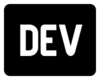

DEV Community

Posted on Sep 21, 2021
Getting started with Spring Boot: Creating a simple movies list API.
Spring boot is widely considered an entry to the Spring ecosystem, simple yet effective and powerful! Spring boot makes it possible to create Spring-based stand-alone, quality production apps with minimum needed configuration specifications. Let's keep it simple, just like the movies list API we're about to create!
Before we start
Your project structure matters, Spring boot gives you all the freedom to structure your project the way you see fit, you may want to use that wisely.
There's not a particular structure you need to follow, I'll be introducing mine on this project but that doesn't mean you have to follow it the way it is, as I said you're free!
Most tech companies, including mine, use guiding dev rules to keep the code clean and pretty, the rules are there to help you, don't go rogue.
Phase 0 : Introduction to the global architecture
Before we get busy with the layers specifications, let's have a bird's-eye view :
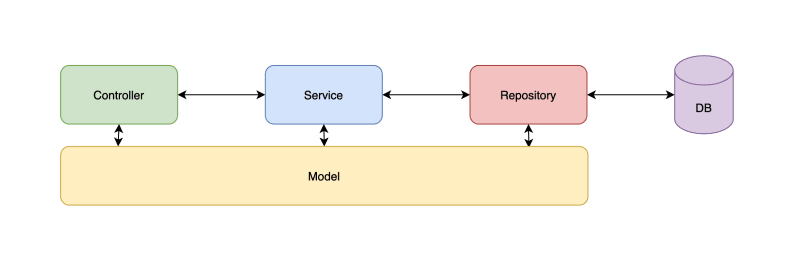
Phase I : Model definition
Models are the data structure which you're project is dealing with (example: you're building a user management system, the user is your model among others).
Usually, the software architect will be there to provide you with the needed resources to build your Spring boot models, those resources mainly are UML class diagrams. Here's ours :
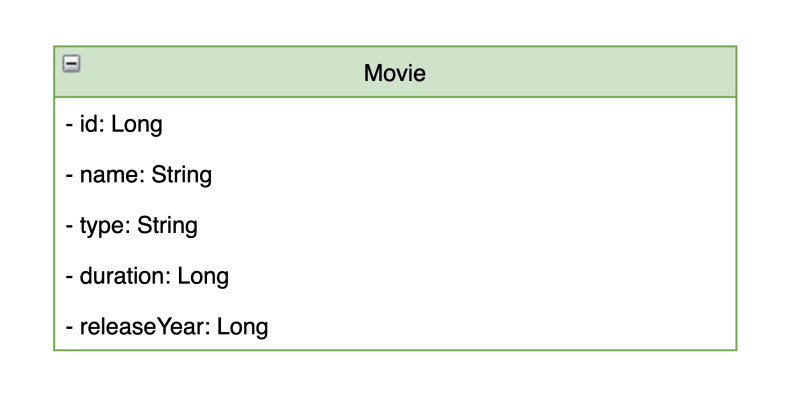
NOTE: I decided for this model layer to be as simple as possible, to not give a wall to climb instead of a first step. In real-life projects, things get ugly and much more complicated.
Phase II : Service definition
After the model layer, now it's time to blueprint our services' structure, in other words, we need to define what service our API is going to mainly provide.
The services usually are fully invocated by the controller layer which means they usually have the same structure, but not all the time.
This one also will be provided by the software architect, and it comes as a detailed list with descriptions, a set of UML sequence diagrams, or both.
We need our API to be able to provide us with a list of saved movies, save, update and delete a movie.
Our end-points will be the following:
- Save EP : This allows saving a movie by sending a JSON object using an HTTP/POST request.
- Update EP : This allows updating a movie by sending a JSON object using an HTTP/PUT request.
- Delete EP : This allows deleting a movie by sending its id using an HTTP/DELETE request.
- Find all EP : This allows retrieving all the existing movies by sending an HTTP/GET request. (It's not recommended to open a find all end-point because it will slow down your application, instead set a limit, Example: 10 movies per request).
Why are we using multiple HTTP verbs?
If I were in your shoes right now, I'd be asking the same question. First, you can use it as you want with no restrictions, use an HTTP/POST request to retrieve movies, yes you can do that but, there's a reason why we don’t.
Dev rules and conventions are there to help you and keep things in order, otherwise, all we'll be building is chaos!
HTTP verbs are meant to describe the purpose of the request:
Each request requires a response, in both success and error scenarios, HTTP response codes are there to define what type of response we are getting.
Know more about HTTP response codes here
Phase III: Technical benchmarking
Now, we need to define the project technical requirements, what communication protocol we should use, what Database is suitable to store data, etc.
We will be using REST as our main communication protocol, H2/file database to store our data. I chose those technologies for instructional purposes so that I could offer a simple yet working example, but in real-life projects, we select technologies only on the basis of their utility and ability to aid in the development of our API. That was the second factor that influenced my decision.
NOTE: The more technology experience you have, the more accurate your decisions will be.
Phase IV: Development
Github repository initialization.
To make this code accessible for all of you, and insure its version, we'll be using git and GitHub, find all the code here : Github Repo
Spring Initializr
Spring provides us with an Initialization suite that helps us start our project quicker than usual.

After the init and downloading the basic version of our project, there're three important files you need to know about :
1. The application main class (aka. the backbone)
This class is the main class of the project and it represents its center. NOTE: All packages should be under the main package of your application so they can be bootstrapped.
2. The application properties file
This file is a way to put your configuration values which the app will use during the execution (example: URL to the database, server's port, etc.). NOTE: You can add more properties files, I'll be covering that up in an upcoming article. NOTE1: By default the file is empty.
3. The pom file
Since we are using Maven as our dependency manager (you can use Gradle instead) we have a pom.xml file containing all the data about our dependencies.
Building the model layer
The model layer is the direct implementation of our class diagrams as Java classes.
package io.xrio.movies.repository;
import io.xrio.movies.model.Movie; import org.springframework.data.jpa.repository.JpaRepository; import org.springframework.stereotype.Repository;
@Repository public interface MoviesRepository extends JpaRepository { }
Movie findByName(String name);
package io.xrio.movies.exception;
import lombok.Data; import lombok.EqualsAndHashCode;
- @author : Elattar Saad
- @version 1.0
@since 10/9/2021 */ @EqualsAndHashCode(callSuper = true) @data @AllArgsConstructor public class MovieDuplicatedException extends Exception{
- The duplicated movie's id */ private Long mid;
@EqualsAndHashCode(callSuper = true) @data @AllArgsConstructor public class MovieNotFoundException extends Exception{
package io.xrio.movies.service;
import io.xrio.movies.exception.MovieDuplicatedException; import io.xrio.movies.exception.MovieNotFoundException; import io.xrio.movies.model.Movie;
import java.util.List;
public interface MovieService {
package io.xrio.movies.service.impl;
import io.xrio.movies.exception.MovieDuplicatedException; import io.xrio.movies.exception.MovieNotFoundException; import io.xrio.movies.model.Movie; import io.xrio.movies.repository.MoviesRepository; import io.xrio.movies.service.MoviesService; import lombok.Data; import org.springframework.stereotype.Service;
@Service @data public class MovieServiceImpl implements MovieService {
@Service public class MovieServiceImpl implements MovieService {
package io.xrio.movies.controller;
import io.xrio.movies.exception.MovieDuplicatedException; import io.xrio.movies.exception.MovieNotFoundException; import io.xrio.movies.model.Movie; import io.xrio.movies.service.MovieService; import lombok.Data; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*;
@RestController @RequestMapping("movie") @data public class MovieController {
package io.xrio.movies.controller.advice;
import io.xrio.movies.exception.MovieDuplicatedException; import io.xrio.movies.exception.MovieNotFoundException; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.servlet.mvc.method.annotation.ResponseEntityExceptionHandler;
@ControllerAdvice public class MovieControllerExceptionHandler {
Top comments (0)
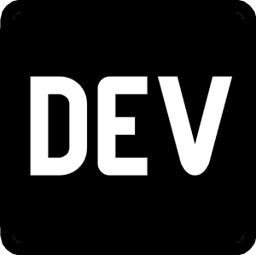
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
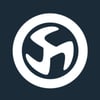
Collecting JavaScript code coverage with Capybara in Ruby on Rails application
JT Dev - May 14
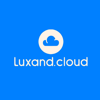
How paranoid is it to not use facial recognition on an Iphone?
Luxand.cloud - May 14
4 Tricks to Write Catchy Headlines

How to validate Node + Express requests with Joi middleware
Mattia Malonni - May 14
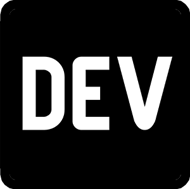
We're a place where coders share, stay up-to-date and grow their careers.
reassignment surgery movie
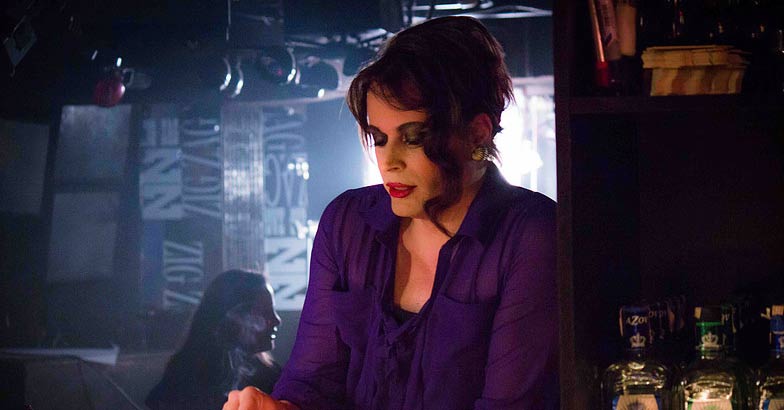
Rest API for Movie Ticket Booking project using Spring boot, JPA, JWT, Postman and MYSQL
RestAPI Project in Spring boot for movie ticket booking. In this article will discuss the feature, functionality, tools, and technologies to create a Movie ticket booking project backend into spring boot and JPA with JWT and Spring security to create an authentication token for login.
Online Movie ticket booking project in spring boot, RestAPI, and JPA
Simple Spring Boot application which uses an in-memory database H2 or MySQL DB to manage their movie-related data.
It uses RestController to create backend APIS, JWT to create authentication tokens, JPA to perform database transactions and PostMan to run the APIS.
Let’s understand more features and functionalities according to the user’s role in the application.
Admin Stories:
As Admin Must need different endpoints/web services/APIs to manage data.
- POST mapping to save details of the movie.
- PUT mapping to update the movie release date.
- GET mapping to get a movie by movie name.
- DELETE mapping to delete movie details.
- GET mapping to get all movies that fall under the community genre.
Validate the data entered and allow accessing the options.
User Stories:
- As a user, I need to register, log in, and log out of the application.
- As a user, I need to see the list of movies
- As a user, I should be able to add a movie to my favorites list.
- As a User, I should be able to remove a movie from my favorite list
Instructions:
- Please don’t use any UI in the application
- Create 2 roles, User and Admin.
- Use the database to store information about movies.
- Use proper table structure to manage the data.
- create a custom exception for handling the improper inputs.
- Test the RESTful endpoints on the postman.
- Please use spring Boot JPA to store the data.
Leave a Comment Cancel Reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

Movie Review and Rating Portal project in Java using JSP and Servlet with Source Code
- Bhupendra Patidar
- March 17, 2022
- Java Web Project
Movie Review and Rating Portal in Java using JSP and Servlet with Source Code. This project is designed for final year students, keeping in mind the requirements and complexity. It provides movie reviews and ratings based on the experience of a user.
In this project, Admin will be able to add/remove movies to the list whereas, a user would be able to watch and share their review for a particular movie in the form of a comment. Also, it will allow users to rate a movie based on their experience.
The whole project is developed using Servlet and JSP. At the front end, we have used HTML, CSS, and Bootstrap. At the data access layer, we have used JDBC API. The Database used here is MYSQL. The whole project is following the MVC(Model View & Controller) design pattern.
Movie Review and Rating Portal in Java
Gathering information related to any topic let it be the best places in the world, the best gadgets available, or the best movie to watch online, one would always look up to get it through social media platforms or any sources available online. Movies indeed dominate the world of entertainment and, to choose which one to watch from the list of millions is confusing. Out of many, one way to tackle this confusion is to go by reviews and ratings available online for a movie.
So, for this, we on Codebun have developed Movie Review and Rating Portal in Java to help the users save their time. Here Admin has the control of adding new movies or removing the existing ones and where a user can share their reviews and rate the movie.
The following are the major objective of this application:
- To provide a bug-free application to the admin.
- The main objective is to build a secured, robust Movie Review and Rating Portal allowing Admin to have control over it and allowing users to have a trouble-free experience.
- It maintains the record of movies their reviews and ratings and, users.
Modules of Movie Review and Rating Portal in Java
- Manage Admin User : It will allow Admin to manage the portal.
- Manage Register User : In this module, Admin can manage each and every user registered on the portal.
- Manage Movies Category : This module will allow Admin to categorize movies based on genres, language, or ratings.
- Manage Movies : Admin can add or remove movies in this module.
- Manage Movie Ratings : In this module, Admin can manage movies based on their ratings.
- Manage User Reviews : Here Admin would be able to manage reviews given by users for movies.
- Request for Registration: In this module, Users can register themselves on the portal.
- Login to the Portal: Registered Users can log in to the portal with this module.
- Manage Profile: In this module, every registered User can manage their profiles by editing names and other details.
- View Reviews: In this module, a user would be able to view reviews for different movies.
- Give Reviews: This module will allow the user to give their reviews based on their movie experience.
- Give Ratings: Similarly, in this module, a user can rate a movie depending on their experience.
Tools and Technologies
Technology/Domain: Java Front-End: JSP, Html, CSS, JS, Bootstrap. Server-side: Servlet. Back-end: MYSQL. Server: Tomcat 8.5.
Contact to get the Source Code
Skype Id: jcodebun Email: [email protected] WhatsApp: +91 8827363777 Price: 1999 INR
Note: If you need the source code you can contact Us. We will provide complete source code and all the required things like Database and project reports with all the diagrams. Also, we have created a STEP by STEP configuration tutorial to help you in the configuration process.
If you find any kind of difficulties during the configuration, we will provide a complete project configuration guide remotely using any Desk or Zoom.
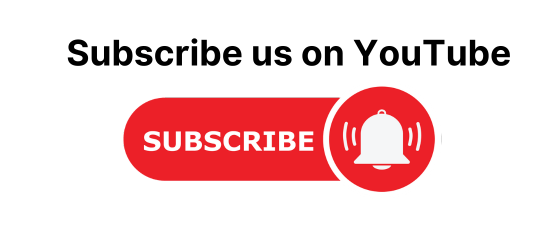
Recent Post
Cannot find the declaration of element ‘project’ in pom.xml, failed to configure a datasource: ‘url’ attribute is not specified, solve “non-resolvable parent pom” error in pom.xml with spring boot 3, jsp and servlet project configuration tutorial, how to solve error dependencies.dependency.version’ for mysql:mysql-connector-java:jar is missing in pom.xml, atm management backend restapi project in spring boot, jpa, mysql, postman with source code, home service booking project in spring boot, hibernate, jpa and jsp with source code, ecommerce project in reactjs and spring boot using jpa, jwt, mysql, restapi, postman, current request is not a multipart request in spring boot and reactjs, submit form data with image or file in spring boot, reactjs and postman.
- [email protected]
- +91 8827363777
- Who We Are?
- Services And Plan
- Privacy Policy
- Terms And Conditions
- Java program
- Selenium Tutorial
- Selenium Web Driver With Java
- Selenium Web Driver With C#
- Katalon studio
- Puppeteer and Jest
WhatsApp For Projects
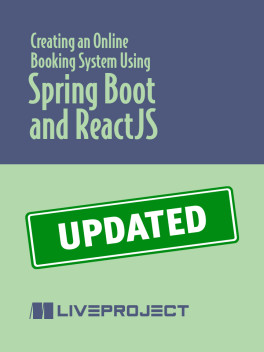
Creating an Online Booking System Using Spring Boot and ReactJS you own this product $(document).ready(function() { $.ajax({ url: "/ajax/getWishListDetails" }).done(function (data) { if (!jQuery.isEmptyObject(data) && data['wishlistProductIds']) { $(".wishlist-container").each(function() { if (data.wishlistProductIds.indexOf($(this).find('.wishlist-toggle').data('product-id')) > -1) { $(this).addClass("on-wishlist"); } }); } }); $.ajax({ url: "/ajax/getProductOwnershipDetails?productId=1466" }).done(function (data) { if (!jQuery.isEmptyObject(data)) { if (data['ownership']) { $(".wishlist-container").hide(); $(".ownership-indicator").addClass('owned'); $(document.body).addClass("user-owns-product"); } } }); }); document.addEventListener("subscription-status-loaded", function(e){ var status = e && e.detail && e.detail['status']; if(status != "ACTIVE" && status != "PAUSED"){ return; } if(window.readingListsServerVars != null){ $(document).ready(function() { var $readingListToggle = $(".reading-list-toggle"); $(document.body).append(' '); $(document.body).append(' loading reading lists ... '); function adjustReadingListIcon(isInReadingList){ $readingListToggle.toggleClass("fa-plus", !isInReadingList); $readingListToggle.toggleClass("fa-check", isInReadingList); var tooltipMessage = isInReadingList ? "edit in reading lists" : "add to reading list"; $readingListToggle.attr("title", tooltipMessage); $readingListToggle.attr("data-original-title", tooltipMessage); } $.ajax({ url: "/readingList/isInReadingList", data: { productId: 1466 } }).done(function (data) { adjustReadingListIcon(data && data.hasProductInReadingList); }).catch(function(e){ console.log(e); adjustReadingListIcon(false); }); $readingListToggle.on("click", function(){ if(codePromise == null){ showToast() } loadCode().then(function(store){ store.requestReadingListSpecificationForProduct({ id: window.readingListsServerVars.externalId, manningId: window.readingListsServerVars.productId, title: window.readingListsServerVars.title }); ReadingLists.ReactDOM.render( ReadingLists.React.createElement(ReadingLists.ManningOnlineReadingListModal, { store: store, }), document.getElementById("reading-lists-modal") ); }).catch(function(e){ console.log("Error loading code reading list code"); }); }); var codePromise var readingListStore function loadCode(){ if(codePromise) { return codePromise } return codePromise = new Promise(function (resolve, reject){ $.getScript(window.readingListsServerVars.libraryLocation).done(function(){ hideToast() readingListStore = new ReadingLists.ReadingListStore( new ReadingLists.ReadingListProvider( new ReadingLists.ReadingListWebProvider( ReadingLists.SourceApp.marketplace, getDeploymentType() ) ) ); readingListStore.onReadingListChange(handleChange); readingListStore.onReadingListModalChange(handleChange); resolve(readingListStore); }).catch(function(){ hideToast(); console.log("Error downloading reading lists source"); $readingListToggle.css("display", "none"); reject(); }); }); } function handleChange(){ if(readingListStore != null) { adjustReadingListIcon(readingListStore.isInAtLeastOneReadingList({ id: window.readingListsServerVars.externalId, manningId: window.readingListsServerVars.productId })); } } var $readingListToast = $("#reading-list-toast"); function showToast(){ $readingListToast.css("display", "flex"); setTimeout(function(){ $readingListToast.addClass("shown"); }, 16); } function hideToast(){ $readingListToast.removeClass("shown"); setTimeout(function(){ $readingListToast.css("display", "none"); }, 150); } function getDeploymentType(){ switch(window.readingListsServerVars.deploymentType){ case "development": case "test": return ReadingLists.DeploymentType.dev; case "qa": return ReadingLists.DeploymentType.qa; case "production": return ReadingLists.DeploymentType.prod; case "docker": return ReadingLists.DeploymentType.docker; default: console.error("Unknown deployment environment, defaulting to production"); return ReadingLists.DeploymentType.prod; } } }); } });
- Development
- project for $49.99
- subscription from $19.99 includes this product
pro $24.99 per month
- access to all Manning books, MEAPs, liveVideos, liveProjects, and audiobooks!
- choose one free eBook per month to keep
- exclusive 50% discount on all purchases
lite $19.99 per month
- access to all Manning books, including MEAPs!
5, 10 or 20 seats+ for your team - learn more
In this liveProject, you’ll take on the role of a web developer with a new client: a spa company called AR Salon. AR Salon wants to update its old over-the-phone manual booking system into a modern web-based service. Your challenge is to design and build this system, which will allow users to view available slots for spa services, pay for services, and retrieve customer information. To accomplish this, you’ll use Java and Spring Boot to construct a reliable backend, and JavaScript and React to build a responsive user interface.
UPDATED: July 2023 to the latest version of all technologies and text.
book resources
When you start your liveProject, you get full access to the following books for 90 days.
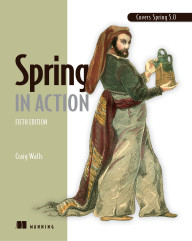
project author
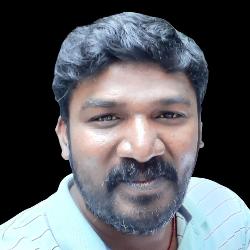
prerequisites
This liveProject is for programmers familiar with Java and JavaScript, who want experience in full-stack web development. To begin this liveProject, you will need to be familiar with the following:
- Basics of Java
- Basics of Spring Boot
- Basics of JavaScript
- Basics of ReactJS
- Basics of JPA
- Basics of Swagger
- Basics of Payment Gateway Integration
- Basics of Barcode Software
you will learn
In this liveProject, you’ll learn important concepts for creating production-ready web apps. The skills you master are essential for any web developer, and at the end of the project you’ll have a professional-level app for your portfolio.
- Spring Boot and REST operations
- Gradle dependency management
- CRUD operations
- Responsive UI with React
- Stripe Payment Integration
choose your plan
- choose another free product every time you renew
- choose twelve free products per year
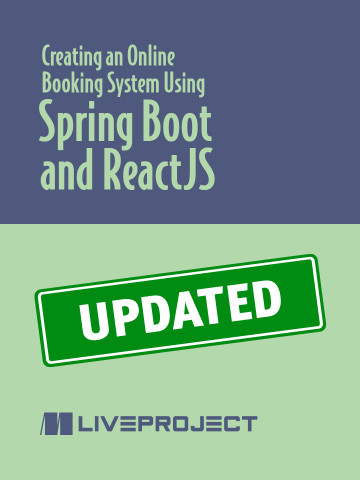
- five seats for your team
- Subscribe to our Newsletter
- Manning on LinkedIn
- Manning on Instagram
- Manning on Facebook
- Manning on Twitter
- Manning on YouTube
- Manning on Twitch
- Manning on Mastodon
how to play
- guess the geekle in 5-, 6-, 7- tries.
- each guess must be a valid 4-6 letter tech word. hit enter to submit.
- after each guess, the color of the tiles will change to show how close your guess was to the word.
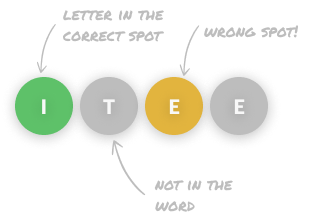
geekle is based on a wordle clone .
- United States
BUY 2 OR MORE, GET 30% OFF SITEWIDE
Online & in stores. Auto-applied at checkout.
Chasing The Sun
New summer arrivals.
Wherever you’re headed this season, don’t forget to take us with you. Our newest warm-weather fits love to be out and about.
DRESS WEATHER
HERITAGE GRAPHICS
MAY FEATURES
Levi’s® x ERL
Levi’s® and ERL join forces for a second collaborative capsule. The latest collection expands on ERL’s imaginative approach to form, finish & texture. Featuring a five-piece range of light wash denim pieces, that pay homage to California’s sun-faded energy.
CELEBRITY STYLIST MARK AVERY
In honor of the upcoming 501® Day, learn more about his lifelong love story with our first-ever fit.
AAPI Heritage Month 2024
Levi’s® highlights three Asian-American artists breaking barriers in music, Hollywood and skateboarding.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
电影推荐系统(Movie Recommendation System,后端:Spring Boot + Spring Security + Redis + MyBatis-Plus)前后端分离项目
junweizeng/movie_recommendation_system_server
Folders and files, repository files navigation.
总项目名称: 电影推荐系统
项目采用 前后端分离 :
- 仓库地址: movie_recommendation_system_vue
- 技术栈: Vue3 + Element Plus + axios 等
- 仓库地址: movie_recommendation_system_server
- 技术栈: Spring Boot + Spring Security + Redis + MyBatis-Plus 等
- 简介:爬取项目所需的电影基本信息数据和用户评价数据等并存储。
- 仓库地址: douban_movie_spider_mrs
- 技术栈: requests + lxml

IDEA右边栏中选择 Maven → 按住 Ctrl → 选择 Lifecycle 下的 clean 和 package → 点击上方的 绿色运行按钮 → 等待项目打包成jar包 → 打包好的jar包会在项目的 target 目录中

将打包好的 项目jar包 上传到自己的服务器上。
通过以下命令时项目在服务器后台运行,并且输出日志到 out.txt 文件(可修改):
nohup java -jar 项目名.jar >out.txt &
- 用户登录、注册、个人信息修改等接口实现。
- 基于内容推荐和基于用户协同过滤推荐等推荐算法实现,整合两种算法实现混合式推荐,并解决冷启动问题。
- 查询各种电影信息接口实现(如电影搜索、推荐给用户的电影信息查询等)。
- 用户点赞功接口实现(点赞信息暂存,再按时持久化到数据库)。
- Java 100.0%

IMAGES
VIDEO
COMMENTS
Movie Recommendation System is a Java-based project developed using Spring Boot (version: 2.3.4) and MySQL (version: 8.0). It provides a platform for users to register, rate movies, and receive personalized movie recommendations based on their preferences and ratings.
🔴 Check out Data Science Course from Odin School - https://hubs.la/Q022FBYf0🔴 60% in Class 12 to Working for Google and Amazon , My Journey - https://youtu...
Spring boot is widely considered an entry to the Spring ecosystem, simple yet effective and powerful! Spring boot makes it possible to create Spring-based stand-alone, quality production apps with minimum needed configuration specifications. Let's keep it simple, just like the movies list API we're about to create!
Workshop recorded live on Jan 26, 2019. This course is still in progress.We'll build the second microservice that contains an API to return movie information...
In today's world, booking movie tickets online has become a common practice, and managing an online movie ticket booking platform can be a daunting task. However, the Online Movie Ticket Booking Project, built using Spring Boot and JPA, RestAPI, Postman, and MYSQL, is designed to simplify the process. The Online Movie Ticket Booking Project ...
Summary. Spring Boot is a powerful apps making tool, takes away all the times consuming tasks and leaves you with the logical ones to handle. Don't get fouled, building APIs is not that easy, and from what I saw, that's just the tip of the Iceberg! Find the source code here.
In this video, i have created a demo of Movie-seat-select Java project.Made some modification in last Bus-seat projectsource-code:- https://github.com/vishal...
Movie. There are 4 rest APIs that will Add, Edit, Update, Delete, and the Movie records. that contains the theater entities: movie name, code, Screen number, Movie language, Director name, Casting, price, and Image URL. Movie booking Order Details. Create order and View order details APIs will be used for Movie ticket booking. Below is a sample ...
DEV Community. Posted on Sep 21, 2021. Getting started with Spring Boot: Creating a simple movies list API. Spring boot is widely considered an entry to the Spring ecosystem, simp
Inside this course we will build an advance movie review app like IMDB where you will have your own admin pannel to upload movies and users can rate the movie from 1 to 10. As admin you can see the progress, add, update or delete the movies. Also you can create new actors whom you can then add inside movies as they are the actors of that ...
1,266 5 21 45. 2. Spring Boot is designed for large scale production-ready features. Spring's scheduling module is quite robust. Your Cron expression question is really broad and unclear, you may want to consider rewording. There are tons of articles/tutorials online that explain Cron expressions, maybe some googleing will get you the guidance ...
Backend Project in Java, spring boot for booking movies ticket using JPA, Postman, MYSQL, MySQL workbench, Maven, MVC, Tomcat, and Eclipse as IDE.It's a paid...
Online Movie ticket booking project in spring boot, RestAPI, and JPA. Simple Spring Boot application which uses an in-memory database H2 or MySQL DB to manage their movie-related data. It uses RestController to create backend APIS, JWT to create authentication tokens, JPA to perform database transactions and PostMan to run the APIS.
The Online Cinema Ticket Booking System is a web-based application developed using Spring Boot and various technologies such as core Java, RESTful web services, Hibernate, JSP, HTML, and CSS. This system enables users to browse available movies, select theaters, and book movie tickets seamlessly.
At the same time, Admin can manage all the users and movies from the backend admin panel. Technology Stack. Spring Boot: For rapid development of the application with minimal configuration. Hibernate: For object-relational mapping (ORM) and database interactions. Spring MVC: To implement the Model-View-Controller architecture.
Modules of Movie Review and Rating Portal in Java Admin. Manage Admin User: It will allow Admin to manage the portal.; Manage Register User: In this module, Admin can manage each and every user registered on the portal.; Manage Movies Category: This module will allow Admin to categorize movies based on genres, language, or ratings.; Manage Movies: Admin can add or remove movies in this module.
Movies review and rating project in java with source code using JSP, HTML, CSS, Servlet, MYSQL, Maven, MVC, and Tomcat.https://codebun.com/product/movie-revi...
In this liveProject, you'll take on the role of a web developer with a new client: a spa company called AR Salon. AR Salon wants to update its old over-the-phone manual booking system into a modern web-based service. Your challenge is to design and build this system, which will allow users to view available slots for spa services, pay for services, and retrieve customer information. To ...
AAPI Heritage Month 2024. Levi's® highlights three Asian-American artists breaking barriers in music, Hollywood and skateboarding. The official Levi's® US website has the best selection of Levi's® jeans, jackets, and clothing for men, women, and kids. Shop the entire collection today.
仓库地址:movie_recommendation_system_vue; 技术栈:Vue3 + Element Plus + axios等; 后端: 仓库地址:movie_recommendation_system_server; 技术栈:Spring Boot + Spring Security + Redis + MyBatis-Plus等; 数据爬虫: 简介:爬取项目所需的电影基本信息数据和用户评价数据等并存储。