you will learn how to build a program that collects user data on a specific location and outputs the weather details of that provided location. This is a great project to start learning how to get data from API's.
In this Beau Carnes tutorial , you will learn how to build your own bot that works in Discord which is a platform where people can come together and chat online. This project will teach you how to work with the Discord API and Replit IDE.
After this video was released, Replit changed how you can store your environments variables in your program. Please read through this tutorial on how to properly store environment variables in Replit.
Space Invaders Game Python Project
In this buildwithpython tutorial , you will learn how to build a space invaders game using Pygame. You will learn a lot of basics in game development like game loops, collision detection, key press events, and more.
I am a musician and a programmer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Python »
- 3.12.2 Documentation »
- The Python Language Reference »
- 7. Simple statements
- Theme Auto Light Dark |
7. Simple statements ¶
A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is:
7.1. Expression statements ¶
Expression statements are used (mostly interactively) to compute and write a value, or (usually) to call a procedure (a function that returns no meaningful result; in Python, procedures return the value None ). Other uses of expression statements are allowed and occasionally useful. The syntax for an expression statement is:
An expression statement evaluates the expression list (which may be a single expression).
In interactive mode, if the value is not None , it is converted to a string using the built-in repr() function and the resulting string is written to standard output on a line by itself (except if the result is None , so that procedure calls do not cause any output.)
7.2. Assignment statements ¶
Assignment statements are used to (re)bind names to values and to modify attributes or items of mutable objects:
(See section Primaries for the syntax definitions for attributeref , subscription , and slicing .)
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right.
Assignment is defined recursively depending on the form of the target (list). When a target is part of a mutable object (an attribute reference, subscription or slicing), the mutable object must ultimately perform the assignment and decide about its validity, and may raise an exception if the assignment is unacceptable. The rules observed by various types and the exceptions raised are given with the definition of the object types (see section The standard type hierarchy ).
Assignment of an object to a target list, optionally enclosed in parentheses or square brackets, is recursively defined as follows.
If the target list is a single target with no trailing comma, optionally in parentheses, the object is assigned to that target.
If the target list contains one target prefixed with an asterisk, called a “starred” target: The object must be an iterable with at least as many items as there are targets in the target list, minus one. The first items of the iterable are assigned, from left to right, to the targets before the starred target. The final items of the iterable are assigned to the targets after the starred target. A list of the remaining items in the iterable is then assigned to the starred target (the list can be empty).
Else: The object must be an iterable with the same number of items as there are targets in the target list, and the items are assigned, from left to right, to the corresponding targets.
Assignment of an object to a single target is recursively defined as follows.
If the target is an identifier (name):
If the name does not occur in a global or nonlocal statement in the current code block: the name is bound to the object in the current local namespace.
Otherwise: the name is bound to the object in the global namespace or the outer namespace determined by nonlocal , respectively.
The name is rebound if it was already bound. This may cause the reference count for the object previously bound to the name to reach zero, causing the object to be deallocated and its destructor (if it has one) to be called.
If the target is an attribute reference: The primary expression in the reference is evaluated. It should yield an object with assignable attributes; if this is not the case, TypeError is raised. That object is then asked to assign the assigned object to the given attribute; if it cannot perform the assignment, it raises an exception (usually but not necessarily AttributeError ).
Note: If the object is a class instance and the attribute reference occurs on both sides of the assignment operator, the right-hand side expression, a.x can access either an instance attribute or (if no instance attribute exists) a class attribute. The left-hand side target a.x is always set as an instance attribute, creating it if necessary. Thus, the two occurrences of a.x do not necessarily refer to the same attribute: if the right-hand side expression refers to a class attribute, the left-hand side creates a new instance attribute as the target of the assignment:
This description does not necessarily apply to descriptor attributes, such as properties created with property() .
If the target is a subscription: The primary expression in the reference is evaluated. It should yield either a mutable sequence object (such as a list) or a mapping object (such as a dictionary). Next, the subscript expression is evaluated.
If the primary is a mutable sequence object (such as a list), the subscript must yield an integer. If it is negative, the sequence’s length is added to it. The resulting value must be a nonnegative integer less than the sequence’s length, and the sequence is asked to assign the assigned object to its item with that index. If the index is out of range, IndexError is raised (assignment to a subscripted sequence cannot add new items to a list).
If the primary is a mapping object (such as a dictionary), the subscript must have a type compatible with the mapping’s key type, and the mapping is then asked to create a key/value pair which maps the subscript to the assigned object. This can either replace an existing key/value pair with the same key value, or insert a new key/value pair (if no key with the same value existed).
For user-defined objects, the __setitem__() method is called with appropriate arguments.
If the target is a slicing: The primary expression in the reference is evaluated. It should yield a mutable sequence object (such as a list). The assigned object should be a sequence object of the same type. Next, the lower and upper bound expressions are evaluated, insofar they are present; defaults are zero and the sequence’s length. The bounds should evaluate to integers. If either bound is negative, the sequence’s length is added to it. The resulting bounds are clipped to lie between zero and the sequence’s length, inclusive. Finally, the sequence object is asked to replace the slice with the items of the assigned sequence. The length of the slice may be different from the length of the assigned sequence, thus changing the length of the target sequence, if the target sequence allows it.
CPython implementation detail: In the current implementation, the syntax for targets is taken to be the same as for expressions, and invalid syntax is rejected during the code generation phase, causing less detailed error messages.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are ‘simultaneous’ (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2] :
The specification for the *target feature.
7.2.1. Augmented assignment statements ¶
Augmented assignment is the combination, in a single statement, of a binary operation and an assignment statement:
(See section Primaries for the syntax definitions of the last three symbols.)
An augmented assignment evaluates the target (which, unlike normal assignment statements, cannot be an unpacking) and the expression list, performs the binary operation specific to the type of assignment on the two operands, and assigns the result to the original target. The target is only evaluated once.
An augmented assignment expression like x += 1 can be rewritten as x = x + 1 to achieve a similar, but not exactly equal effect. In the augmented version, x is only evaluated once. Also, when possible, the actual operation is performed in-place , meaning that rather than creating a new object and assigning that to the target, the old object is modified instead.
Unlike normal assignments, augmented assignments evaluate the left-hand side before evaluating the right-hand side. For example, a[i] += f(x) first looks-up a[i] , then it evaluates f(x) and performs the addition, and lastly, it writes the result back to a[i] .
With the exception of assigning to tuples and multiple targets in a single statement, the assignment done by augmented assignment statements is handled the same way as normal assignments. Similarly, with the exception of the possible in-place behavior, the binary operation performed by augmented assignment is the same as the normal binary operations.
For targets which are attribute references, the same caveat about class and instance attributes applies as for regular assignments.
7.2.2. Annotated assignment statements ¶
Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement:
The difference from normal Assignment statements is that only a single target is allowed.
For simple names as assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated annotations. This attribute is writable and is automatically created at the start of class or module body execution, if annotations are found statically.
For expressions as assignment targets, the annotations are evaluated if in class or module scope, but not stored.
If a name is annotated in a function scope, then this name is local for that scope. Annotations are never evaluated and stored in function scopes.
If the right hand side is present, an annotated assignment performs the actual assignment before evaluating annotations (where applicable). If the right hand side is not present for an expression target, then the interpreter evaluates the target except for the last __setitem__() or __setattr__() call.
The proposal that added syntax for annotating the types of variables (including class variables and instance variables), instead of expressing them through comments.
The proposal that added the typing module to provide a standard syntax for type annotations that can be used in static analysis tools and IDEs.
Changed in version 3.8: Now annotated assignments allow the same expressions in the right hand side as regular assignments. Previously, some expressions (like un-parenthesized tuple expressions) caused a syntax error.
7.3. The assert statement ¶
Assert statements are a convenient way to insert debugging assertions into a program:
The simple form, assert expression , is equivalent to
The extended form, assert expression1, expression2 , is equivalent to
These equivalences assume that __debug__ and AssertionError refer to the built-in variables with those names. In the current implementation, the built-in variable __debug__ is True under normal circumstances, False when optimization is requested (command line option -O ). The current code generator emits no code for an assert statement when optimization is requested at compile time. Note that it is unnecessary to include the source code for the expression that failed in the error message; it will be displayed as part of the stack trace.
Assignments to __debug__ are illegal. The value for the built-in variable is determined when the interpreter starts.
7.4. The pass statement ¶
pass is a null operation — when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically, but no code needs to be executed, for example:
7.5. The del statement ¶
Deletion is recursively defined very similar to the way assignment is defined. Rather than spelling it out in full details, here are some hints.
Deletion of a target list recursively deletes each target, from left to right.
Deletion of a name removes the binding of that name from the local or global namespace, depending on whether the name occurs in a global statement in the same code block. If the name is unbound, a NameError exception will be raised.
Deletion of attribute references, subscriptions and slicings is passed to the primary object involved; deletion of a slicing is in general equivalent to assignment of an empty slice of the right type (but even this is determined by the sliced object).
Changed in version 3.2: Previously it was illegal to delete a name from the local namespace if it occurs as a free variable in a nested block.
7.6. The return statement ¶
return may only occur syntactically nested in a function definition, not within a nested class definition.
If an expression list is present, it is evaluated, else None is substituted.
return leaves the current function call with the expression list (or None ) as return value.
When return passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the function.
In a generator function, the return statement indicates that the generator is done and will cause StopIteration to be raised. The returned value (if any) is used as an argument to construct StopIteration and becomes the StopIteration.value attribute.
In an asynchronous generator function, an empty return statement indicates that the asynchronous generator is done and will cause StopAsyncIteration to be raised. A non-empty return statement is a syntax error in an asynchronous generator function.
7.7. The yield statement ¶
A yield statement is semantically equivalent to a yield expression . The yield statement can be used to omit the parentheses that would otherwise be required in the equivalent yield expression statement. For example, the yield statements
are equivalent to the yield expression statements
Yield expressions and statements are only used when defining a generator function, and are only used in the body of the generator function. Using yield in a function definition is sufficient to cause that definition to create a generator function instead of a normal function.
For full details of yield semantics, refer to the Yield expressions section.
7.8. The raise statement ¶
If no expressions are present, raise re-raises the exception that is currently being handled, which is also known as the active exception . If there isn’t currently an active exception, a RuntimeError exception is raised indicating that this is an error.
Otherwise, raise evaluates the first expression as the exception object. It must be either a subclass or an instance of BaseException . If it is a class, the exception instance will be obtained when needed by instantiating the class with no arguments.
The type of the exception is the exception instance’s class, the value is the instance itself.
A traceback object is normally created automatically when an exception is raised and attached to it as the __traceback__ attribute. You can create an exception and set your own traceback in one step using the with_traceback() exception method (which returns the same exception instance, with its traceback set to its argument), like so:
The from clause is used for exception chaining: if given, the second expression must be another exception class or instance. If the second expression is an exception instance, it will be attached to the raised exception as the __cause__ attribute (which is writable). If the expression is an exception class, the class will be instantiated and the resulting exception instance will be attached to the raised exception as the __cause__ attribute. If the raised exception is not handled, both exceptions will be printed:
A similar mechanism works implicitly if a new exception is raised when an exception is already being handled. An exception may be handled when an except or finally clause, or a with statement, is used. The previous exception is then attached as the new exception’s __context__ attribute:
Exception chaining can be explicitly suppressed by specifying None in the from clause:
Additional information on exceptions can be found in section Exceptions , and information about handling exceptions is in section The try statement .
Changed in version 3.3: None is now permitted as Y in raise X from Y .
Added the __suppress_context__ attribute to suppress automatic display of the exception context.
Changed in version 3.11: If the traceback of the active exception is modified in an except clause, a subsequent raise statement re-raises the exception with the modified traceback. Previously, the exception was re-raised with the traceback it had when it was caught.
7.9. The break statement ¶
break may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop.
It terminates the nearest enclosing loop, skipping the optional else clause if the loop has one.
If a for loop is terminated by break , the loop control target keeps its current value.
When break passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the loop.
7.10. The continue statement ¶
continue may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop. It continues with the next cycle of the nearest enclosing loop.
When continue passes control out of a try statement with a finally clause, that finally clause is executed before really starting the next loop cycle.
7.11. The import statement ¶
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary
define a name or names in the local namespace for the scope where the import statement occurs.
When the statement contains multiple clauses (separated by commas) the two steps are carried out separately for each clause, just as though the clauses had been separated out into individual import statements.
The details of the first step, finding and loading modules, are described in greater detail in the section on the import system , which also describes the various types of packages and modules that can be imported, as well as all the hooks that can be used to customize the import system. Note that failures in this step may indicate either that the module could not be located, or that an error occurred while initializing the module, which includes execution of the module’s code.
If the requested module is retrieved successfully, it will be made available in the local namespace in one of three ways:
If the module name is followed by as , then the name following as is bound directly to the imported module.
If no other name is specified, and the module being imported is a top level module, the module’s name is bound in the local namespace as a reference to the imported module
If the module being imported is not a top level module, then the name of the top level package that contains the module is bound in the local namespace as a reference to the top level package. The imported module must be accessed using its full qualified name rather than directly
The from form uses a slightly more complex process:
find the module specified in the from clause, loading and initializing it if necessary;
for each of the identifiers specified in the import clauses:
check if the imported module has an attribute by that name
if not, attempt to import a submodule with that name and then check the imported module again for that attribute
if the attribute is not found, ImportError is raised.
otherwise, a reference to that value is stored in the local namespace, using the name in the as clause if it is present, otherwise using the attribute name
If the list of identifiers is replaced by a star ( '*' ), all public names defined in the module are bound in the local namespace for the scope where the import statement occurs.
The public names defined by a module are determined by checking the module’s namespace for a variable named __all__ ; if defined, it must be a sequence of strings which are names defined or imported by that module. The names given in __all__ are all considered public and are required to exist. If __all__ is not defined, the set of public names includes all names found in the module’s namespace which do not begin with an underscore character ( '_' ). __all__ should contain the entire public API. It is intended to avoid accidentally exporting items that are not part of the API (such as library modules which were imported and used within the module).
The wild card form of import — from module import * — is only allowed at the module level. Attempting to use it in class or function definitions will raise a SyntaxError .
When specifying what module to import you do not have to specify the absolute name of the module. When a module or package is contained within another package it is possible to make a relative import within the same top package without having to mention the package name. By using leading dots in the specified module or package after from you can specify how high to traverse up the current package hierarchy without specifying exact names. One leading dot means the current package where the module making the import exists. Two dots means up one package level. Three dots is up two levels, etc. So if you execute from . import mod from a module in the pkg package then you will end up importing pkg.mod . If you execute from ..subpkg2 import mod from within pkg.subpkg1 you will import pkg.subpkg2.mod . The specification for relative imports is contained in the Package Relative Imports section.
importlib.import_module() is provided to support applications that determine dynamically the modules to be loaded.
Raises an auditing event import with arguments module , filename , sys.path , sys.meta_path , sys.path_hooks .
7.11.1. Future statements ¶
A future statement is a directive to the compiler that a particular module should be compiled using syntax or semantics that will be available in a specified future release of Python where the feature becomes standard.
The future statement is intended to ease migration to future versions of Python that introduce incompatible changes to the language. It allows use of the new features on a per-module basis before the release in which the feature becomes standard.
A future statement must appear near the top of the module. The only lines that can appear before a future statement are:
the module docstring (if any),
blank lines, and
other future statements.
The only feature that requires using the future statement is annotations (see PEP 563 ).
All historical features enabled by the future statement are still recognized by Python 3. The list includes absolute_import , division , generators , generator_stop , unicode_literals , print_function , nested_scopes and with_statement . They are all redundant because they are always enabled, and only kept for backwards compatibility.
A future statement is recognized and treated specially at compile time: Changes to the semantics of core constructs are often implemented by generating different code. It may even be the case that a new feature introduces new incompatible syntax (such as a new reserved word), in which case the compiler may need to parse the module differently. Such decisions cannot be pushed off until runtime.
For any given release, the compiler knows which feature names have been defined, and raises a compile-time error if a future statement contains a feature not known to it.
The direct runtime semantics are the same as for any import statement: there is a standard module __future__ , described later, and it will be imported in the usual way at the time the future statement is executed.
The interesting runtime semantics depend on the specific feature enabled by the future statement.
Note that there is nothing special about the statement:
That is not a future statement; it’s an ordinary import statement with no special semantics or syntax restrictions.
Code compiled by calls to the built-in functions exec() and compile() that occur in a module M containing a future statement will, by default, use the new syntax or semantics associated with the future statement. This can be controlled by optional arguments to compile() — see the documentation of that function for details.
A future statement typed at an interactive interpreter prompt will take effect for the rest of the interpreter session. If an interpreter is started with the -i option, is passed a script name to execute, and the script includes a future statement, it will be in effect in the interactive session started after the script is executed.
The original proposal for the __future__ mechanism.
7.12. The global statement ¶
The global statement is a declaration which holds for the entire current code block. It means that the listed identifiers are to be interpreted as globals. It would be impossible to assign to a global variable without global , although free variables may refer to globals without being declared global.
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Names listed in a global statement must not be defined as formal parameters, or as targets in with statements or except clauses, or in a for target list, class definition, function definition, import statement, or variable annotation.
CPython implementation detail: The current implementation does not enforce some of these restrictions, but programs should not abuse this freedom, as future implementations may enforce them or silently change the meaning of the program.
Programmer’s note: global is a directive to the parser. It applies only to code parsed at the same time as the global statement. In particular, a global statement contained in a string or code object supplied to the built-in exec() function does not affect the code block containing the function call, and code contained in such a string is unaffected by global statements in the code containing the function call. The same applies to the eval() and compile() functions.
7.13. The nonlocal statement ¶
When the definition of a function or class is nested (enclosed) within the definitions of other functions, its nonlocal scopes are the local scopes of the enclosing functions. The nonlocal statement causes the listed identifiers to refer to names previously bound in nonlocal scopes. It allows encapsulated code to rebind such nonlocal identifiers. If a name is bound in more than one nonlocal scope, the nearest binding is used. If a name is not bound in any nonlocal scope, or if there is no nonlocal scope, a SyntaxError is raised.
The nonlocal statement applies to the entire scope of a function or class body. A SyntaxError is raised if a variable is used or assigned to prior to its nonlocal declaration in the scope.
The specification for the nonlocal statement.
Programmer’s note: nonlocal is a directive to the parser and applies only to code parsed along with it. See the note for the global statement.
7.14. The type statement ¶
The type statement declares a type alias, which is an instance of typing.TypeAliasType .
For example, the following statement creates a type alias:
This code is roughly equivalent to:
annotation-def indicates an annotation scope , which behaves mostly like a function, but with several small differences.
The value of the type alias is evaluated in the annotation scope. It is not evaluated when the type alias is created, but only when the value is accessed through the type alias’s __value__ attribute (see Lazy evaluation ). This allows the type alias to refer to names that are not yet defined.
Type aliases may be made generic by adding a type parameter list after the name. See Generic type aliases for more.
type is a soft keyword .
New in version 3.12.
Introduced the type statement and syntax for generic classes and functions.
Table of Contents
- 7.1. Expression statements
- 7.2.1. Augmented assignment statements
- 7.2.2. Annotated assignment statements
- 7.3. The assert statement
- 7.4. The pass statement
- 7.5. The del statement
- 7.6. The return statement
- 7.7. The yield statement
- 7.8. The raise statement
- 7.9. The break statement
- 7.10. The continue statement
- 7.11.1. Future statements
- 7.12. The global statement
- 7.13. The nonlocal statement
- 7.14. The type statement
Previous topic
6. Expressions
8. Compound statements
- Report a Bug
- Show Source
- Contributors
Basic Statements in Python
Table of contents, what is a statement in python, statement set, multi-line statements, simple statements, expression statements, the assert statement, the try statement.
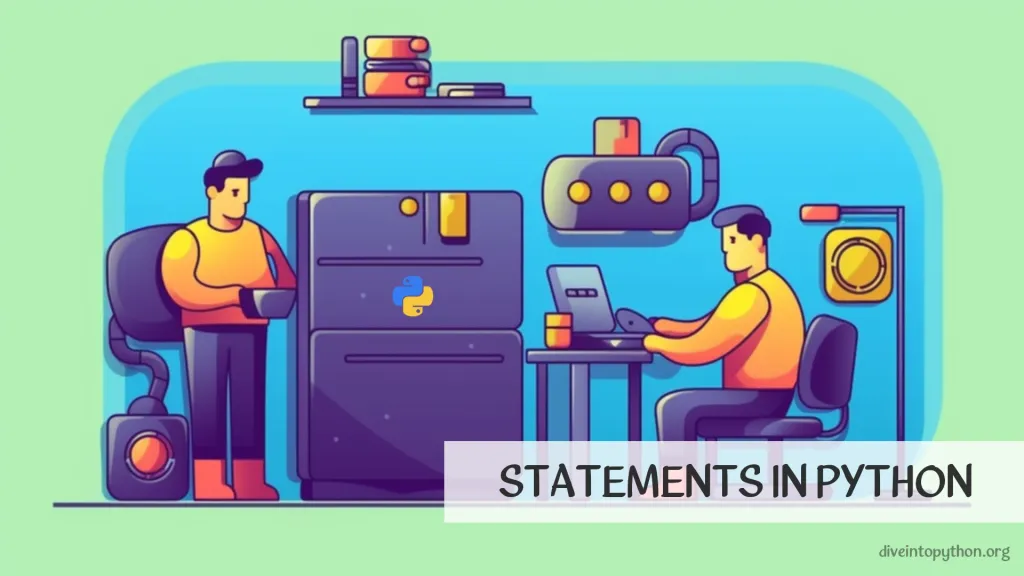
In Python, statements are instructions or commands that you write to perform specific actions or tasks. They are the building blocks of a Python program.
A statement is a line of code that performs a specific action. It is the smallest unit of code that can be executed by the Python interpreter.
Assignment Statement
In this example, the value 10 is assigned to the variable x using the assignment statement.
Conditional Statement
In this example, the if-else statement is used to check the value of x and print a corresponding message.
By using statements, programmers can instruct the computer to perform a variety of tasks, from simple arithmetic operations to complex decision-making processes. Proper use of statements is crucial to writing efficient and effective Python code.
Here's a table summarizing various types of statements in Python:
Please note that this table provides a brief overview of each statement type, and there may be additional details and variations for each statement.
Multi-line statements are a convenient way to write long code in Python without making it cluttered. They allow you to write several lines of code as a single statement, making it easier for developers to read and understand the code. Here are two examples of multi-line statements in Python:
- Using backslash:
- Using parentheses:
Simple statements are the smallest unit of execution in Python programming language and they do not contain any logical or conditional expressions. They are usually composed of a single line of code and can perform basic operations such as assigning values to variables , printing out values, or calling functions .
Examples of simple statements in Python:
Simple statements are essential to programming in Python and are often used in combination with more complex statements to create robust programs and applications.
Expression statements in Python are lines of code that evaluate and produce a value. They are used to assign values to variables, call functions, and perform other operations that produce a result.
In this example, we assign the value 5 to the variable x , then add 3 to x and assign the result ( 8 ) to the variable y . Finally, we print the value of y .
In this example, we define a function square that takes one argument ( x ) and returns its square. We then call the function with the argument 5 and assign the result ( 25 ) to the variable result . Finally, we print the value of result .
Overall, expression statements are an essential part of Python programming and allow for the execution of mathematical and computational operations.
The assert statement in Python is used to test conditions and trigger an error if the condition is not met. It is often used for debugging and testing purposes.
Where condition is the expression that is tested, and message is the optional error message that is displayed when the condition is not met.
In this example, the assert statement tests whether x is equal to 5 . If the condition is met, the statement has no effect. If the condition is not met, an error will be raised with the message x should be 5 .
In this example, the assert statement tests whether y is not equal to 0 before performing the division. If the condition is met, the division proceeds as normal. If the condition is not met, an error will be raised with the message Cannot divide by zero .
Overall, assert statements are a useful tool in Python for debugging and testing, as they can help catch errors early on. They are also easily disabled in production code to avoid any unnecessary overhead.
The try statement in Python is used to catch exceptions that may occur during the execution of a block of code. It ensures that even when an error occurs, the code does not stop running.
Examples of Error Processing
Dive deep into the topic.
- Match Statements
- Operators in Python Statements
- The IF Statement
Contribute with us!
Do not hesitate to contribute to Python tutorials on GitHub: create a fork, update content and issue a pull request.
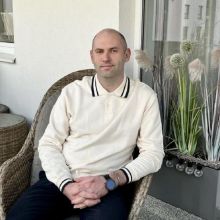
Python Lab Assignments
You can not learn a programming language by only reading the language construct. It also requires programming - writing your own code and studying those of others. Solve these assignments, then study the solutions presented here.
Python Operators: Arithmetic, Assignment, Comparison, Logical, Identity, Membership, Bitwise
Operators are special symbols that perform some operation on operands and returns the result. For example, 5 + 6 is an expression where + is an operator that performs arithmetic add operation on numeric left operand 5 and the right side operand 6 and returns a sum of two operands as a result.
Python includes the operator module that includes underlying methods for each operator. For example, the + operator calls the operator.add(a,b) method.
Above, expression 5 + 6 is equivalent to the expression operator.add(5, 6) and operator.__add__(5, 6) . Many function names are those used for special methods, without the double underscores (dunder methods). For backward compatibility, many of these have functions with the double underscores kept.
Python includes the following categories of operators:
Arithmetic Operators
Assignment operators, comparison operators, logical operators, identity operators, membership test operators, bitwise operators.
Arithmetic operators perform the common mathematical operation on the numeric operands.
The arithmetic operators return the type of result depends on the type of operands, as below.
- If either operand is a complex number, the result is converted to complex;
- If either operand is a floating point number, the result is converted to floating point;
- If both operands are integers, then the result is an integer and no conversion is needed.
The following table lists all the arithmetic operators in Python:
The assignment operators are used to assign values to variables. The following table lists all the arithmetic operators in Python:
The comparison operators compare two operands and return a boolean either True or False. The following table lists comparison operators in Python.
The logical operators are used to combine two boolean expressions. The logical operations are generally applicable to all objects, and support truth tests, identity tests, and boolean operations.
The identity operators check whether the two objects have the same id value e.i. both the objects point to the same memory location.
The membership test operators in and not in test whether the sequence has a given item or not. For the string and bytes types, x in y is True if and only if x is a substring of y .
Bitwise operators perform operations on binary operands.
- Compare strings in Python
- Convert file data to list
- Convert User Input to a Number
- Convert String to Datetime in Python
- How to call external commands in Python?
- How to count the occurrences of a list item?
- How to flatten list in Python?
- How to merge dictionaries in Python?
- How to pass value by reference in Python?
- Remove duplicate items from list in Python
- More Python articles
- Python Questions & Answers
- Python Skill Test
- Python Latest Articles
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Related Pages

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python Bitwise Operators
- Relational Operators in Python
- Python - Star or Asterisk operator ( * )
- Difference between "__eq__" VS "is" VS "==" in Python
- How To Do Math in Python 3 with Operators?
- Python 3 - Logical Operators
- Understanding Boolean Logic in Python 3
- Logical Operators in Python with Examples
- Modulo operator (%) in Python
- Concatenate two strings using Operator Overloading in Python
- Python Operators
- A += B Assignment Riddle in Python
- Python | Operator.countOf
- Format a Number Width in Python
- New '=' Operator in Python3.8 f-string
- Operator Overloading in Python
- Python | a += b is not always a = a + b
- Python Object Comparison : "is" vs "=="
- Python Arithmetic Operators
Assignment Operators in Python
Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, bitwise computations. The value the operator operates on is known as Operand .
Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables.
Now Let’s see each Assignment Operator one by one.
1) Assign: This operator is used to assign the value of the right side of the expression to the left side operand.
2) Add and Assign: This operator is used to add the right side operand with the left side operand and then assigning the result to the left operand.
Syntax:
3) Subtract and Assign: This operator is used to subtract the right operand from the left operand and then assigning the result to the left operand.
Example –
4) Multiply and Assign: This operator is used to multiply the right operand with the left operand and then assigning the result to the left operand.
5) Divide and Assign: This operator is used to divide the left operand with the right operand and then assigning the result to the left operand.
6) Modulus and Assign: This operator is used to take the modulus using the left and the right operands and then assigning the result to the left operand.
7) Divide (floor) and Assign: This operator is used to divide the left operand with the right operand and then assigning the result(floor) to the left operand.
8) Exponent and Assign: This operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
9) Bitwise AND and Assign: This operator is used to perform Bitwise AND on both operands and then assigning the result to the left operand.
10) Bitwise OR and Assign: This operator is used to perform Bitwise OR on the operands and then assigning result to the left operand.
11) Bitwise XOR and Assign: This operator is used to perform Bitwise XOR on the operands and then assigning result to the left operand.
12) Bitwise Right Shift and Assign: This operator is used to perform Bitwise right shift on the operands and then assigning result to the left operand.
13) Bitwise Left Shift and Assign: This operator is used to perform Bitwise left shift on the operands and then assigning result to the left operand.
Please Login to comment...
- Python-Operators
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Special Features
Vendor voice.
Applications

Good news: HMRC offers a Linux version of Basic PAYE Tools. Bad news: It broke
Python 2 has been dead for four years.
Updated Did you know that His Majesty's Revenue and Customs (HMRC) offers free Linux tools? Sadly, though, they recently stopped working.
Reg reader Pete Donnell alerted us to the fact that the UK tax authority offers a Linux version of its Basic PAYE Tools, which the download page describes as "free payroll software from HM Revenue and Customs (HMRC) for businesses with fewer than 10 employees."
Sounds good. We were impressed to see that, alongside its Windows and Mac versions, there's also a version of the software that supports "Ubuntu Linux version 20.04 LTS and 23.10." There's a detailed User Guide too, and more than one service status page, ranging from rather dated to extremely dated .
Of course, we wouldn't be writing about this if there weren't a snag. This story has been brewing for some time, but Pete told us that on February 5:
Basic PAYE Tools updated itself (per HMRC's recommendations), and promptly broke. I spent hours trying to fix it without success. The bug is definitely in the software itself rather than my setup: I installed a clean copy of Ubuntu 20.04 in VirtualBox, installed the latest version of the tools, and it still wouldn't work.
First release candidate of Linux kernel 6.9 looks 'fairly normal,' says Torvalds
Redis tightens its license terms, pleasing basically no one.
- Cloud Software Group snubs GPL obligations, say critics
- Linux kernel 4.14 gets a life extension, thanks to OpenELA
Despite the service status pages not showing any problems, it's been broken ever since. He tells us that he's had the software set to automatically update for about 15 years, and it's never failed before. He's tried it on both Ubuntu 20.04 and a clean install of Xubuntu 23.10, among other things. Mac users are also reporting problems .
Worryingly, Donnell notes that the software is seemingly written in Python 2. That version has been unsupported and dead for just over four years .
We contacted HMRC and put them in touch with Donnell. He subsequently spoke with them, and provided them with some troubleshooting information. A month later, he let us know that nothing had changed yet.
It seemed like a welcome surprise to find that a government department not merely acknowledges the existence of Linux, but supports it and offers Linux-specific downloads … but every silver lining has a cloud.
If anyone else is using the PAYE tools and has similar problems or, indeed, if you have the software working or can help Donnell troubleshoot what looks to be a Python 2 and Django app, do let us know. It's a free download and he tells us that it fails before any form of employer's account is needed. ®
Update to add
Mr Donnell has got back in touch to let us know that the combined powers of The Reg readership helped him to isolate the issue and resolve it. He tells us:
Turns out it's a locale issue. My test VM was in English, but it defaulted to the locale from the host machine ... If I override the locale to an English one when running the `rti.linux` executable then it seems to work.
Thanks to "pigeon" and Natalie Gritpants Jr for setting me on the right track.
I can't fault HMRC too much for not testing this particular case as it's a bit of a niche area for a specifically British piece of software.
- Open Source
Narrower topics
- AdBlock Plus
- Application Delivery Controller
- Asahi Linux
- Digital Public Goods
- Graphics Interchange Format
- Legacy Technology
- LibreOffice
- Linux Foundation
- Microsoft 365
- Microsoft Office
- Microsoft Teams
- Mobile Device Management
- One Way Forward
- Programming Language
- Retro computing
- Search Engine
- Software bug
- Software License
- Text Editor
- User interface
- Visual Studio
- Visual Studio Code
- WebAssembly
- Web Browser
- Windows Subsystem for Linux
Broader topics
- Government of the United Kingdom
- Linus Torvalds
- Operating System
Send us news
Other stories you might like
Malicious xz backdoor reveals fragility of open source, malicious ssh backdoor sneaks into xz, linux world's data compression library, a different view from the edge.
Easy-to-use make-me-root exploit lands for recent Linux kernels. Get patching
Flox rocks the nix box by conquering code chaos, canonical cracks down on crypto cons following snap store scam spree, truenas core 13 is the end of the freebsd version, progress outbids private equity in offer for mariadb plc, canva acquires affinity, further wounding a regulator-bruised adobe, fujitsu's 30-year-old uk customs system just keeps hanging on.

- Advertise with us
Our Websites
- The Next Platform
- Blocks and Files
Your Privacy
- Cookies Policy
- Privacy Policy
- Ts & Cs

Copyright. All rights reserved © 1998–2024

Want a more sustainable meat for the grill? Try a 13-foot python steak.

They’re scaly, fork-tongued and can measure upward of 20 feet long . Pythons may also be one of the most Earth-friendly meats to farm on the planet.
A group of researchers studied two large python species over 12 months on farms in Thailand and Vietnam — where snake meat is considered a delicacy — and found that they were more efficient to raise than other livestock.
Their research, published Thursday in the journal Scientific Reports , suggests that python farming could offer a solution to rising food insecurity around the globe, exacerbated by climate change.
The researchers, who studied more than 4,600 pythons, found that both Burmese and reticulated pythons grew rapidly in their first year of life, and they required less food (in terms of what’s known as feed conversion: the amount of feed to produce a pound of meat) than other farmed products, including chicken, beef , pork, salmon — and even crickets.
The snakes were fed a mix of locally sourced food, including wild-caught rodents, pork byproducts and fish pellets. They gained up to 1.6 ounces a day, with the females growing faster than their male counterparts.
The snakes were never force-fed, and the researchers found that the reptiles could fast for long periods without losing much body mass, which meant they required less labor for feeding than traditional farmed animals.
“They need very little water. A python can live off the dew that forms on its scales. In the morning, it just drinks off its scales and that’s enough,” said Daniel Natusch, a herpetologist and biodiversity expert who was involved in the research. “Theoretically you could just stop feeding it for a year.”
In a world where scientists predict climate change will lead to more extreme weather and environmental shocks, a species that is heat-tolerant, resilient to food shortages and able to produce protein “far more efficiently than anything else studied to date” is “almost a dream come true,” Natusch said.
Tastes like chicken
Snakes have long been prized in Asia, where they are used in traditional medicines, as well as in dishes such as Hong Kong’s famed snake soup . In recent years, snake farms have sprung up across Southeast Asia and China, catering to growing demand for snake meat and skins, used in luxury leather goods.
During his research, Natusch ate snake barbecued, sauteed on skewers, in curries and as jerky. He described the taste as similar to chicken, but a little more gamy. Because snakes don’t have limbs, very little is wasted in butchering, he said. And it is remarkably easy to fillet: “You just bring your knife along that backstrap and you get a four-meter-long piece of meat.”
Even so, Natusch acknowledges that snakes are unlikely to form a big part of Western diets any time soon. In his native Australia, he said, “the only good snake is a dead snake. People are pretty afraid of them .” (Pythons are nonvenomous and generally slow-moving, but they do have large teeth and may bite if provoked . They have been known to eat small pets including cats and dogs.)
In the United States, Burmese pythons are considered an invasive species, having proliferated in Florida’s Everglades, where they are hunted to cull the population. In a study last year, the U.S. Geological Survey described Florida’s python problem as “one of the most challenging invasive species management issues worldwide.”
Because store-bought meat is relatively inexpensive and easier to come by than catching these slippery creatures, Natusch doesn’t envision a future in which snake farming becomes a fix to America’s python woes. But he does see the snakes as a potential climate solution for farmers in places like Africa, where food insecurity is a growing problem as climate disasters outpace any innovation in farming techniques.
“As long as [farmers are] happy to catch a few pest rodents in their corn or their maize, and feed them to a python every now and again, you’ve got some high-quality, resilient protein right there,” he said.
Simple needs
A python’s needs are fairly basic. They’re sedentary by nature and coexist happily with other snakes, displaying “few of the complex animal welfare issues commonly seen in caged birds and mammals,” the researchers said.
Although some conservationists have expressed concerns that commercial snake farming could lead to the illegal harvesting of wild populations, Natusch — who chairs a group of snake specialists for the International Union for Conservation of Nature — argues that the opposite is true: It gives local communities a financial incentive to conserve wild populations and the habitats on which they depend.
The barriers to entry for snake farming are low in comparison with lab-grown meat , which carries significant costs and requires technical expertise. In Asia, snakes are housed in simple enclosures in warehouses. And even without the kind of genetic engineering applied over the years to domesticated animals like cows and chickens, snakes stand out, he said.
“We’re just scratching the surface here, basically the baseline product: The animal in its natural form without any domestication or anything, still outperforms all those other taxa.”


IMAGES
VIDEO
COMMENTS
What questions are included in this Python fundamental exercise? The exercise contains 15 programs to solve. The hint and solution is provided for each question. I have added tips and required learning resources for each question, which will help you solve the exercise. When you complete each question, you get more familiar with the basics of ...
To run a cell of code, click the "play button" icon to the left of the cell or click on the cell and press "Shift+Enter" on your keyboard. This will execute the Python code contained in the cell. Executing a cell that defines a variable is important before executing or authoring a cell that depends on that previously created variable assignment.
Exercise 1: User Input and Conditional Statements. Write a program that asks the user for a number then prints the following sentence that number of times: 'I am back to check on my skills!'. If the number is greater than 10, print this sentence instead: 'Python conditions and loops are a piece of cake.'.
These actions can range from simple assignments of values to variables to more complex control flow structures and iterations. Understanding different types of statements is essential for writing effective and expressive Python code. Assignment Statements. Assignment statements are the most basic type of statement in Python.
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
All Examples. Files. Python Program to Print Hello world! Python Program to Add Two Numbers. Python Program to Find the Square Root. Python Program to Calculate the Area of a Triangle. Python Program to Solve Quadratic Equation. Python Program to Swap Two Variables. Python Program to Generate a Random Number.
Exercise 1: print () function | (3) : Get your feet wet and have some fun with the universal first step of programming. Exercise 2: Variables | (2) : Practice assigning data to variables with these Python exercises. Exercise 3: Data Types | (4) : Integer (int), string (str) and float are the most basic and fundamental building blocks of data in ...
4. Write a Python program that calculates the area of a circle based on the radius entered by the user. Sample Output : r = 1.1 Area = 3.8013271108436504 Click me to see the sample solution. 5. Write a Python program that accepts the user's first and last name and prints them in reverse order with a space between them.
Section 1. Fundamentals. Syntax - introduce you to the basic Python programming syntax.; Variables - explain to you what variables are and how to create concise and meaningful variables.; Strings - learn about string data and some basic string operations.; Numbers - introduce to you the commonly-used number types including integers and floating-point numbers.
An Example of a Variable in Python is a representational name that serves as a pointer to an object. Once an object is assigned to a variable, it can be referred to by that name. Rules for Python variables. A Python variable name must start with a letter or the underscore character. A Python variable name cannot start with a number.
Python Projects You Can Build. Mad Libs. Guess the Number Game (computer) Guess the Number Game (user) Rock, paper, scissors. Hangman. Countdown Timer. Password Generator. QR code encoder / decoder.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
The difference from normal Assignment statements is that only a single target is allowed. For simple names as assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated ...
Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java. Python supports multiple programming paradigms, including object-oriented ...
Expression statements in Python are lines of code that evaluate and produce a value. They are used to assign values to variables, call functions, and perform other operations that produce a result. x = 5. y = x + 3. print(y) In this example, we assign the value 5 to the variable x, then add 3 to x and assign the result ( 8) to the variable y.
Exercise 1: Create a function in Python. Exercise 2: Create a function with variable length of arguments. Exercise 3: Return multiple values from a function. Exercise 4: Create a function with a default argument. Exercise 5: Create an inner function to calculate the addition in the following way. Exercise 6: Create a recursive function.
Assignment creates object references instead of copying the objects. Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms :-. 1. Basic form: Unmute.
python programs with output for class 12 and 11 students. Simple Assignements are for beginners starting from basics hello world program to game development using class and object concepts. A list of assignment solutions with source code are provided to learn concept by examples in an easy way.
Python Operators: Arithmetic, Assignment, Comparison, Logical, Identity, Membership, Bitwise. Operators are special symbols that perform some operation on operands and returns the result. For example, 5 + 6 is an expression where + is an operator that performs arithmetic add operation on numeric left operand 5 and the right side operand 6 and ...
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
So, Assignment Operators are used to assigning values to variables. Now Let's see each Assignment Operator one by one. 1) Assign: This operator is used to assign the value of the right side of the expression to the left side operand. Syntax: Example: Output: 2) Add and Assign: This operator is used to add the right side operand with the left ...
Data Analysis has always been a very important field and a highly demanded skill. Until recently, it has been practiced using mostly closed, expensive, and limited tools like Excel or Tableau. Python, pandas, and other open-source libraries have changed Data Analysis forever and have become must-have tools for anyone looking to build a career as a Data Analyst.
This Object-Oriented Programming (OOP) exercise aims to help you to learn and practice OOP concepts. All questions are tested on Python 3. Python Object-oriented programming (OOP) is based on the concept of "objects," which can contain data and code: data in the form of instance variables (often known as attributes or properties), and code, in the form method.
Updated Did you know that His Majesty's Revenue and Customs (HMRC) offers free Linux tools? Sadly, though, they recently stopped working. Reg reader Pete Donnell alerted us to the fact that the UK tax authority offers a Linux version of its Basic PAYE Tools, which the download page describes as "free payroll software from HM Revenue and Customs (HMRC) for businesses with fewer than 10 employees."
A python's needs are fairly basic. They're sedentary by nature and coexist happily with other snakes, displaying "few of the complex animal welfare issues commonly seen in caged birds and ...