Top 50 JavaScript Interview Questions With Example Answers

Preparing for a JavaScript interview requires a lot of work. It’s important to be well-versed in the fundamentals but you also should have some grasp on how to debug JavaScript code, what some of the advanced functions are and how to build projects in it.

Common JavaScript Interview Questions
- What are the different data types in JavaScript?
- What is hoisting in JavaScript?
- What is the difference between null and undefined?
- What are closures in JavaScript?
- What is a callback function in JavaScript?
- What are promises in JavaScript?
- What is the purpose of the setTimeout() function in Javascript?
- How can you check if an array includes a certain value?
- How can you remove duplicates in an array?
- What is the purpose of async and await in JavaScript?
Below are some tips for preparing for the interview along with some common questions and answers to help you ace your next interview.
JavaScript Interview Questions and Answers With Examples
JavaScript interview questions range from the basics like explaining the different data types in JavaScript to more complicated concepts like generator functions and async and await. Each question will have answers and examples you can use to prepare for your own interview.
More on JavaScript How to Use the Ternary Operator in JavaScript
JavaScript Fundamentals
1. what is javascript.
A high-level, interpreted programming language called JavaScript makes it possible to create interactive web pages and online apps with dynamic functionality. Commonly referred to as the universal language, Javascript is primarily used by developers for front-end and back-end work.
2. What are the different data types in JavaScript?
JavaScript has six primitive data types:
It also has two compound data types:
3. What is hoisting in JavaScript?
Hoisting is a JavaScript concept that refers to the process of moving declarations to the top of their scope. This means that variables and functions can be used before they are declared, as long as they are declared before they are used in a function.
For example, the following code will print "Hello, world!" even though the greeting variable is not declared until after the console.log() statement.
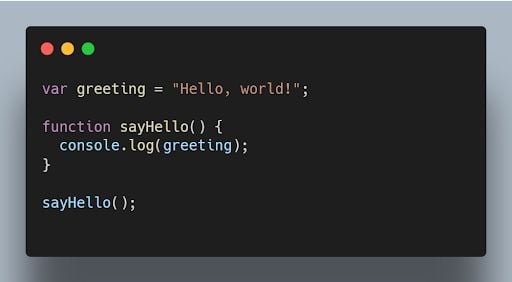
4. What is the difference between null and undefined?
null is an assignment value that represents no value or an empty value , while undefined is a variable that has been declared but not assigned a value.
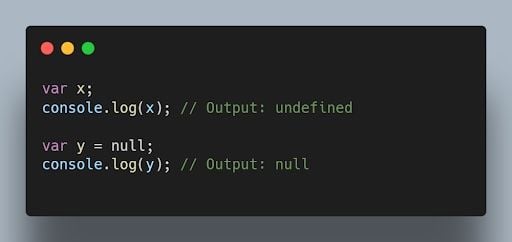
5. Why do we use the word “ debugger ” in JavaScript?
The word “debugger” is used in JavaScript to refer to a tool that can be used to step through JavaScript code line by line. This can be helpful for debugging JavaScript code, which is the process of finding and fixing errors in JavaScript code. To use the debugger , you need to open the JavaScript console in your browser. Then, you can use debugger commands to comb through your code line by line.
It's essential to know debugging techniques as well as the more general ideas behind code optimization and speed improvement. In addition to operating smoothly, efficient code significantly enhances the user experience.
For example, the following code will print the value of the x variable at each step of the debugger .
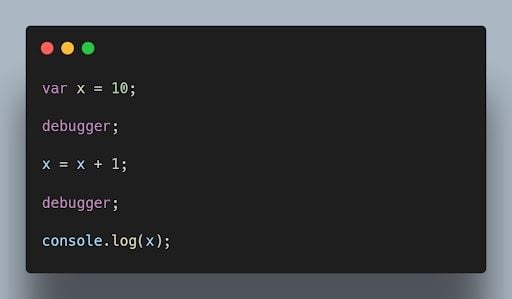
6. What is the purpose of the “ this” keyword in JavaScript?
The this keyword refers to the object that is executing the current function or method. It allows access to object properties and methods within the context of that object.
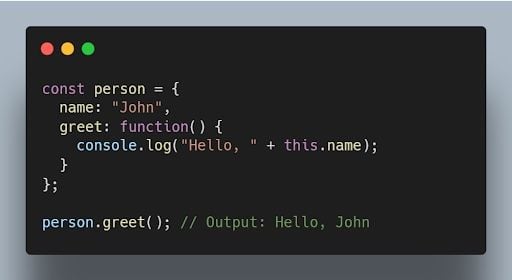
7. What is the difference between == and === operators in JavaScript?
The equality == operator is a comparison operator that compares two values and returns true if they are equal. The strict equality === operator is also a comparison operator, but it compares two values and returns true only if they are equal and of the same type.
For example , the following code will return true, because the values of the x and y variables are equal.
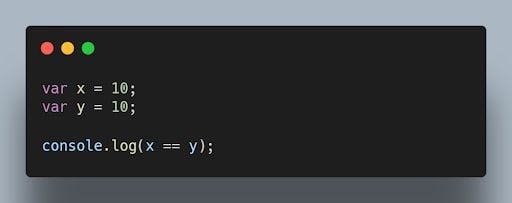
8. What is the difference between “ var” and “ let” keywords in JavaScript?
The var and let keywords are both used to declare variables in JavaScript. However, there are some key differences between the two keywords.
The var keyword declares a global variable, which means that the variable can be accessed from anywhere in the code. The let keyword declares a local variable, which means that the variable can only be accessed within the block of code where it is declared.
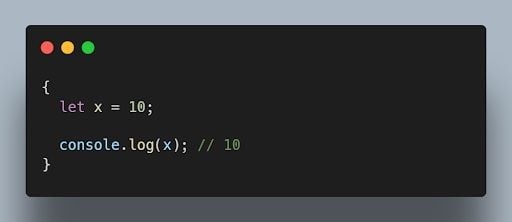
9. What are closures in JavaScript?
Closures ( closureFn ) are functions that have access to variables from an outer function even after the outer function has finished executing. They “remember” the environment in which they were created.
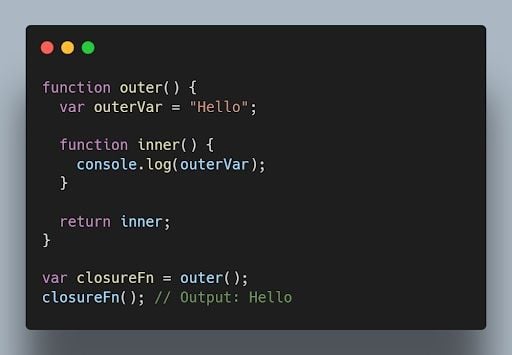
10. What is event delegation in JavaScript?
Event delegation is a technique where you attach a single event listener to a parent element, and that event listener handles events occurring on its child elements. It helps optimize performance and reduce memory consumption.
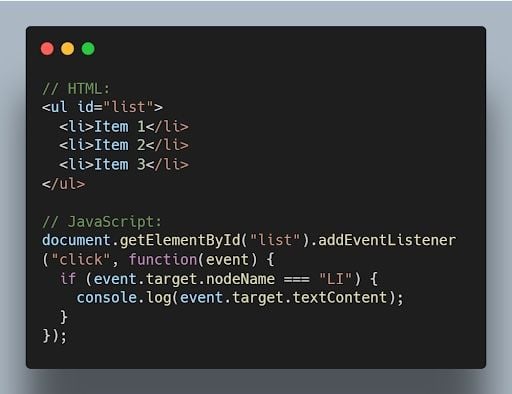
11. What is the difference between “ let” , “ const” , and “ var” ?
let and const were introduced in ES6 and have block scope. let is reassignable, and const is non-reassignable. var is function-scoped and can be redeclared and reassigned throughout the function.
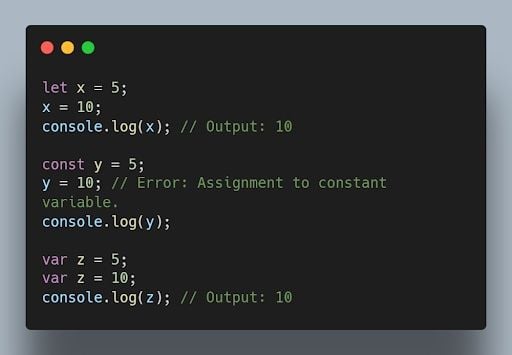
12. What is implicit type coercion in JavaScript?
Implicit type coercion is a JavaScript concept that refers to the process of converting a value from one type to another. I f you try to add a string to a number, JavaScript will implicitly coerce the string to a number before performing the addition operation.
For example, the following code will add the string "10" to the number 5 . This is because JavaScript will implicitly coerce the string "10" to a number before performing the addition operation.
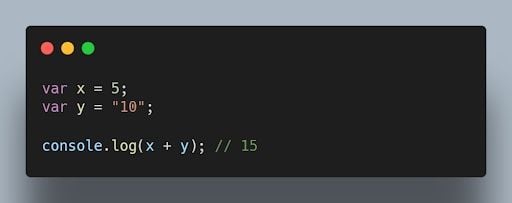
13. Explain the concept of prototypes in JavaScript.
Prototypes are a mechanism used by JavaScript objects for inheritance. Every JavaScript object has a prototype, which provides properties and methods that can be accessed by that object.
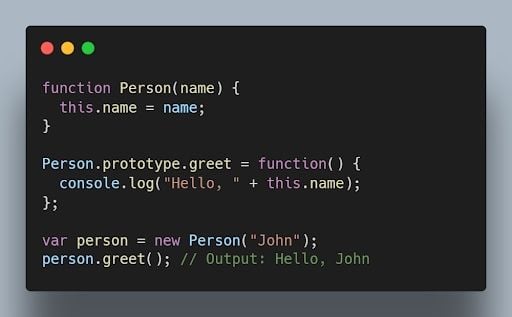
14. What is the output of the following code?
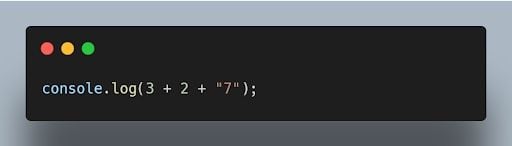
The output will be "57" . The addition operation is performed from left to right, and when a string is encountered, it performs concatenation.
15. How can you clone an object in JavaScript?
There are multiple ways to clone an object in JavaScript. One common method is using the Object.assign() method or the spread operator (...) .
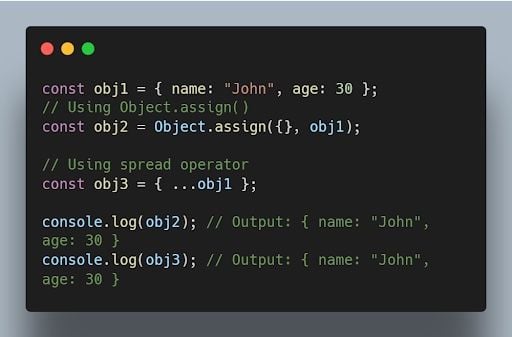
More on JavaScript JavaScript Question Mark (?) Operator Explained
Intermediate Concepts
16. what are higher-order functions in javascript.
Higher order functions are functions that can accept other functions as arguments or return functions as their results. They enable powerful functional programming patterns in JavaScript.
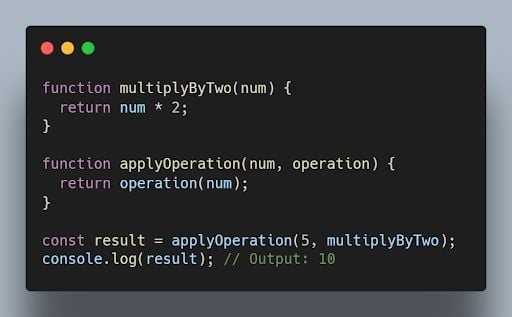
17. What is the purpose of the bind() method in JavaScript?
The bind() method is used to create a new function with a specified this value and an initial set of arguments. It allows you to set the context of a function permanently.
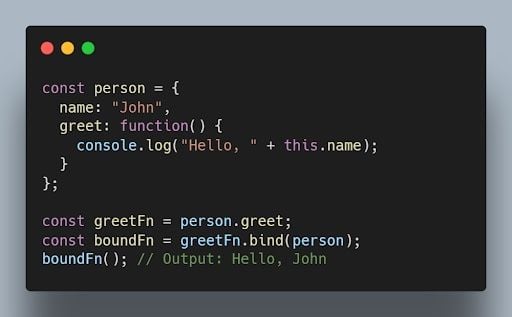
18. What is the difference between function declarations and function expressions?
Function declarations are defined using the function keyword, while function expressions are defined by assigning a function to a variable. Function declarations are hoisted, while function expressions are not.
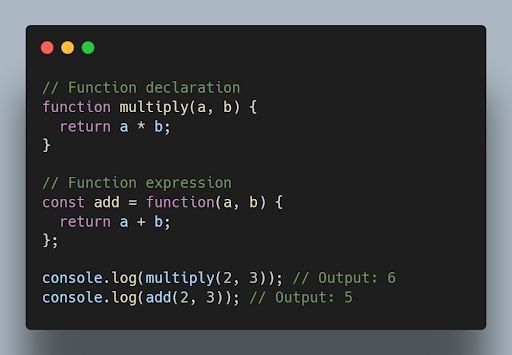
19. What are the different types of errors in JavaScript?
JavaScript can throw a variety of errors, including:
- Syntax errors: These errors occur when the JavaScript code is not syntactically correct.
- Runtime errors: These errors occur when the JavaScript code is executed and there is a problem.
- Logical errors: These errors occur when the JavaScript code does not do what it is supposed to do.
20. What is memoization in JavaScript?
Memoization is a technique that can be used to improve the performance of JavaScript code. Memoization works by storing the results of expensive calculations in a cache. This allows the JavaScript code to avoid re-performing the expensive calculations if the same input is provided again.
For example , the following code calculates the factorial of a number. The factorial of a number is the product of all the positive integers from one to the number.
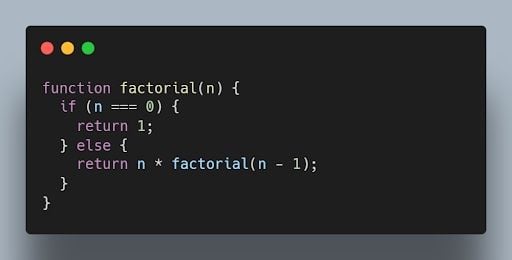
This code can be memoized as follows:
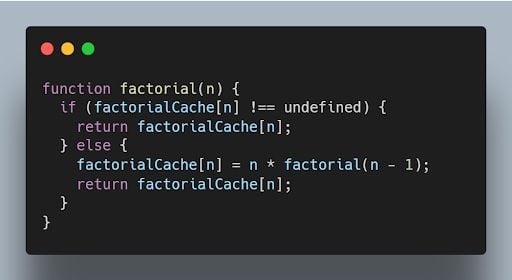
21. What is recursion in JavaScript?
Recursion is a programming technique that allows a function to call itself. Recursion can be used to solve a variety of problems, such as finding the factorial of a number or calculating the Fibonacci sequence .
The following code shows how to use recursion to calculate the factorial of a number:
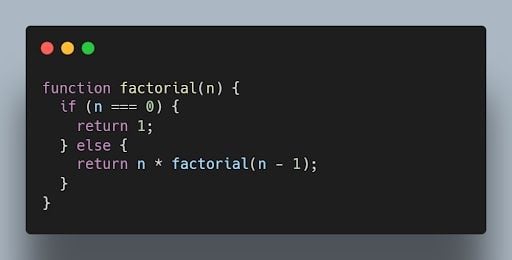
22. What is the use of a constructor function in JavaScript?
A constructor function is a special type of function that is used to create objects. Constructor functions are used to define the properties and methods of an object.
The following code shows how to create a constructor function:
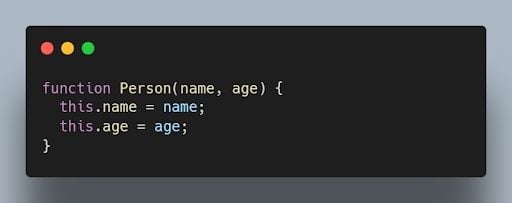
23. What is the difference between a function declaration and a function expression in JavaScript?
A function declaration is a statement that defines a function. A function expression is an expression that evaluates to a function.
The following code shows an example of a function declaration. This code defines a function named factorial. The factorial function calculates the factorial of a number.
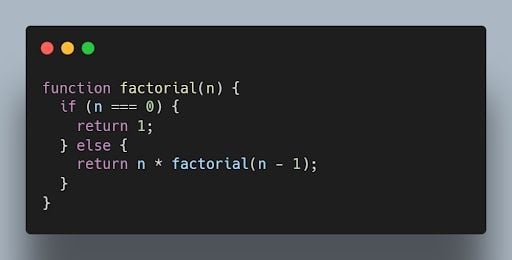
The following code shows an example of a function expression:
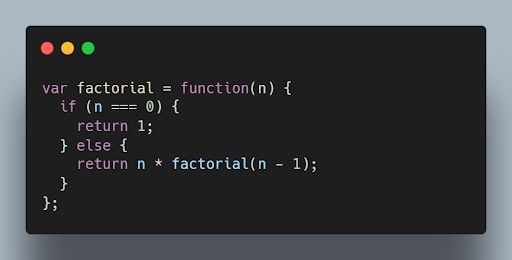
24. What is a callback function in JavaScript?
A callback function is a function passed as an argument to another function, which is then invoked inside the outer function. It allows asynchronous or event-driven programming.
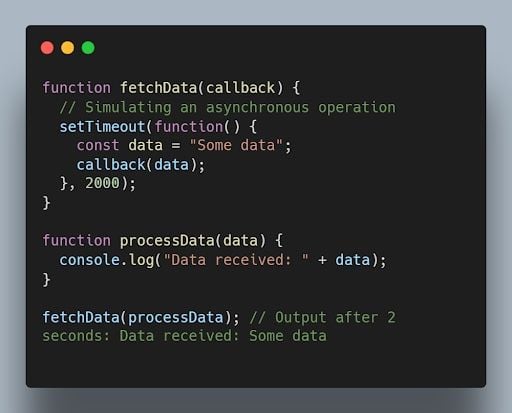
25. What are promises in JavaScript?
Promises are objects used for asynchronous operations. They represent the eventual completion or failure of an asynchronous operation and allow chaining and handling of success or error cases.
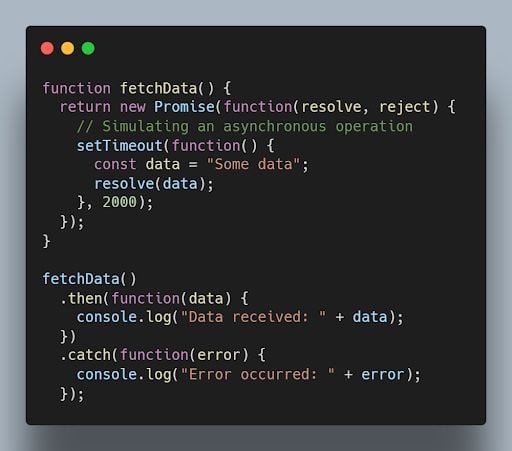
26. What is the difference between synchronous and asynchronous programming?
In synchronous programming, the program execution occurs sequentially, and each statement blocks the execution until it is completed. In asynchronous programming, multiple tasks can be executed concurrently, and the program doesn’t wait for a task to finish before moving to the next one.
Synchronous coding example:
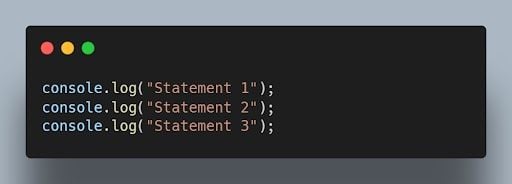
Asynchronous code example:
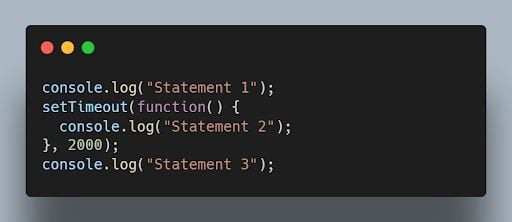
27. How do you handle errors in JavaScript?
Errors in JavaScript can be handled using try - catch blocks. The try block contains the code that may throw an error, and the catch block handles the error and provides an alternative execution path.
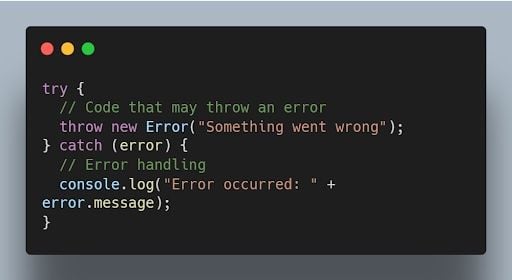
28. Explain the concept of event bubbling in JavaScript.
Event bubbling is the process where an event triggers on a nested element, and then the same event is propagated to its parent elements in the document object model (DOM) tree. It starts from the innermost element and goes up to the document root.
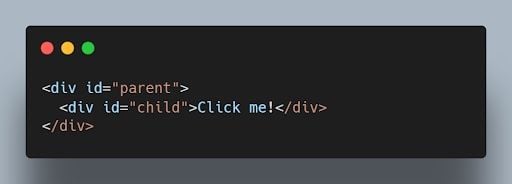
When you click on the child element, both the child and parent event handlers will be triggered, and the output will be:
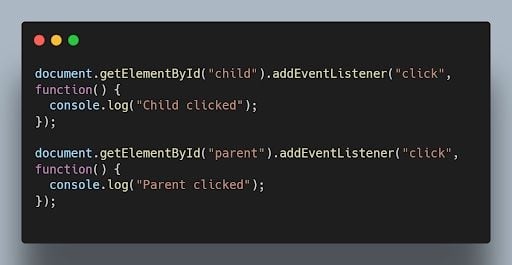
29. What are arrow functions in JavaScript?
Arrow functions are a concise syntax for writing JavaScript functions. They have a more compact syntax compared to traditional function expressions and inherit the this value from their surrounding scope.
For example:
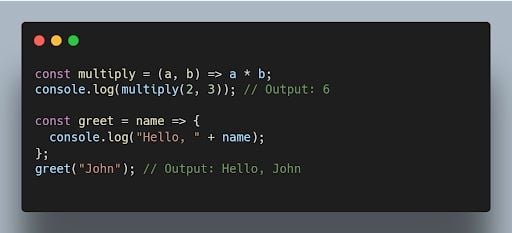
30. What is the difference between querySelector and getElementById ?
querySelector is a more versatile method that allows you to select elements using CSS -like selectors, while getElementById specifically selects an element with the specified ID.
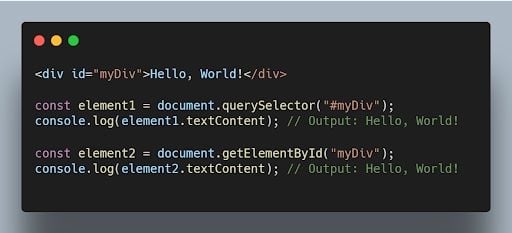
31. What is the purpose of the setTimeout() function in JavaScript?
The setTimeout() function is used to delay the execution of a function or the evaluation of an expression after a specified amount of time in milliseconds.
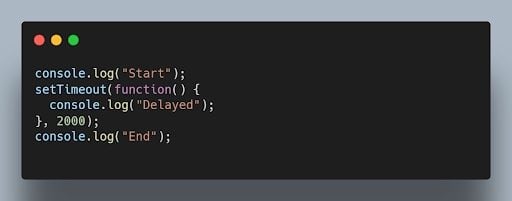
Output after two seconds:
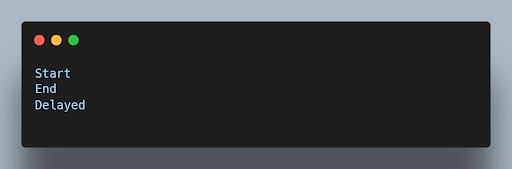
32. What is event delegation and why is it useful?
Event delegation is a technique where you attach a single event listener to a parent element to handle events occurring on its child elements. It’s useful for dynamically created elements or when you have a large number of elements.
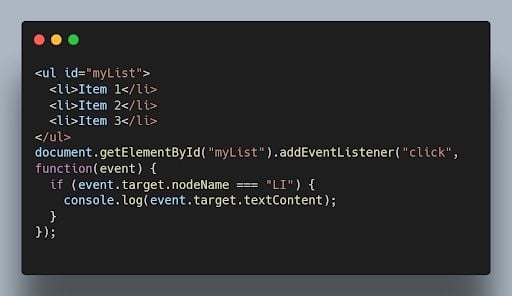
33. How can you prevent the default behavior of an event in JavaScript?
You can use the preventDefault() method on the event object within an event handler to prevent the default behavior associated with that event.
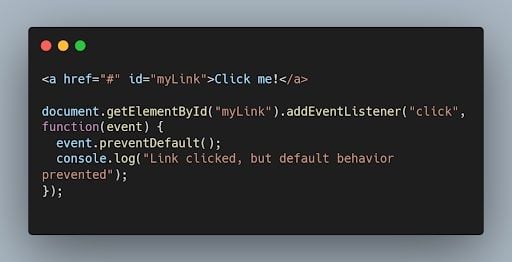
34. What is the difference between localStorage and sessionStorage in JavaScript?
Both localStorage and sessionStorage are web storage objects in JavaScript, but they have different scopes and lifetimes.
- localStorage persists data even after the browser window is closed and is accessible across different browser tabs/windows of the same origin.
- sessionStorage stores data for a single browser session and is accessible only within the same tab or window.
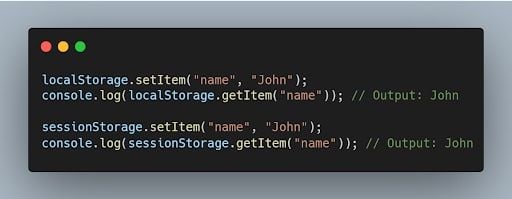
35. How can you convert a string to lowercase in JavaScript?
You can use the toLowerCase() method to convert a string to lowercase in JavaScript.
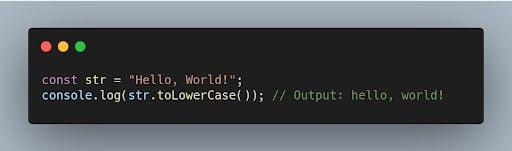
Advanced Concepts
36. what is the purpose of the map() function in javascript.
The map() function is used to iterate over an array and apply a transformation or computation on each element. It returns a new array with the results of the transformation.
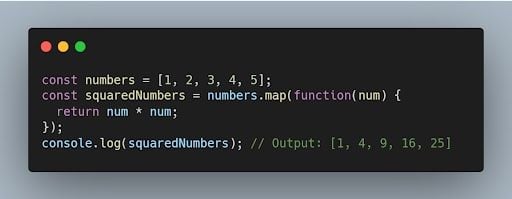
37. What is the difference between splice() and slice() ?
- splice() is used to modify an array by adding, removing, or replacing elements at a specific position.
- slice() is used to create a new array that contains a portion of an existing array, specified by the starting and ending indices.
Example of splice() :
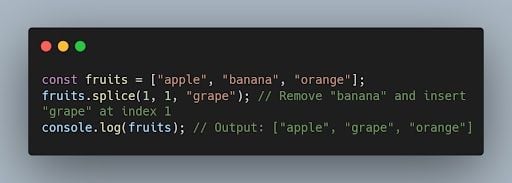
Example of slice() :
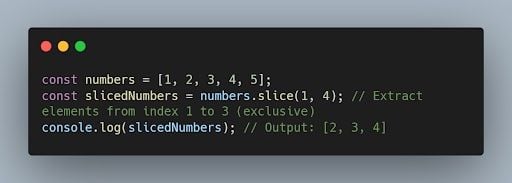
38. What is the purpose of the reduce() function in JavaScript?
The reduce() function is used to reduce an array to a single value by applying a function to each element and accumulating the result.
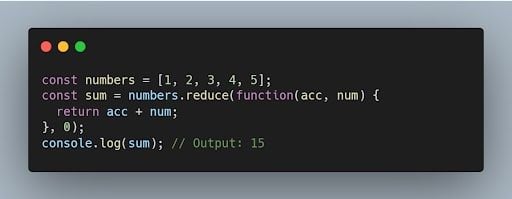
39. How can you check if an array includes a certain value in JavaScript?
You can use the includes() method to check if an array includes a specific value. It returns true if the value is found, and false otherwise.
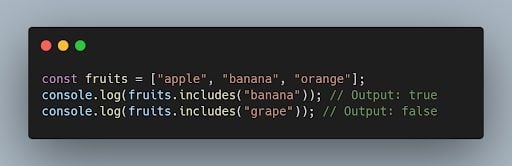
40. What is the difference between prototype and instance properties in JavaScript?
A prototype property is a property that is defined on the prototype object of a constructor function. Instance properties are properties that are defined on individual objects that are created by a constructor function.
Prototype properties are shared by all objects that are created by a constructor function. Instance properties are not shared by other objects.
41. What is the difference between an array and an object in JavaScript?
An array is a data structure that can store a collection of values. An object is a data structure that can store a collection of properties.
Arrays are indexed by numbers. Objects are indexed by strings. Arrays can only store primitive data types and objects. Objects can store primitive data types, objects and arrays.
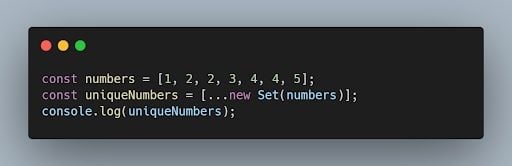
42. How can you remove duplicates from an array in JavaScript?
One way to remove duplicates from an array is by using the Set object or by using the filter() method with the indexOf() method.
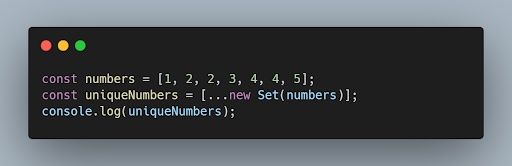
43. What is the purpose of the fetch() function in JavaScript?
The fetch() function is used to make asynchronous HTTP requests in JavaScript. It returns a Promise that resolves to the response from the server.
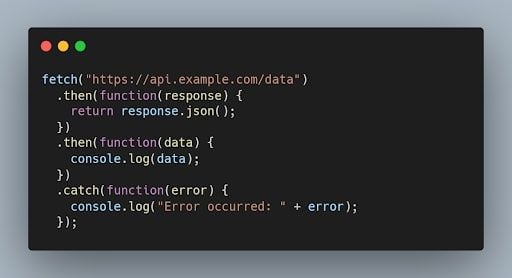
44. What is a generator function in JavaScript?
A generator function is a special type of function that can be paused and resumed during its execution. It allows generating a sequence of values over time, using the yield keyword.
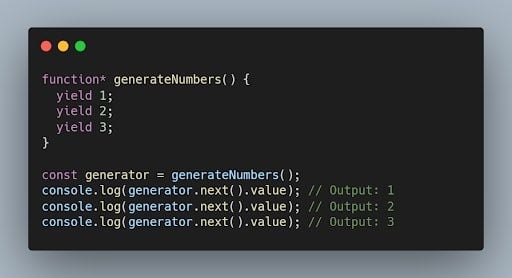
45. What are the different events in JavaScript?
There are many different events in JavaScript, but some of the most common events include:
- Click : The click event occurs when a user clicks on an HTML element.
- Mouseover : The mouseover event occurs when a user's mouse pointer moves over an HTML element.
- Keydown : The keydown event occurs when a user presses a key on the keyboard.
- Keyup : The keyup event occurs when a user releases a key on the keyboard.
- Change : The change event occurs when a user changes the value of an HTML input element.
46. What are the different ways to access an HTML element in JavaScript?
There are three main ways to access an HTML element in JavaScript:
- Using the getElementById() method: The getElementById() method takes a string as an argument and returns the HTML element with the specified ID.
- Using the getElementsByTagName() method: The getElementsByTagName() method takes a string as an argument and returns an array of all the HTML elements with the specified tag name.
- Using the querySelector() method : The querySelector() method takes a CSS selector as an argument and returns the first HTML element that matches the selector.
47. What is the scope of a variable in JavaScript?
The scope of a variable in JavaScript is the part of the code where the variable can be accessed. Variables declared with the var keyword have a local scope, which means that they can only be accessed within the block of code where they are declared. Variables declared with the let keyword have a block scope, which means that they can only be accessed within the block of code where they are declared and any nested blocks. Variables declared with the const keyword have a global scope, which means that they can be accessed from anywhere in the code.
48. What are the different ways to create objects in JavaScript?
There are multiple ways to create objects in JavaScript, including object literals, constructor functions, the Object.create() method and the class syntax introduced in ECMAScript 2015 (ES6).
Example using object literals:
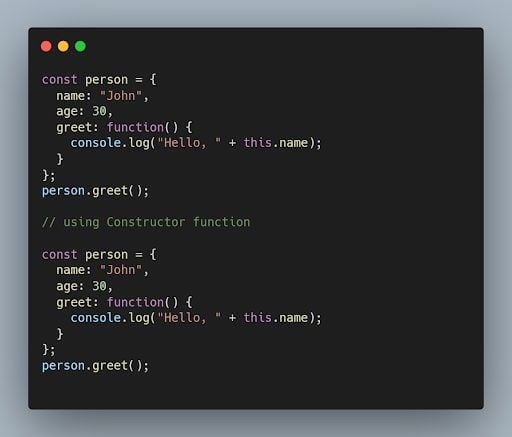
49. What is the purpose of the window object in JavaScript?
The window object represents the browser window. The window object can be used to access the browser’s features, such as the location bar, the status bar and the bookmarks bar.
50. What is the purpose of the async and await keywords in JavaScript?
The async and await keywords are used for handling asynchronous operations in a more synchronous-like manner. The async keyword is used to define an asynchronous function, and the await keyword is used to pause the execution of an async function until a promise is fulfilled or rejected.
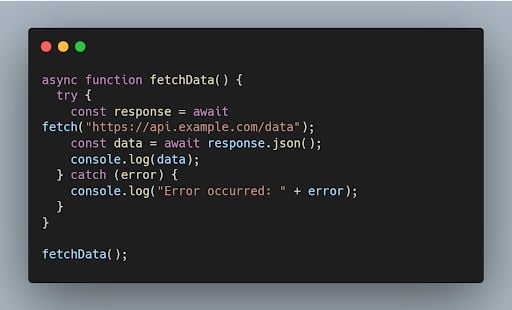
More on JavaScript JavaScript PreventExtensions Method Explained
How to Prepare for a JavaScript Interview
In order to ace a JavaScript interview, you need to be ready for anything. It’s important to practice your code, but you should also be able to clearly explain how different functions work, have real world experience working in JavaScript and understand how to debug.
7 Ways to Prepare for a JavaScript Interview
- Review JavaScript fundamentals.
- Master key concepts.
- Study common interview questions.
- Master debugging skills.
- Practice coding.
- Build projects.
- Mock interviews.
Fortunately, there are some basic steps you can take to be prepared and stand out from other applicants.
1. Review JavaScript Fundamentals
Make sure you are well-versed in the foundational concepts of JavaScript, such as data types , variables , operators, control structures, functions and objects .
2. Master Key Concepts
It’s also important to study up on key JavaScript topics like promises, asynchronous programming , hoisting, scope, closures, prototypes and ES6 features. You should understand how each of these works.
3. Study Common Interview Topics
Take the time to review JavaScript interview questions that are regularly asked, including those about closures, prototypes, callbacks, promises, AJAX (asynchronous JavaScript and XML), error handling and module systems. Most interviews follow a similar pattern. Preparing answers for those questions will help you stand out from other candidates.
4. Master Debugging Skills
Interviewers for JavaScript will often look to assess your code debugging skills. Practice using IDEs or browser developer tools to find and correct common JavaScript code issues. Learn how to read error messages and review basic debugging techniques.
5. Practice Coding
To develop your coding abilities, complete coding tasks and challenges. Concentrate on standard JavaScript data structures and algorithms such arrays, strings, objects, recursion and sorting techniques.
Online resources like LeetCode , CodeChef and HackerRank can be used to practice coding and get ready for interviews. These websites provide a wide variety of coding puzzles and challenges covering a range of subjects and levels of complexity. They are great resources for developing your coding expertise, problem-solving skills, and algorithmic thinking, all of which are necessary for acing technical interviews.
6. Build Projects
Take on modest JavaScript projects to get experience and show that you can create useful applications. Showing off your portfolio at the interview is also beneficial. In addition, developers can also work on JavaScript projects to obtain practical experience and show that they are capable of building effective applications. A diversified portfolio can be quite helpful when applying for jobs. Platforms like LeetCode, CodeChef, HackerRank and others enable users to develop projects gradually, starting with minor ones and eventually progressing to more ambitious ones.
7. Mock Interviews
With a friend or mentor, practice mock interviews paying particular attention to both behavioral and technical components. This enables you to hear useful criticism and become accustomed to the interview process.
It’s not just mastering the technical aspect of JavaScript, it’s about your body language and how you explain your answers. Many companies are also assessing your ability to work within a team and pair program . The better you can explain your actions and thought process, the more likely you’ll be to win over the interviewer.
Built In’s expert contributor network publishes thoughtful, solutions-oriented stories written by innovative tech professionals. It is the tech industry’s definitive destination for sharing compelling, first-person accounts of problem-solving on the road to innovation.
Great Companies Need Great People. That's Where We Come In.
Free Javascript challenges
Learn Javascript online by solving coding exercises.
Javascript for all levels
Solve Javascript tasks from beginner to advanced levels.
Accross various subjects
Select your topic of interest and start practicing.
Start your learning path here
Why jschallenger, a hands-on javascript experience.
JSchallenger provides a variety of JavaScript exercises, including coding tasks, coding challenges, lessons, and quizzes.
Structured learning path
JSchallenger provides a structured learning path that gradually increases in complexity. Build your skills and knowledge at your own pace.
Build a learning streak
JSchallenger saves your learning progress. This feature helps to stay motivated and makes it easy for you to pick up where you left off.
Type and execute code yourself
Type real JavaScript and see the output of your code. JSchallenger provides syntax highlighting for easy understanding.
Join 1.000s of users around the world
Most popular challenges, most failed challenges, what users say about jschallenger.
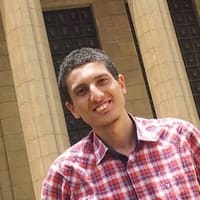
Mohamed Ibrahim
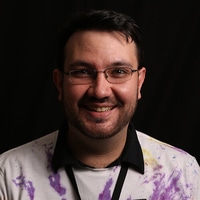
Tobin Shields
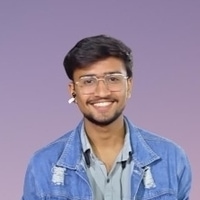
Practice 146 exercises in JavaScript
Learn and practice JavaScript by completing 146 exercises that explore different concepts and ideas.
Explore the JavaScript exercises on Exercism
Unlock more exercises as you progress. They’re great practice and fun to do!
Top 30 JavaScript Interview Questions + Answers (with Code Snippets)
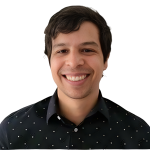
Jacinto Wong
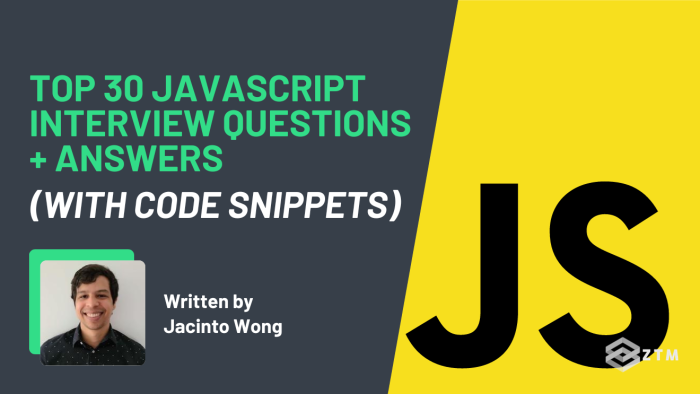
In This Guide:
Beginner javascript interview questions, intermediate javascript interview questions, advanced javascript interview questions, so how did you do.
Are you preparing for a job interview as a front-end developer, and want to brush up on your knowledge?
Well, good news! In this guide, I’m going to share 30 of the most common and relevant interview questions related to JavaScript.
And better still? For each question, I've provided a concise and easy-to-understand answer, along with a code snippet that illustrates the concept in action. This means, no more awkward situations of actually knowing an answer, but not being able to clearly explain it!
Ideally, you should know the answers to all these questions before sitting your interview, and just use this guide as a reminder / last minute prep.
However, if you find that you’re really struggling to understand some of the concepts, then be sure to check out Andrei’s complete Web Developer bootcamp to get a better understanding of the core elements of JavaScript, as well as his advanced JavaScript concepts course .
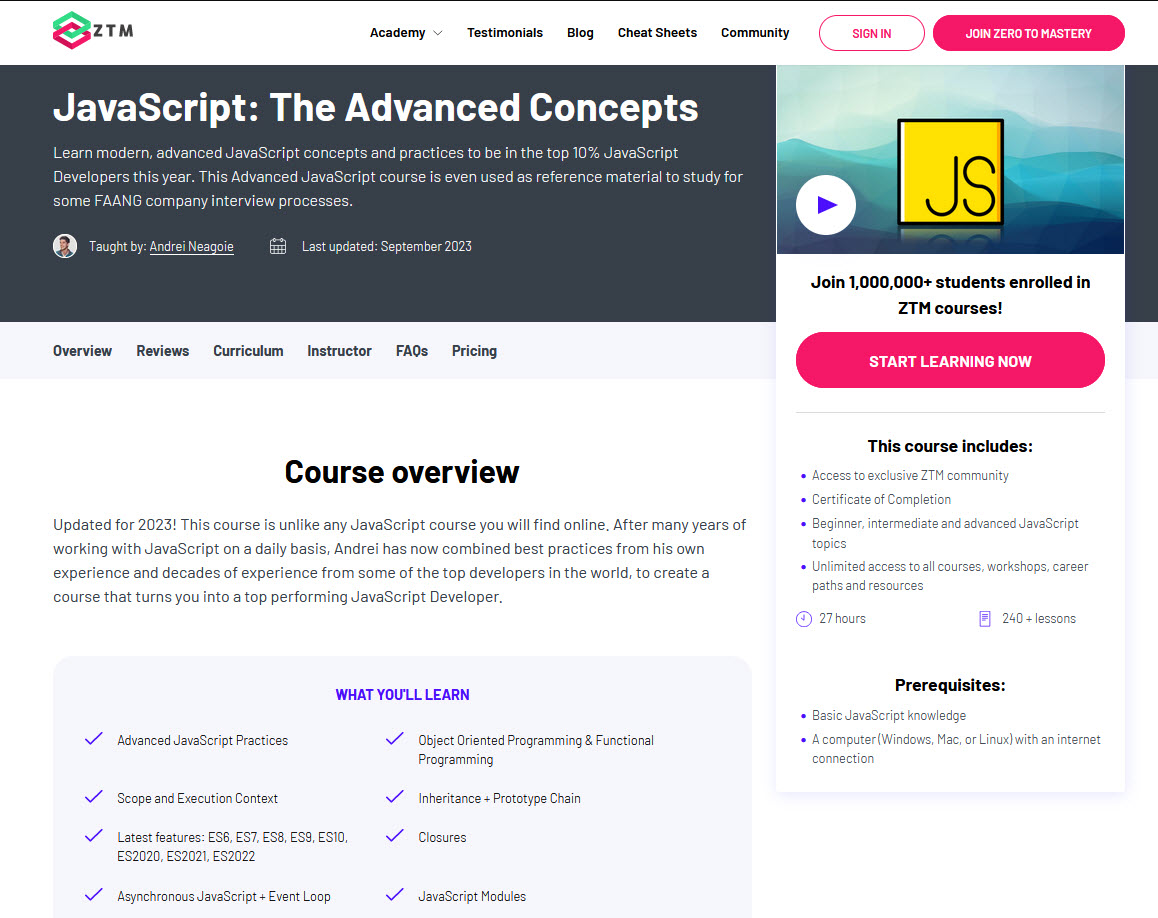
Also, if you want to put these principles into practice and build a stand out portfolio, then check out my top 20 JavaScript projects course .
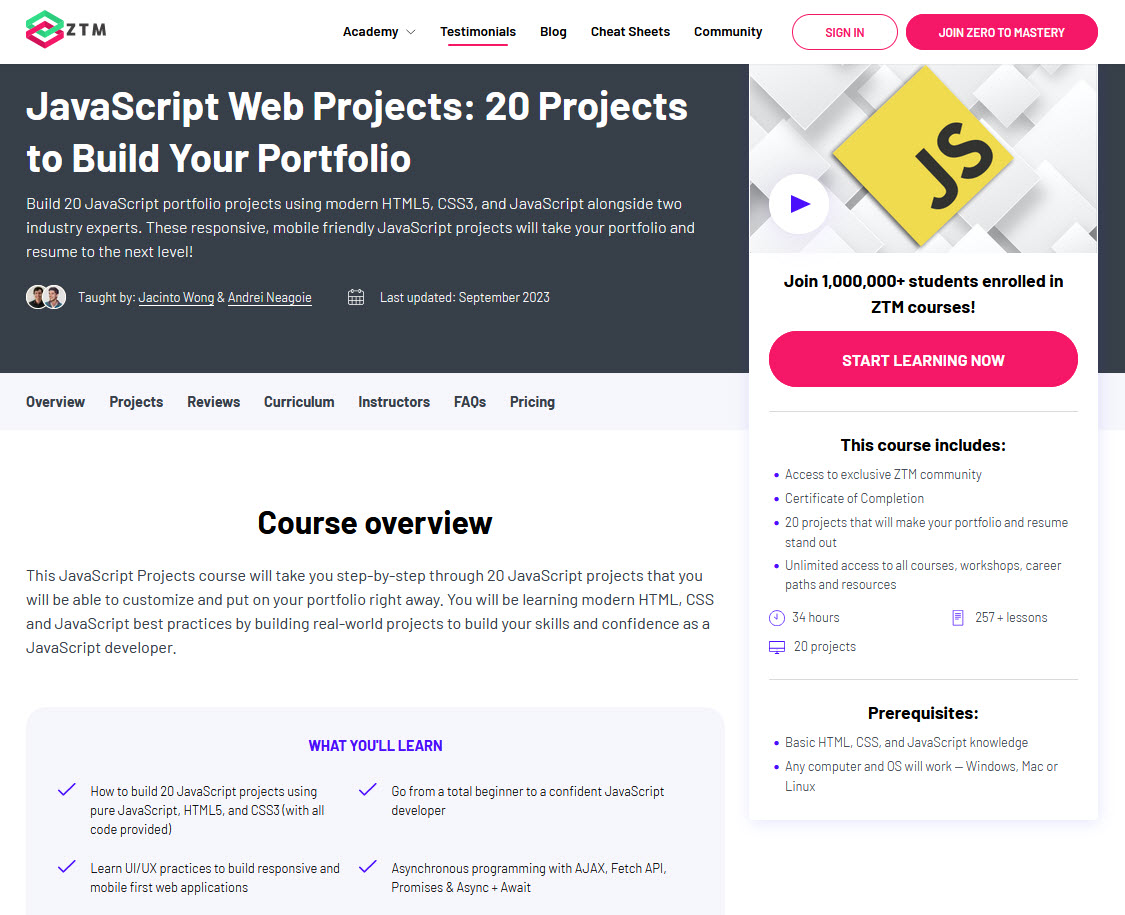
Those 3 resources will not only cover any issues that you may encounter while learning this language, but they’ll also get you to the level where interviewers are seriously blown away with your skills.
(No joke, you can go from trying to get hired, to recruiters actively trying to bring you in instead!)

Anyways, with all that out of the way, let’s get into these interview questions!
Some of these questions will seem super basic or obvious, and you might think “ Why on earth would an interviewer ever ask these of me? ”.
The simple fact is that some initial interviews are done by non-technical people first as a quality filter, and they might ask simple questions around the language.
This means that it's worth being able to answer the basics very confidently and concisely to make sure you get past the gatekeeper and advance through to a 2nd interview with team leads where you'll get more advanced questions and/or live coding tests.
#1. What is JavaScript? What are some of its core ‘stand-out’ features?
JavaScript (also commonly known as JS), is a high-level programming language that is used to create interactive elements on the web.
One of the cool things about JavaScript is that it can change the content of a web page without needing to reload the whole thing.
For example: when you click on a button on a website and something happens, such as a new message appearing, that's often because of JavaScript, and it leads to a much better user experience.
JavaScript also has some other special features that make it powerful:
- It can ‘handle events’, which means it can respond to things like mouse clicks and keystrokes
- It can create objects, which are like containers that can hold data and perform actions
- JavaScript is also flexible in how it handles data. Unlike some other programming languages, you don't need to specify what kind of data a variable will hold. For example, you could create a variable called "x" and set it equal to a number, a string of text, or even an entire object
#2. What is the difference between let and var ?
In JavaScript, both let and var are used to declare variables, but they have some key differences in how they are scoped.
For example
Variables declared with var are function-scoped , meaning that they are visible throughout the entire function in which they are declared.
While variables declared with let are block-scoped , meaning that they are visible only within the block in which they are declared. (A block is typically defined by curly braces, such as in a loop or an if statement).
Another practical difference between let and var is that using let can help avoid bugs caused by variable hoisting .
(Variable hoisting is a behavior of JavaScript where variables declared with var are "hoisted" to the top of the function scope, even if they are declared later in the code.)
As you can imagine, this can lead to unexpected results if you are not careful, so here's a walkthrough of what might happen.
In this code snippet, we’ve defined a function called example that declares two variables, x and y .
x is declared with var , while y is declared with let . We then use console.log to output the values of x and y within the if block, and again after the if block.
As you can see in the image above, because x is declared with var , it is visible throughout the entire function, so we can output its value both inside and outside the if block.
However, because y is declared with let , it is only visible within the if block. This means that if you try to output the y value outside the block it will result in an error, because y is not defined in that scope.
#3. What is the difference between null and undefined ?
In JS, null and undefined are both used to represent the absence of a value, but they have different meanings.
null represents a deliberate non-value, often used to indicate that a variable intentionally has no value.
On the other hand, undefined represents a value that has not been initialized, often caused by a variable that has been declared but not assigned a value as in the previous answer.
In this code snippet, we define two variables, x and y . We set x equal to null , which explicitly indicates that x has no value.
We do not set a value for y , which means that it is automatically initialized to undefined.
We then use console.log to compare x and y to null and undefined , respectively.
In both cases, the comparison evaluates to true, indicating that x and y have the expected values.
#4. What is an example of an asynchronous function?
setTimeout is a great example of an asynchronous function in JavaScript.
It’s used to delay the execution of a piece of code by a specified amount of time, and is often used to simulate a long-running operation, such as a network request.
The setTimeout function is called with a delay time of 1000 milliseconds (1 second), and a callback function that outputs the message "Inside setTimeout" to the console.
This means that the message will be delayed by 1 second before it is output to the console.
#5. How would you expect == to behave differently from === ?
Although you might not think it, the amount of equal signs is very important.
Using just one = will assign a value, (which admittedly, is something I’ve done unintentionally once or twice), while == and === are both used to compare values, but they have different rules.
== is known as the "loose equality" operator, because it will try to convert the values being compared to a common type before making the comparison.
On the other hand, === is known as the "strict equality" operator, because it does not allow for type coercion, and requires that the types of the values being compared match exactly.
In this example, we use console.log to compare the values 1 and '1' using both == and === .
As you can see, when we use == , the comparison returns true, because JavaScript converts the string '1' to a number before making the comparison.
However, when we use === , the comparison returns false, because the types of the values do not match exactly.
This demonstrates the importance of understanding the difference between == and === when comparing values in JavaScript.
If you are not careful, using the wrong operator can lead to unpredictable results, especially when dealing with different data types. As a best practice, it is generally recommended to use === for strict equality comparisons whenever possible.
#6. What are the benefits of using a ternary operator?
The ternary operator is a shorthand way of writing an if statement that has a single expression for both the true and false cases.
Also, the ternary operator can make your code more concise and easier to read, especially in cases where you need to make a simple comparison and return a value based on the result.
However, it's important to note that the ternary operator is not always the best choice for every situation, and in some cases, an if statement may be more appropriate.
In the image above, you can see how both the ternary operator and if statements can be used to achieve the same result, and how you can choose the appropriate approach based on the specific requirements of your code.
In general though, the ternary operator is a good choice for simple comparisons that return a single value, while if statements are better suited for more complex logic or multiple expressions.
#7. What is a template literal and how is it used?
A template literal allows for string interpolation, which means that you can embed expressions inside the string literal.
This can be useful for creating more dynamic and flexible strings, such as HTML or SQL queries , where you need to include dynamic values or expressions.
In this code snippet, we declare a variable num and set its value to 42.
We then use a template literal to create a string that includes the value of the num variable.
(The syntax for a template literal is to enclose the string in backticks instead of single or double quotes, and to use ${} to embed expressions inside the string).
You can use template literals to create more readable and maintainable code, by avoiding the need for concatenation or complex string manipulation.
This is especially useful when you are giving some kind of alert to the user about updating a record, you can always pass the name of whatever they just updated dynamically.
#8. Why is it better to use textContent instead of innerHTML ?
Both textContent and innerHTML are used to manipulate the contents of an HTML element. However, there are some important differences between the two that make textContent a better choice.
The main difference between textContent and innerHTML is that textContent sets or retrieves only the text content of an element, while innerHTML sets or retrieves both the HTML content and the text content of an element.
This means that because textContent only deals with the text content of an element, it is generally faster and safer to use than innerHTML .
Not only that, but it's also more secure, thanks to the fact that textContent does not parse HTML tags or execute scripts, which can lead to security vulnerabilities and performance issues when using innerHTML .
In general, it is a good practice to use textContent whenever you need to manipulate the text content of an element, and to use innerHTML only when you need to manipulate the HTML content of an element.
#9. What are some common libraries and frameworks used in JavaScript?
Everyone will have a different answer if asked what their library or framework of choice is. I’m personally most familiar with Angular, after starting out learning React.
Both of these (and many others) are somewhat similar and at their core in that they are all JavaScript. They simply allow you to automate more of the process, using and re-using pre-built components, services, etc.
jQuery is a widely used library that makes it easier to manipulate HTML documents, handle events, and create animations.
Developers can write less code and achieve more functionality in some cases. It is actually easier in the sense that you can target any HTML element by almost any property and interact with it.
React is a library that enables developers to build fast, scalable, and dynamic user interfaces.
It is often used for creating single-page applications, mobile apps, and other interactive web experiences. Originally invented by Facebook as they were scaling their platform.
Angular is a popular framework that is well-documented and has a lot of tooling that automates things like making a progressive web app using the Angular CLI.
Google maintains it, and has a large and active community of developers contributing to its development.
There are many other libraries and frameworks available in JavaScript, each with their own strengths and weaknesses.
At the end of the day, your choice of library or framework depends mainly on your specific project requirements, your familiarity with the tool, and the overall community support and development behind it.
Andrei does a great job breaking down the pros and cons of React vs. Angular vs. Vue in this article here , if you want to dive deeper into three of the most popular JavaScript frameworks.
#10. How do you keep up with the latest developments in JavaScript?
Interviewers are trying to guage your curiosity and interest level. Generally speaking, people who truly "geek out" will end up being more successful than people who just want a job.
And since the industry evolves and changes so fast, you constantly need to be learning new things.
So it's important to show interviews how you're staying up-to-date.
There are a few different options:
- Reading popular blogs (ex: JavaScript Weekly, Hackernoon, etc.) and newsletters is the easiest way (ex: Andrei's Web Developer Monthly newsletter )
- Attending conferences and meetups is a great way to meet other developers and learn new things
- There are many online communities for JavaScript developers , including forums, chat rooms, and social media groups
- Applying what you learn in new projects! It’s not a new source, but showing that you're actually trying out new things on your own projects (and being able to explain why they are good or not good) is infinitely more valuable than only knowing what they are
#11. What are higher-order functions? Can you give an example?
A higher-order function takes one or more functions as arguments, or returns a function as the result, which allows for more concise and reusable code.
There are many ways to use higher order functions, but they are commonly used in functional programming, as well as in popular libraries and frameworks like React and Redux.
multiplyBy is a higher-order function that returns a new function that multiplies a given number, while the returned function is a closure that has access to the factor argument even after the multiplyBy function has returned.
We then use the multiplyBy function to create two new functions, double and triple, that multiply a given number by 2 and 3.
We can then call these functions with a number argument to get the result.
#12. What's the difference between a callback function and a Promise?
In JavaScript, a callback function is a function that is passed as an argument to another function and is called when that function has completed its task.
A Promise, on the other hand, is an object that represents the eventual completion or failure of an asynchronous operation, and allows for more flexibility when handling an error by using the built-in .catch() method.
In both examples, we are using the hard-coded data array as the value, but you would usually have a GET call instead that is returning data.
In the first example, it would receive this data and then execute the callback function once complete to console.log the output in this case.
But in the second example, you would have some logic to decide whether or not to resolve or reject the promise. The benefit of the promise is that it has built-in methods to catch the error or execute the console.log when data is returned.
#13. How does the ‘this’ keyword work?
In JavaScript, the this keyword refers to the object that the current function or method is a property of, or the object that the function is invoked on, and can have different values depending on how a function is called.
In this example, we are able to pass the name John correctly and the this keyword refers to the person object.
However, when we try to assign the sayHello method to the variable greet, it becomes a stand-alone function and the name becomes undefined. The reason is that this refers to the global variable and not the person object the sayHello function is a method of.
This is something I’d commonly use in Angular to define global variables, created above the constructor, which are very helpful in allowing for different functions to share the same value.
#14. Explain the concept of prototypal inheritance and why you might use it
Prototypal inheritance is a mechanism where an object can inherit properties and methods from another prototype object. This means that every object has a prototype, which is an object that serves as a template for the new object…
Let me clear this up with an example.
Here you can see that animal is a prototype object and the dog object is inheriting the type property, as well as the eat method.
And because the dog object is created using Object.create , the type is then passed and when the dog.eat() method is called, it is able to console.log the type correctly, as so:
“The dog is eating”.
The benefits of using prototype objects like this is that it allows you to create hierarchies and reuse code in a more flexible and modular way.
#15. Can you give an example of when you would use map() , filter() , reduce() ?
map() , filter() , and reduce() are three commonly used array methods that allow developers to work with arrays in a more concise and functional way.
map() example
map() is used to create a new array by mapping each element of an existing array to a new value using a callback function, which takes three arguments:
- the current element
- the index of the current element, and
- the original array
Here's an example of using map() to create a new array of squared values:
filter() example
filter() is used to create a new array containing only the elements of an existing array that pass a certain condition, as determined by a callback function.
Note: The callback function takes the same three arguments as the map() method.
Here's an example of using filter() to create a new array of even numbers:
reduce() example
reduce() is used to reduce an array to a single value by applying a callback function to each element of the array, accumulating the results as it goes.
The callback function takes four arguments:
- the accumulator (which holds the current accumulated value)
Here's an example of using reduce() to find the sum of an array of numbers:
#16. What are the different ways to create an object? Which one do you prefer?
There are several ways to create objects and the simplest and most used method is object literals. They are created with curly braces ‘{}’ and include key-value pairs separated by ‘:’ as below:
Another option, introduced in ES6 is the ability to use class syntax. This is an option with more flexibility as it allows you to dynamically pass values.
In the example below, we’re passing the name and age separately, meaning this could be re-used in this case for many users.
The choice of which option to use is more personal preference, but I believe that if your goal is reusability then using the class syntax is the way to go .
#17. What is an example of how errors can be handled?
There is more than one way for errors to be handled, so here are a few methods.
Option #1. Catch blocks
Catch blocks are used for errors that occur during the execution of a method.
If there is an error in the try block, it will automatically pass the error to the next part of the function, in this case, console.logging it, but in the real world, you’d probably have a pop-up message for the users.
Option #2. Error objects
Another example are error objects, which are used to represent and handle errors.
They have properties, including ‘name’ and ‘message’ and can be customized to give more specific information.
The below example is using the ‘message’ property.
#18. When would you use a for loop or a forEach loop?
For loops and forEach loops are both used to iterate through arrays and perform some operation on each item within the array.
For loops use a counter variable, in this case represented by the ‘i’ variable.
This does make it easier to always pass this value as an index within the function itself. It also allows you to more easily go backwards through the loop, or it’s helpful if you have to loop through multiple arrays at once. (The console log would happen 5 separate times with a new number each time).
In this example, the console output is the same using a forEach loop, but you can see that the code is a little more concise, we don’t have to worry about adding the length of the array and iterating the index.
Ultimately, for loops are useful when you need to loop over an array and perform complex operations on each element, such as sorting or filtering, while ForEach loops are useful when you need to perform a simple operation on each element, such as logging or modifying the values.
#19. What is the difference between the setTimeout() and setInterval() methods?
The setTimeout() and setInterval() methods are like JavaScript's in-house timekeepers, helping you execute code after a certain amount of time has passed.
Although they share some similarities, there are key differences between these two methods.
setTimeout() swoops in to run a function just once after a specified number of milliseconds. It needs two arguments: the function to run and the milliseconds to wait before doing so.
In our example, setTimeout() is called to run the greet() function after a 1000-millisecond pause (aka one second). The function only gets executed once.
On the other hand, setInterval() is the go-to method for running a function repeatedly at a specified interval. It also takes two arguments: the function to run and the milliseconds to wait between each execution.
In this case, setInterval() is used to run the incrementCount() function every 1000 milliseconds (yes, one second again). The function keeps going, upping the count variable each time.
The key distinction between setTimeout() and setInterval() is that the first runs the specified function just once, while the latter keeps the party going at the specified interval.
In general, reach for setTimeout() when you need a function to run after a delay, and setInterval() when you want a function to run repeatedly at a specific interval.
Just remember to use these methods wisely, as overuse can lead to performance hiccups and other headaches.
#20. What is the event loop? How does it work?
The event loop is an important part of the runtime that handles asynchronous code execution in a single-threaded environment.
It works by always looping over two main components:
- the call stack, and
- the task queue
The call stack is a data structure that tracks the execution of function calls. When a function is called, it is added to the top of the call stack, and when it finishes executing, it is removed from the stack.
(The task queue is a data structure that tracks events that have completed, but have not yet been added to the call stack).
These events are typically asynchronous in nature, such as user input or network requests, and so when an asynchronous event occurs, it is added to the task queue.
In this example, we have a setTimeout() function that is set to execute after 0 milliseconds, and a Promise that is resolved immediately. We also have some console.log() statements to track the order of execution.
It starts with executing the ‘Start’ and ‘End’ logs, then the call stack is empty, so the task queue is then checked to find the Promise which gets resolved to output ‘Promise’.
With the call stack empty again, it loops through to find the setTimeout which gets executed last.
#21. Explain the difference between shallowCopy and deepCopy in JavaScript?
When we copy an object or an array, we can create either a shallowCopy or a deepCopy .
The main difference between them is that the shallowCopy of the object will continue to affect the original object, whereas the deepCopy will remain entirely separate.
We can see this occur when we try to modify the nested city property in the image above.
In the shallowCopy , the original city is changed from New York to San Francisco, whereas the deepCopy city of Los Angeles does not affect the original city.
#22. What design pattern do you prefer to use in JavaScript?
Design patterns are like secret recipes for crafting high-quality, manageable, and scalable code.
Among my favorites is the Module Pattern. It’s a powerful design pattern for creating organized, maintainable, and scalable code. It neatly bundles code into reusable modules for various applications or parts of the same app, while also preventing naming collisions, simplifies code, and enhances reusability.
The magic of the Module Pattern lies in the immediately invoked function expression (IIFE), which protects a module's variables and functions from global scope pollution and collisions.
In our example, we have a calculator module with add and subtract functions, which update a private result variable. The getResult method makes this variable public.
Our IIFE bubble wraps the module's code, keeping add and subtract functions hidden from outsiders.
#23. What are generators in JavaScript? Can you give an example of when you would use one?
Generators let developers wield control over JavaScript's flow of iteration, so that we can pause and play on demand, conjuring up a sequence of values over time.
We can implement this by using an asterisk (*) after the function keyword. We can then hit the pause button with the yield keyword, which returns a value and bookmarks the generator's state.
To hit play again, we just use the next() method, picking up where we left off.
Generators shine in various scenarios where we need to unveil a sequence of values over time, such as handling asynchronous data or processing giant datasets.
By letting us pause and play the value generation, generators are our secret weapon for controlling JavaScript's flow of iteration.
For example, we could create a generator function called fibonacci to whip up a sequence of Fibonacci numbers.
With variables a and b set to 0 and 1, our generator dives into an infinite loop, yielding a's current value and updating a and b to the next pair of Fibonacci numbers.
Next, we summon a generator instance using the fibonacci function and store it in a variable named fib. This means that with the next() method, we can stroll through the Fibonacci sequence, starting at 0 and 1.
#24. How would you effectively use every() or some() methods?
The every() and some() methods are like the dynamic duo of JavaScript array methods, helping developers quickly figure out if all or just a few elements in an array meet specific criteria.
They're perfect for writing sleek and efficient code for common array tasks, like searching for a particular value or seeing if all elements pass a certain test.
every() explained
The every() method checks if all elements in an array meet a particular condition.
It uses a callback function that runs for each element, and returns true if the callback is true for all elements, and false otherwise.
In our example, we employ the every() method to see if all elements in the numbers array are greater than zero or greater than three.
The callback function says "yay" (true) if the current element is greater than the given value, and "nay" (false) otherwise.
The every() method gives a thumbs up (true) when all elements pass, and a thumbs down (false) otherwise.
some() explained
The some() method is quite similar to every() , but it's satisfied with just one element in the array meeting the condition.
Here's an example:
In this case, we use the some() method to check if any elements in the numbers array are greater than three or greater than five. The callback function gives a "yup" (true) if the current element is greater than the given value, and "nope" (false) otherwise.
The some() method is content (true) with at least one element meeting the condition, and unhappy (false) otherwise.
To sum it up, the every() and some() methods are great for verifying if all or some elements in an array meet specific conditions. They provide a snappy and effective way to handle common array operations, boosting code readability and minimizing the risk of bugs.
#25. What is the event-driven programming paradigm? How does it work?
JavaScript uses event-driven programming to craft dynamic, interactive user interfaces, where the program's flow is driven by events happening in the system, rather than a fixed set of instructions.
In this paradigm, the program patiently awaits events like a user clicking a button or typing into a form field. When an event pops up, the program jumps into action, executing a predefined function or a callback function to handle the event.
JavaScript leans on the browser's Document Object Model (DOM) to manage events. The DOM is a family tree-like structure that represents the web page and lets you mingle with the different elements on it.
- When an event takes place, say, a user clicking a button, the DOM whips up an event object filled with info about the event, like the target element and event type
- This event object is handed over to a registered callback function
- The callback function then jazzes up the web page in response to the event, such as changing an element's text or updating a form field's value
In our example, we grab a button element using the document.getElementById() method.
Next, we sign up an event listener for the "click" event with the addEventListener() method.
When the button is clicked, the callback function we passed to addEventListener() springs into action, and "Button clicked!" is logged to the console. Huzzah!
All in all, event-driven programming is a powerful way to create lively, interactive web apps in JavaScript. By reacting to events in the system, we can design user interfaces that are responsive, intuitive, and delightful.
#26. What is the difference between a module and a namespace in JavaScript?
Modules and namespaces both offer ways of organizing code and dodging naming collisions, but there are subtle differences.
Modules explained
A module is a self-contained code unit packed with variables, functions, classes, or other code catering to a specific task or feature. Modules are great for breaking a large app into smaller, easier-to-handle pieces and keeping naming collisions at bay.
With ES6, JavaScript rolled out a built-in module system, letting developers effortlessly create, import, and export modules. Just use the export keyword to export a module and the import keyword to bring it in.
Namespaces explained
Namespaces help avoid naming collisions in sprawling applications. They are all about grouping related variables, functions, or objects under one roof.
You can create namespaces using an object literal and even nest them for a hierarchy.
In our example, we whip up a namespace called "MyApp" using an object literal. Next, we add some properties, like a message property and a sayHello() function.
By bundling these properties within a namespace, we dodge naming collisions with other parts of the app.
So, in a nutshell, modules and namespaces both keep JavaScript code organized and naming collisions at arm's length, but they serve distinct purposes. Modules craft self-contained code units that can be imported and exported in one piece, while namespaces corral related variables, functions, or objects under one name.
#27. What are decorators? How do you use them?
Decorators in JavaScript let you tweak the behavior of a class or function using a simple, elegant syntax. And as an added bonus, decorators play nice with classes, methods, and properties.
To use a decorator, just pop the @ symbol in front of the decorator function's name.
In our example, we whip up a decorator function called "log" that logs a message to the console. We then apply the decorator to a class named "MyClass" using the @log syntax.
As MyClass comes to life, the decorator function springs into action, logging "MyClass was called" to the console.
Decorators can also mingle with methods and properties, modifying their behavior. You could conjure a decorator that caches a function's result or one that adds validation to a property.
In another example, we craft a decorator function named "validate" that bestows validation on a property.
The decorator function tweaks the property's descriptor to intercept setter method calls and validate the input. If the input doesn't pass muster, an error is thrown. Next, we apply the decorator to the "name" property of a Person class, ensuring only strings are assigned to the property.
In a nutshell, decorators are a fantastic JavaScript feature that modifies the behavior of classes, methods, and properties in a flexible and reusable manner. With decorators, you can add nifty features like logging, caching, or validation to your code without drowning it in boilerplate.
#28. What is the difference between the async and defer attributes on a <script> tag in HTML?
The async and defer attributes work with HTML's <script> tag to manage script loading and execution.
The async attribute instructs the browser to download the script asynchronously while parsing the rest of the HTML document.
Why do this?
Simply because the script runs as soon as it's downloaded, even if the document hasn't fully loaded. This approach can speed up page load times, especially for non-critical scripts.
Conversely, the defer attribute tells the browser to download the script asynchronously but hold off on execution until the entire HTML document is parsed.
This means the script runs in the order it appears in the HTML document, after the DOM is fully constructed. This method also boosts page load times, particularly for DOM-manipulating scripts.
In essence, the distinction between async and defer lies in script execution timing. Async scripts run as soon as they're downloaded, while defer scripts wait until the DOM is completely constructed.
The choice between the two depends on your script's specific needs and its interaction with the page.
#29. What is the difference between localStorage and sessionStorage ?
localStorage and sessionStorage are JavaScript APIs that let web apps store data client-side, right in the browser, but they do have some key differences.
localStorage explained
localStorage is a persistent storage buddy, hanging onto your data even after you close the browser or restart your computer.
It's great for long-term data like user preferences or settings. Plus, it's a social butterfly, sharing data across tabs and windows in the same browser.
sessionStorage explained
sessionStorage , though, is more of a short-term pal. It sticks around only during the current browsing session, and its data disappears once you close the window or tab.
It's perfect for temporary data, like user input or state data for specific workflows.
They do have some similarities of course.
localStorage and sessionStorage both use key-value pairs and offer similar APIs like setItem() , getItem() , and removeItem() for data manipulation.
The main difference is simply how long the data sticks around.
In short, localStorage is your go-to for long-term storage, while sessionStorage has your back for short-term needs.
#30. What are some common performance optimization techniques for JavaScript, and why would you want to use them?
JavaScript is an amazing language that adds dynamic interactivity to web applications, creating truly engaging experiences. However, it's important to optimize JavaScript code to avoid performance issues, such as slow page load times and unresponsive user interfaces.
The good news is, there are plenty of performance optimization techniques for JavaScript to ensure a silky-smooth experience:
Minification: Make your code lean and mean by stripping away unnecessary characters and whitespace. This results in faster downloads and snappy load times
Bundling: Merge multiple JavaScript files into one, cutting down HTTP requests and reducing your code's overall size for a performance boost
Caching: Store JavaScript files in the browser's cache, enabling reuse on subsequent page loads. This significantly speeds up load times, especially for those returning visitors
Lazy loading: Wait to load JavaScript files until they're truly needed, rather than loading everything upfront. This helps cut down initial load time and delights users with a seamless experience
Code optimization: Fine-tune your JavaScript code to get even more performance gains. Techniques include reducing variable count, minimizing loop usage, and avoiding costly operations like DOM manipulation
Event delegation: Attach event listeners to parent elements instead of individual child elements. This helps keep the number of event listeners in check, giving your application a performance edge
Throttling and debouncing: Keep function execution rates in check with throttling and debouncing. Throttling limits the frequency of function calls, while debouncing delays execution until a specified time has passed since the last call. These techniques help improve performance by cutting down on unnecessary function calls
So there you have it - 30 of the most common JavaScript interview questions that you might face during a frontend developer interview.
Did you answer all 30 correctly? Congratulations! You’re ready to ace that interview.
Or did you struggle with some of them? If you knew the answers but forgot, just give yourself some time to recap before the interview.
Or did none of this make sense?
Not a problem either. As I mentioned earlier, Zero To Mastery has 3 JavaScript courses that can help you up your skills and advanced JS knowledge , so that you blow away any interview questions.
Heck… we even have detailed resources on how to ace the technical interview , how to get hired at FAANG , and even how to get through the door and wow them with your projects!
You’ll not only get access to step-by-step tutorials, but you can ask questions from me and other instructors, as well as other students inside our private Discord .
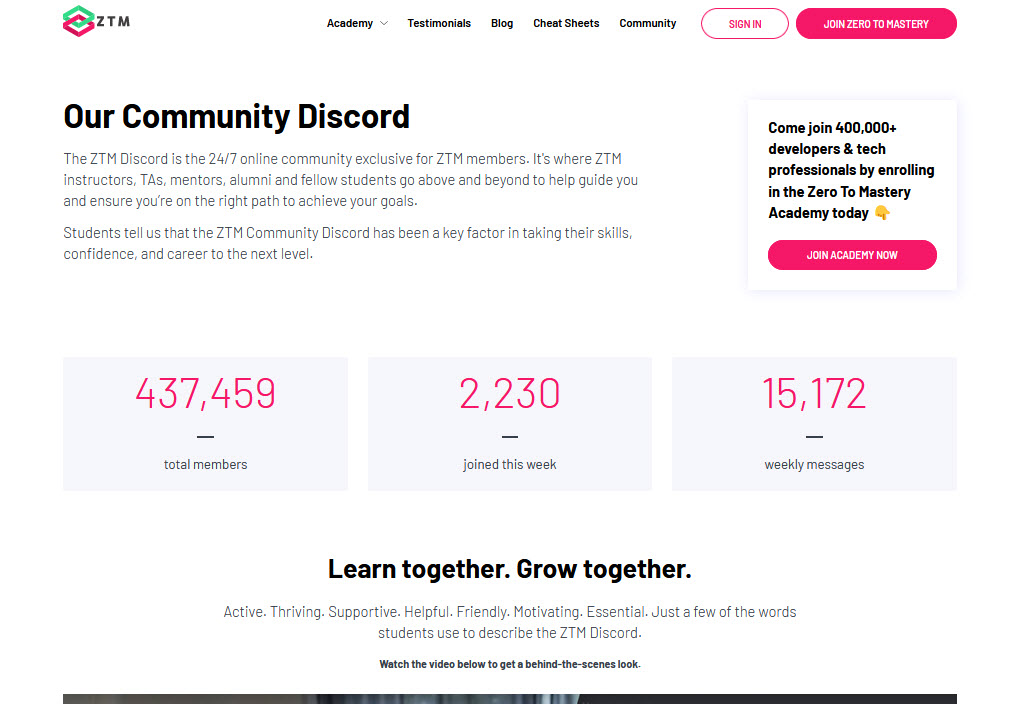
Either way, if you decide to join or not, good luck with your interview and go get that job!
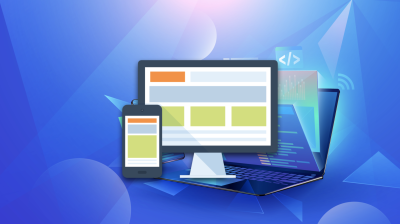
The Complete Web Developer in 2024: Zero to Mastery
Learn to code from scratch and get hired as a Web Developer in 2024. This full-stack coding bootcamp will teach you HTML, CSS, JavaScript, React, Node.js, Machine Learning & more.
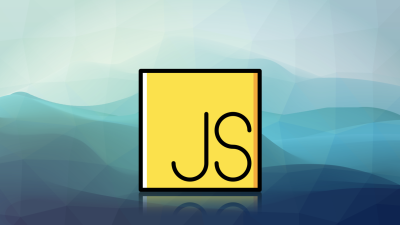
JavaScript: The Advanced Concepts
Learn modern, advanced JavaScript practices to become a top 10% JavaScript Developer. Fun fact: this course is even used as a reference for some FAANG company interview processes.
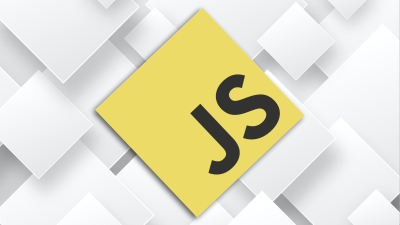
JavaScript Web Projects: 20 Projects to Build Your Portfolio
Get hired or your first client by building your dream portfolio of modern, real-world JavaScript projects. Never spend time on confusing, out of date, incomplete tutorials anymore!
More from Zero To Mastery
![javascript assignment questions [Guide] Computer Science For Beginners preview](https://images.ctfassets.net/aq13lwl6616q/3SYSMGBVgnRvlAY0JiEBOi/7065751f30d315eb4e7fb7f6b2e164c4/Computer_science_for_beginners.png?w=400&h=225&q=50&fm=png&bg=transparent)
You DO NOT need a CS Degree to get hired as a Developer. Learn Computer Sciences Basics today with this free guide by a Senior Dev with 10+ years of experience.

Hey Web Dev - do you want to sharpen your JavaScript skills? Then come join me and let's start building some awesome beginner friendly JavaScript projects to boost your portfolio & skills.

Learning how closures work will with simple examples and real-world cases will make your life a lot easier and save you stress when solving daily problems.
37 Essential JavaScript Interview Questions *
Toptal sourced essential questions that the best javascript developers and engineers can answer. driven from our community, we encourage experts to submit questions and offer feedback..

Interview Questions
What is a potential pitfall with using typeof bar === "object" to determine if bar is an object? How can this pitfall be avoided?
Although typeof bar === "object" is a reliable way of checking if bar is an object, the surprising gotcha in JavaScript is that null is also considered an object!
Therefore, the following code will, to the surprise of most developers, log true (not false ) to the console:
As long as one is aware of this, the problem can easily be avoided by also checking if bar is null :
To be entirely thorough in our answer, there are two other things worth noting:
First, the above solution will return false if bar is a function. In most cases, this is the desired behavior, but in situations where you want to also return true for functions, you could amend the above solution to be:
Second, the above solution will return true if bar is an array (e.g., if var bar = []; ). In most cases, this is the desired behavior, since arrays are indeed objects, but in situations where you want to also false for arrays, you could amend the above solution to be:
However, there’s one other alternative that returns false for nulls, arrays, and functions, but true for objects:
Or, if you’re using jQuery:
ES5 makes the array case quite simple, including its own null check:
What will the code below output to the console and why?
Since both a and b are defined within the enclosing scope of the function, and since the line they are on begins with the var keyword, most JavaScript developers would expect typeof a and typeof b to both be undefined in the above example.
However, that is not the case. The issue here is that most developers incorrectly understand the statement var a = b = 3; to be shorthand for:
But in fact, var a = b = 3; is actually shorthand for:
As a result (if you are not using strict mode), the output of the code snippet would be:
But how can b be defined outside of the scope of the enclosing function? Well, since the statement var a = b = 3; is shorthand for the statements b = 3; and var a = b; , b ends up being a global variable (since it is not preceded by the var keyword) and is therefore still in scope even outside of the enclosing function.
Note that, in strict mode (i.e., with use strict ), the statement var a = b = 3; will generate a runtime error of ReferenceError: b is not defined , thereby avoiding any headfakes/bugs that might othewise result. (Yet another prime example of why you should use use strict as a matter of course in your code!)
The above code will output the following to the console:
In the outer function, both this and self refer to myObject and therefore both can properly reference and access foo .
In the inner function, though, this no longer refers to myObject . As a result, this.foo is undefined in the inner function, whereas the reference to the local variable self remains in scope and is accessible there.
Apply to Join Toptal's Development Network
and enjoy reliable, steady, remote Freelance JavaScript Developer Jobs
What is the significance of, and reason for, wrapping the entire content of a JavaScript source file in a function block?
This is an increasingly common practice, employed by many popular JavaScript libraries (jQuery, Node.js, etc.). This technique creates a closure around the entire contents of the file which, perhaps most importantly, creates a private namespace and thereby helps avoid potential name clashes between different JavaScript modules and libraries.
Another feature of this technique is to allow for an easily referenceable (presumably shorter) alias for a global variable. This is often used, for example, in jQuery plugins. jQuery allows you to disable the $ reference to the jQuery namespace, using jQuery.noConflict() . If this has been done, your code can still use $ employing this closure technique, as follows:
What is the significance, and what are the benefits, of including 'use strict' at the beginning of a JavaScript source file?
The short and most important answer here is that use strict is a way to voluntarily enforce stricter parsing and error handling on your JavaScript code at runtime. Code errors that would otherwise have been ignored or would have failed silently will now generate errors or throw exceptions. In general, it is a good practice.
Some of the key benefits of strict mode include:
- Makes debugging easier. Code errors that would otherwise have been ignored or would have failed silently will now generate errors or throw exceptions, alerting you sooner to problems in your code and directing you more quickly to their source.
- Prevents accidental globals. Without strict mode, assigning a value to an undeclared variable automatically creates a global variable with that name. This is one of the most common errors in JavaScript. In strict mode, attempting to do so throws an error.
- Eliminates this coercion . Without strict mode, a reference to a this value of null or undefined is automatically coerced to the global. This can cause many headfakes and pull-out-your-hair kind of bugs. In strict mode, referencing a a this value of null or undefined throws an error.
- Note: It used to be (in ECMAScript 5) that strict mode would disallow duplicate property names (e.g. var object = {foo: "bar", foo: "baz"}; ) but as of ECMAScript 2015 this is no longer the case.
- Makes eval() safer. There are some differences in the way eval() behaves in strict mode and in non-strict mode. Most significantly, in strict mode, variables and functions declared inside of an eval() statement are not created in the containing scope (they are created in the containing scope in non-strict mode, which can also be a common source of problems).
- Throws error on invalid usage of delete . The delete operator (used to remove properties from objects) cannot be used on non-configurable properties of the object. Non-strict code will fail silently when an attempt is made to delete a non-configurable property, whereas strict mode will throw an error in such a case.
Consider the two functions below. Will they both return the same thing? Why or why not?
Surprisingly, these two functions will not return the same thing. Rather:
will yield:
Not only is this surprising, but what makes this particularly gnarly is that foo2() returns undefined without any error being thrown.
The reason for this has to do with the fact that semicolons are technically optional in JavaScript (although omitting them is generally really bad form). As a result, when the line containing the return statement (with nothing else on the line) is encountered in foo2() , a semicolon is automatically inserted immediately after the return statement.
No error is thrown since the remainder of the code is perfectly valid, even though it doesn’t ever get invoked or do anything (it is simply an unused code block that defines a property bar which is equal to the string "hello" ).
This behavior also argues for following the convention of placing an opening curly brace at the end of a line in JavaScript, rather than on the beginning of a new line. As shown here, this becomes more than just a stylistic preference in JavaScript.
What will the code below output? Explain your answer.
An educated answer to this question would simply be: “You can’t be sure. it might print out 0.3 and true , or it might not. Numbers in JavaScript are all treated with floating point precision, and as such, may not always yield the expected results.”
The example provided above is classic case that demonstrates this issue. Surprisingly, it will print out:
A typical solution is to compare the absolute difference between two numbers with the special constant Number.EPSILON :
In what order will the numbers 1-4 be logged to the console when the code below is executed? Why?
The values will be logged in the following order:
Let’s first explain the parts of this that are presumably more obvious:
1 and 4 are displayed first since they are logged by simple calls to console.log() without any delay
2 is displayed after 3 because 2 is being logged after a delay of 1000 msecs (i.e., 1 second) whereas 3 is being logged after a delay of 0 msecs.
OK, fine. But if 3 is being logged after a delay of 0 msecs, doesn’t that mean that it is being logged right away? And, if so, shouldn’t it be logged before 4 , since 4 is being logged by a later line of code?
The answer has to do with properly understanding JavaScript events and timing .
The browser has an event loop which checks the event queue and processes pending events. For example, if an event happens in the background (e.g., a script onload event) while the browser is busy (e.g., processing an onclick ), the event gets appended to the queue. When the onclick handler is complete, the queue is checked and the event is then handled (e.g., the onload script is executed).
Similarly, setTimeout() also puts execution of its referenced function into the event queue if the browser is busy.
When a value of zero is passed as the second argument to setTimeout() , it attempts to execute the specified function “as soon as possible”. Specifically, execution of the function is placed on the event queue to occur on the next timer tick. Note, though, that this is not immediate; the function is not executed until the next tick. That’s why in the above example, the call to console.log(4) occurs before the call to console.log(3) (since the call to console.log(3) is invoked via setTimeout, so it is slightly delayed).
Write a simple function (less than 160 characters) that returns a boolean indicating whether or not a string is a palindrome .
The following one line function will return true if str is a palindrome; otherwise, it returns false.
For example:
Write a sum method which will work properly when invoked using either syntax below.
There are (at least) two ways to do this:
In JavaScript, functions provide access to an arguments object which provides access to the actual arguments passed to a function. This enables us to use the length property to determine at runtime the number of arguments passed to the function.
If two arguments are passed, we simply add them together and return.
Otherwise, we assume it was called in the form sum(2)(3) , so we return an anonymous function that adds together the argument passed to sum() (in this case 2) and the argument passed to the anonymous function (in this case 3).
When a function is invoked, JavaScript does not require the number of arguments to match the number of arguments in the function definition. If the number of arguments passed exceeds the number of arguments in the function definition, the excess arguments will simply be ignored. On the other hand, if the number of arguments passed is less than the number of arguments in the function definition, the missing arguments will have a value of undefined when referenced within the function. So, in the above example, by simply checking if the 2nd argument is undefined, we can determine which way the function was invoked and proceed accordingly.
Consider the following code snippet:
(a) What gets logged to the console when the user clicks on “Button 4” and why?
(b) Provide one or more alternate implementations that will work as expected.
(a) No matter what button the user clicks the number 5 will always be logged to the console. This is because, at the point that the onclick method is invoked (for any of the buttons), the for loop has already completed and the variable i already has a value of 5. (Bonus points for the interviewee if they know enough to talk about how execution contexts, variable objects, activation objects, and the internal “scope” property contribute to the closure behavior.)
(b) The key to making this work is to capture the value of i at each pass through the for loop by passing it into a newly created function object. Here are four possible ways to accomplish this:
Alternatively, you could wrap the entire call to btn.addEventListener in the new anonymous function:
Or, we could replace the for loop with a call to the array object’s native forEach method:
Lastly, the simplest solution, if you’re in an ES6/ES2015 context, is to use let i instead of var i :
Assuming d is an “empty” object in scope, say:
…what is accomplished using the following code?
The snippet of code shown above sets two properties on the object d . Ideally, any lookup performed on a JavaScript object with an unset key evaluates to undefined . But running this code marks those properties as “own properties” of the object.
This is a useful strategy for ensuring that an object has a given set of properties. Passing this object to Object.keys will return an array with those set keys as well (even if their values are undefined ).
The logged output will be:
arr1 and arr2 are the same (i.e. ['n','h','o','j', ['j','o','n','e','s'] ] ) after the above code is executed for the following reasons:
Calling an array object’s reverse() method doesn’t only return the array in reverse order, it also reverses the order of the array itself (i.e., in this case, arr1 ).
The reverse() method returns a reference to the array itself (i.e., in this case, arr1 ). As a result, arr2 is simply a reference to (rather than a copy of) arr1 . Therefore, when anything is done to arr2 (i.e., when we invoke arr2.push(arr3); ), arr1 will be affected as well since arr1 and arr2 are simply references to the same object.
And a couple of side points here that can sometimes trip someone up in answering this question:
Passing an array to the push() method of another array pushes that entire array as a single element onto the end of the array. As a result, the statement arr2.push(arr3); adds arr3 in its entirety as a single element to the end of arr2 (i.e., it does not concatenate the two arrays, that’s what the concat() method is for).
Like Python, JavaScript honors negative subscripts in calls to array methods like slice() as a way of referencing elements at the end of the array; e.g., a subscript of -1 indicates the last element in the array, and so on.
What will the code below output to the console and why ?
Here’s why…
The fundamental issue here is that JavaScript (ECMAScript) is a loosely typed language and it performs automatic type conversion on values to accommodate the operation being performed. Let’s see how this plays out with each of the above examples.
Example 1: 1 + "2" + "2" Outputs: "122" Explanation: The first operation to be performed in 1 + "2" . Since one of the operands ( "2" ) is a string, JavaScript assumes it needs to perform string concatenation and therefore converts the type of 1 to "1" , 1 + "2" yields "12" . Then, "12" + "2" yields "122" .
Example 2: 1 + +"2" + "2" Outputs: "32" Explanation: Based on order of operations, the first operation to be performed is +"2" (the extra + before the first "2" is treated as a unary operator). Thus, JavaScript converts the type of "2" to numeric and then applies the unary + sign to it (i.e., treats it as a positive number). As a result, the next operation is now 1 + 2 which of course yields 3 . But then, we have an operation between a number and a string (i.e., 3 and "2" ), so once again JavaScript converts the type of the numeric value to a string and performs string concatenation, yielding "32" .
Example 3: 1 + -"1" + "2" Outputs: "02" Explanation: The explanation here is identical to the prior example, except the unary operator is - rather than + . So "1" becomes 1 , which then becomes -1 when the - is applied, which is then added to 1 yielding 0 , which is then converted to a string and concatenated with the final "2" operand, yielding "02" .
Example 4: +"1" + "1" + "2" Outputs: "112" Explanation: Although the first "1" operand is typecast to a numeric value based on the unary + operator that precedes it, it is then immediately converted back to a string when it is concatenated with the second "1" operand, which is then concatenated with the final "2" operand, yielding the string "112" .
Example 5: "A" - "B" + "2" Outputs: "NaN2" Explanation: Since the - operator can not be applied to strings, and since neither "A" nor "B" can be converted to numeric values, "A" - "B" yields NaN which is then concatenated with the string "2" to yield “NaN2”.
Example 6: "A" - "B" + 2 Outputs: NaN Explanation: As exlained in the previous example, "A" - "B" yields NaN . But any operator applied to NaN with any other numeric operand will still yield NaN .
The following recursive code will cause a stack overflow if the array list is too large. How can you fix this and still retain the recursive pattern?
The potential stack overflow can be avoided by modifying the nextListItem function as follows:
The stack overflow is eliminated because the event loop handles the recursion, not the call stack. When nextListItem runs, if item is not null, the timeout function ( nextListItem ) is pushed to the event queue and the function exits, thereby leaving the call stack clear. When the event queue runs its timed-out event, the next item is processed and a timer is set to again invoke nextListItem . Accordingly, the method is processed from start to finish without a direct recursive call, so the call stack remains clear, regardless of the number of iterations.
What is a “closure” in JavaScript? Provide an example.
A closure is an inner function that has access to the variables in the outer (enclosing) function’s scope chain. The closure has access to variables in three scopes; specifically: (1) variable in its own scope, (2) variables in the enclosing function’s scope, and (3) global variables.
Here is an example:
In the above example, variables from innerFunc , outerFunc , and the global namespace are all in scope in the innerFunc . The above code will therefore produce the following output:
What would the following lines of code output to the console?
Explain your answer.
The code will output the following four lines:
In JavaScript, both || and && are logical operators that return the first fully-determined “logical value” when evaluated from left to right.
The or ( || ) operator. In an expression of the form X||Y , X is first evaluated and interpreted as a boolean value. If this boolean value is true , then true (1) is returned and Y is not evaluated, since the “or” condition has already been satisfied. If this boolean value is “false”, though, we still don’t know if X||Y is true or false until we evaluate Y , and interpret it as a boolean value as well.
Accordingly, 0 || 1 evaluates to true (1), as does 1 || 2 .
The and ( && ) operator. In an expression of the form X&&Y , X is first evaluated and interpreted as a boolean value. If this boolean value is false , then false (0) is returned and Y is not evaluated, since the “and” condition has already failed. If this boolean value is “true”, though, we still don’t know if X&&Y is true or false until we evaluate Y , and interpret it as a boolean value as well.
However, the interesting thing with the && operator is that when an expression is evaluated as “true”, then the expression itself is returned. This is fine, since it counts as “true” in logical expressions, but also can be used to return that value when you care to do so. This explains why, somewhat surprisingly, 1 && 2 returns 2 (whereas you might it expect it to return true or 1 ).
What will be the output when the following code is executed? Explain.
The code will output:
In JavaScript, there are two sets of equality operators. The triple-equal operator === behaves like any traditional equality operator would: evaluates to true if the two expressions on either of its sides have the same type and the same value. The double-equal operator, however, tries to coerce the values before comparing them. It is therefore generally good practice to use the === rather than == . The same holds true for !== vs != .
What is the output out of the following code? Explain your answer.
The output of this code will be 456 ( not 123 ).
The reason for this is as follows: When setting an object property, JavaScript will implicitly stringify the parameter value. In this case, since b and c are both objects, they will both be converted to "[object Object]" . As a result, a[b] and a[c] are both equivalent to a["[object Object]"] and can be used interchangeably. Therefore, setting or referencing a[c] is precisely the same as setting or referencing a[b] .
What will the following code output to the console:
The code will output the value of 10 factorial (i.e., 10!, or 3,628,800).
Here’s why:
The named function f() calls itself recursively, until it gets down to calling f(1) which simply returns 1 . Here, therefore, is what this does:
Consider the code snippet below. What will the console output be and why?
The output will be 1 , even though the value of x is never set in the inner function. Here’s why:
As explained in our JavaScript Hiring Guide , a closure is a function, along with all variables or functions that were in-scope at the time that the closure was created. In JavaScript, a closure is implemented as an “inner function”; i.e., a function defined within the body of another function. An important feature of closures is that an inner function still has access to the outer function’s variables.
Therefore, in this example, since x is not defined in the inner function, the scope of the outer function is searched for a defined variable x , which is found to have a value of 1 .
What will the following code output to the console and why:
What is the issue with this code and how can it be fixed.
The first console.log prints undefined because we are extracting the method from the hero object, so stoleSecretIdentity() is being invoked in the global context (i.e., the window object) where the _name property does not exist.
One way to fix the stoleSecretIdentity() function is as follows:
Create a function that, given a DOM Element on the page, will visit the element itself and all of its descendents ( not just its immediate children ). For each element visited, the function should pass that element to a provided callback function.
The arguments to the function should be:
- a DOM element
- a callback function (that takes a DOM element as its argument)
Visiting all elements in a tree (DOM) is a classic Depth-First-Search algorithm application. Here’s an example solution:
Testing your this knowledge in JavaScript: What is the output of the following code?
Why isn’t it 10 and 5 ?
In the first place, as fn is passed as a parameter to the function method , the scope ( this ) of the function fn is window . var length = 10; is declared at the window level. It also can be accessed as window.length or length or this.length (when this === window .)
method is bound to Object obj , and obj.method is called with parameters fn and 1 . Though method is accepting only one parameter, while invoking it has passed two parameters; the first is a function callback and other is just a number.
When fn() is called inside method , which was passed the function as a parameter at the global level, this.length will have access to var length = 10 (declared globally) not length = 5 as defined in Object obj .
Now, we know that we can access any number of arguments in a JavaScript function using the arguments[] array.
Hence arguments[0]() is nothing but calling fn() . Inside fn now, the scope of this function becomes the arguments array, and logging the length of arguments[] will return 2 .
Hence the output will be as above.
Consider the following code. What will the output be, and why?
var statements are hoisted (without their value initialization) to the top of the global or function scope it belongs to, even when it’s inside a with or catch block. However, the error’s identifier is only visible inside the catch block. It is equivalent to:
What will be the output of this code?
Neither 21, nor 20, the result is undefined
It’s because JavaScript initialization is not hoisted.
(Why doesn’t it show the global value of 21? The reason is that when the function is executed, it checks that there’s a local x variable present but doesn’t yet declare it, so it won’t look for global one.)
What will this code print?
It will print 0 1 2 3 4 , because we use let instead of var here. The variable i is only seen in the for loop’s block scope.
What do the following lines output, and why?
The first statement returns true which is as expected.
The second returns false because of how the engine works regarding operator associativity for < and > . It compares left to right, so 3 > 2 > 1 JavaScript translates to true > 1 . true has value 1 , so it then compares 1 > 1 , which is false .
How do you add an element at the begining of an array? How do you add one at the end?
With ES6, one can use the spread operator:
Or, in short:
Imagine you have this code:
a) Will this result in a crash?
b) What will this output?
a) It will not crash. The JavaScript engine will make array slots 3 through 9 be “empty slots.”
b) Here, a[6] will output undefined , but the slot still remains empty rather than filled with undefined . This may be an important nuance in some cases. For example, when using map() , empty slots will remain empty in map() ’s output, but undefined slots will be remapped using the function passed to it:
What is the value of typeof undefined == typeof NULL ?
The expression will be evaluated to true, since NULL will be treated as any other undefined variable.
Note: JavaScript is case-sensitive and here we are using NULL instead of null .
What would following code return?
typeof 1 will return "number" and typeof "number" will return string .
What will be the output of the following code:
Explain your answer. How could the use of closures help here?
The code sample shown will not display the values 0, 1, 2, 3, and 4 as might be expected; rather, it will display 5, 5, 5, 5, and 5.
The reason for this is that each function executed within the loop will be executed after the entire loop has completed and all will therefore reference the last value stored in i , which was 5.
Closures can be used to prevent this problem by creating a unique scope for each iteration, storing each unique value of the variable within its scope, as follows:
This will produce the presumably desired result of logging 0, 1, 2, 3, and 4 to the console.
In an ES2015 context , you can simply use let instead of var in the original code:
What is NaN ? What is its type? How can you reliably test if a value is equal to NaN ?
The NaN property represents a value that is “not a number”. This special value results from an operation that could not be performed either because one of the operands was non-numeric (e.g., "abc" / 4 ), or because the result of the operation is non-numeric.
While this seems straightforward enough, there are a couple of somewhat surprising characteristics of NaN that can result in hair-pulling bugs if one is not aware of them.
For one thing, although NaN means “not a number”, its type is, believe it or not, Number :
Additionally, NaN compared to anything – even itself! – is false:
A semi-reliable way to test whether a number is equal to NaN is with the built-in function isNaN() , but even using isNaN() is an imperfect solution .
A better solution would either be to use value !== value , which would only produce true if the value is equal to NaN. Also, ES6 offers a new Number.isNaN() function, which is a different and more reliable than the old global isNaN() function.
What will the following code output and why?
Output to the console will be “3”.
There are three closures in the example, each with it’s own var b declaration. When a variable is invoked closures will be checked in order from local to global until an instance is found. Since the inner closure has a b variable of its own, that is what will be output.
Furthermore, due to hoisting the code in inner will be interpreted as follows:
Discuss possible ways to write a function isInteger(x) that determines if x is an integer.
This may sound trivial and, in fact, it is trivial with ECMAscript 6 which introduces a new Number.isInteger() function for precisely this purpose. However, prior to ECMAScript 6, this is a bit more complicated, since no equivalent of the Number.isInteger() method is provided.
The issue is that, in the ECMAScript specification, integers only exist conceptually; i.e., numeric values are always stored as floating point values.
With that in mind, the simplest and cleanest pre-ECMAScript-6 solution (which is also sufficiently robust to return false even if a non-numeric value such as a string or null is passed to the function) would be the following use of the bitwise XOR operator:
The following solution would also work, although not as elegant as the one above:
The following function (or with Math.ceil() or Math.floor() in place of Math.round() ) might also seem useful, but the results are not exactly the same as with the above two functions:
The difference is, these Math -based solutions return true for Infinity and -Infinity , whereas the others (and notably ES6’s Number.isInteger() ) return false .
Another fairly common incorrect solution is the following:
While this parseInt -based approach will work well for many values of x , once x becomes quite large, it will fail to work properly. The problem is that parseInt() coerces its first parameter to a string before parsing digits. Therefore, once the number becomes sufficiently large, its string representation will be presented in exponential form (e.g., 1e+21 ). Accordingly, parseInt() will then try to parse 1e+21 , but will stop parsing when it reaches the e character and will therefore return a value of 1 . Observe:
How do you clone an object?
Now the value of objclone is {a: 1 ,b: 2} but points to a different object than obj .
Note the potential pitfall, though: Object.assign() will just do a shallow copy, not a deep copy. This means that nested objects aren’t copied. They still refer to the same nested objects as the original:
There is more to interviewing than tricky technical questions, so these are intended merely as a guide. Not every “A” candidate worth hiring will be able to answer them all, nor does answering them all guarantee an “A” candidate. At the end of the day, hiring remains an art, a science — and a lot of work .
Tired of interviewing candidates? Not sure what to ask to get you a top hire?
Let Toptal find the best people for you.
Our Exclusive Network of JavaScript Developers
Looking to land a job as a JavaScript Developer?
Let Toptal find the right job for you.
Job Opportunities From Our Network
Submit an interview question
Submitted questions and answers are subject to review and editing, and may or may not be selected for posting, at the sole discretion of Toptal, LLC.
Looking for JavaScript Developers?
Looking for JavaScript Developers ? Check out Toptal’s JavaScript developers.
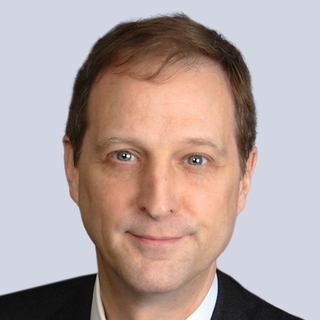
Jay Johnston
Coding HTML, CSS, and JavaScript since his armed forces days in 1997, Jay enjoys bringing value to clients via eCommerce solutions, legacy integrations, and optimized PHP and JavaScript-driven applications. His preferred DevOps environment is AWS, where he has strong skills in (and not limited to): Relational Database Services (RDS), Redshift, Dynamo DB, Data Migration Services (DMS), Lambda (serverless and microservices), Cloudwatch, Cloudtrail, and Event Bridge.
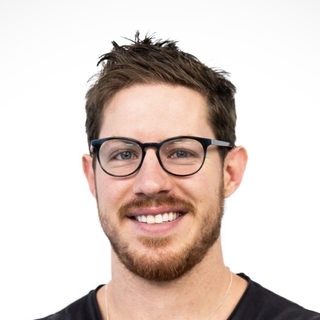
Tyler Standley
Along with strong communication skills and an exemplary work ethic, Tyler brings his hands-on experience with a wide range of programming languages. Recently, though, his focus has been directed towards JavaScript libraries. Throughout his career, he’s worked on multiple agile teams as a core developer and is now interested in working on anything JavaScript-related.
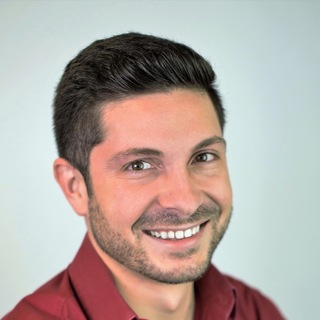
Justin Michela
Justin is a technical professional with a passion for learning and 18+ years of experience leading teams to build enterprise-grade distributed applications that solve real-world problems. Justin firmly believes that collaboration across all facets of a business, from development to marketing to sales, is required to succeed in this endeavor.
Toptal Connects the Top 3% of Freelance Talent All Over The World.
Join the Toptal community.
JavaScript Interview Prep Cheatsheet – Ace Your Coding Interviews with These Concepts
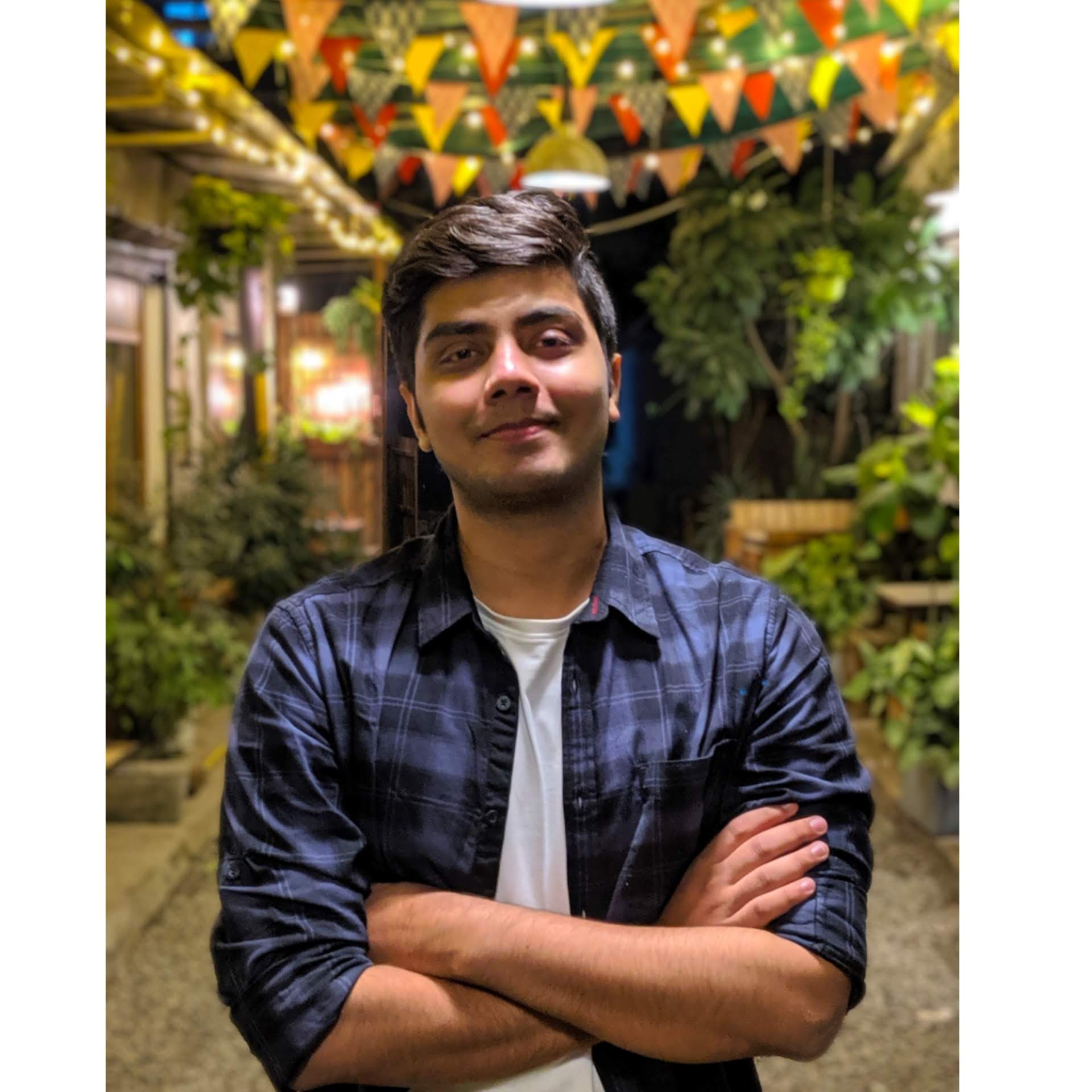
I've carefully gone through over 50 resources, I've been through 10 JavaScript interviews, and I've landed a job at a unicorn startup.
And throughout this entire process, I started to see a pattern in the most frequently asked JS interview questions.
In this article, I have tried to list the concepts which will cover 80% of any good JS interview.
So, if you are prepping for your next JS interview this is the perfect cheatsheet for you to review and solidify your skills. Go through this and you'll be ready to rock. 💃
📝Prerequisites
- Basic knowledge of the web and programming
- Familiarity with HTML/CSS and JavaScript (especially ES6+ syntax)
Table Of Contents 📜
- JavaScript Basics – JS Variables and Array Methods
- Functional Programming in JavaScript – Scope, Closures, and Hoisting
- Objects in JavaScript – Prototypes and "this"
- Asynchronous JavaScript – Event Loops, Timers, and Promises
- Advanced JavaScript Concepts to Know - Async/defer, Polyfills, Debouncing, and Throttling
- Storage in JavaScript
Caveat: The focus here will largely be to cover concepts relevant to the interview and not to create a comprehensive booklet for learning the language. Treat this more like a cheatsheet.
If you want to dive in deeply and learn more JS concepts, check out freeCodeCamp's curriculum .
With that out of the way - let's go!
JavaScript Basics 👶
Let's start off with some basic concepts every JS developer needs to know.
Variables in JavaScript 📥
Variables are the building blocks of every programming language. You use them to store values. A variable can be a number, string, and many other types.
Now, JS is a loosely-typed language. You don't have to state the type of variable. You can just declare it, and JS will figure it out on its own.
Now, in JavaScript we have 3 ways to declare variables: var , let , and const .
Here are the key differences:

Let's try to understand them through examples.
We will cover scope later on. For now, let's focus on the other differences.
Note: In JavaScript, putting a semi-colon after the end of statement is optional. I will be skipping it here for the sake of readability.
== vs === in JavaScript
Let's compare some variables. There are two ways you can do that.
== only checks for the value
=== checks for value + type
Arrays in JavaScript
Now that we know a bit about variables, let's move on to arrays and array-methods.
If we have declared a lot of variables, it makes sense to store them somewhere. Otherwise it will be difficult to keep track of all of them. Arrays are one way of storing a variable.
But only storing variables in an array is kind of boring. We can do more stuff with this array (like accessing these variables or changing the order in which they are stored or how they are stored).
For that, JS has a lot of methods. Let's look at some of them now.
JavaScript Array Methods 🧰
The most frequently used array methods in JS are: map , filter , find , reduce , and forEach .
Let's cover map , filter , and forEach . You can explore more in this helpful article .
The map array method
map creates a new copy of the original array. We use it when we want to do something with the elements of the original array but don't want to change it.
map iterates over the original array and takes a callback function (which we'll cover later) as an argument. In the callback function, we tell it what to do with the elements.
The filter array method
filter creates a new array with elements that meet the given condition(s).
Let's look at an example. I have used arrow functions here. If you are a little uncomfortable with functions, you can cover the next section first and come back.
Try to do the exercises yourself first to test your knowledge. If you come up with different or better solutions, let me know!
Generally, a follow up to this: can you do it without the array method?
The forEach array method
forEach is very similar to map but has two key differences:
First of all, map returns a new Array, but forEach doesn't.
And second, you can do method chaining in map but not in forEach .
Note: map and forEach don't mutate (change) the original array.
Functional Programming in JavaScript 🛠
We have already used functions above. Let's cover them in more detail now.
Just like how we used variables to store values, we can use functions to store a piece of code which we can reuse.
You can make function in two ways:
Now, let's cover some important concepts related to functions.
Function Scope in JavaScript 🕵️
Scope determines from where the variables are accessible.
There are three types of scope:
- Global (declaration outside of any function)
- Function (declaration inside a function)
- Block (declaration inside a block)
Remember from before that var is globally scoped whereas let and const are block scoped. Let's understand that now.
Closures in JavaScript (❗important) 🔒
We have already used a closure without even realizing it. In the example below, prefix is a closed-over-variable.
This section will have a lot of fancy words, so bear with me. We will cover them one by one.
A function bundled together with its lexical environment forms a closure.
Okay, what is a lexical environment?
It is essentially the surrounding state – the local memory along with the lexical environment of its parent.
Whaaat? 🤯 I know it's a bit of a doozy. Let's understand it with a simple example.
When x is invoked, y is returned. Now, y is waiting to be executed. Kind of like a loaded gun waiting to be shot! 🔫
So, when we finally invoke z, y is invoked. Now, y has to log a so it first tries to find 🔍 it in the local memory but it's not there. It goes to its parent function. It finds a there.
Voila! There you have it - this is closure .
Even when functions are returned (in the above case y) they still remember their lexical scope (where it came from)
Totally unrelated quote for kicks 👻:
They may forget what you said - but they will never forget how you made them feel - Carl W. Buehner
I swear the rest of the article is legit 🤞 Keep reading.
Advantages of Closures in JavaScript 😎
- Data Hiding/Encapsulation
Suppose you want to create a counter application. Every time you call it, the count increases by 1. But you don't want to expose the variable outside the function. How to do it?
You guessed it – closures!
Don't worry about this and new . We have a whole section devoted to them down below.
Disadvantages of Closures in JavaScript 😅
- Overconsumption of memory or memory leaks can happen.
For example, the closed-over-variable will not be garbage collected. This is because, even if the outer function has run, the returned inner function still has a reference to the closed-over-variable.
Note: Garbage collection basically removes unused variables from the memory automatically.
Hoisting in JavaScript 🚩
This is JavaScript's default behavior of moving declarations to the top of the program.
- var declaration is hoisted up and initialized with undefined .
- let and const declarations are hoisted up but not initialized.
- function definitions are also hoisted up and stored as they are.
Let's look at an example:
Phew! I am done with functions here, but if you want more check out this amazing talk by Anjana Vakil on functional programming.
Objects in JavaScript 🔮
Just like arrays, objects are a way of storing data. We do so with the help of key-value pairs.
name is the key and Raj is the value . Keys are generally the name of the properties of the object.
We can store all sorts of data like functions inside an object. You can explore more here on the MDN .
What is this in JavaScript?
Now, working with objects is different in JS than in other popular programming languages like C++. And to understand that properly, we need a good grasp of the this keyword.
Let's try to understand it step-by-step.
In a program, at times, we need a way to point at stuff. Like saying this function right here belongs to this object. this helps us get this context.
You will understand what I am saying better when we look at some examples.
For now, think of this as something which provides context. And remember this important thing: its value depends on how and where it is called.
I know, I know. A lot of this 😬. Let's go over all this slowly.
Start a new program and just log this .
It will point to the window object.
Now, let's take an example with an object:
Now, this will point to the object. So what's happening here?
In the first example, we had nothing left of the . so it defaulted to the window object. But in this example, we have the object obj .
We again get the window object. So, we can see that the value of this depends on how and where are we doing the calling.
What we just did above is called Implicit Binding . The value of this got bound to the object.
There is another way to use this . Explicit binding is when you force a function to use a certain object as its this .
Let's understand why we need explicit binding through an example.
We are using this properly, but can you see the problem with the above code?
We are repeating code. And one of the principles of good programming is keep your code DRY! (Don't Repeat Yourself)
So, let's get rid of displayName_2 and simply do:
call forced displayName_1 to use the second object as its this .
There are a lot of other ways we can do this.
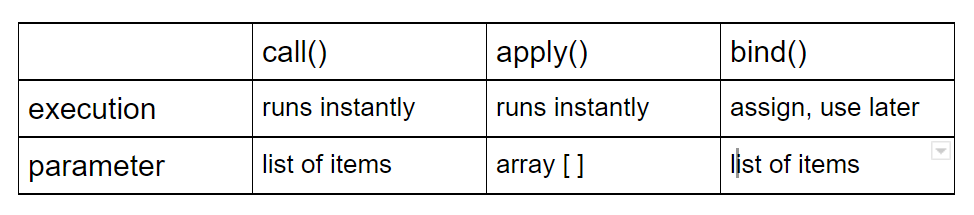
Try to solve the given problem yourself.
Finally, remember that I said that there are differences between arrow and regular functions.
The case of this is one of them.
For an arrow function, the value depends on the lexical scope – that is to say, the outer function where the arrow function is declared.
So, if we make the displayName() from above an arrow function, nothing will work.
Arrow functions basically inherit the parent's context which in the above case is the window .
Prototypes and Prototypal Inheritance in JavaScript 👪
Whenever we create anything (like an object or function) in JavaScript, the JS Engine automatically attaches that thing with some properties and methods.
All this comes via prototypes .
__proto__ is the object where JS is putting it all.
Let's see some examples. Fire up your consoles!
All this is called a prototype chain .
We can do the same with objects and functions as well.
We will always find Object.prototype behind the scenes. That's why you may have heard that everything in JS is an object. 🤯
What is Prototypal Inheritance in JavaScript?
Note: Don't modify prototypes this way. It's just for understanding. Here's the right way to do it .
By doing this, object2 gets access to the object's properties. So, now we can do:
This is prototypal inheritance .
Asynchronous JavaScript ⚡
So, JS is a single-threaded language. Things happen one at a time. Only after one thing is done can we move to the next thing.
But this creates problems in the real world, especially, when we are working with browsers.
For example, when we need to fetch data from the web - often times we don't know how long will it take to get it. And whether we will be able to get the data successfully.
To help with this, asynchronous JS comes into play.
And the most important concept to understand is the event loop.
Event Loops in JavaScript ➰
Instead of providing a half-baked explanation here, I highly recommend watching this video by Philip Roberts if you haven't already:
Learn all about event loops in JS here .
Timers in JavaScript – setTimeout, setInterval, clearInterval ⏱️
I hope you watched the video. It mentioned timers. Let's talk about them more now. These are very frequently asked about in interviews.
The setTimeout() method calls a function or evaluates an expression after a specified number of milliseconds.
setInterval() does the same for specified intervals.
You use clearInterval() to stop the timer.
Let's go over some questions that use these concepts.
Here's a slightly trickier one:
And here's a short explanation of what's going on there: when setTimeout comes again into the picture, the entire loop has run and the value of i has become 6,
Now, let's say we want the outcome to be 1 2 3 4 5 – what do we do?
Instead of var ➡️ use let .
Why this will work?
var is globally scoped but let is locally scoped. So for let a new i is created for every iteration.
Promises in JavaScript (❗important) 🤝
Promises are at the heart of Asynchronous JS.
The Promise object represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
A promise can be in one of these three states:
- Pending: initial state, neither fulfilled nor rejected
- Fulfilled: operation was completed successfully
- Rejected: operation failed
Note: resolve and reject are just conventional names. Call it pizza🍕 if you like.
Instead of then/catch , we can also use async/await :
One of the advantages of promises is that they are a much cleaner syntax. Before we had promises, we could easily get stuck in callback hell 🌋
Advanced JavaScript Concepts to Know
📚 polyfills in javascript.
A polyfill is a piece of code (usually JavaScript on the Web) used to provide modern functionality on older browsers that do not natively support it. MDN
- Let's implement it for map :
Notice how we use this . Here, we have basically created a new array and are adding values to it.
Async and defer in JavaScript ✔️
These concepts are frequently asked about in interviews by big corporations like Amazon, Walmart, and Flipkart. 🏢
To understand async and defer , we need to have an idea of how browsers render a webpage. First, they parse the HTML and CSS. Then DOM trees are created. From these, a render tree is created. Finally, from the render tree - a layout is created and the painting happens.
For a more detailed look, check out this video .
Async and defer are boolean attributes which can be loaded along with the script tags. They are useful for loading external scripts into your web page.
Let's understand with the help of pictures.
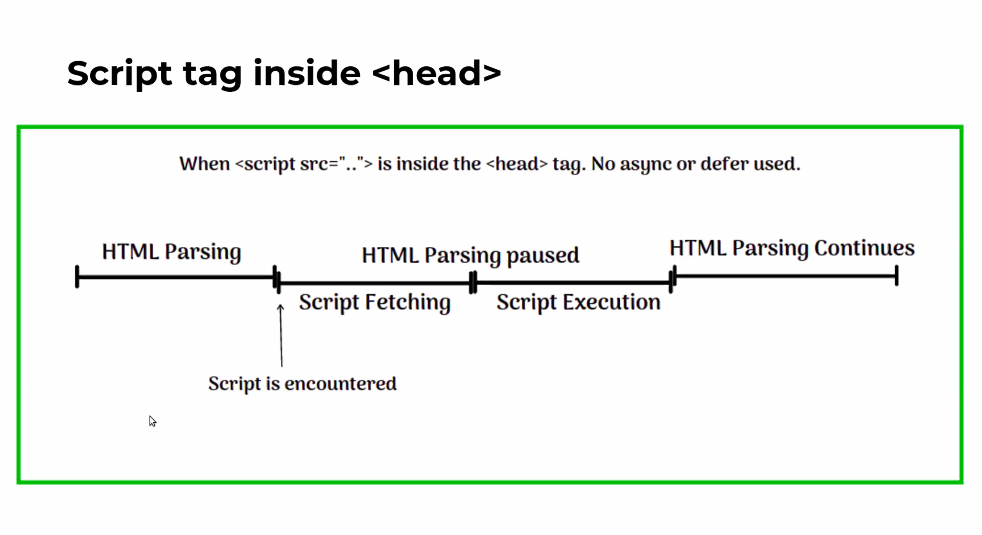
If there are multiple scripts which are dependant on each other, use defer . Defer script are executed in the order which they are defined.
If you want to load external script which is not dependant on the execution of any other scripts, use async .
Note: The async attribute does not guarantee the order of execution of scripts.
Debouncing in JavaScript ⛹️♂️
Debouncing is another favorite topic of interviewers.
Let's understand it by creating a search bar.
Demo: https://codesandbox.io/s/debounce-input-field-o5gml
Create a simple input field in index.html like this:
Now, in index.js . Don't forget to add it to index.html first:
First, we have selected the input and added an event listener to it. Then we created a debounce function which takes a callback function and delay.
Now, inside the debounce function we create a timer using setTimeout . Now, this timer's job is to make sure that the next call for getData only happens after 300 ms. This is what debouncing is.
Also, we use clearTimeout to remove it. Don't want too many of them hanging out there taking up memory space!
Phew! Lots of theory. Let's do a fun challenge. You must have seen the countdown before a game starts (it goes like 10, 9, 8, .... with some delay in between). Try to write a program for it.
Here's how you'd do it:
Were you able to solve it? Did you do it differently? Let me know your solution.
Throttling in JavaScript 🛑
Let's look at an example again. Suppose that on every window resize event we call an expensive function. Now, we want it such that the expensive function will only be executed once in the given time interval. This is what throttling is.
Create an index.html and an index.js with the following code:
Almost the same as debouncing. The key difference is the flag variable. Only, when it's true we are invoking the callback function. And it is set to true inside the setTimeout . So the value is true only after the desired time limit.
So, what's the difference between debounce and throttling❓
Let's take the search bar 🔍 example from above. When we are debouncing the input field, we are saying to only fetch the data when the difference between two keyup events is at least 300 ms.
In the case of throttling, we make a function call only after a certain period of time.
Suppose that you are searching for an encyclopedia in the search bar. The first call is made on e and it took us 300 ms to reach p . The next call will be made then only. All the events in between will be ignored.
So, to summarize, debouncing is when the difference between two keyup events is 300 ms. And throttling is when the difference between two function calls is 300 ms. Basically, the function is called after a certain interval of time.
Storage in JavaScript 💾
Finally, a small but important topic to wrap things up.
localStorage: Data persists even after closing your session
sessionStorage: You lose your data when your session is over, like when you close the browser on the tab.
And we are done! 🏁 I hope you feel more confident about your next JS interview now. I wish you all the best.
If you have any queries / suggestions / feedback, you can reach me on Twitter: https://twitter.com/rajatetc .
🗃️ Main References
- Akshay Saini
- Coding Addict
- Javascript_Interviews
software engineer, unacademy • rajatgupta.xyz • twitter.com/rajatetc • development, design, psychology, and startups
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Top 100 JavaScript Interview Questions and Answers
Dive into the core of JavaScript with these 100 essential Javascript interview questions and answers, perfect for acing your next tech interview.
Top 100 JavaScript Developer Interview Questions and Answers in 2024" offers a snapshot of the most pressing inquiries faced by JavaScript developers today. From basic JavaScript interview questions tailored for freshers to more complex JS practical questions designed for those with 5 years of experience, JavaScript Developer Interview Questions compilation is a robust reflection of the industry's evolving demands. JavaScript questions span across diverse proficiency levels, with both the theoretical foundations and hands-on coding challenges covered. Whether a JavaScript Developer is looking to understand fundamental javascript concepts or dive into specific JS interview scenarios, this guide is designed to empower both interviewers and candidates, ensuring a transparent and efficient hiring process for all involved.
What are the General JavaScript Interview questions for the JavaScript Developer?
General JavaScript interview questions are on JavaScript’s foundational concepts and basic functionalities. The questions probe an applicant's understanding of core topics such as variables, data types, functions, control structures, and basic DOM manipulation . JavaScript Developer Interview questions are fundamental in assessing an individual's grasp of the elementary aspects of JavaScript programming.
JavaScript plays a crucial role in creating effective websites, with its versatility and functionality.
General JavaScript questions are especially pertinent for freshers. These inquiries ensure that a novice developer possesses the essential skills to excel in the industry.
It is crucial to grasp the fundamental concepts of JavaScript if you're new to web development. These fundamental concepts are the building blocks and will lay a strong foundation for more complex technical discussions and improve the confidence as a beginner developers. JavaScript developers will be better prepared to tackle advanced topics with a solid understanding of these core concepts.
The most important JavaScript interview questions are listed below.
What is JavaScript?
View Answer
Hide Answer
JavaScript is a high-level, interpreted scripting language primarily used for web development to create interactive and dynamic web pages. JavaScript runs in browsers and can be used server-side with environments like Node.js.
How would you explain the difference between JavaScript and ECMAScript?
The difference between JavaScript and ECMAScript is that JavaScript is a general-purpose scripting language, while ECMAScript (often abbreviated as ES) is the standardized specification that defines its core features. Think of ECMAScript as the blueprint and JavaScript as one of the implementations of that blueprint.
How is JavaScript different from Java ?
The difference between JavaScript and Java is that Java is an OOP programming language while JavaScript is an OOP scripting language. JavaScript is interpreted and follows dynamic typing. Java is compiled and strongly typed.
What are the primitive data types in JavaScript?
The primitive data types in JavaScript are string, number, boolean, undefined, null, symbol, Object, and BigInt.
How would you explain the concept of undefined in JavaScript?
Undefined in JavaScript is a primitive value automatically assigned to variables that have been declared but not yet initialized. It represents the absence of a defined value.
What is null in JavaScript?
Null in JavaScript is a special value that signifies no value or no object. It needs to be explicitly set and denotes the intentional absence of any value.
How can you differentiate null from undefined?
You can differentiate null from undefined as undefined is the default state of a declared variable that hasn't been assigned a value, whereas null is an explicit assignment indicating the deliberate absence of a value.
What is NaN? How can you check if a value is NaN?
NaN stands for "Not-a-Number" and indicates a value that cannot be represented as a valid number. To check if a value is NaN, use the global function NaN() or the more reliable Number is NaN().
Explain variable hoisting.
Variable Hoisting is a concept or behavior in JavaScript where variable and function declarations are moved to the top of their containing scope during the compilation phase.
Your engineers should not be hiring. They should be coding
Help your team focus on what they were hired for. Flexiple will manage your entire hiring process and scale your tech team.
What is the difference between let, const, and var?
The difference between let, const, and var is that var declarations are globally scoped or function scoped while let and const are block scoped. var and let allows for reassignment, const creates a read-only reference, meaning the value it holds cannot be changed after declaration.
What is an Immediately Invoked Function Expression (IIFE)?
An Immediately Invoked Function Expression (or IIFE) is a JavaScript function that is defined and executed immediately after its creation.
How can you explain closures in JavaScript?
Closures in JavaScript are functions which retains access to variables from its enclosing scope, even after that outer function has finished executing
What is the this keyword? How does it work?
The this keyword in JavaScript refers to the current execution context or the object that the function is bound to. The value of this keyword changes if its call type is a method, constructor or a standalone function.
How does bind(), call(), and apply() work?
bind(), call(), and apply() are methods used to change the context of this keyword within a function. bind() creates a new function with a specified `this` value, while call() and apply() immediately invoke the function with the provided `this` value and arguments.
What is the prototype chain?
The prototype chain is a mechanism that defines how objects inherit properties and methods from their prototype objects. JavaScript looks up the prototype chain to find it in higher-level prototypes, when a property or method is not found on an object.
How can you explain event delegation?
Event delegation is a method where a single event handler is placed on a common parent element of multiple child elements. The parent element captures and handles events triggered by the child elements.
What are JavaScript Promises?
JavaScript Promises are objects representing the eventual completion or failure of an asynchronous operation. Promises in JavaScript provide a cleaner and more structured way to handle asynchronous code compared to callback functions, making it easier to work with asynchronous tasks like HTTP requests.
How do you handle errors in JavaScript?
Use try-catch block to handle errors in JavaScript. Errors are thrown explicitly using the throw statement. The try statement is used to define a code block to be executed. The catch statement defines a code block that handles any errors that occur during execution. The finally block defines a code block that should always be executed, regardless of the outcome of the try statement. The throw statement can be used to define a custom error caught and handled by the catch statement.
How does the Event Loop work in JavaScript?
Event Loop in JavaScript continuously checks the call stack for executed functions and the message queue for events or tasks to process ensuring that JavaScript remains single-threaded while handling asynchronous operations efficiently.
What is the difference between == and ===?
The difference between == and === in JavaScript is that == is a loose equality operator that compares values after type coercion, while === is a strict equality operator that compares both values and types. === requires both values to be of the same type and have the same value, making it a safer choice for most comparisons to avoid unexpected type conversions.
How can you explain the importance of the use strict directive?
The importance of use strict directive is that it enforces a stricter set of rules and helps catch common coding mistakes. It prevents the use of undeclared variables, eliminates ambiguous behavior, and encourages a cleaner, more reliable codebase.
How would you explain the concept of Callback Hell or Pyramid of Doom?
Callback Hell, or Pyramid of Doom, occurs when multiple nested callback functions are used in asynchronous JavaScript code, leading to deeply indented and hard-to-read code structures making code maintenance and debugging challenging.
How do you avoid Callback Hell?
To avoid callback Hell use Promises, async/await, or modularize the code into smaller functions.
What is a callback function?
A callback function is a function passed as an argument to another function, which is then invoked or executed at a later point in the program's execution.
Can you explain how map, reduce, and filter methods work?
map(), reduce(), and filter() are array methods in JavaScript. map() transforms each element in an array into a new array based on a provided function. reduce() reduces an array to a single value by applying a function cumulatively to elements. filter() creates a new array with elements that pass a given test.
What is a closure? Can you give a practical example?
Closure in JavaScript is when a function retains access to variables from its enclosing scope, even after that outer function has finished executing. Practical examples include creating private variables and functions in JavaScript, maintaining state in event handlers, and implementing data hiding patterns.
How do you clone an object in JavaScript?
To clone an object in JavaScript use methods like Object.assign(), the spread operator (...), or by create a custom cloning functions.
Can you explain how to create and use JavaScript Promises?
To create a Promise, use the Promise constructor with two parameters: resolve and reject. Then use .then() and .catch() to handle successful and failed outcomes, respectively.
How does JavaScript handle asynchronous operations?
JavaScript handles asynchronous operations using mechanisms like callbacks, Promises, async/await, and the Event Loop.
What distinguishes a function expression from a function declaration?
A function expression is distinguished from a function declaration as function declaration defines a named function with a specific name and is hoisted to the top of its containing scope. A function expression assigns an anonymous or named function to a variable, and it is not hoisted.
What are JavaScript Developer Questions About JavaScript DOM Manipulation?
JavaScript Developer Questions about JavaScript DOM Manipulation focus on the understanding and application of JavaScript to interact with the Document Object Model (DOM). These questions revolve around how developers use JavaScript to create, modify, delete, or retrieve elements and attributes within a web page.
JavaScript DOM Manipulation refers to the methods and techniques employed by developers to dynamically change the structure, content, or styling of a web page. The DOM represents the structure of a web page, and JavaScript offers a range of methods and properties to alter this structure in real-time.
The importance of JavaScript DOM Manipulation in JavaScript development cannot be overstated. It is the foundation for creating interactive and dynamic web applications. A solid grasp of DOM Manipulation ensures developers can efficiently and effectively build user interfaces that respond to user actions and provide a seamless user experience.
The most important questions for a JavaScript DOM Manipulation are listed below.
How can you explain the Document Object Model (DOM)?
The Document Object Model (DOM) is a programming interface and representation of structured documents. It represents a web page's structure as a tree of objects, where each object corresponds to a part of the page, such as an element or an attribute.
How do you select an element in the DOM?
To select an element in the DOM use methods like document.getElementById(), document.querySelector(), or document.getElementsByClassName() , depending on the selection criteria.
What is the difference between innerHTML and textContent?
The difference between innerHTML and textContent is that innerHTML retrieves or sets the HTML content within an element, while textContent retrieves or sets only the text content, excluding any HTML tags.
How do you add or remove a class from an element in the DOM?
To add a class using the element.classList.add('classname') method and remove it using the element.classList.remove('classname') method.
How would you explain event bubbling and event capturing?
Event bubbling and event capturing are two phases of event propagation in the DOM. In event bubbling, the event starts from the target element that triggered the event and bubbles up to the root of the DOM. In event capturing, the process is reversed and the event descends from the root to the target element.
What is the purpose of the data-* attribute?
The data-* attribute allows to storage of custom data on an element, providing a way to store extra information that doesn't have any visual representation.
How do you create a new element and add it to the DOM?
To create a new element and add it to the DOM, use the document.createElement('elementName') method. Use the parentNode.appendChild(newElement) method after setting its properties.
How do you attach an event handler to a DOM element?
To attach an event handler to a DOM element use the element.addEventListener('eventname', handlerFunction) method.
How can you prevent the default behavior in an event handler?
To prevent the default behavior in an event handler use the event.preventDefault() method within the handler function.
How would you explain the importance of document.ready in jQuery?
document.ready in jQuery ensures that the DOM is fully loaded and ready for manipulation. It guarantees that scripts run only after the entire page's elements are available, preventing potential errors or unexpected behaviors.
What are Advanced JavaScript Questions for Advanced JavaScript Developers?
JavaScript questions for Advanced JavaScript developers dive deep into the intricacies and nuances of the language. Advanced questions challenge a developer's in-depth understanding, experience, and expertise in JavaScript's more complex areas, unlike basic queries that touch on foundational concepts.
General JavaScript questions often revolve around fundamental concepts, syntax, and basic functionalities, providing a foundational grasp of the language. Advanced questions probe areas like asynchronous programming, closures, prototypal inheritance, design patterns, and performance optimizations.
These advanced interview questions are tailored for developers with 3 to 5 years of experience. A developer is expected to handle complex scenarios, design decisions, and troubleshoot intricate issues, making these advanced questions crucial to gauge their expertise and problem-solving capabilities.
The most important questions for Advanced JavaScript Developers are listed below.
Can you explain the concept of shadow DOM?
The shadow DOM enables web developers to encapsulate their code by attaching separate DOM trees to specific elements. This mechanism isolates the internal structure, composition, and styling of these elements from the main document, ensuring functional and styling independence. It becomes easier to manage and maintain the code, by keeping them hidden from the rest of the document.
What is a JavaScript generator and how is it different from a regular function?
a JavaScript generator is a special type of function that can pause its execution and later resume from where it left off. Regular functions run to completion when invoked and generators produce a sequence of values using the yield keyword and are controlled by the next() method.
What is a Proxy in JavaScript?
A Proxy in JavaScript is an object that wraps another object (target) and intercepts operations, like reading or writing properties, offering a way to customize default behaviors.
How does JavaScript’s async and await work?
An asynchronous function in JavaScript returns a promise. The await keyword can be used inside the function to pause its execution until the promise settles, simplifying the flow of asynchronous code.
Can you explain JavaScript’s Module pattern?
JavaScript’s Module pattern provides a way to create private scopes and expose only chosen parts to the external world. It's a design pattern that utilizes closures to encapsulate functionality, allowing for public and private access levels.
What is a Service Worker and what is it used for?
A Service Worker is a script running in the background of a browser, separate from the web page. A Service Worker is used for caching resources, enabling offline capabilities, and intercepting network requests to enhance performance and user experience.
What is a Web Worker?
A Web Worker is a script executed in the background, on a separate thread from the main execution thread. A Web Worker ensures long-running tasks don't block the main thread, keeping web applications responsive.
How can you explain the concept of memoization?
Memoization in JavaScript is an optimization technique that stores the results of expensive function calls and returns the cached result when the same inputs reoccur thus reducing the computational time.
How do you handle state management in large applications?
Large applications handle state management using centralized state management solutions such as Redux, Vuex, or MobX. These libraries offer a structured and predictable method for managing and updating state across components.
What are the advantages and disadvantages of using JavaScript frameworks/libraries like React or Angular?
Using JavaScript frameworks/libraries like React or Angular provides advantages such as rapid development, reusable components, and robust ecosystems. The main disadvantages include steep learning curves, potential overengineering, and increased initial load times due to library overheads.
What are JavaScript Developer Questions About ES6 and Beyond?
JavaScript Developer Questions about ES6 and Beyond are questions that probe a JavaScript developer's understanding of the latest and most advanced features introduced in ECMAScript 2015 (known as ES6). It is essential for a JavaScript Developer to have a good understanding of the latest and most advanced features introduced in ECMAScript 2015 (known as ES6) and the subsequent updates thereafter.
ES6 and Beyond refers to the versions of ECMAScript, the standard upon which JavaScript is based, starting from the 2015 release (ES6) and encompassing all later versions. These updates brought a plethora of new features, such as arrow functions, promises, destructuring, and modules, to name a few.
The importance of ES6 and Beyond in JavaScript is such that grasping these modern features is essential for developers to write cleaner, more efficient, and more maintainable code. Understanding these newer aspects ensures compatibility with contemporary frameworks, tools, and best practices in the ever-evolving world of web development.
The most important JavaScript questions about ES6 features are listed below.
What are template literals in ES6?
Template literals in ES6 are string literals allowing embedded expressions. They utilize backticks ( ) instead of quotes and can span multiple lines, making string interpolation more intuitive.
Can you explain the spread and rest operators?
The spread operator (...) expands an array or object into its elements or properties. The rest operator, also represented by ..., collects the remaining elements or properties into an array or object.
What are arrow functions? How are they different from regular functions?
Arrow functions are a concise way to write functions in ES6. Arrow functions differ from regular functions in syntax and behavior, particularly in their handling of the this keyword, which arrow functions do not bind.
How can you explain destructuring assignment?
Destructuring assignment in ES6 allows unpacking values from arrays or properties from objects into distinct variables, simplifying the extraction of multiple properties or values.
What are default parameters in ES6?
Default parameters in ES6 allow functions to have predefined values for arguments that are not passed, ensuring the function behaves correctly even if some parameters are missing.
How would you explain the import and export statements in ES6 modules?
The import and export statements in ES6 modules facilitate modular programming by allowing developers to split code into multiple files and then import or export functionalities as required.
What are JavaScript Symbols?
JavaScript Symbols are unique and immutable data types introduced in ES6. They often serve as object property keys to ensure uniqueness and avoid overwriting existing properties.
What is the concept of iteration in ES6?
Iteration in ES6 is a new mechanism for traversing over data. New constructs like the for...of loop, are used to seamlessly iterable objects, such as arrays, strings, maps, and sets, providing a more intuitive way to loop through items.
What are JavaScript Sets and Maps?
JavaScript Sets are collections of values where each value must be unique. JavaScript Maps are collections of key-value pairs where keys can be any type. Both of these collections are introduced in ES6 to supplement traditional objects and arrays.
What is async functions in ES2017?
Async functions are an enhancement to promises, allowing to write asynchronous code in a more synchronous-like fashion using the async and await keywords.
What are JavaScript Developer Questions About JavaScript Frameworks and Libraries?
JavaScript developer questions about JavaScript frameworks and libraries are aimed at understanding a developer's proficiency in using the various tools available in the JavaScript ecosystem. These queries are designed to assess a developer's knowledge and application of specific tools, their underlying principles, and their ability to utilize these tools in creating robust applications.
JavaScript Frameworks and Libraries are collections of pre-written JavaScript code that offer predefined structures or functionalities. While frameworks provide a comprehensive template that dictates application design, libraries are more flexible, offering specific functionalities that developers can incorporate as needed.
JavaScript Frameworks and Libraries expedite development processes, ensure adherence to best practices, and address common challenges such as cross-browser compatibility, state management, and user interface design.
The most important JavaScript questions about JavaScript Frameworks and Libraries are listed below.
What is the Virtual DOM in React?
The Virtual DOM in React is a lightweight representation of the actual DOM. Virtual DOM enables efficient updates and rendering by minimizing direct interactions with the actual DOM.
How can you explain the difference between state and props in React?
The difference between state and props is that the state in React represents the internal data of a component that can change over time. Props are immutable data passed from parent to child components.
How does Angular’s two-way data binding work?
Angular's two-way data binding automatically synchronizes the model and the view. When the model changes, the view reflects the change, and vice versa, without additional code to detect and respond to these changes.
What is the difference between Angular and React?
The difference between Angular and React is that Angular is a full-fledged framework offering a wide array of built-in tools and features, while React is a library primarily focused on building user interfaces. They have different philosophies, such as Angular using two-way data binding and React opting for one-way data flow.
How can you explain the concept of a hook in React?
A hook in React is a function that lets you tap into React features, like state and lifecycle methods, from function components, making them more versatile without converting them to class components.
How do you handle state management in React?
React handles state management using component local state, context, or third-party libraries like Redux or MobX, depending on the application's complexity and requirements.
What is Vue.js and how is it different from React and Angular?
Vue.js is different from Angular and React as Vue.js is a progressive JavaScript framework for building user interfaces. Unlike React, which is a library, and Angular, a comprehensive framework, Vue offers a middle ground with an easier learning curve, combined with a flexible and modular approach.
How would you explain the concept of a service in Angular?
A service in Angular is a class that provides reusable data or functionalities across the application. It is used for tasks like HTTP requests, logging, or data sharing between components.
What is Redux and how does it work?
Redux is a predictable state management library for JavaScript applications. Redux maintains application state in a single immutable object, with changes made through pure functions called reducers, ensuring a consistent and centralized data flow.
What is the purpose of a reducer in Redux?
A reducer in Redux is a pure function that takes the current state and an action, then returns a new state. It defines how the application's state changes in response to actions dispatched to the store.
What are JavaScript Developer Questions About Web Development and Performance?
JavaScript Developer Questions About Web Development and Performance are questions related to web development and performance go beyond coding skills and explore your understanding of optimization strategies, best practices, and performance considerations that are fundamental to modern web development. The proficiency in building efficient, fast, and responsive web applications, as a JavaScript developer, is crucial.
Web Development and Performance revolve around the creation of websites and applications that not only function as intended but also deliver content and interactions quickly and seamlessly. It encompasses aspects like load times, smooth animations, and responsiveness to user actions.
User expectations are sky-high, a split-second delay can make the difference between user retention and abandonment. Therefore, developers must possess not only the skills to build but also the wisdom to optimize, ensuring users receive the best possible experience across all devices and network conditions.
The most important JavaScript questions About Web Development and Performance are listed below.
How can you explain the concept of the Critical Rendering Path?
The Critical Rendering Path is the sequence of steps browsers undergo to convert HTML, CSS, and JavaScript into pixels rendered on the screen. Optimizing this path ensures web pages render quickly and responsively.
What are the different ways to include JavaScript in HTML?
JavaScript is included in HTML using the <script> tag either inline, by placing code directly between the tags, or externally, by linking to an external .js file using the src attribute.
What are Progressive Web Apps (PWAs)?
Progressive Web Apps (PWAs) are web applications that offer native-app-like experiences with features like offline access, push notifications, and installation on the home screen, while still being accessible via browsers.
How can the performance of a JavaScript application be improved?
Performance of a JavaScript application is improved by optimizing code, minimizing DOM interactions, leveraging browser caching, deferring non-critical JavaScript, and using Webpack tool for bundling and minification.
How can you explain the concept of Lazy Loading?
Lazy Loading is a performance optimization technique where specific assets, like images or scripts, are loaded only when needed or when they appear in the viewport, reducing initial load times.
How do you ensure that your JavaScript code is cross-browser compatible?
Ensuring cross-browser compatibility involves using feature detection, leveraging Babel for transpilation, and testing the code across various browsers and browser versions.
How do you debug JavaScript code?
JavaScript code is debugged using browser developer tools, setting breakpoints, inspecting variables, and utilizing console.log() statements or more advanced methods like profiling and monitoring.
What are the common performance bottlenecks in JavaScript applications?
Common performance bottlenecks include extensive DOM manipulations, memory leaks, unoptimized images, synchronous blocking calls, and redundant or unused code.
What is the importance of Webpack in modern web development?
Webpack is vital in modern web development as it's a module bundler and task runner. Webpack bundles JavaScript files, stylesheets, images, and other assets into a single package, optimizing and transforming them for optimal performance.
How can you explain the concept of tree shaking?
Tree shaking is an optimization technique used in module bundling, where dead code, or unused modules, are eliminated from the final bundled file, resulting in smaller and more efficient output.
What are JavaScript Developer Questions About Testing and Quality?
JavaScript Developer Questions About Testing and Quality are questions to evaluate the capability of a JavaScript Developer to ensure that the code functions correctly while also meeting the highest standards of reliability and maintainability. This is where testing and quality come into play. Questions relating to testing and quality are aimed at deciphering a developer's dedication to building resilient and error-free applications.
Testing and Quality in the realm of JavaScript encompass the strategies, tools, and methodologies employed to identify issues, ensure functionality, and maintain code excellence. Testing often involves various types, including unit, integration, and end-to-end tests, while quality addresses code clarity, efficiency, and maintainability.
The most important questions about Testing and Quality in JavaScript are listed below.
What is unit testing in JavaScript?
Unit testing in JavaScript involves testing individual units or components of code in isolation to ensure that each part functions as intended.
How do you perform testing in JavaScript?
Testing in JavaScript is performed using testing libraries or frameworks, writing test cases, and then running these tests to verify code correctness.
What are some JavaScript testing libraries/frameworks?
Popular JavaScript testing libraries/frameworks are Jasmine, Jest, Mocha, Chai, and Karma.
What is Test-Driven Development (TDD)?
Test-Driven Development (TDD) is a software development methodology where tests are written before the actual code, ensuring that code is developed with testing in mind and meets defined requirements.
Explain the concept of mocking in testing?
Mocking in testing involves creating mock objects or functions to replicate and control the behavior of real objects, enabling isolated testing of specific parts without actual dependencies.
What is the difference between end-to-end testing and unit testing?
The difference between end-to-end testing and unit testing is that end-to-end testing evaluates the entire application's flow in a real-world scenario, while unit testing focuses on individual components in isolation.
What is the importance of code linting?
Code linting is crucial for maintaining code quality, as it detects and warns about stylistic errors, potential bugs, and deviations from coding standards.
How do you ensure that your JavaScript code follows coding standards?
Ensuring JavaScript code adheres to coding standards involves using tools like ESLint or TSLint, setting up custom rules, and integrating them into the development process.
What is the importance of Continuous Integration (CI) and Continuous Deployment (CD) in software development?
Continuous Integration (CI) and Continuous Deployment (CD) are vital for ensuring code consistency, automating testing, and speeding up the delivery of software updates or features to end users.
What are the key principles of writing clean code?
Key principles of writing clean code are writing readable and understandable code, keeping functions and modules short, using meaningful names, avoiding code duplication, and adhering to the Single Responsibility Principle.
What are JavaScript Developer Questions About Coding/Practical?
JavaScript Developer Questions About Coding/Practical are questions that measure the coding and practical skills of a JavaScript developer. This involves the ability to apply theoretical knowledge to real-world coding challenges, tasks, and problem-solving scenarios. Such hands-on exercises provide valuable insights into a developer's logic, efficiency, and code quality.
Coding/Practical questions revolve around real-world coding tasks and challenges. Rather than merely discussing abstract concepts, these questions require developers to demonstrate their expertise through action, crafting solutions, writing code snippets, or refactoring existing code.
The most critical coding/ practical questions in JavaScript are listed below.
Write a function to reverse a string.
Use the built-in JavaScript methods, to reverse a string .
How would you find the first non-repeating character in a string?
Iterate through the string and use a frequency counter.
How can you write a function to determine if a string is a palindrome?
A string is a palindrome if it reads the same backward as forward.
How would you merge two sorted arrays?
Use a two-pointer technique to merge sorted arrays.
How to write a function to implement a debounce?
Debounce ensures a function doesn't fire too frequently.
How to implement a basic version of Promise?
A basic Promise implementation involves resolve and reject functions.
How to write a function to deep clone an object?
You can use recursion to deep clone objects.
How to implement a function to perform a binary search on a sorted array?
Binary search halves the search interval with each step.
How would you implement a basic pub-sub system?
A pub-sub system involves subscribing to events and publishing them.
How can you write a function to calculate the Fibonacci sequence?
A recursive approach to compute Fibonacci.
Why JavaScript Developer Interview Questions are Necessary?
JavaScript Developer Interview Questions are essential to assess a candidate's grasp over the language, its nuances, and its application in real-world scenarios. Their necessity stems from JavaScript's pivotal role in web development and the need for developers to be well-versed with its vast ecosystem.
The complexity of modern web applications demands that developers not only know "how" but also "why". It's not just about writing code; it's about writing efficient, maintainable, and scalable code. Through pointed questions, interviewers can discern a candidate's approach to challenges, ensuring they align with industry best practices and standards.
How does the Interview Questions Intended for JavaScript Developers Work?
The interview questions designed for JavaScript developers are meticulously crafted to assess a candidate's depth and breadth of knowledge, as well as their problem-solving skills in the context of JavaScript development. These questions are anchored in real-world scenarios and challenges, aim to go beyond mere theoretical knowledge.
These questions encompass various facets of JavaScript - from its core concepts to advanced topics, from foundational to practical applications. This design ensures a comprehensive evaluation, highlighting both the strengths and potential gaps in a candidate's skill set. These questions can also shed light on the ability of freshers in JavaScript to assimilate and apply new information, a crucial trait in the ever-evolving domain of web development.
What is a JavaScript Developer?
A JavaScript Developer is a Software Developer who specializes in designing, developing, testing, and optimizing web applications using the JavaScript programming language.
A JavaScript Developer often works in tandem with other professionals, such as front-end and back-end developers in the realm of web development. The primary focus of a JavaScript Developer lies in implementing functionalities that enhance user experience, from simple animations to complex single-page applications. Given the expansive ecosystem of JavaScript, the developers might also be proficient in various frameworks and libraries, like React, Angular, or Vue.js, to expedite development and introduce advanced features.
Are Java Script Developers Programmers?
Yes. JavaScript developers are programmers who write, test, debug, and maintain code that is executed in web browsers, servers, and even hardware. A programmer is someone who creates computer software, and given the ubiquity and versatility of JavaScript in modern web development, mobile app development, server-side scripting, and more, JavaScript developers fit squarely within this definition.
What System does JavaScript Developer Work?
JavaScript developers predominantly work on various operating systems based on personal preference and specific project requirements. Common operating systems include Windows, macOS, and Linux.
The choice of the operating system is often influenced by the tools, frameworks, and environments that a developer is accustomed to. For instance, macOS is popular among developers who appreciate its Unix-based system and seamless integration with iOS development. Linux is favored for its open-source nature, allowing for deep customization and often used in server-side operations. Windows, with the introduction of Windows Subsystem for Linux (WSL), has become increasingly popular as it offers a blend of both worlds.
What are JavaScript App Development Tools and Software JavaScript Developers Use?
JavaScript App Development Tools and Software are essential instruments that assist developers in crafting, optimizing, and maintaining applications. Commonly used tools are listed below.
- IDEs & Editors: Visual Studio Code, Atom, and WebStorm.
- Package Managers: npm and Yarn.
- Task Runners: Grunt and Gulp.
- Frameworks: React, Angular, and Vue.js.
- Version Control: Git.
- Build Tools: Webpack and Babel.
- Testing: Jasmine, Jest, and Mocha.
How do JavaScript Developers Test their Applications?
JavaScript developers ensure application reliability and robustness through rigorous testing methodologies. They typically use the below-listed methods.
- Unit Tests to validate individual components.
- Integration Tests to ensure modules work harmoniously.
- End-to-end Tests to assess complete functionality.
- Performance Tests to measure speed and responsiveness.
What are the Benefits of JavaScript Developer Interview Questions for Hiring?
The benefits of using JavaScript Developer Interview Questions during hiring are listed below.
- Skill Verification: Ensuring the candidate possesses the necessary technical knowledge.
- Problem-solving Insight: Understanding the developer's approach to challenges.
- Cultural Fit: Evaluating interpersonal skills and team compatibility.
- Efficiency: Streamlining the hiring process by pre-filtering qualified candidates.
What are the Limitations of JavaScript Developer Interview Questions for Hiring?
The Limitations of JavaScript Developer interview questions are listed below.
- Not Entirely Indicative: A successful interview doesn't guarantee on-the-job success.
- Potential Bias: Questions might favor certain backgrounds or experiences.
- Pressure Situation: Talented developers might underperform due to interview anxiety.
- Overemphasis on Theory: Practical skills might be undervalued.
What Skills do JavaScript Developers Possess?
JavaScript developers are equipped with a blend of technical and soft skills. These are listed below.
- Technical Proficiency: Mastery of JavaScript and its ecosystems.
- Problem-solving: Efficiently addressing and rectifying challenges.
- Version Control: Utilizing tools like Git for collaborative coding.
- Frameworks Knowledge: Proficiency in React, Angular, or Vue.js.
- Soft Skills: Effective communication, teamwork, and adaptability.
How does a JavaScript Developer Different Compare to an IOS Developer?
A JavaScript developer primarily focuses on web development using the JavaScript language, crafting interactive and dynamic web applications. In contrast, an iOS developer specializes in creating applications for Apple's iOS platform, typically using Swift or Objective-C. While both aim at delivering high-quality user experiences, their domains and toolsets differ. The iOS developer deals with platform-specific challenges like adhering to Apple's guidelines, while a JavaScript developer navigates the intricacies of cross-browser compatibility.
How does a JavaScript Developer Different Compare to a Java Developer?
A JavaScript developer is engrossed in web-based application development, leveraging the capabilities of the JavaScript language, often complemented by frameworks like React or Angular. A Java developer works with the Java programming language, which is platform-independent and commonly used for a wide range of applications, from web servers to Android apps. JavaScript and Java, despite their similar C-like syntax, function in separate ecosystems. Java is a class-based, object-oriented language, whereas JavaScript is prototype-based.
How does a JavaScript Developer Different from a PHP Developer?
A JavaScript developer, especially one focused on the frontend, uses JavaScript to create interactive elements and enhance the user interface of web applications. PHP developers work on server-side scripting, building the backend logic of websites using the PHP language. Both can be used for web development, JavaScript often manages dynamic content delivery on the client side, while PHP handles server-side operations and database interactions. There's overlap when JavaScript developers venture into Node.js, bringing JavaScript to the server side, converging some aspects of their role with PHP developers.
Is JavaScript Development Open Source?
Yes. JavaScript development is open-source. Many tools, libraries, and frameworks in the JavaScript ecosystem are available as open-source projects, meaning developers can freely access, modify, and distribute their source code. This open-source nature has fostered a vibrant community, leading to the rapid evolution of tools and best practices within the JavaScript world.
Is JavaScript Development Front-End?
No. While JavaScript originally gained popularity as a front-end language for enhancing web interactivity, it has expanded its realm. With the advent of technologies like Node.js, JavaScript is now a powerful server-side language as well, enabling full-stack development. Thus, JavaScript development encompasses both front-end and back-end domains.
Are JavaScript Developers Considered Software Engineers?
Yes. JavaScript developers are considered software engineers . They design, implement, test, and maintain software applications, which are fundamental tasks of software engineering. With the increasing complexity of web applications and the broader roles JavaScript developers play in the full cycle of software development, their position as software engineers is well-established.
How Much do JavaScript Developers Make?
The average salary for a JavaScript developer in India is ₹7,51,785 per year. The average additional cash compensation is ₹1,46,359, with a range from ₹40,000 - ₹3,67,618. The salary of a JavaScript developer can vary based on several factors, including experience, location, and specific domain expertise. JavaScript developers are in high demand due to the ubiquity of web applications, leading to competitive compensation packages in many regions.
Entry-level JavaScript developers can expect to earn a different wage compared to mid-level or senior developers on a global scale. Location plays a crucial role; for instance, developers in tech hubs like Silicon Valley may earn significantly more than those in regions with a lower cost of living. Specialization in certain JavaScript frameworks or libraries, like React or Angular, can command higher salaries due to niche expertise.
Where to Find a JavaScript Developer?
Finding a JavaScript developer involves various channels, depending on the specific needs, project requirements, and organizational preferences. The proliferation of web technologies and the ubiquitous nature of JavaScript ensure a broad pool of talent available worldwide.
Several platforms and networks cater specifically to tech talent. Websites like Stack Overflow, GitHub, and specialized job boards like JSJobs highlight developers' skills and contributions, making it a hub for scouting talent. Similarly, professional networks like LinkedIn feature profiles of JavaScript developers with their portfolios, making candidate evaluation efficient. Tech conferences, hackathons, and meetups are excellent venues to meet passionate developers in person. For organizations preferring a more structured approach, hiring agencies specializing in tech roles can streamline the recruitment process, ensuring that only the most qualified candidates are shortlisted. Regardless of the chosen avenue, understanding project requirements and aligning them with a developer's expertise is crucial to ensure a successful collaboration.
Can a JavaScript Developer Work Remotely?
Yes, a JavaScript developer can work remotely. With the ubiquity of online collaboration tools, cloud platforms, and version control systems like Git, JavaScript developers can efficiently collaborate, code, and deploy applications from anywhere in the world. The nature of web development, combined with the digital tools available, makes remote work a viable and often preferred option for many JavaScript developers.
Do JavaScript Developers Need Continuous Learning?
Yes, JavaScript developers need continuous learning. The world of web development, and particularly JavaScript, is dynamic and rapidly evolving. New frameworks, tools, and best practices emerge regularly. JavaScript developers must stay updated with the latest advancements, adopt new methodologies, and continuously hone their skills, to remain relevant and efficient. This ongoing learning ensures they deliver optimal solutions and keep pace with the ever-changing landscape of web technology.

How does Flexiple Help you Find the Right JavaScript Developer?
Flexiple is the perfect platform to discover the ideal JavaScript developer for your project. Here's how Flexiple can assist you in the following ways.
- Curated Talent: Flexiple carefully selects top-tier JavaScript developers, ensuring that every developer undergoes a rigorous vetting process to guarantee access to the best talent available.
- Efficient Matching: Based on your project's specific requirements, Flexiple swiftly connects you with JavaScript developers who possess the precise skills and experience necessary for your project's success.
- Flexible Engagements: You have the flexibility to hire JavaScript developers on a full-time or part-time basis, tailored to your project's unique needs. This adaptable approach ensures you have the right developer for the required duration.
- Quality Assurance: Flexiple provides comprehensive support throughout your project, ensuring the delivery of high-quality results. The platform serves as an intermediary to ensure your project progresses smoothly and effectively.
- Transparent Pricing: Flexiple offers clear and transparent pricing models, empowering you to manage your budget effectively for your JavaScript development requirements.
- Flexiple streamlines the process of finding the perfect JavaScript developer, whether you're tackling a short-term project or embarking on a long-term engagement. With Flexiple, you'll have the convenience and reliability needed to kickstart your JavaScript project.
Is it Easy to Hire a JavaScript Developer with Flexiple?
Yes. It is easy to hire a JavaScript developer with Flexiple. Flexiple's carefully selected talent pool, streamlined matching, adaptable engagement alternatives, and quality assurance streamline the search for the perfect JavaScript developer for your project. The platform simplifies the hiring process, ensuring prompt connections with highly skilled JavaScript developers who meet your project's precise needs. Featuring transparent pricing and comprehensive support, Flexiple offers a hassle-free experience when it comes to hiring JavaScript developers.
How can a JavaScript Developer Join Flexiple?
JavaScript developers are welcome to join the Flexiple network to gain access to high-paying jobs with vetted companies by following the below steps.
- Create your profile: Creating your profile has never been easier! Build your professional identity effortlessly by sharing your skills, experience, and aspirations with us. Simply click the link to create your profile.
- Choose from the Flexiple network: Looking for your dream job? We've got you covered! With our curated list of job opportunities that match your profile and preferences, you can choose from the best of the best.
- Work on your dream job: Ready to take the first step on your career journey? Secure your ideal role and start working on your dream job as a JavaScript developer. Let us help you pave the path to success!
Interview Resources
Want to upskill further through more interview questions and resources? Check out our collection of resources curated just for you.
- Salary Insights
- Hourly Rate Guide
- Tech Deep-Dive
- Interview Questions
- Job Description
- Certifications and Courses
Browse Flexiple's talent pool
While you build a world-class company, we help you assemble a dream tech team. Because, you deserve it.
- Programmers
- React Native
- Ruby on Rails
- Other Developers
Smart Devpreneur
Exploring JavaScript Libraries
50 Difficult JavaScript Questions to Test Your JS Knowledge
I meticulously searched the JavaScript docs to create these questions. I hope they push the limits of your JS knowledge. Let me know in the comments if you enjoyed this list!
Related: Test your TypeScript knowledge against these 50 difficult TypeScript questions .
1. What will the code below output to the console and why?
It will print Joe . The peopleArray has three elements, but all three elements are references to the same memory space, which is the person object. The following will create an array with three unique objects and outputs Ben .
2. What will the code below output to the console and why?
It will print the object below. Any property names common to both destructured objects will be overwritten by the second object.
3. Will the following code compile? If not, why not?
The code does not compile. Interestingly, Line 3 fails because let myName is not initialized to undefined (unlike var myName would have been). If Line 4 were removed, then Line 3 would fail due to the inability to override myName declared as a constant outside the code block. Constants cannot be changed.
4. What will the code below output to the console and why?
The code will actually throw an error. for…of can only be used on objects that implement the @@iterator method . See this great article that covers four different ways to loop in JavaScript for more information. for…in would have printed off each key:value pair.
5. Write an arrow function that successfully prints “hello world” given the following function call:
Here is one way to accomplish this:
The questions continue below, but first I recommend you check out my book, 300 JavaScript Interview Mastery Questions (This link and the links below are affiliate links for which I might be compensated). The questions explore a wide variety of JavaScript syntax, functions, APIs, and theory. You WILL learn something new in this book and be better prepared for your next interview.

6. What will the code below output to the console and why?
The code will output [9, 2]. Array.splice returns the original array, but Array.slice returns a new array, so changing arrTwo does not affect myArr.
7. What will the code below output to the console and why?
The output will be ‘Fort Worth’. Creating a new object using … is the equivalent of using Object.assign({}, object); and creates a shallow copy. person1 and person2 are not the same object, but both person1.address and person2.address are pointed to the same object in memory. See this article for a deeper explanation of shallow and deep copies in JavaScript.
7. What is the difference in the ‘~’ and the ‘^’ in the semver syntax , such as that used in npm package.json file?
Semver syntax goes as follows: major.minor.patch. The ‘~’, such as “lodash”: “~1.2.0”, will only update the patch version. The ‘^’, such as “lodash”: “^1.2.0”, will update the minor and patch version.
8. Name a JavaScript String function that modifies the original string.
Admittedly, this is a trick question. Strings are immutable in JavaScript, so there are no functions that modify the original string. There are some functions that extract or change portions of the original string and return it as a new string, leaving the original string unmodified.
9. True or False? In the code below, myColor is initialized with a boolean value of true .
This is false. The || operator does not actually return a boolean value, but rather the first truthy value it encounters. ‘Purple’ is a truthy value, so myColor is initialized to ‘Purple’.
10. Optimize the following code:
Here is a simple optimization:
Many devs write redundant lines of code, forgetting that ‘===’ itself returns a boolean.
11. Given the below example, what is the result of setting enumerable: false in Object obj?
Object obj will not be enumerated over in a for…in loop.
12. What will the code below output to the console and why?
The code will output {a: 1, b: 1, c: 1, d: 2}. Object.assign can take multiple sources, and later sources overwrite earlier sources.
13. What will the code below output to the console and why?
It will throw an error saying ‘cannot access obj1 before initialization’. The code is attempting to immediately invoke the arrow function at prop2 while obj1 is still being initialized.
14. Consider the code below. How can object properties be accessed using a variable?
console.log(obj1[key]); will access prop1 on obj1 . This is useful in many situations, such as when enumerating using a for…in loop.
15. What are some limitations placed by using Strict Mode in JavaScript?
Take a look here for an exhaustive list. Most of the limitations revolve around variables. For example:
- all variables must be declared (the compiler won’t assume an undeclared variable is a global
- reserved words cannot be used as variable names
- certain preceding values are not allowed, such as let badVal = /010;
- deleting undeletable properties is not allowed
16. Consider the code below. Do arr4 and arrControl have the same values?
They do indeed have the same values: [“y”, “m”, “m”, “i”, “j”] . It doesn’t matter whether the individual function returns are assigned to a const and then used, the result is the same either way.
17. Consider the code below. Do Line 8 and Line 9 log the same value to the console?
Line 8 logs a value of 36. Line 9 initially logs a value of 30 and then throws an error. innerMemoryFunction is assigned a value of (z) => { console.log(y*z); } . This function has captured the closure value of y = 5 and uses that value when calculating.
18. What will the code below output to the console and why?
At first glance you may try to evaluate whether 1 && 0 is equivalent to 1 || 0 . However, that’s not what the above code is doing. The code above is actually equivalent to the below:
Which is the same as:
The || operator returns the second value it checks when the first value is false, and since 1 && false is falsy, the above returns 0 .
19. What are two ways to push elements of one array into another array using only one line of code?
Here are two possible ways to do it.
This is simply just destructuring assignment.
This option is a little more complex. arr.push on line 2 simply calls the push function. The first param (arr) in the apply function actually tells the compiler which array to call the push on.
19. Consider the code below. Why doesn’t toString throw an error even though we have not defined it on Car?
toString is an instance method of Object. Car is of type Object, so it has all methods that Object has.
20. Consider the code below. Why does Line 1 return true while Line 2 returns false ?
These evaluate as follows for Line 1:
- null == undefined // true
- true == 0 // false
- false == ” //true
So Line 1 returns true
Line 2 then evaluates true == NaN which is false.
21. Can functions be passed as parameters?
Yes, and here is an example:
This is an example where TypeScript would provide value. A developer looking at the Person constructor might guess that getUniqueID should be a function, but if the parameter had a type it would significantly reduce opportunity for confusion and bugs.
22. Why should developers use ‘===’ instead of ‘==’?
‘===’ is stricter and checks if value and type are the same, ‘==’ only checks value and will do type coercion. But WHY do we want it stricter? Because developers need to write code that works as intended. Higher standards on code is a good thing and ensures developers don’t get sloppy.
23. True or False? A catch block is required after a try{}.
This is false. However, either a catch block or a finally block is required after a try{}.
24. Why does console.log(~8) output -9?
~ is a bitwise operator that flips bits in the bit representation of a number. In JavaScript all bitwise operations are performed on 32-bit binary numbers.
8 is represented as 00000000000000000000000000001000. The ~ operator flips this to 11111111111111111111111111110111, which is -9
25. What will the code below output to the console and why?
This will throw a syntax error. The return statement is not a valid way of ending a for block. If you want to end a loop, use break.
26. What are two methods for extracting the keys from an Object?
There are a handful of ways this could be accomplished, but two are below.
Object.keys(obj1) will extract the keys and return an array populated with a string representation of the keys.
Using for…in , each key is iterated over and a string representation of its value is set to the constant ‘key’ in the above example.
27. What will the code below output to the console and why?
It prints “4/1/1995, 12:00:00 AM” . When only a year is provided, it maps to the 20th century. Also, January is month 0. Therefore, new Date(95, 3) creates a Date object set to April 1995.
28. What will the code below output to the console and why?
It will print ‘object’. We have created a string Object, which is different than a string primitive in JavaScript.
29. What will the code below output to the console and why?
The output is 5 . The timeout callback is not evaluated until 1000 milliseconds have passed, so x is never set to 10. It is only set once, to 5, and that is when the callback is evaluated.
30. Will the below code execute?
It will not. When attempting to immediately invoke an arrow function, don’t forget the needed parenthesis, like below:
31. What will the code below output to the console and why?
The output is “function”. While this may seem strange at first, Object is the name of the Object constructor function.
32. Will the following code run?
The code will not run, but instead has a compile time error. let does not get hoisted, but the compiler doesn’t try to use the hoister variable specified outside the function.
33. What does the accumulator do in Array.prototype.reduce()?
The return value of the each iteration of the reducer function is assigned to the accumulator and remembered across each iteration of the array.
34. A user is typing into a textbox, and the onChange is being fired more often than desired. What function could be used to only fire the onChange at the end of the user’s typing?
debounce . The debounce function forces a function to wait a certain amount of time before being called or before being called again. Scroll events are another common use for debounce.
35. What function tells the browser to call a specified function to update an animation before the next repaint?
window.requestAnimationFrame() . This is a useful function for making animations render more smoothly and more often.
36. If a button exists inside a div, and both the button and div have click listeners, which one handles the event first? Do they both handle the event?
The button click handler will be invoked, then the div click handler will be invoked. If you do not wish for the div to also have it’s click handler invoked, add event.stopPropagation() in the button click handler.
37. What will the code below output to the console and why?
This will output false . The value false will be coerced to 0 , and NaN(0) will return false.
38. Given that console.log(new Number(5)); logs the value 5 , what will the code below output to the console and why?
This logs the value false . new Number(5) is an object, when it is logged to the console it’s toString() method is called so the value 5 is logged.
39. Write a function that determines if a character is an upper case letter.
One possible solution:
40. What convenience method was added with ES6 to arrays to create an array from array-like and iterable objects?
Array.from . Consider the following example:
41. What will the code below output to the console and why?
This will output [“April”, “May”, “daylight”] . Adding parenthesis around the element param is not required because it is the only parameter passed in. Also, the return of the arrow function has an implied return when there are no brackets.
42. Evaluate the code below. Does it print as intended or throw an error?
The code indeed prints one two three as intended. The parameters are passed in and destructured. Then interpolation is used to construct the string.
43. What is the difference between the two imports in the below code?
In the first import, exampleLib must have this syntax: ‘export default Example…..’. This is what is known as a default export from a package. In the second import, Example is a named export instead of the default export. The exampleLib package would need the following syntax: ‘export const Example….’ Functionally speaking, there’s not much difference other than syntax.
44. What will the code below output and why?
It will print step 3 . Switch statements fall through all remaining steps after the first ‘true’ case they encounter, until the switch statement encounters a break . In the example, there was no break statement, so switcheroo was assigned ‘step 1’, then assign ‘step 2’, then assigned ‘step 3’.
45. True or False? event.preventDefault() stops event propagation.
The answer is False. preventDefault prevents the default action from being taken but does not prevent propogation.
46. What is the syntax for assigning a default parameter value?
It is as simple as parameter b in the below example:
47. What will the code below output to the console and why?
This will output testB . Other properties are accessible within an object’s function properties depending on when the function is invoked.
48. What is the syntax for creating a property that can never be changed in an object?
49. What will the code below output to the console and why?
The code will print “thing 1”, “thing 2”, “thing 3”, and “not a thing”. It does not fail, but the results may be unexpected. for…in loops through the keys of an object, and in this case thing4 has been added to the myArray object.
50. What does Line 5 of the code below output to the console?
Line 5 prints the following:
done: true is not printed until Line 6.
6 thoughts on “50 Difficult JavaScript Questions to Test Your JS Knowledge”
let name=’GANESH’ console.log(name.toUpperCase() ===name);
I feel this is the best way to check if a string is upper case
That’s a great solution too!
can you explain from where the value 5 comes into the picture const memoryFunction = (x) => { const y = x; return (z) => { console.log(y * z); } }
const innerMemoryFunction = memoryFunction(5);
memoryFunction(6)(6); // Line 8 innerMemoryFunction(6)(6); // Line 9
Sure Rohan,
Take a look at this line: const innerMemoryFunction = memoryFunction(5); 5 is passed into memoryFunction, and y is then assigned a value of 5. This value is ‘remembered’ when the inner function is returned and assigned to innerMemoryFunction.
Very good interview questions. Thanks
Really Helpful Thanks!!
Leave a Comment Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed .

JavaScript Practice for Beginners: 8 Free JavaScript Exercise Sites
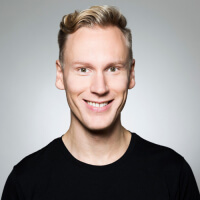
updated Apr 17, 2024
What are the best sites for JavaScript practice for beginners?
Where can you find the best JavaScript exercises and coding challenges?
Whether you are new to coding or have been learning for a while already, you want to have a go-to list of fun JavaScript practice sites to improve your skills continuously.
Because the truth is:
The only way to become a skilled JavaScript developer is to use the language for as many real-world projects as you can.
Sure, you will start small in the beginning. But as your skills progress, you will build more complex projects that require a deeper understanding of the language.
To help you get started, I’ll share my favorite JavaScript practice sites with free and fun JS exercises, challenges, and quizzes in this guide.
By the time you finish reading it, you will have a list of go-to resources to brush up your JavaScript and discover new ways to use the language for real-life projects.
If you truly want to stand out from others and become a professional JavaScript developer, these websites can help you take your skills to the next level.
FYI: I’ve already covered JavaScript projects for beginners in another article . If you haven’t already, definitely check it out, too.
Now let’s get started!
Please note: This post contains affiliate links to products I use and recommend. I may receive a small commission if you purchase through one of my links, at no additional cost to you. Thank you for your support!
What is JavaScript? What can you do with JavaScript?
If you’re not familiar with JavaScript, let’s take a few moments to go through the fundamentals first.
JavaScript is a programming language used for making websites more interactive and dynamic.
What does that mean exactly?
To understand how JavaScript works, you need to know how front-end developers build websites in general .
Here’s a quick overview of the three languages used for creating and building web pages:
- HTML or HyperText Markup Language: You can use HTML to create the basic structure and the content for a web page: menu bar, content area, sidebar, bottom footer, etc.
- CSS or Cascading Style Sheets: CSS allows you to select individual HTML elements and change their appearance. In other words, CSS makes HTML pretty.
- JavaScript : JS takes those static HTML/CSS elements and adds life to them. For example, it allows you to create elements that the user can interact with, such as drop-down menus, contact forms, modal windows, animations, interactive maps, and more.
All in all, JavaScript makes websites more engaging and interesting.
For more details, make sure to read my complete guide on front-end and backend web development .
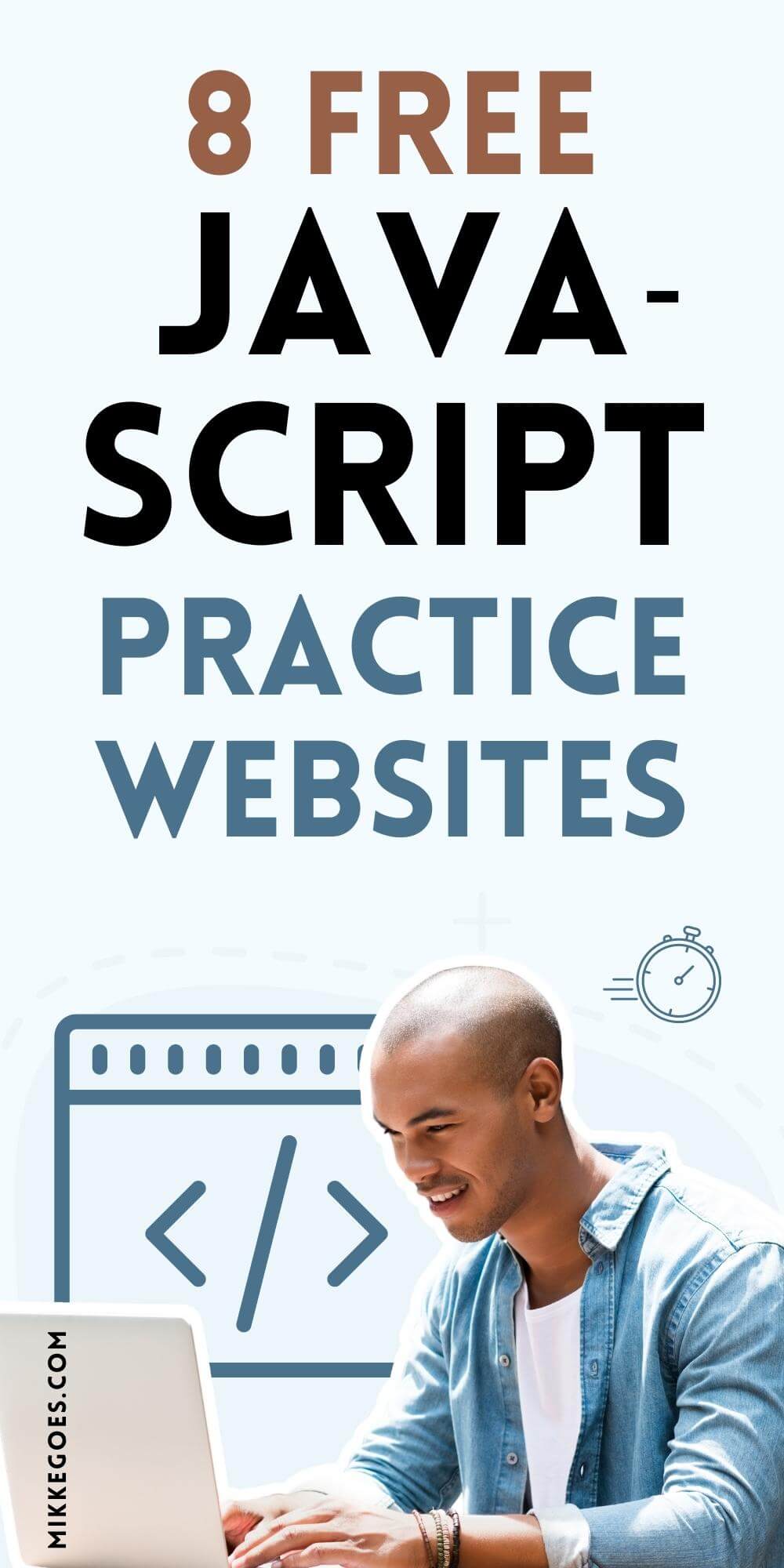
Why learn JavaScript?
If you are new to coding and not sure whether JavaScript is the right programming language to learn, don’t worry.
Before you choose your first programming language , make sure you know what you want to create and build with code in the future.
Depending on your specialization, you need to learn a specific set of programming languages and other tools.
Hence, only learn JavaScript if it’s a language you can use for the type of projects you want to work on.
With that said, here are 5 good reasons to learn JavaScript this year:
1: JavaScript is in high demand
If you are serious about becoming a JavaScript developer, you are going to love the fact that JavaScript is one of the most sought-after skills in tech today.
According to the Devskiller IT Skills Report , 75% of companies are looking for JavaScript developers:
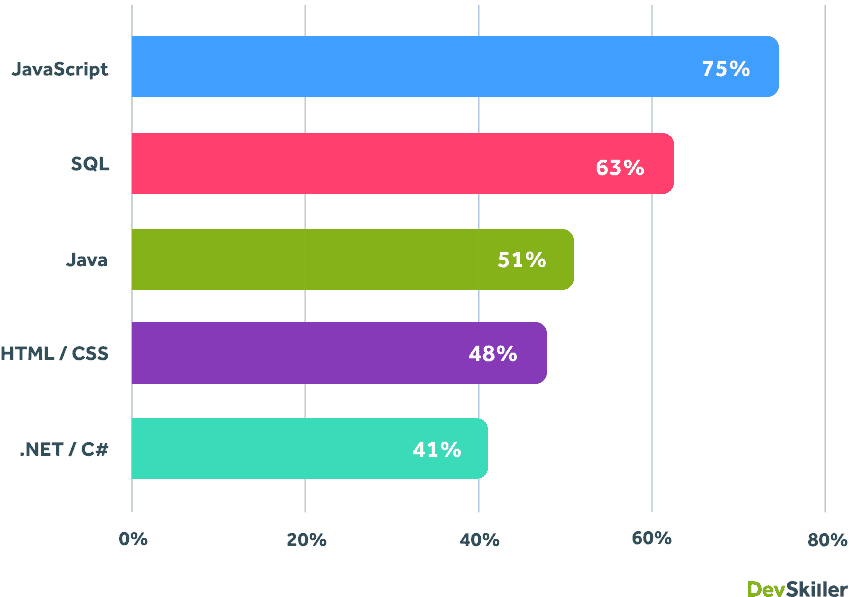
Furthermore, searching for “JavaScript developer” on the Indeed job portal returns over 20,000 jobs requiring this skill – alone in the US.
2: JavaScript developers are well-paid
So we know that there are plenty of job openings for skilled JavaScript developers. But how much do they make?
According to Indeed, the average base salary for JavaScript developers in the US is $110,687 per year .
You can find out more in this super comprehensive JavaScript salary guide .
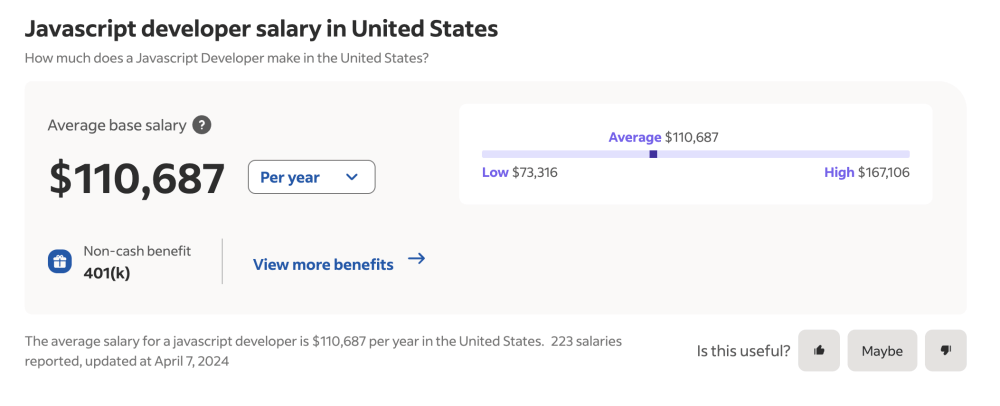
3: JavaScript is beginner-friendly
If you are new to coding, I recommend picking a language that allows you to focus on learning how coding works and how you can build your own, useful projects asap.
Choosing an easy language means you will spend less time troubleshooting your code and trying to understand the syntax. Thus, you’ll have more time to actually learning how to code.
Luckily, JavaScript is one of the easiest programming languages for beginners .
Now, while the syntax isn’t always the easiest to grasp, you will always find answers to your JavaScript questions thanks to the massive global community of JavaScript experts. This helps you troubleshoot your code faster and makes learning how to code much easier.
4: JavaScript is versatile
JavaScript is an extremely versatile programming language. You can use it for front-end web development to create stunning website interfaces, but also for backend coding using Node.js.
Also, you can develop web apps, mobile apps, and desktop apps using React, React Native, and Electron.
If you want to become a front-end web developer , JavaScript is a must-have skill.
But you could use your JavaScript expertise in the future to work as a full-stack developer, software engineer, or game developer, for example.
Hence, even if you are new to coding and not sure what you want to do in the future, JavaScript doesn’t restrict you to just one area in tech.
5: You can learn JavaScript for free
Last but not least, learning JavaScript doesn’t have to be expensive!
If you are new to coding, I recommend starting with free resources to get a hang of how JavaScript works. You will find tons of ideas in my guide with 100+ free places to learn how to code .
If you enjoy working with the language, consider investing in a paid course that includes as many practical coding projects as possible.
Building small projects and solving coding problems is the only way to improve your skills and learn how to use JavaScript for building real-life projects.
Therefore, you want to pick a course that offers as many portfolio-ready projects as possible , such as these two courses on Udemy:
- JavaScript Web Projects: 20 Projects to Build for Your Portfolio You get to build 20 practical portfolio projects throughout the course – even if you are entirely new to programming. That kind of a portfolio is going to look great when you apply for your first entry-level job.
- Modern JavaScript From The Beginning Whether you’re a beginner or an established JS developer already, this course has something for everyone. You will build 10 portfolio projects throughout the course, ranging from a simple calculator to more demanding exercises.
For more resources, check out these recommended JavaScript courses and tutorials .
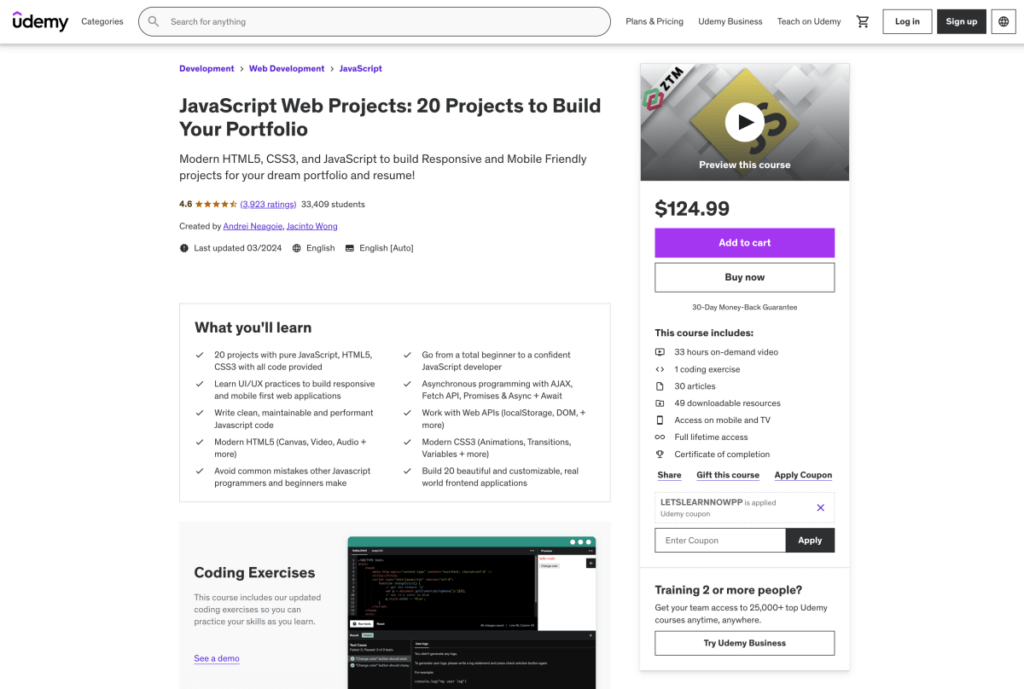
JavaScript practice for beginners: 8 free sites with JavaScript exercises
Here are the 8 top JavaScript practice sites we’ll cover in this post:
- Learn JavaScript
- freeCodeCamp.org
- JavaScript Hero
- Learn JavaScript on Codecademy
- Khan Academy: JavaScript Quiz
Remember to share and save this guide for later!
1. Edabit JavaScript Challenges
Edabit offers 2,500+ hands-on JavaScript practice challenges ranging from very easy to expert-level tasks.
You can practice directly in your web browser, and all exercises are free.
Creating an account isn’t necessary, but you can track your progress more easily when logged in. Also, you will get access to the solutions for all practice challenges as a registered user.
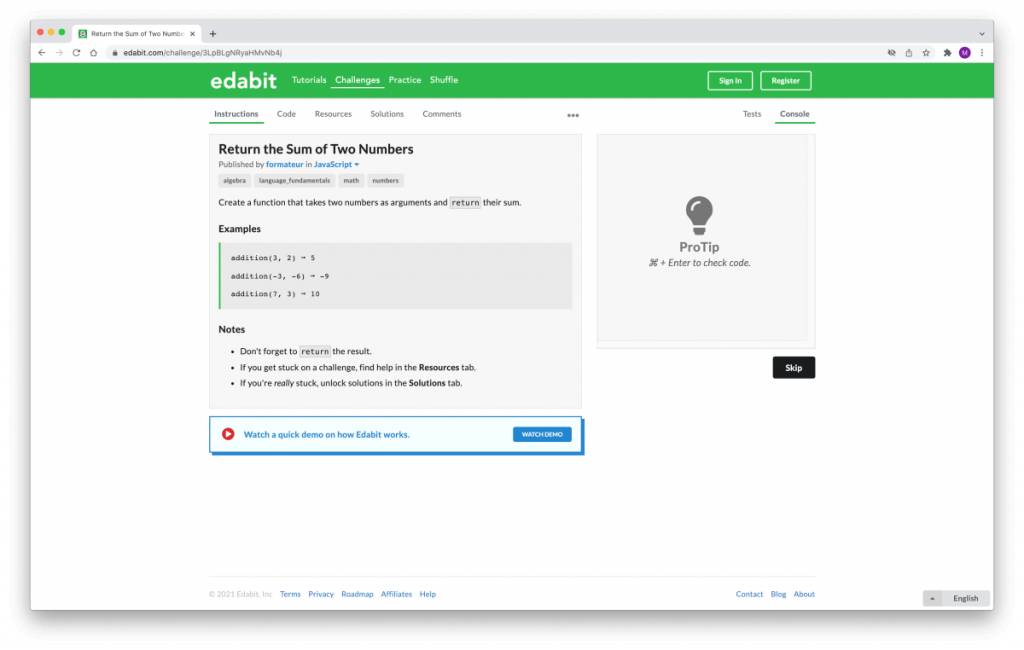
In addition to JavaScript, you can also find practice exercises for C#, C++, Java, PHP, Python, Ruby, and Swift on Edabit.
Price: Free
Skill level: Beginner to expert
→ Start practising JavaScript with Edabit here
☝️ back to top ☝️
2. w3resource JavaScript Exercises, Practice, Solution
w3resource’s JavaScript practice directory includes hundreds of free JavaScript challengers ranging from beginner to intermediate in difficulty.
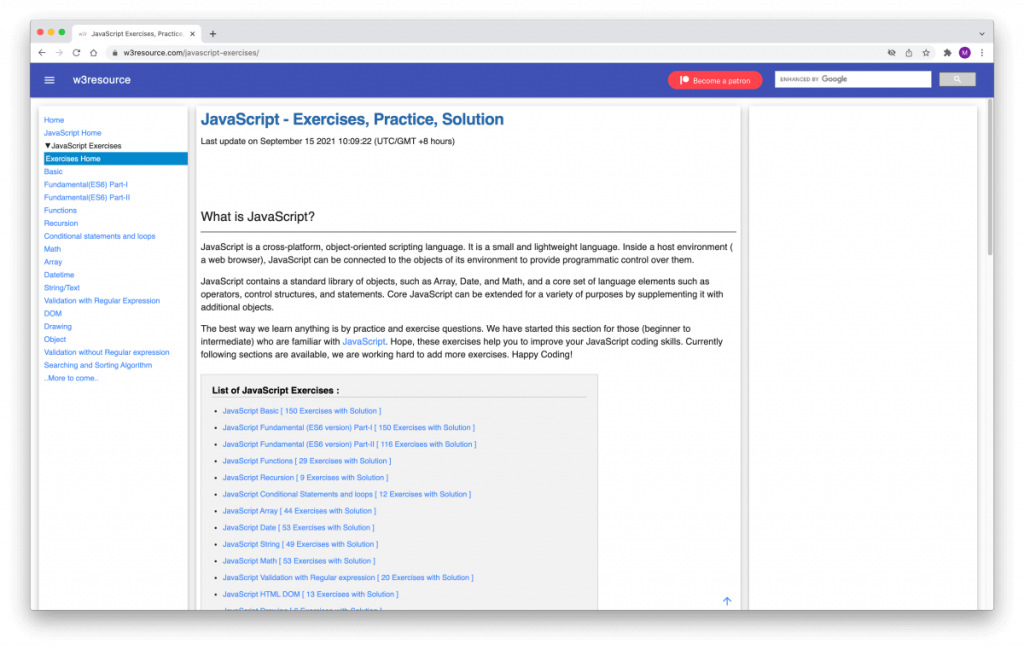
If you are entire new to coding, make sure you are somewhat familiar with JavaScript and its syntax before these exercises.
One of the best places to learn JavaScript is freeCodeCamp.org , for example. Their courses are 100% free and you can start learning in 2 minutes.
w3resource also offers free tutorials for other popular technologies, such as:
- Responsive web design
Skill level: Beginner to intermediate
→ Start practising JavaScript with w3resource here
3. Learn JavaScript
If you are looking for a complete online course with beginner-level JavaScript practice exercises, Learn JavaScript is definitely worth checking out.
Their JavaScript course covers the fundamentals of the syntax, giving you a solid foundation for practising your skills while building real-life projects as you go.
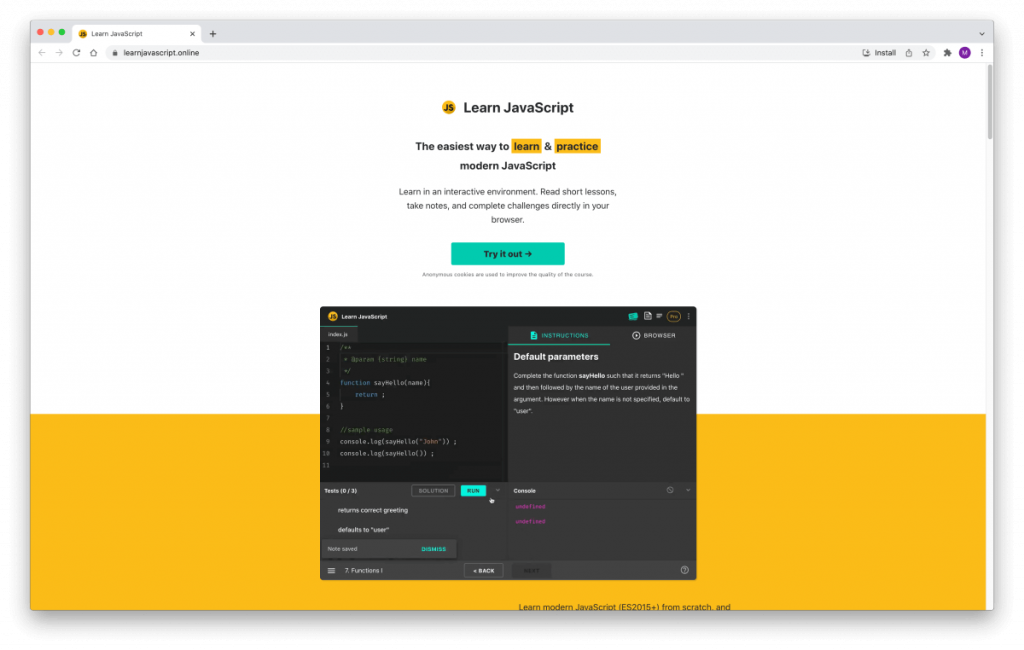
The lessons are to the point, fluff-free, and you will get a quick recap after each module to support your learning.
You can practice JavaScript directly in your web browser, solving interactive challenges and building meaningful coding projects.
Price: Free trial, Pro plan unlocks all content
→ Start practising JavaScript with Learn JavaScript here
4. W3Schools JavaScript Exercises
W3Schools is one of the best places to find free JavaScript exercises and practice challenges.
If you are entirely new to JavaScript, start learning with their free JS tutorial for absolute beginners . You will go through quick lessons to learn the syntax and apply what you learn right away to hands-on coding exercises and quizzes.
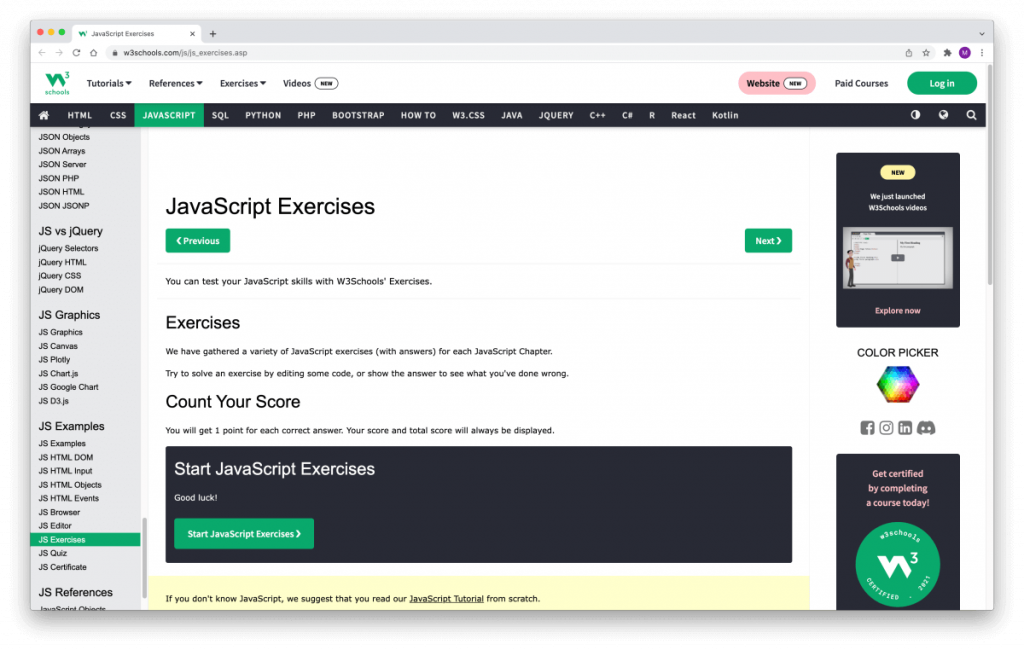
If you are new to coding, W3Schools also offers free tutorials in other popular languages like:
→ Start practising JavaScript with W3Schools here
5. freeCodeCamp.org
freeCodeCamp is one of the best websites to learn to code from scratch, and they offer a ton of free JavaScript practice material ranging from beginner to intermediate level skills.
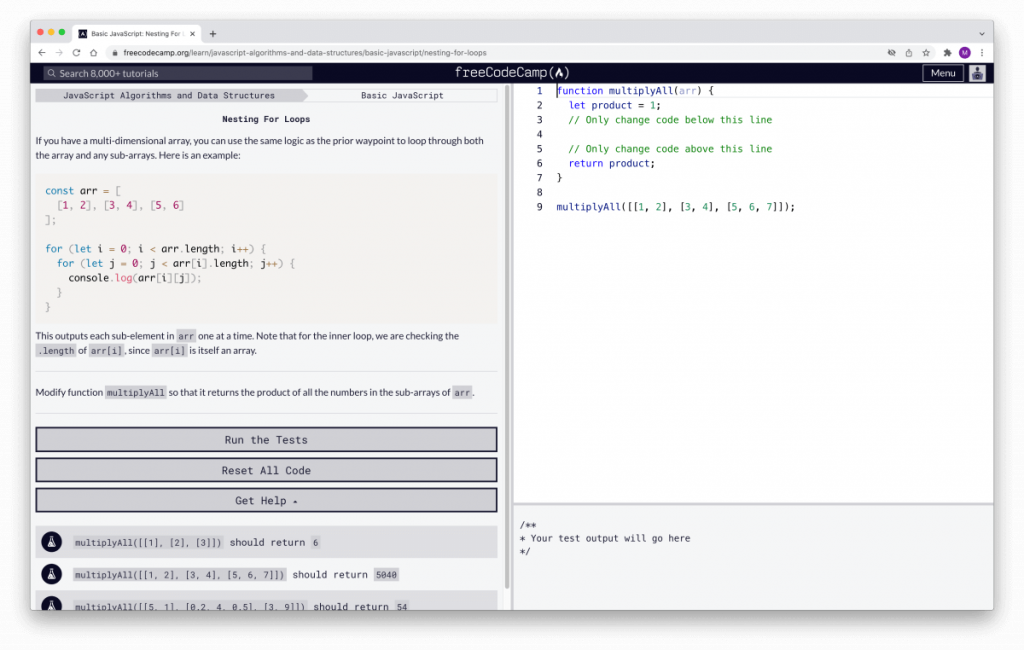
The freeCodeCamp curriculum starts with HTML and CSS, after which you will start learning and practising JavaScript.
Hence, if you are new to programming, remember that you need to learn at least some HTML and CSS before tackling JavaScript. That’s another reason why freeCodeCamp is a fantastic place to start. You can learn everything in one place – for 100% free.
→ Start practising JavaScript with freeCodeCamp here
6. JavaScript Hero
JavaScript Hero is a simple but effective JavaScript practice platform for beginners. You will start with the basics and learn how variables, functions, parameters, and other syntax fundamentals work.
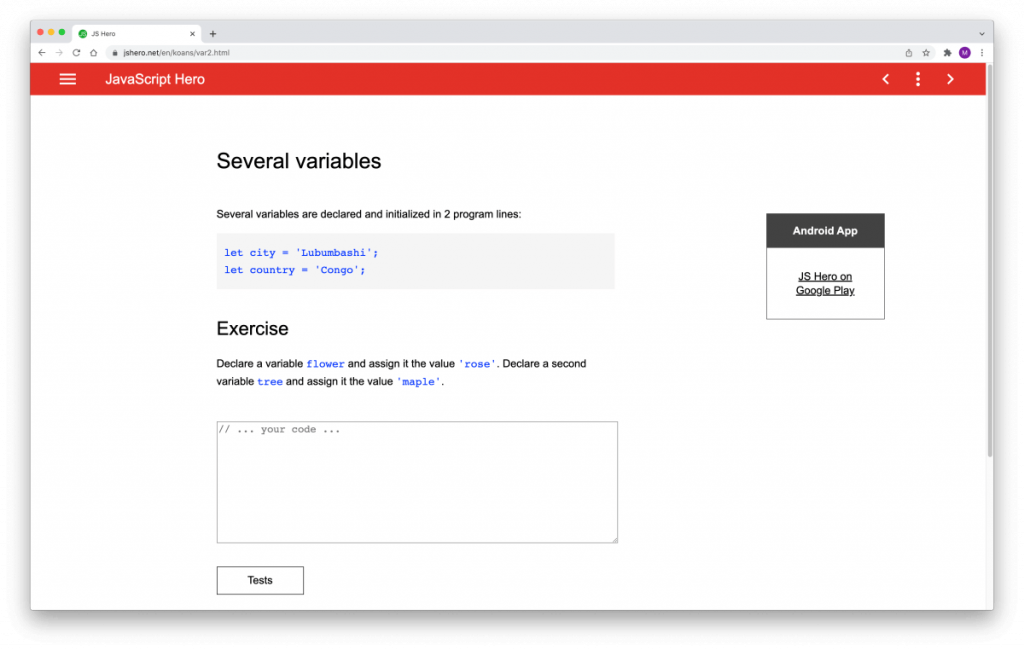
Each lesson includes a quick exercise based on what you just learned. If you run into difficulties, you’ll find a helpful hint for each exercise. Also, JavaScript Hero provides a solution for all lessons and practice challenges.
→ Start practising JavaScript with JavaScript hero here
7. Codecademy: Learn JavaScript
Learn JavaScript is Code c ademy’s free JavaScript course for beginners. Although the course is free per se, you only get access to a limited number of JavaScript exercises, practice challenges, and projects. If you are new to coding, this is more than enough to get started.
And don’t forget to grab your free JavaScript cheatsheet on Codecademy, too.
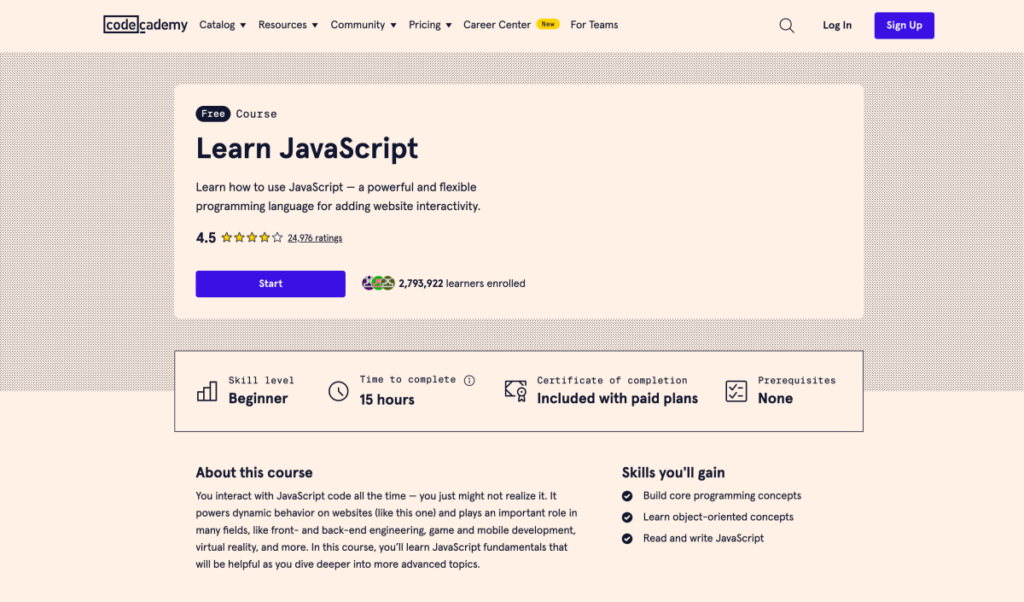
If you enjoy learning with Codecademy, you can unlock the entire course catalog with hundreds of real-life projects with the Pro plan .
Codecademy Pro also gives you unlimited access to the Front-End Engineer career path that teaches you everything you need to know to create beautiful web experiences and start a career as a front-end developer.
If you aren’t familiar with them, you can learn more in my complete Codecademy review .
Price: Free (with limited content), Pro plans start at $19.99 when paid annually
Skill-level: Beginner to intermediate
→ Start practising JavaScript with Codecademy here
8. Khan Academy: JavaScript Quiz
Khan Academy is a free online course platform for popular topics such as computer programming , math, economics, and science. Although a lot of their content is aimed at kids and students, it’s perfectly suited for any age.
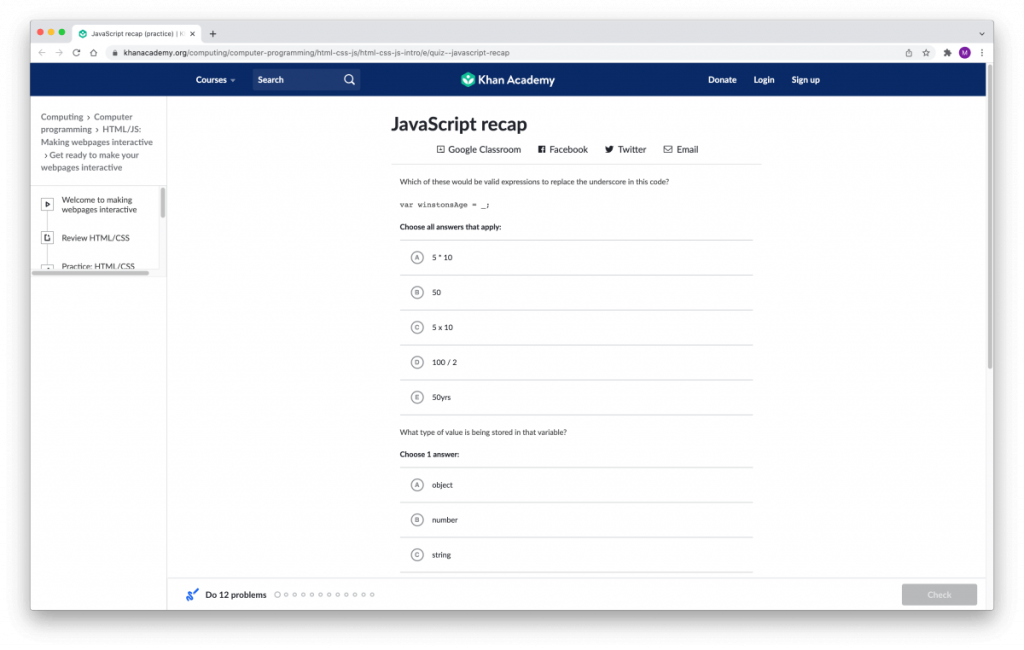
Their free Intro to JavaScript course teaches you JS from the ground up, even if you are completely new to tech. You will learn about variables, data types, functions, conditionals, loops, and more.
After the course, you can finish a set of JavaScript practice challenges to test your skills.
→ Start practising JavaScript with Khan Academy here
FAQ: JavaScript practice exercises
Some of the best places to practice JavaScript include Edabit , w3resource , freeCodecamp.org , Codecademy , and W3Schools . They all offer free JavaScript exercises and coding challengers for beginner and intermediate skill levels.
The best way to practice JavaScript is to learn the theory and syntax first. As you go, practice your skills with simple exercises, and start building more complex projects as your skills improve. The concepts build on top of each other. Thus, make sure you feel comfortable with a given topic before moving on to the next one.
If you are new to JavaScript, the easiest way to practice is to take breaks from following tutorials. Take notes of what you learn and try to solve small coding problems with JavaScript on your own without referring to your course or tutorial. Hence, start very small and only proceed to the next topic once you feel comfortable with what you just learned.
You can learn how JavaScript works in 1 week, yes. You may feel comfortable enough to solve small, simple coding problems with JavaScript after a few days of learning the syntax. But it takes much longer to become job-ready and to launch a career as a JavaScript developer. Hence, one week is enough to get an understanding of whether you enjoy working with JavaScript and if you want to continue learning more about it.
In theory you can, yes, but it wouldn’t make much sense. JavaScript is so closely intertwined with HTML and CSS that you shouldn’t learn just one of them. Remember, JavaScript is used to make static websites more dynamic, interactive, and engaging. Without HTML and CSS, you won’t have anything on your website to apply JavaScript to.
Whether you learn Java or JavaScript depends on what you want to build with code in the future. If you want to become a front-end web developer and create gorgeous websites, JavaScript is a must-have tool in your skillset. Java is used for back-end (server-side) development. Thus, if you are more interested back-end programming and want to build desktop apps or Android mobile apps, for example, Java can be a valuable skill on the job market.
I have written a separate article with the best JavaScript project ideas with source code – check it out!
What you need to start practising JavaScript
1. code editor.
I recommend installing a code editor on your computer, although you can use online code editors, too.
Here are a few free code editors you may want to try out:
- Sublime Text
For more options, check out my article with the best text editors for coding and web development .
2. Web browser
Since JavaScript runs directly in your web browser, you can simply write your code and instantly test it in your browser.
Make sure you use a modern web browser such as Google Chrome or Mozilla Firefox. You need good developer tools so that you can check the console for any errors in your code and debug them.
3. JavaScript tutorials and courses
If you are new to JavaScript, I recommend trying a few different resources to learn how the language works.
We all have different learning styles, and it’s important that you find out how you learn best.
If video tutorials are easier to follow than going through a physical book, be mindful of that and use materials that support your learning.
I’ve listed some of my recommended JavaScript courses here .
For even more resources, check out these top web development courses , too.
4. JavaScript syntax cheatsheet
Wrapping your head around the syntax can be tricky in the beginning. Using a cheatsheet is a quick way to save time when you need a quick refresher or want to double-check something.
I love this free JavaScript cheatsheet on Codecademy. For a compact PDF version, check out this free JS cheatsheet .
5. Portfolio for your JavaScript projects
As you work through your JavaScript practices and your skills improve, you will start building more complex JavaScript projects.
To showcase your skills and best work samples to potential employers, you want to set up a professional-looking portfolio website. It doesn’t have to be anything too fancy – a simple one-page website is more than enough to get started.
Keep your design clean and clear, and don’t be afraid to show some personality!
To help you get started, I’ve written a free step-by-step guide for creating a portfolio website from scratch .
Summing it up: Where to find the best JavaScript exercises and practice projects
This article showed you a handful of great JavaScript practice sites for improving your coding skills at your own pace.
I hope you found a few helpful resources for beginner-friendly and fun JavaScript exercises and coding challenges!
So the question is: what are you going to build first? Please share your thoughts in the comments below!
If you need some help with your JavaScript practice projects, check out these top JavaScript and jQuery courses and tutorials .
For my proven learning strategy, check out the best way to learn JavaScript from scratch .
Make sure to bookmark this article and share it with others, too. Thank you for your support!
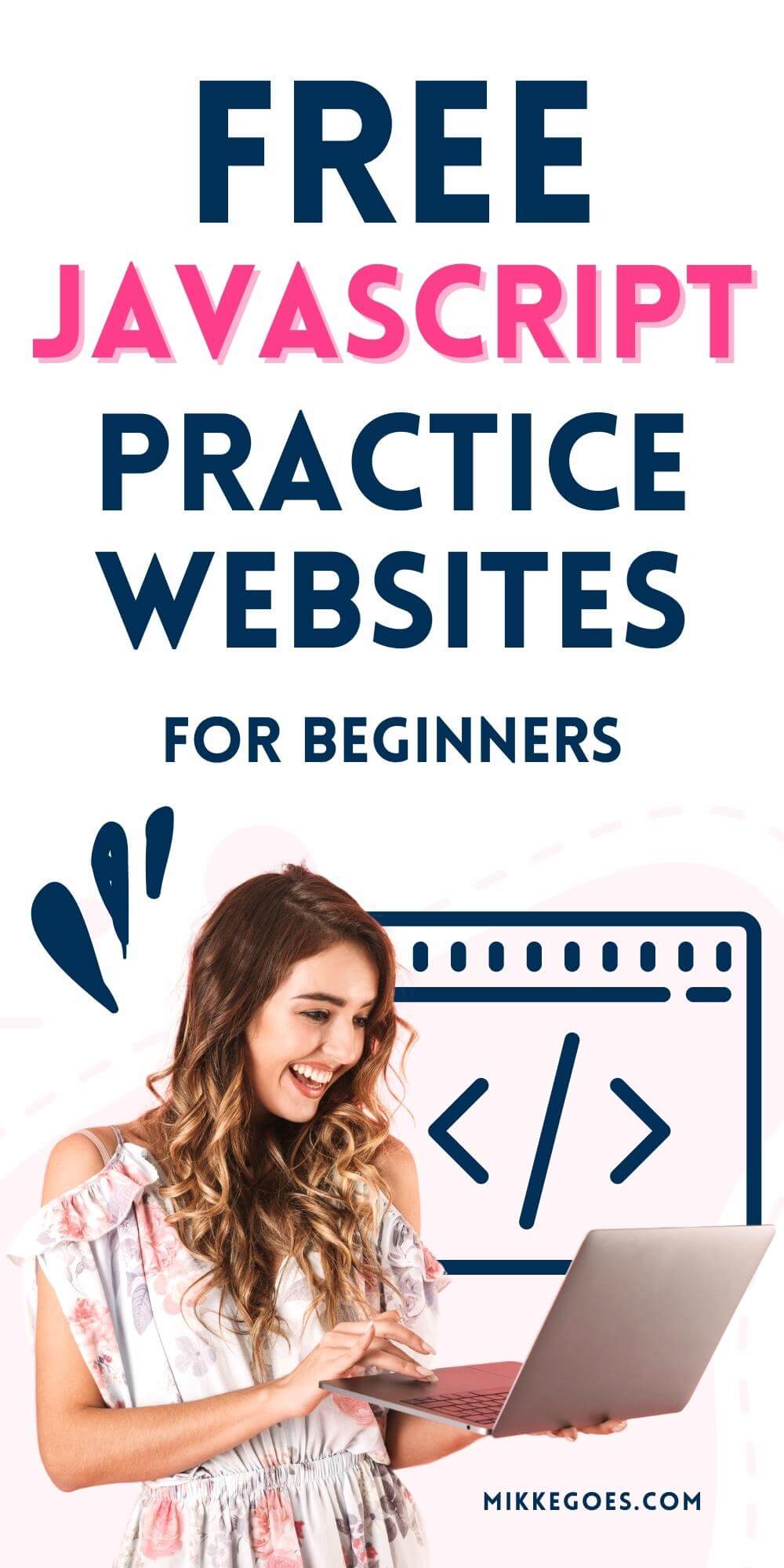
Share this post with others:
About mikke.
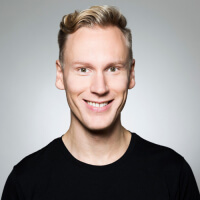
Hi, I’m Mikke! I’m a blogger, freelance web developer, and online business nerd. Join me here on MikkeGoes.com to learn how to code for free , build a professional portfolio website , launch a tech side hustle , and make money coding . When I’m not blogging, you will find me sipping strong coffee and biking around town in Berlin. Learn how I taught myself tech skills and became a web dev entrepreneur here . And come say hi on Twitter !
Leave a reply:
Download 15 tips for learning how to code:.
GET THE TIPS NOW
Sign up now to get my free guide to teach yourself how to code from scratch. If you are interested in learning tech skills, these tips are perfect for getting started faster.
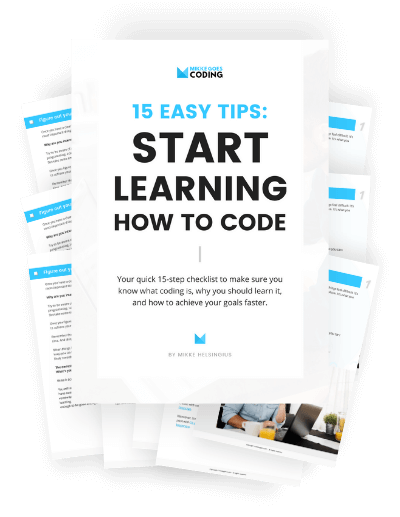

25+ JavaScript Coding Interview Questions (SOLVED with CODE)
Having a JavaScript Coding Interview Session on this week? Fear not, we got your covered! Check that ultimate list of 25 advanced and tricky JavaScript Coding Interview Questions and Challenges to crack on your next senior web developer interview and got your next six-figure job offer in no time!
Q1 : Explain what a callback function is and provide a simple example
A callback function is a function that is passed to another function as an argument and is executed after some operation has been completed. Below is an example of a simple callback function that logs to the console after some operations have been completed.
Q2 : Given a string, reverse each word in the sentence
For example Welcome to this Javascript Guide! should be become emocleW ot siht tpircsavaJ !ediuG
Q3 : How to check if an object is an array or not? Provide some code.
The best way to find whether an object is instance of a particular class or not using toString method from Object.prototype
One of the best use cases of type checking of an object is when we do method overloading in JavaScript. For understanding this let say we have a method called greet which take one single string and also a list of string, so making our greet method workable in both situation we need to know what kind of parameter is being passed, is it single value or list of value?
However, in above implementation it might not necessary to check type for array, we can check for single value string and put array logic code in else block, let see below code for the same.
Now it's fine we can go with above two implementations, but when we have a situation like a parameter can be single value , array , and object type then we will be in trouble.
Coming back to checking type of object, As we mentioned that we can use Object.prototype.toString
If you are using jQuery then you can also used jQuery isArray method:
FYI jQuery uses Object.prototype.toString.call internally to check whether an object is an array or not.
In modern browser, you can also use:
Array.isArray is supported by Chrome 5, Firefox 4.0, IE 9, Opera 10.5 and Safari 5
Q4 : How to empty an array in JavaScript?
How could we empty the array above?
Above code will set the variable arrayList to a new empty array. This is recommended if you don't have references to the original array arrayList anywhere else because It will actually create a new empty array. You should be careful with this way of empty the array, because if you have referenced this array from another variable, then the original reference array will remain unchanged, Only use this way if you have only referenced the array by its original variable arrayList .
For Instance:
Above code will clear the existing array by setting its length to 0. This way of empty the array also update all the reference variable which pointing to the original array. This way of empty the array is useful when you want to update all the another reference variable which pointing to arrayList .
Above implementation will also work perfectly. This way of empty the array will also update all the references of the original array.
Above implementation can also empty the array. But not recommended to use often.
Q5 : How would you check if a number is an integer?
A very simply way to check if a number is a decimal or integer is to see if there is a remainder left when you divide by 1.
Q6 : Implement enqueue and dequeue using only two stacks
Enqueue means to add an element, dequeue to remove an element.
Q7 : Make this work
Q8 : write a "mul" function which will properly when invoked as below syntax.
Here mul function accept the first argument and return anonymous function which take the second parameter and return anonymous function which take the third parameter and return multiplication of arguments which is being passed in successive
In JavaScript function defined inside has access to outer function variable and function is the first class object so it can be returned by function as well and passed as argument in another function.
- A function is an instance of the Object type
- A function can have properties and has a link back to its constructor method
- Function can be stored as variable
- Function can be pass as a parameter to another function
- Function can be returned from function
Q9 : Write a function that would allow you to do this?
You can create a closure to keep the value passed to the function createBase even after the inner function is returned. The inner function that is being returned is created within an outer function, making it a closure, and it has access to the variables within the outer function, in this case the variable baseNumber .
Q10 : FizzBuzz Challenge
Create a for loop that iterates up to 100 while outputting "fizz" at multiples of 3 , "buzz" at multiples of 5 and "fizzbuzz" at multiples of 3 and 5 .
Check out this version of FizzBuzz:
Q11 : Given two strings, return true if they are anagrams of one another
For example: Mary is an anagram of Army
Q12 : How would you use a closure to create a private counter?
You can create a function within an outer function (a closure) that allows you to update a private variable but the variable wouldn't be accessible from outside the function without the use of a helper function.
Q13 : Provide some examples of non-bulean value coercion to a boolean one
The question is when a non-boolean value is coerced to a boolean, does it become true or false , respectively?
The specific list of "falsy" values in JavaScript is as follows:
- "" (empty string)
- 0 , -0 , NaN (invalid number)
- null , undefined
Any value that's not on this "falsy" list is "truthy." Here are some examples of those:
- [ ] , [ 1, "2", 3 ] (arrays)
- { } , { a: 42 } (objects)
- function foo() { .. } (functions)
Q14 : What will be the output of the following code?
Above code would give output 1undefined . If condition statement evaluate using eval so eval(function f() {}) which return function f() {} which is true so inside if statement code execute. typeof f return undefined because if statement code execute at run time, so statement inside if condition evaluated at run time.
Above code will also output 1undefined .
Q15 : What will the following code output?
The code above will output 5 even though it seems as if the variable was declared within a function and can't be accessed outside of it. This is because
is interpreted the following way:
But b is not declared anywhere in the function with var so it is set equal to 5 in the global scope .
Q16 : Write a function that would allow you to do this
You can create a closure to keep the value of a even after the inner function is returned. The inner function that is being returned is created within an outer function, making it a closure, and it has access to the variables within the outer function, in this case the variable a .
Q17 : How does the this keyword work? Provide some code examples
In JavaScript this always refers to the “owner” of the function we're executing, or rather, to the object that a function is a method of.
Q18 : How would you create a private variable in JavaScript?
To create a private variable in JavaScript that cannot be changed you need to create it as a local variable within a function. Even if the function is executed the variable cannot be accessed outside of the function. For example:
To access the variable, a helper function would need to be created that returns the private variable.
Q19 : What is Closure in JavaScript? Provide an example
A closure is a function defined inside another function (called parent function) and has access to the variable which is declared and defined in parent function scope.
The closure has access to variable in three scopes:
- Variable declared in his own scope
- Variable declared in parent function scope
- Variable declared in global namespace
innerFunction is closure which is defined inside outerFunction and has access to all variable which is declared and defined in outerFunction scope. In addition to this function defined inside function as closure has access to variable which is declared in global namespace .
Output of above code would be:
Q20 : What will be the output of the following code?
Above code will output 0 as output. delete operator is used to delete a property from an object. Here x is not an object it's local variable . delete operator doesn't affect local variable.
Q21 : What will be the output of the following code?
Above code will output xyz as output. Here emp1 object got company as prototype property. delete operator doesn't delete prototype property.
emp1 object doesn't have company as its own property. You can test it like:
However, we can delete company property directly from Employee object using delete Employee.company or we can also delete from emp1 object using __proto__ property delete emp1.__proto__.company .
Q22 : What will the following code output?
This will surprisingly output false because of floating point errors in internally representing certain numbers. 0.1 + 0.2 does not nicely come out to 0.3 but instead the result is actually 0.30000000000000004 because the computer cannot internally represent the correct number. One solution to get around this problem is to round the results when doing arithmetic with decimal numbers.
Q23 : When would you use the bind function?
The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
A good use of the bind function is when you have a particular function that you want to call with a specific this value. You can then use bind to pass a specific object to a function that uses a this reference.
Q24 : Write a recursive function that performs a binary search
Q25 : describe the revealing module pattern design pattern.
A variation of the module pattern is called the Revealing Module Pattern . The purpose is to maintain encapsulation and reveal certain variables and methods returned in an object literal. The direct implementation looks like this:
An obvious disadvantage of it is unable to reference the private methods
Rust has been Stack Overflow’s most loved language for four years in a row and emerged as a compelling language choice for both backend and system developers, offering a unique combination of memory safety, performance, concurrency without Data races...
Clean Architecture provides a clear and modular structure for building software systems, separating business rules from implementation details. It promotes maintainability by allowing for easier updates and changes to specific components without affe...
Azure Service Bus is a crucial component for Azure cloud developers as it provides reliable and scalable messaging capabilities. It enables decoupled communication between different components of a distributed system, promoting flexibility and resili...
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time.
Coded with 🧡 using React in Australia 🇦🇺
by @aershov24 , Full Stack Cafe Pty Ltd 🤙, 2018-2023
Privacy • Terms of Service • Guest Posts • Contacts • MLStack.Cafe

Download Interview guide PDF
Javascript interview questions, download pdf.
JavaScript , created by Brendan Eich in 1995, is one of the most widely used web development languages. It was designed to build dynamic web pages at first. A script is a JS program that may be added to the HTML of any web page. When the page loads, these scripts execute automatically.
A language that was originally designed to build dynamic web pages may now be run on the server and on almost any device that has the JavaScript Engine installed.
After HTML and CSS, JavaScript is the third biggest web technology. JavaScript is a scripting language that may be used to construct online and mobile apps, web servers, games, and more. JavaScript is an object-oriented programming language that is used to generate websites and applications. It was created with the intention of being used in a browser. Even today, the server-side version of JavaScript known as Node.js may be used to create online and mobile apps, real-time applications, online streaming applications, and videogames. Javascript frameworks , often known as inbuilt libraries, may be used to construct desktop and mobile programs. Developers may save a lot of time on monotonous programming jobs by using these code libraries, allowing them to focus on the production work of development.
The InterviewBit team has compiled a thorough collection of top Javascript Interview Questions and Answers to assist you in acing your interview and landing your desired job as a Javascript Developer.
JavaScript Interview Questions for Freshers
1. what are the different data types present in javascript.
To know the type of a JavaScript variable, we can use the typeof operator.
1. Primitive types
String - It represents a series of characters and is written with quotes. A string can be represented using a single or a double quote.
- Number - It represents a number and can be written with or without decimals.
- BigInt - This data type is used to store numbers which are above the limitation of the Number data type. It can store large integers and is represented by adding “n” to an integer literal.
- Boolean - It represents a logical entity and can have only two values : true or false. Booleans are generally used for conditional testing.
- Undefined - When a variable is declared but not assigned, it has the value of undefined and it’s type is also undefined.
- Null - It represents a non-existent or a invalid value.
- Symbol - It is a new data type introduced in the ES6 version of javascript. It is used to store an anonymous and unique value.
- typeof of primitive types :
2. Non-primitive types
- Primitive data types can store only a single value. To store multiple and complex values, non-primitive data types are used.
- Object - Used to store collection of data.
Note- It is important to remember that any data type that is not a primitive data type, is of Object type in javascript.
2. Explain Hoisting in javascript.
Hoisting is the default behaviour of javascript where all the variable and function declarations are moved on top.
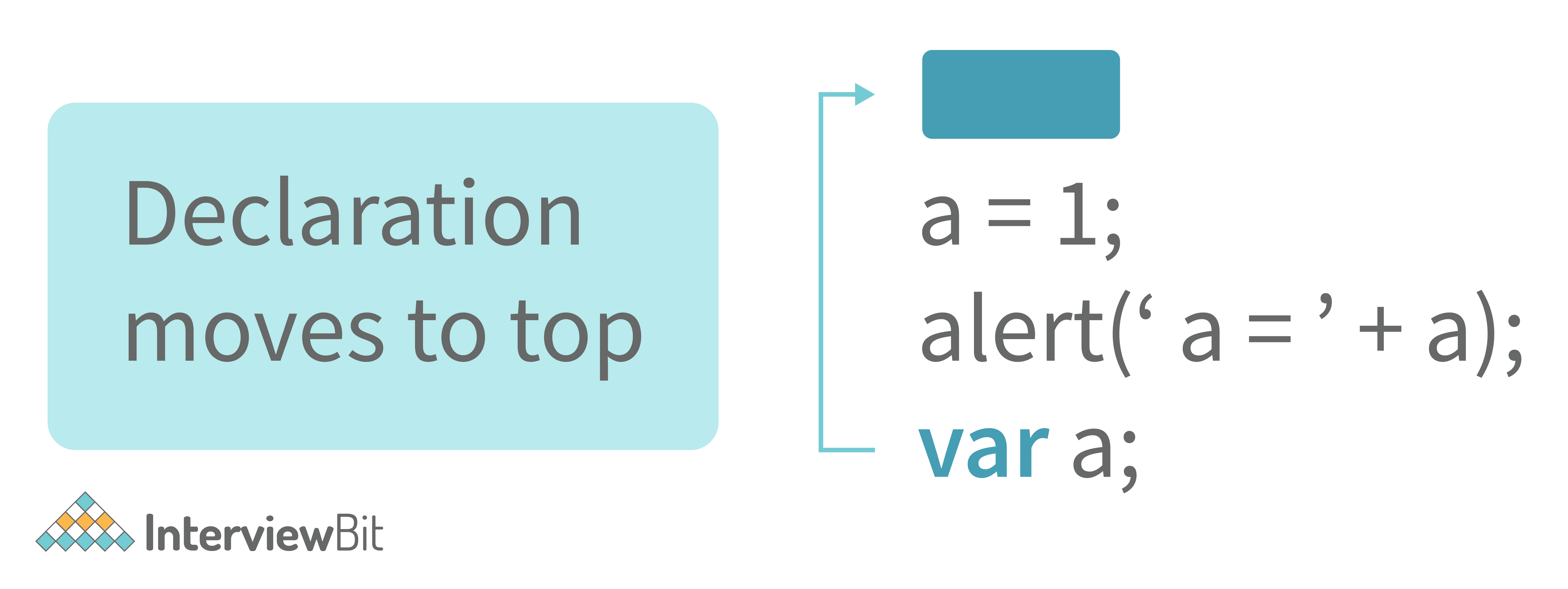
This means that irrespective of where the variables and functions are declared, they are moved on top of the scope. The scope can be both local and global. Example 1:
doSomething(); // Outputs 33 since the local variable “x” is hoisted inside the local scope
Note - Variable initializations are not hoisted, only variable declarations are hoisted:
Note - To avoid hoisting, you can run javascript in strict mode by using “use strict” on top of the code:
3. Why do we use the word “debugger” in javascript?
The debugger for the browser must be activated in order to debug the code. Built-in debuggers may be switched on and off, requiring the user to report faults. The remaining section of the code should stop execution before moving on to the next line while debugging.
4. Difference between “ == “ and “ === “ operators.
Both are comparison operators. The difference between both the operators is that “==” is used to compare values whereas, “ === “ is used to compare both values and types.
5. Difference between var and let keyword in javascript.
Some differences are
- From the very beginning, the 'var' keyword was used in JavaScript programming whereas the keyword 'let' was just added in 2015.
- The keyword 'Var' has a function scope. Anywhere in the function, the variable specified using var is accessible but in ‘let’ the scope of a variable declared with the 'let' keyword is limited to the block in which it is declared. Let's start with a Block Scope.
- In ECMAScript 2015, let and const are hoisted but not initialized. Referencing the variable in the block before the variable declaration results in a ReferenceError because the variable is in a "temporal dead zone" from the start of the block until the declaration is processed.
- Software Dev
- Data Science
6. Explain Implicit Type Coercion in javascript.
Implicit type coercion in javascript is the automatic conversion of value from one data type to another. It takes place when the operands of an expression are of different data types.
- String coercion
String coercion takes place while using the ‘ + ‘ operator. When a number is added to a string, the number type is always converted to the string type.
Note - ‘ + ‘ operator when used to add two numbers, outputs a number. The same ‘ + ‘ operator when used to add two strings, outputs the concatenated string:
Let’s understand both the examples where we have added a number to a string,
When JavaScript sees that the operands of the expression x + y are of different types ( one being a number type and the other being a string type ), it converts the number type to the string type and then performs the operation. Since after conversion, both the variables are of string type, the ‘ + ‘ operator outputs the concatenated string “33” in the first example and “24Hello” in the second example.
Note - Type coercion also takes place when using the ‘ - ‘ operator, but the difference while using ‘ - ‘ operator is that, a string is converted to a number and then subtraction takes place.
- Boolean Coercion
Boolean coercion takes place when using logical operators, ternary operators, if statements, and loop checks. To understand boolean coercion in if statements and operators, we need to understand truthy and falsy values. Truthy values are those which will be converted (coerced) to true . Falsy values are those which will be converted to false . All values except false, 0, 0n, -0, “”, null, undefined, and NaN are truthy values.
If statements:
- Logical operators:
Logical operators in javascript, unlike operators in other programming languages, do not return true or false. They always return one of the operands. OR ( | | ) operator - If the first value is truthy, then the first value is returned. Otherwise, always the second value gets returned. AND ( && ) operator - If both the values are truthy, always the second value is returned. If the first value is falsy then the first value is returned or if the second value is falsy then the second value is returned. Example:
- Equality Coercion
Equality coercion takes place when using ‘ == ‘ operator. As we have stated before The ‘ == ‘ operator compares values and not types. While the above statement is a simple way to explain == operator, it’s not completely true The reality is that while using the ‘==’ operator, coercion takes place. The ‘==’ operator, converts both the operands to the same type and then compares them. Example:
Coercion does not take place when using the ‘===’ operator. Both operands are not converted to the same type in the case of ‘===’ operator.
7. Is javascript a statically typed or a dynamically typed language?
JavaScript is a dynamically typed language. In a dynamically typed language, the type of a variable is checked during run-time in contrast to a statically typed language, where the type of a variable is checked during compile-time.
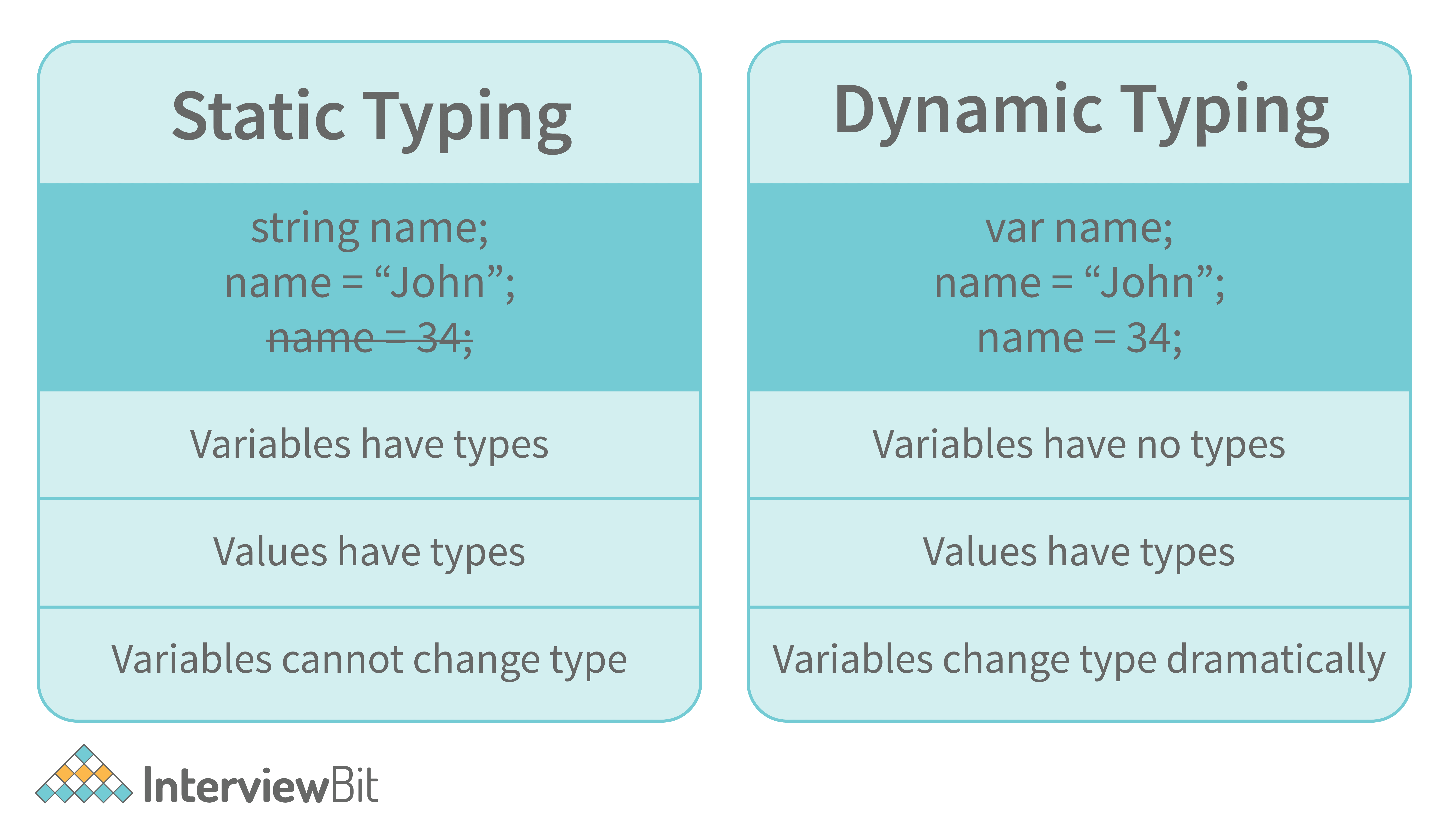
Since javascript is a loosely(dynamically) typed language, variables in JS are not associated with any type. A variable can hold the value of any data type.
For example, a variable that is assigned a number type can be converted to a string type:
8. What is NaN property in JavaScript?
NaN property represents the “Not-a-Number” value. It indicates a value that is not a legal number.
typeof of NaN will return a Number .
To check if a value is NaN, we use the isNaN() function,
Note- isNaN() function converts the given value to a Number type, and then equates to NaN.
9. Explain passed by value and passed by reference.
In JavaScript, primitive data types are passed by value and non-primitive data types are passed by reference. For understanding passed by value and passed by reference, we need to understand what happens when we create a variable and assign a value to it,
In the above example, we created a variable x and assigned it a value of “2”. In the background, the “=” (assign operator) allocates some space in the memory, stores the value “2” and returns the location of the allocated memory space. Therefore, the variable x in the above code points to the location of the memory space instead of pointing to the value 2 directly.
Assign operator behaves differently when dealing with primitive and non-primitive data types, Assign operator dealing with primitive types:
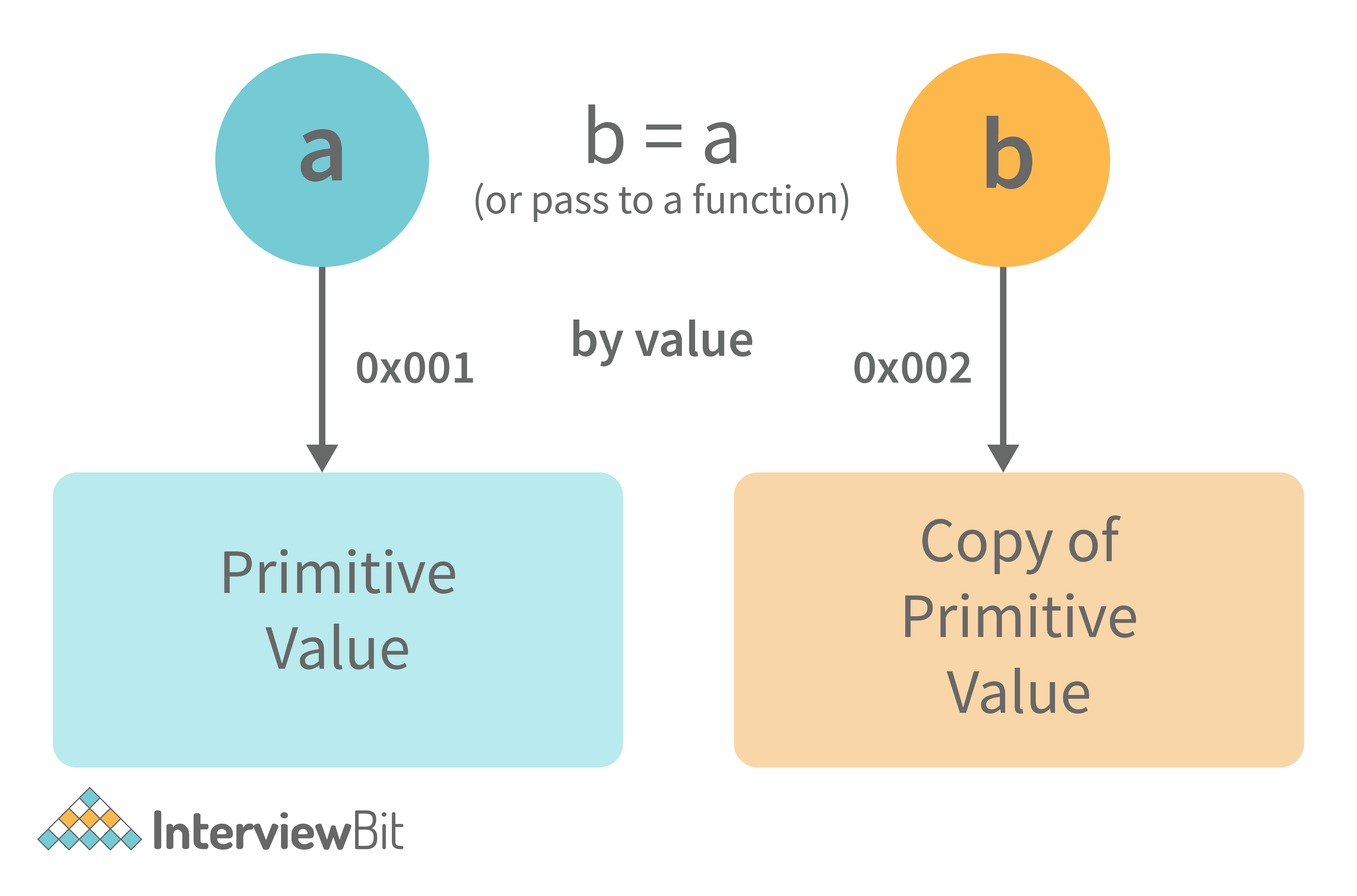
In the above example, the assign operator knows that the value assigned to y is a primitive type (number type in this case), so when the second line code executes, where the value of y is assigned to z, the assign operator takes the value of y (234) and allocates a new space in the memory and returns the address. Therefore, variable z is not pointing to the location of variable y, instead, it is pointing to a new location in the memory.
From the above example, we can see that primitive data types when passed to another variable, are passed by value. Instead of just assigning the same address to another variable, the value is passed and new space of memory is created. Assign operator dealing with non-primitive types:
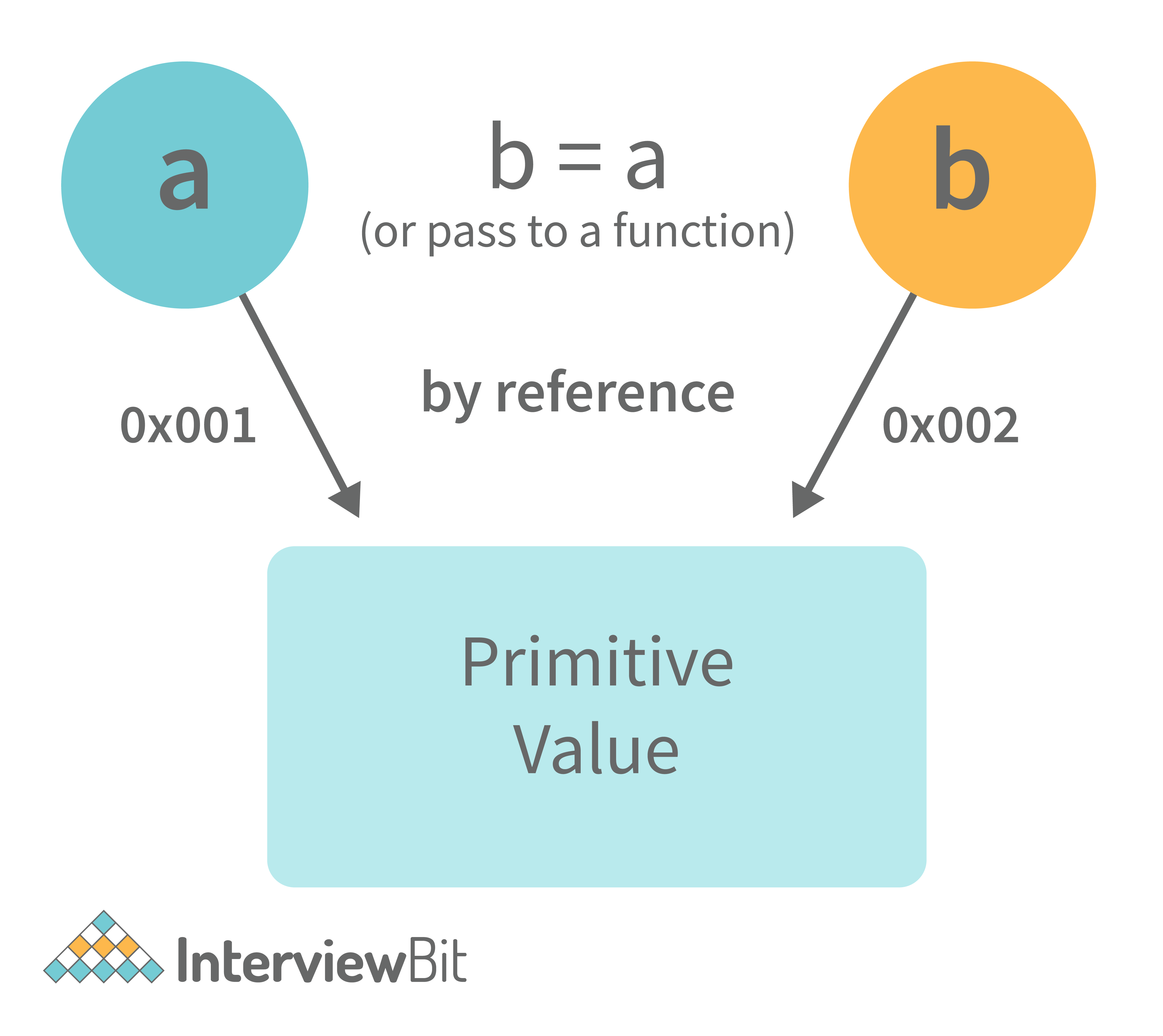
In the above example, the assign operator directly passes the location of the variable obj to the variable obj2. In other words, the reference of the variable obj is passed to the variable obj2.
From the above example, we can see that while passing non-primitive data types, the assigned operator directly passes the address (reference). Therefore, non-primitive data types are always passed by reference.
10. What is an Immediately Invoked Function in JavaScript?
An Immediately Invoked Function ( known as IIFE and pronounced as IIFY) is a function that runs as soon as it is defined.
Syntax of IIFE :
To understand IIFE, we need to understand the two sets of parentheses that are added while creating an IIFE : The first set of parenthesis:
While executing javascript code, whenever the compiler sees the word “function”, it assumes that we are declaring a function in the code. Therefore, if we do not use the first set of parentheses, the compiler throws an error because it thinks we are declaring a function, and by the syntax of declaring a function, a function should always have a name.
To remove this error, we add the first set of parenthesis that tells the compiler that the function is not a function declaration, instead, it’s a function expression. The second set of parenthesis:
From the definition of an IIFE, we know that our code should run as soon as it is defined. A function runs only when it is invoked. If we do not invoke the function, the function declaration is returned:
Therefore to invoke the function, we use the second set of parenthesis.
11. What do you mean by strict mode in javascript and characteristics of javascript strict-mode?
In ECMAScript 5, a new feature called JavaScript Strict Mode allows you to write a code or a function in a "strict" operational environment. In most cases, this language is 'not particularly severe' when it comes to throwing errors. In 'Strict mode,' however, all forms of errors, including silent errors, will be thrown. As a result, debugging becomes a lot simpler. Thus programmer's chances of making an error are lowered.
Characteristics of strict mode in javascript
- Duplicate arguments are not allowed by developers.
- In strict mode, you won't be able to use the JavaScript keyword as a parameter or function name.
- The 'use strict' keyword is used to define strict mode at the start of the script. Strict mode is supported by all browsers.
- Engineers will not be allowed to create global variables in 'Strict Mode.
12. Explain Higher Order Functions in javascript.
Functions that operate on other functions, either by taking them as arguments or by returning them, are called higher-order functions. Higher-order functions are a result of functions being first-class citizens in javascript.
Examples of higher-order functions:
13. Explain “this” keyword.
The “this” keyword refers to the object that the function is a property of. The value of the “this” keyword will always depend on the object that is invoking the function.\
Confused? Let’s understand the above statements by examples:
What do you think the output of the above code will be?
Note - Observe the line where we are invoking the function.
Check the definition again:
The “this” keyword refers to the object that the function is a property of.
In the above code, the function is a property of which object?
Since the function is invoked in the global context, the function is a property of the global object.
Therefore, the output of the above code will be the global object. Since we ran the above code inside the browser, the global object is the window object.
In the above code, at the time of invocation, the getName function is a property of the object obj , therefore, this keyword will refer to the object obj , and hence the output will be “vivek”.
Can you guess the output here?
The output will be “akshay”.
Although the getName function is declared inside the object obj , at the time of invocation, getName() is a property of obj2 , therefore the “this” keyword will refer to obj2 .
The silly way to understand the “ this” keyword is, whenever the function is invoked, check the object before the dot . The value of this . keyword will always be the object before the dot .
If there is no object before the dot-like in example1, the value of this keyword will be the global object.
Can you guess the output?
The output will be an error.
Although in the code above, this keyword refers to the object obj2 , obj2 does not have the property “address”‘, hence the getAddress function throws an error.
14. What do you mean by Self Invoking Functions?
Without being requested, a self-invoking expression is automatically invoked (initiated). If a function expression is followed by (), it will execute automatically. A function declaration cannot be invoked by itself.
Normally, we declare a function and call it, however, anonymous functions may be used to run a function automatically when it is described and will not be called again. And there is no name for these kinds of functions.
15. Explain call(), apply() and, bind() methods.
- It’s a predefined method in javascript.
- This method invokes a method (function) by specifying the owner object.
- call() method allows an object to use the method (function) of another object.
- call() accepts arguments:
apply() The apply method is similar to the call() method. The only difference is that, call() method takes arguments separately whereas, apply() method takes arguments as an array.
- This method returns a new function, where the value of “this” keyword will be bound to the owner object, which is provided as a parameter.
- Example with arguments:
16. What is the difference between exec () and test () methods in javascript?
- test () and exec () are RegExp expression methods used in javascript.
- We'll use exec () to search a string for a specific pattern, and if it finds it, it'll return the pattern directly; else, it'll return an 'empty' result.
- We will use a test () to find a string for a specific pattern. It will return the Boolean value 'true' on finding the given text otherwise, it will return 'false'.
17. What is currying in JavaScript?
Currying is an advanced technique to transform a function of arguments n, to n functions of one or fewer arguments.
Example of a curried function:
For Example, if we have a function f(a,b) , then the function after currying, will be transformed to f(a)(b). By using the currying technique, we do not change the functionality of a function, we just change the way it is invoked. Let’s see currying in action:
As one can see in the code above, we have transformed the function multiply(a,b) to a function curriedMultiply , which takes in one parameter at a time.
18. What are some advantages of using External JavaScript?
External JavaScript is the JavaScript Code (script) written in a separate file with the extension.js, and then we link that file inside the <head> or <body> element of the HTML file where the code is to be placed.
Some advantages of external javascript are
- It allows web designers and developers to collaborate on HTML and javascript files.
- We can reuse the code.
- Code readability is simple in external javascript.
19. Explain Scope and Scope Chain in javascript.
Scope in JS determines the accessibility of variables and functions at various parts of one’s code. In general terms, the scope will let us know at a given part of code, what are variables and functions we can or cannot access. There are three types of scopes in JS:
- Global Scope
- Local or Function Scope
- Block Scope
Global Scope: Variables or functions declared in the global namespace have global scope, which means all the variables and functions having global scope can be accessed from anywhere inside the code.
Function Scope: Any variables or functions declared inside a function have local/function scope, which means that all the variables and functions declared inside a function, can be accessed from within the function and not outside of it.
Block Scope: Block scope is related to the variables declared using let and const. Variables declared with var do not have block scope. Block scope tells us that any variable declared inside a block { }, can be accessed only inside that block and cannot be accessed outside of it.
Scope Chain: JavaScript engine also uses Scope to find variables. Let’s understand that using an example:
As you can see in the code above, if the javascript engine does not find the variable in local scope, it tries to check for the variable in the outer scope. If the variable does not exist in the outer scope, it tries to find the variable in the global scope.
If the variable is not found in the global space as well, a reference error is thrown.
20. Explain Closures in JavaScript.
Closures are an ability of a function to remember the variables and functions that are declared in its outer scope.
Let’s understand closures by example:
Let’s understand the code above, The function randomFunc() gets executed and returns a function when we assign it to a variable:
The returned function is then executed when we invoke initialiseClosure:
The line of code above outputs “Vivian is awesome” and this is possible because of closure.
When the function randomFunc() runs, it seems that the returning function is using the variable obj1 inside it:
Therefore randomFunc(), instead of destroying the value of obj1 after execution, saves the value in the memory for further reference. This is the reason why the returning function is able to use the variable declared in the outer scope even after the function is already executed. This ability of a function to store a variable for further reference even after it is executed is called Closure.
21. Mention some advantages of javascript.
There are many advantages of javascript. Some of them are
- Javascript is executed on the client-side as well as server-side also. There are a variety of Frontend Frameworks that you may study and utilize. However, if you want to use JavaScript on the backend, you'll need to learn NodeJS. It is currently the only JavaScript framework that may be used on the backend.
- Javascript is a simple language to learn.
- Web pages now have more functionality because of Javascript.
- To the end-user, Javascript is quite quick.
22. What are object prototypes?
All javascript objects inherit properties from a prototype. For example,
- Date objects inherit properties from the Date prototype
- Math objects inherit properties from the Math prototype
- Array objects inherit properties from the Array prototype.
- On top of the chain is Object.prototype. Every prototype inherits properties and methods from the Object.prototype.
- A prototype is a blueprint of an object. The prototype allows us to use properties and methods on an object even if the properties and methods do not exist on the current object.
Let’s see prototypes help us use methods and properties:
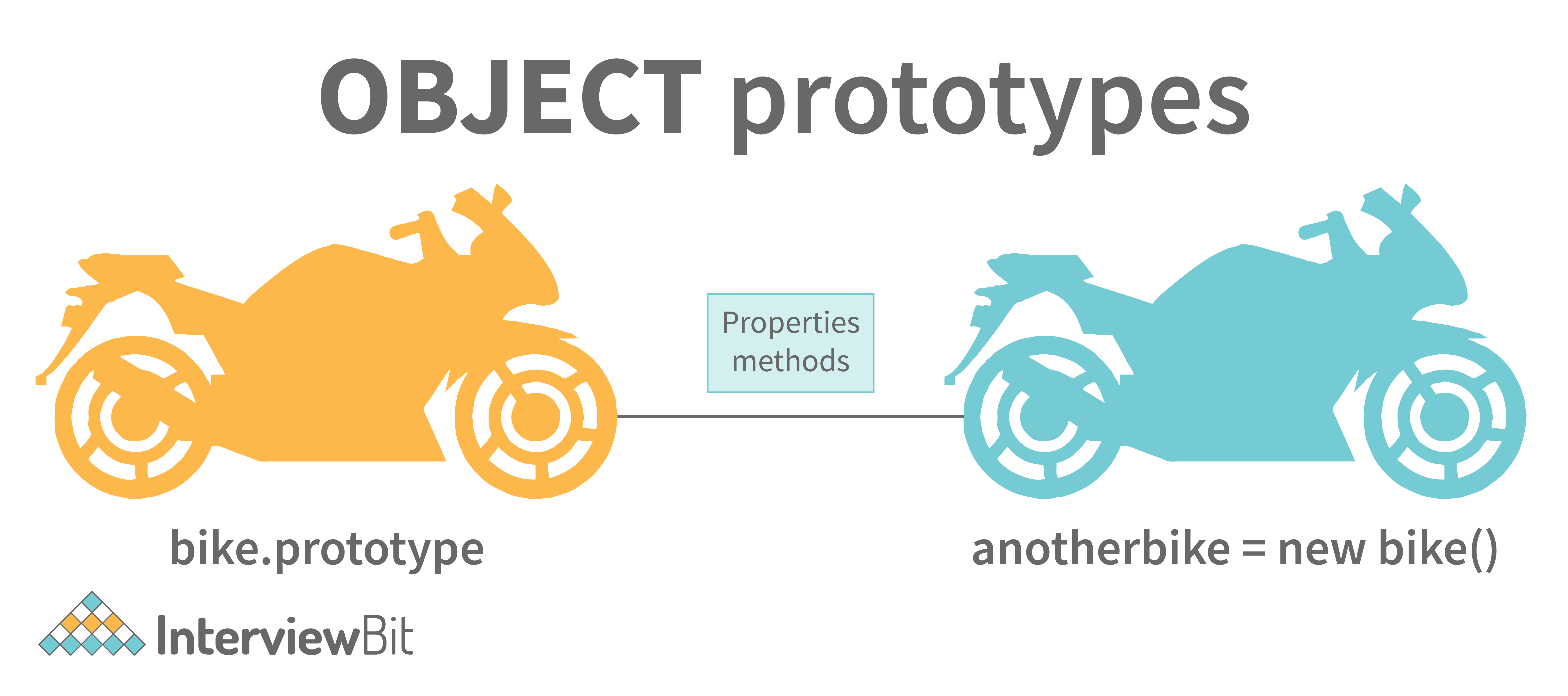
In the code above, as one can see, we have not defined any property or method called push on the array “arr” but the javascript engine does not throw an error.
The reason is the use of prototypes. As we discussed before, Array objects inherit properties from the Array prototype.
The javascript engine sees that the method push does not exist on the current array object and therefore, looks for the method push inside the Array prototype and it finds the method.
Whenever the property or method is not found on the current object, the javascript engine will always try to look in its prototype and if it still does not exist, it looks inside the prototype's prototype and so on.
23. What are callbacks?
A callback is a function that will be executed after another function gets executed. In javascript, functions are treated as first-class citizens, they can be used as an argument of another function, can be returned by another function, and can be used as a property of an object.
Functions that are used as an argument to another function are called callback functions. Example:
- In the code above, we are performing mathematical operations on the sum of two numbers. The operationOnSum function takes 3 arguments, the first number, the second number, and the operation that is to be performed on their sum (callback).
- Both divideByHalf and multiplyBy2 functions are used as callback functions in the code above.
- These callback functions will be executed only after the function operationOnSum is executed.
- Therefore, a callback is a function that will be executed after another function gets executed.
24. What are the types of errors in javascript?
There are two types of errors in javascript.
- Syntax error : Syntax errors are mistakes or spelling problems in the code that cause the program to not execute at all or to stop running halfway through. Error messages are usually supplied as well.
- Logical error : Reasoning mistakes occur when the syntax is proper but the logic or program is incorrect. The application executes without problems in this case. However, the output findings are inaccurate. These are sometimes more difficult to correct than syntax issues since these applications do not display error signals for logic faults.
25. What is memoization?
Memoization is a form of caching where the return value of a function is cached based on its parameters. If the parameter of that function is not changed, the cached version of the function is returned. Let’s understand memoization, by converting a simple function to a memoized function:
Note- Memoization is used for expensive function calls but in the following example, we are considering a simple function for understanding the concept of memoization better.
Consider the following function:
In the code above, we have written a function that adds the parameter to 256 and returns it. When we are calling the function addTo256 again with the same parameter (“20” in the case above), we are computing the result again for the same parameter. Computing the result with the same parameter, again and again, is not a big deal in the above case, but imagine if the function does some heavy-duty work, then, computing the result again and again with the same parameter will lead to wastage of time.
This is where memoization comes in, by using memoization we can store(cache) the computed results based on the parameters. If the same parameter is used again while invoking the function, instead of computing the result, we directly return the stored (cached) value.
Let’s convert the above function addTo256, to a memoized function:
In the code above, if we run the memoizedFunc function with the same parameter, instead of computing the result again, it returns the cached result.
Note- Although using memoization saves time, it results in larger consumption of memory since we are storing all the computed results.
26. What is recursion in a programming language?
Recursion is a technique to iterate over an operation by having a function call itself repeatedly until it arrives at a result.
Example of a recursive function: The following function calculates the sum of all the elements in an array by using recursion:
27. What is the use of a constructor function in javascript?
Constructor functions are used to create objects in javascript.
When do we use constructor functions?
If we want to create multiple objects having similar properties and methods, constructor functions are used.
Note- The name of a constructor function should always be written in Pascal Notation: every word should start with a capital letter.
In the code above, we have created a constructor function named Person. Whenever we want to create a new object of the type Person, We need to create it using the new keyword:
The above line of code will create a new object of the type Person. Constructor functions allow us to group similar objects.
28. What is DOM?
- DOM stands for Document Object Model. DOM is a programming interface for HTML and XML documents.
- When the browser tries to render an HTML document, it creates an object based on the HTML document called DOM. Using this DOM, we can manipulate or change various elements inside the HTML document.
- Example of how HTML code gets converted to DOM:
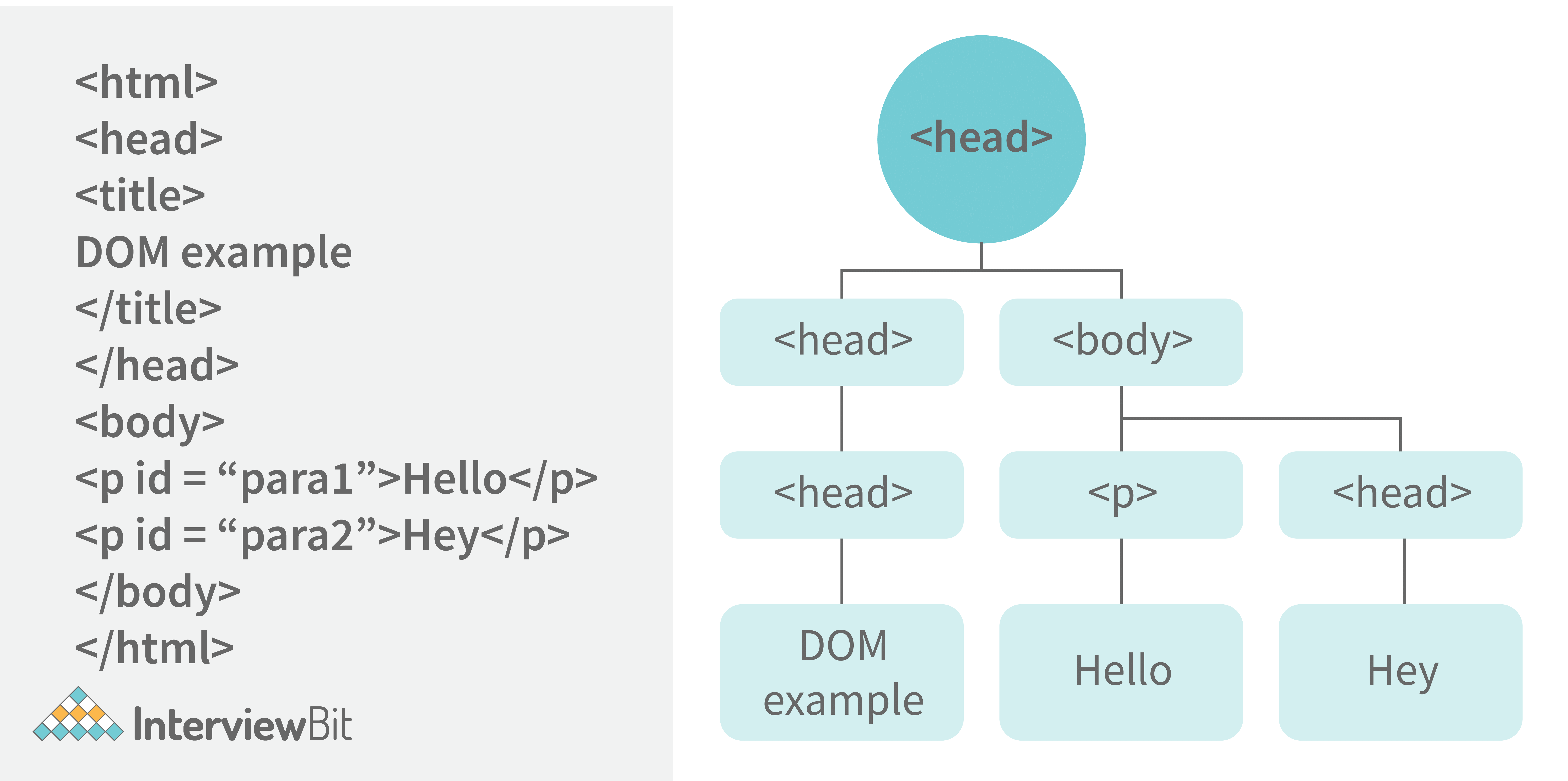
29. Which method is used to retrieve a character from a certain index?
The charAt() function of the JavaScript string finds a char element at the supplied index. The index number begins at 0 and continues up to n-1, Here n is the string length. The index value must be positive, higher than, or the same as the string length.
30. What do you mean by BOM?
Browser Object Model is known as BOM. It allows users to interact with the browser. A browser's initial object is a window. As a result, you may call all of the window's functions directly or by referencing the window. The document, history, screen, navigator, location, and other attributes are available in the window object.
31. What is the distinction between client-side and server-side JavaScript?
Client-side JavaScript is made up of two parts, a fundamental language and predefined objects for performing JavaScript in a browser. JavaScript for the client is automatically included in the HTML pages. At runtime, the browser understands this script.
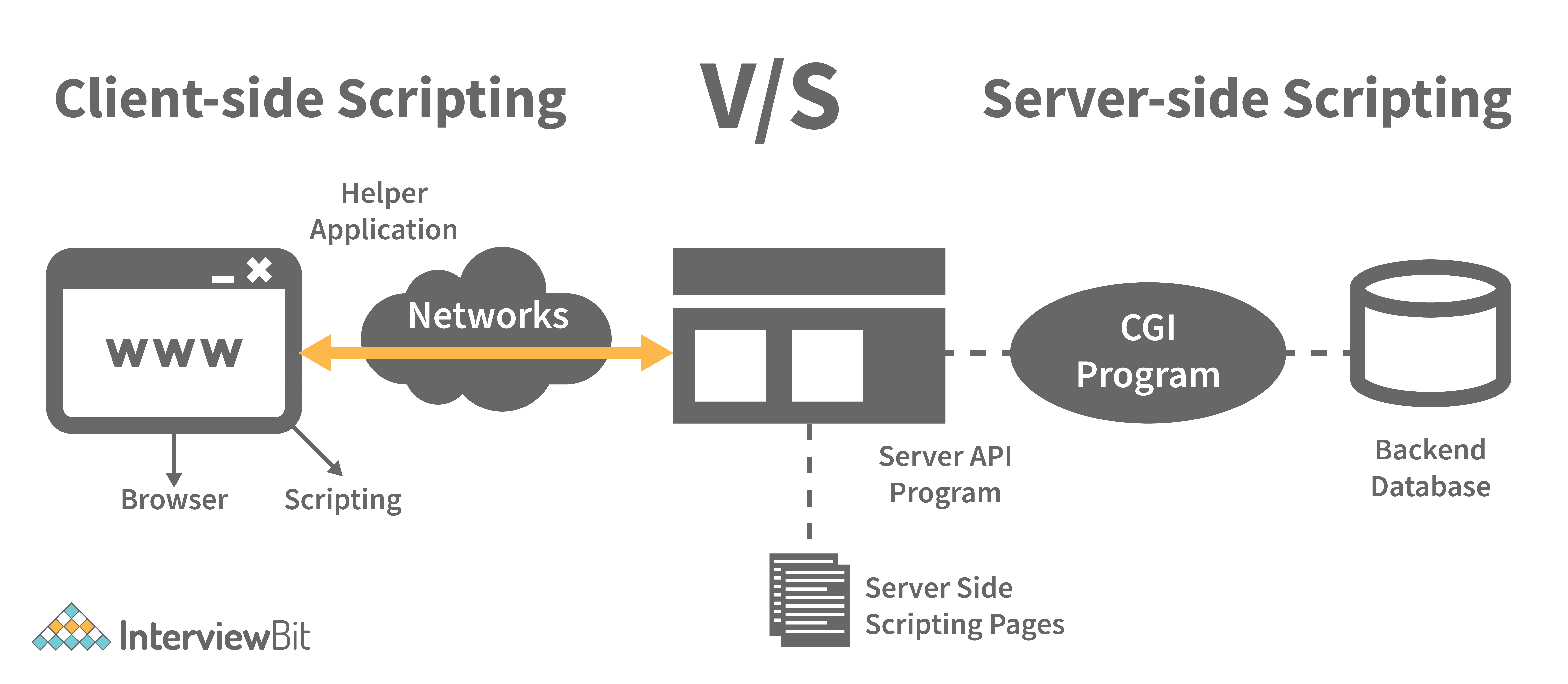
Server-side JavaScript, involves the execution of JavaScript code on a server in response to client requests. It handles these requests and delivers the relevant response to the client, which may include client-side JavaScript for subsequent execution within the browser.
JavaScript Interview Questions for Experienced
1. what are arrow functions.
Arrow functions were introduced in the ES6 version of javascript. They provide us with a new and shorter syntax for declaring functions. Arrow functions can only be used as a function expression. Let’s compare the normal function declaration and the arrow function declaration in detail:
Arrow functions are declared without the function keyword. If there is only one returning expression then we don’t need to use the return keyword as well in an arrow function as shown in the example above. Also, for functions having just one line of code, curly braces { } can be omitted.
If the function takes in only one argument, then the parenthesis () around the parameter can be omitted as shown in the code above.
The biggest difference between the traditional function expression and the arrow function is the handling of this keyword. By general definition, this keyword always refers to the object that is calling the function. As you can see in the code above, obj1.valueOfThis() returns obj1 since this keyword refers to the object calling the function.
In the arrow functions, there is no binding of this keyword. This keyword inside an arrow function does not refer to the object calling it. It rather inherits its value from the parent scope which is the window object in this case. Therefore, in the code above, obj2.valueOfThis() returns the window object.
2. What do mean by prototype design pattern?
The Prototype Pattern produces different objects, but instead of returning uninitialized objects, it produces objects that have values replicated from a template – or sample – object. Also known as the Properties pattern, the Prototype pattern is used to create prototypes.
The introduction of business objects with parameters that match the database's default settings is a good example of where the Prototype pattern comes in handy. The default settings for a newly generated business object are stored in the prototype object.
The Prototype pattern is hardly used in traditional languages, however, it is used in the development of new objects and templates in JavaScript, which is a prototypal language.
3. Differences between declaring variables using var, let and const.
Before the ES6 version of javascript, only the keyword var was used to declare variables. With the ES6 Version, keywords let and const were introduced to declare variables.
Let’s understand the differences with examples:
- The variables declared with the let keyword in the global scope behave just like the variable declared with the var keyword in the global scope.
- Variables declared in the global scope with var and let keywords can be accessed from anywhere in the code.
- But, there is one difference! Variables that are declared with the var keyword in the global scope are added to the window/global object. Therefore, they can be accessed using window.variableName. Whereas, the variables declared with the let keyword are not added to the global object, therefore, trying to access such variables using window.variableName results in an error.
var vs let in functional scope
Variables are declared in a functional/local scope using var and let keywords behave exactly the same, meaning, they cannot be accessed from outside of the scope.
- In javascript, a block means the code written inside the curly braces {} .
- Variables declared with var keyword do not have block scope. It means a variable declared in block scope {} with the var keyword is the same as declaring the variable in the global scope.
- Variables declared with let keyword inside the block scope cannot be accessed from outside of the block.
Const keyword
- Variables with the const keyword behave exactly like a variable declared with the let keyword with only one difference, any variable declared with the const keyword cannot be reassigned.
In the code above, although we can change the value of a property inside the variable declared with const keyword, we cannot completely reassign the variable itself.
4. What is the rest parameter and spread operator?
Both rest parameter and spread operator were introduced in the ES6 version of javascript. Rest parameter ( … ):
- It provides an improved way of handling the parameters of a function.
- Using the rest parameter syntax, we can create functions that can take a variable number of arguments.
- Any number of arguments will be converted into an array using the rest parameter.
- It also helps in extracting all or some parts of the arguments.
- Rest parameters can be used by applying three dots (...) before the parameters.
**Note- Rest parameter should always be used at the last parameter of a function:
- Spread operator (…): Although the syntax of the spread operator is exactly the same as the rest parameter, the spread operator is used to spreading an array, and object literals. We also use spread operators where one or more arguments are expected in a function call.
***Note- Key differences between rest parameter and spread operator: Rest parameter is used to take a variable number of arguments and turns them into an array while the spread operator takes an array or an object and spreads it Rest parameter is used in function declaration whereas the spread operator is used in function calls.
5. In JavaScript, how many different methods can you make an object?
In JavaScript, there are several ways to declare or construct an object.
- using Class.
- create Method.
- Object Literals.
- using Function.
- Object Constructor.
6. What is the use of promises in javascript?
Promises are used to handle asynchronous operations in javascript. Before promises, callbacks were used to handle asynchronous operations. But due to the limited functionality of callbacks, using multiple callbacks to handle asynchronous code can lead to unmanageable code. Promise object has four states -
- Pending - Initial state of promise. This state represents that the promise has neither been fulfilled nor been rejected, it is in the pending state.
- Fulfilled - This state represents that the promise has been fulfilled, meaning the async operation is completed.
- Rejected - This state represents that the promise has been rejected for some reason, meaning the async operation has failed.
- Settled - This state represents that the promise has been either rejected or fulfilled.
A promise is created using the Promise constructor which takes in a callback function with two parameters, resolve and reject respectively.
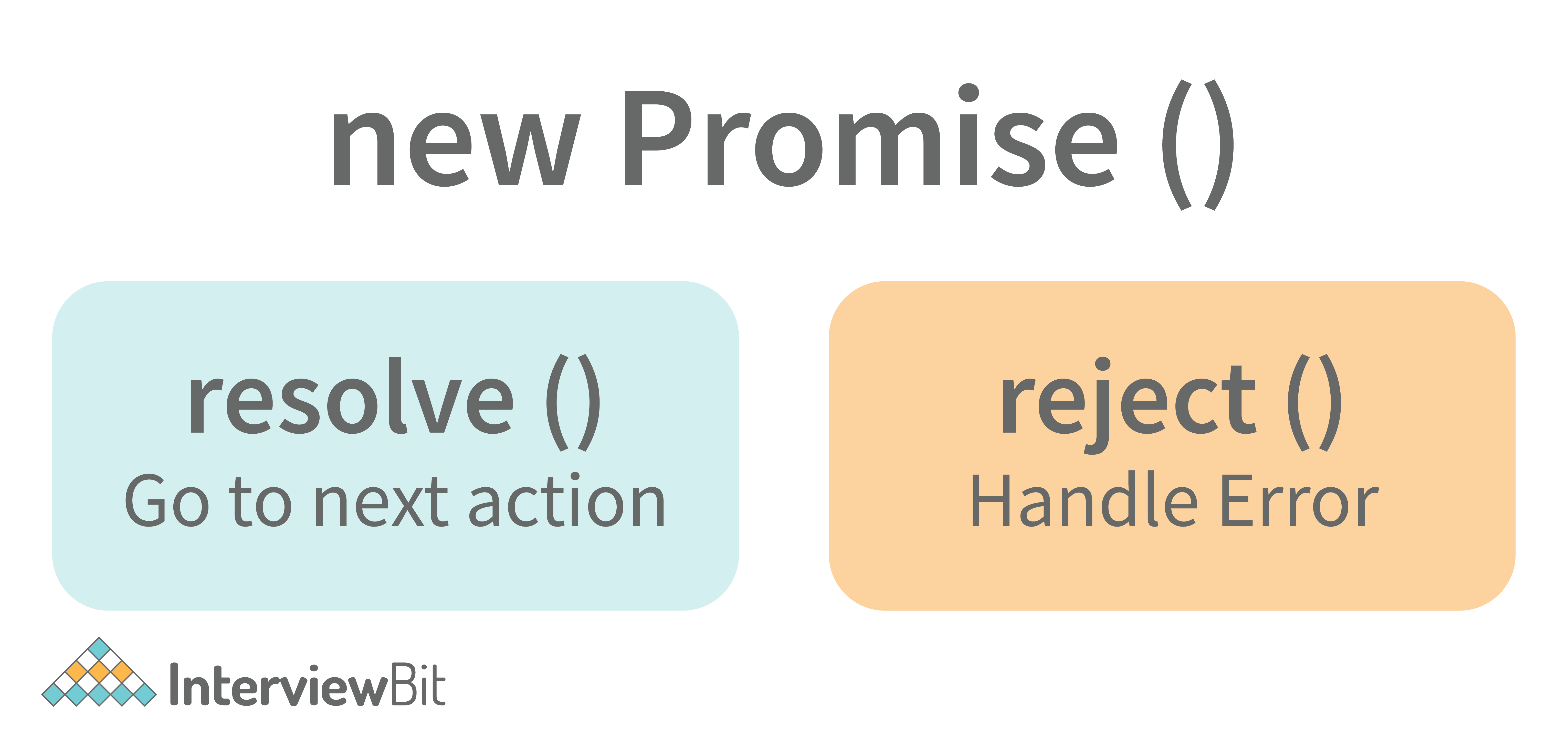
resolve is a function that will be called when the async operation has been successfully completed. reject is a function that will be called, when the async operation fails or if some error occurs. Example of a promise: Promises are used to handle asynchronous operations like server requests, for ease of understanding, we are using an operation to calculate the sum of three elements. In the function below, we are returning a promise inside a function:
In the code above, we are calculating the sum of three elements, if the length of the elements array is more than 3, a promise is rejected, or else the promise is resolved and the sum is returned.
We can consume any promise by attaching then() and catch() methods to the consumer.
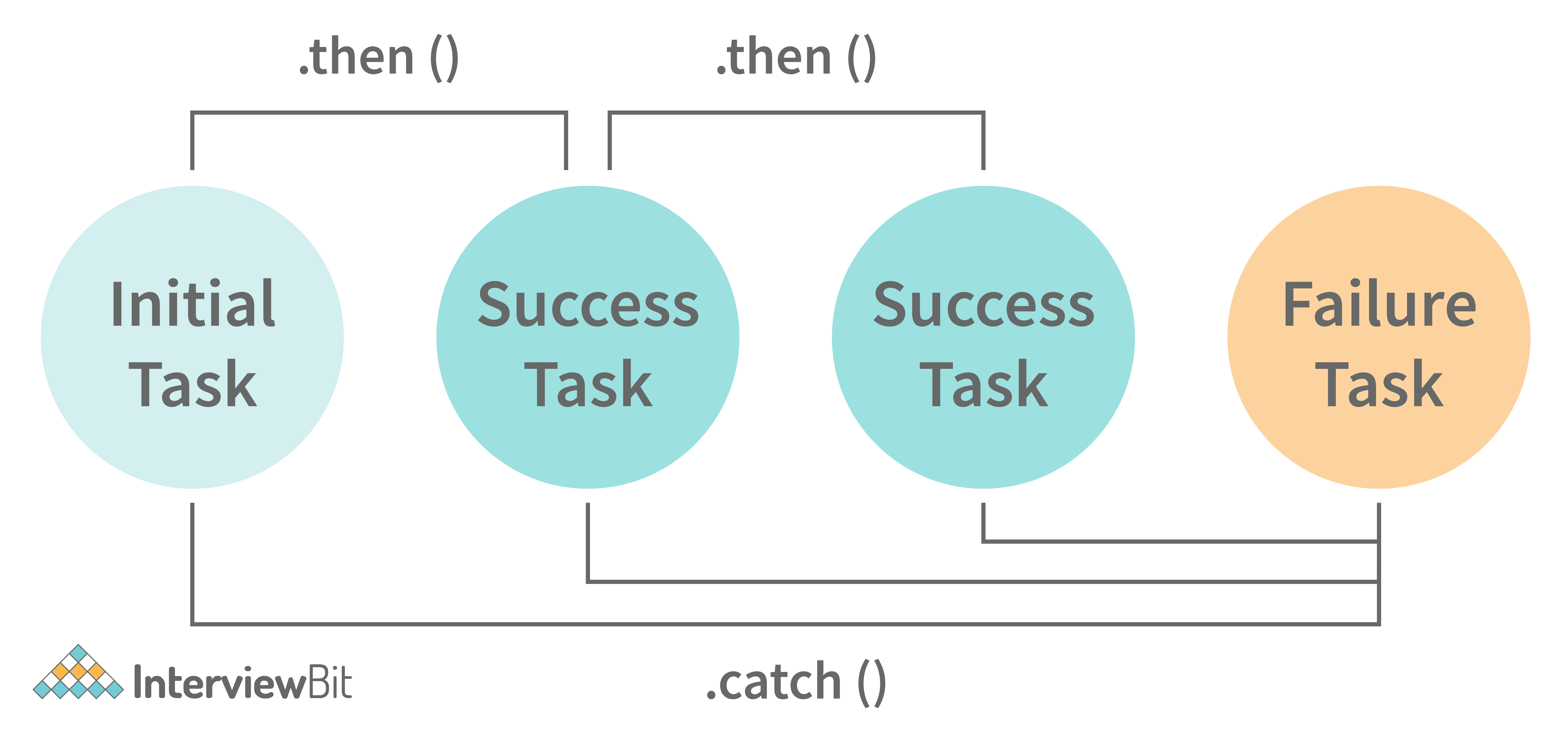
then() method is used to access the result when the promise is fulfilled.
catch() method is used to access the result/error when the promise is rejected. In the code below, we are consuming the promise:
7. What are classes in javascript?
Introduced in the ES6 version, classes are nothing but syntactic sugars for constructor functions. They provide a new way of declaring constructor functions in javascript. Below are the examples of how classes are declared and used:
Key points to remember about classes:
- Unlike functions, classes are not hoisted. A class cannot be used before it is declared.
- A class can inherit properties and methods from other classes by using the extend keyword.
- All the syntaxes inside the class must follow the strict mode(‘use strict’) of javascript. An error will be thrown if the strict mode rules are not followed.
8. What are generator functions?
Introduced in the ES6 version, generator functions are a special class of functions. They can be stopped midway and then continue from where they had stopped. Generator functions are declared with the function* keyword instead of the normal function keyword:
In normal functions, we use the return keyword to return a value and as soon as the return statement gets executed, the function execution stops:
In the case of generator functions, when called, they do not execute the code, instead, they return a generator object . This generator object handles the execution.
The generator object consists of a method called next() , this method when called, executes the code until the nearest yield statement, and returns the yield value. For example, if we run the next() method on the above code:
As one can see the next method returns an object consisting of a value and done properties. Value property represents the yielded value. Done property tells us whether the function code is finished or not. (Returns true if finished).
Generator functions are used to return iterators. Let’s see an example where an iterator is returned:
As you can see in the code above, the last line returns done:true , since the code reaches the return statement.
9. Explain WeakSet in javascript.
In javascript, a Set is a collection of unique and ordered elements. Just like Set, WeakSet is also a collection of unique and ordered elements with some key differences:
- Weakset contains only objects and no other type.
- An object inside the weakset is referenced weakly. This means, that if the object inside the weakset does not have a reference, it will be garbage collected.
- Unlike Set, WeakSet only has three methods, add() , delete() and has() .
10. Why do we use callbacks?
A callback function is a method that is sent as an input to another function (now let us name this other function "thisFunction"), and it is performed inside the thisFunction after the function has completed execution.
JavaScript is a scripting language that is based on events. Instead of waiting for a reply before continuing, JavaScript will continue to run while monitoring for additional events. Callbacks are a technique of ensuring that a particular code does not run until another code has completed its execution.
11. Explain WeakMap in javascript.
In javascript, Map is used to store key-value pairs. The key-value pairs can be of both primitive and non-primitive types. WeakMap is similar to Map with key differences:
- The keys and values in weakmap should always be an object.
- If there are no references to the object, the object will be garbage collected.
12. What is Object Destructuring?
Object destructuring is a new way to extract elements from an object or an array.
- Object destructuring: Before ES6 version:
The same example using object destructuring:
As one can see, using object destructuring we have extracted all the elements inside an object in one line of code. If we want our new variable to have the same name as the property of an object we can remove the colon:
- Array destructuring: Before ES6 version:
13. Difference between prototypal and classical inheritance
Programers build objects, which are representations of real-time entities, in traditional OO programming. Classes and objects are the two sorts of abstractions. A class is a generalization of an object, whereas an object is an abstraction of an actual thing. A Vehicle, for example, is a specialization of a Car. As a result, automobiles (class) are descended from vehicles (object).
Classical inheritance differs from prototypal inheritance in that classical inheritance is confined to classes that inherit from those remaining classes, but prototypal inheritance allows any object to be cloned via an object linking method. Despite going into too many specifics, a prototype essentially serves as a template for those other objects, whether they extend the parent object or not.
14. What is a Temporal Dead Zone?
Temporal Dead Zone is a behaviour that occurs with variables declared using let and const keywords. It is a behaviour where we try to access a variable before it is initialized. Examples of temporal dead zone:
In the code above, both in the global scope and functional scope, we are trying to access variables that have not been declared yet. This is called the Temporal Dead Zone .
15. What do you mean by JavaScript Design Patterns?
JavaScript design patterns are repeatable approaches for errors that arise sometimes when building JavaScript browser applications. They truly assist us in making our code more stable.
They are divided mainly into 3 categories
- Creational Design Pattern
- Structural Design Pattern
- Behavioral Design Pattern.
- Creational Design Pattern: The object generation mechanism is addressed by the JavaScript Creational Design Pattern. They aim to make items that are appropriate for a certain scenario.
- Structural Design Pattern: The JavaScript Structural Design Pattern explains how the classes and objects we've generated so far can be combined to construct bigger frameworks. This pattern makes it easier to create relationships between items by defining a straightforward way to do so.
- Behavioral Design Pattern: This design pattern highlights typical patterns of communication between objects in JavaScript. As a result, the communication may be carried out with greater freedom.
16. Is JavaScript a pass-by-reference or pass-by-value language?
The variable's data is always a reference for objects, hence it's always pass by value. As a result, if you supply an object and alter its members inside the method, the changes continue outside of it. It appears to be pass by reference in this case. However, if you modify the values of the object variable, the change will not last, demonstrating that it is indeed passed by value.
17. Difference between Async/Await and Generators usage to achieve the same functionality.
- Generator functions are run by their generator yield by yield which means one output at a time, whereas Async-await functions are executed sequentially one after another.
- Async/await provides a certain use case for Generators easier to execute.
- The output result of the Generator function is always value: X, done: Boolean, but the return value of the Async function is always an assurance or throws an error.
18. What are the primitive data types in JavaScript?
A primitive is a data type that isn't composed of other data types. It's only capable of displaying one value at a time. By definition, every primitive is a built-in data type (the compiler must be knowledgeable of them) nevertheless, not all built-in datasets are primitives. In JavaScript, there are 5 different forms of basic data. The following values are available:
19. What is the role of deferred scripts in JavaScript?
The processing of HTML code while the page loads are disabled by nature till the script hasn't halted. Your page will be affected if your network is a bit slow, or if the script is very hefty. When you use Deferred, the script waits for the HTML parser to finish before executing it. This reduces the time it takes for web pages to load, allowing them to appear more quickly.
20. What has to be done in order to put Lexical Scoping into practice?
To support lexical scoping, a JavaScript function object's internal state must include not just the function's code but also a reference to the current scope chain.
21. What is the purpose of the following JavaScript code?
Every executing function, code block, and script as a whole in JavaScript has a related object known as the Lexical Environment. The preceding code line returns the value in scope.
JavaScript Coding Interview Questions
1. guess the outputs of the following codes:.
- Code 1 - Outputs 2 and 12 . Since, even though let variables are not hoisted, due to the async nature of javascript, the complete function code runs before the setTimeout function. Therefore, it has access to both x and y.
- Code 2 - Outputs 3 , three times since variable declared with var keyword does not have block scope. Also, inside the for loop, the variable i is incremented first and then checked.
- Code 3 - Output in the following order:
Even though the second timeout function has a waiting time of zero seconds, the javascript engine always evaluates the setTimeout function using the Web API, and therefore, the complete function executes before the setTimeout function can execute.
2. Guess the outputs of the following code:
Answers: Code 1 - Output will be {name: “Akki”}. Adding objects as properties of another object should be done carefully. Writing x[y] = {name:”Vivek”} , is same as writing x[‘object Object’] = {name:”Vivek”} , While setting a property of an object, javascript coerces the parameter into a string. Therefore, since y is an object, it will be converted to ‘object Object’. Both x[y] and x[z] are referencing the same property. Code 2 - Outputs in the following order:
Code 3 - Output in the following order due to equality coercion:
3. Guess the output of the following code:
Output is NaN . random() function has functional scope since x is declared and hoisted in the functional scope. Rewriting the random function will give a better idea about the output:
4. Guess the outputs of the following code:
Answers: Code 1 - Output in the following order:
Reason - The first output is undefined since when the function is invoked, it is invoked referencing the global object:
Code 2 - Outputs in the following order:
Since we are using the arrow function inside func2, this keyword refers to the global object. Code 3 - Outputs in the following order:
Only in the IIFE inside the function f , this keyword refers to the global/window object.
5. Guess the outputs of the following code:
**note - code 2 and code 3 require you to modify the code, instead of guessing the output..
Answers - Code 1 - Outputs 45 . Even though a is defined in the outer function, due to closure the inner functions have access to it. Code 2 - This code can be modified by using closures,
Code 3 - Can be modified in two ways: Using let keyword:
Using closure:
6. Write a function that performs binary search on a sorted array.
function binarySearch ( arr,value,startPos,endPos ) { if (startPos > endPos) return - 1 ; let middleIndex = Math .floor(startPos+endPos)/ 2 ; if (arr[middleIndex] === value) return middleIndex; elsif ( arr[middleIndex] > value ) { return binarySearch(arr,value,startPos,middleIndex- 1 ); } else { return binarySearch(arr,value,middleIndex+ 1 ,endPos); } }
7. Implement a function that returns an updated array with r right rotations on an array of integers a .
Given the following array: [2,3,4,5,7] Perform 3 right rotations: First rotation : [7,2,3,4,5] , Second rotation : [5,7,2,3,4] and, Third rotation: [4,5,7,2,3]
return [4,5,7,2,3]
8. Write the code for dynamically inserting new components.
<html> <head> <title>inserting new components dynamically</title> <script type="text/javascript"> function addNode () { var newP = document. createElement("p"); var textNode = document.createTextNode(" This is other node"); newP.appendChild(textNode); document.getElementById("parent1").appendChild(newP); } </script> </head> <body> <p id="parent1">firstP<p> </body> </html>
9. Write the code given If two strings are anagrams of one another, then return true.
var firstWord = "Deepak" ; var secondWord = "Aman" ; isAnagram(wordOne, wordTwo); // true function isAnagram ( one, two ) { //Change both words to lowercase for case insensitivity.. var a = one.toLowerCase(); var b = two.toLowerCase(); // Sort the strings, then combine the array to a string. Examine the outcomes. a = a.split( "" ).sort().join( "" ); b = b.split( "" ).sort().join( "" ); return a === b; }
10. Write the code to find the vowels
const findVowels = str => { let count = 0 const vowels = [ 'a' , 'e' , 'i' , 'o' , 'u' ] for ( let char of str.toLowerCase()) { if (vowels.includes(char)) { count++ } } return count }
11. In JavaScript, how do you turn an Object into an Array []?
let obj = { id : "1" , name : "user22" , age : "26" , work : "programmer" }; //Method 1: Convert the keys to Array using - Object.keys() console .log( Object .keys(obj)); // ["id", "name", "age", "work"] // Method 2 Converts the Values to Array using - Object.values() console .log( Object .values(obj)); // ["1", "user22r", "26", "programmer"] // Method 3 Converts both keys and values using - Object.entries() console .log( Object .entries(obj)); //[["id", "1"],["name", "user22"],["age", "26"],["work", “programmer"]]
12. What is the output of the following code?
It is preferable to keep the JavaScript, CSS, and HTML in distinct Separate 'javascript' files. Dividing the code and HTML sections will make them easier to understand and deal with. This strategy is also simpler for several programmers to use at the same time. JavaScript code is simple to update. Numerous pages can utilize the same group of JavaScript Codes. If we utilize External JavaScript scripts and need to alter the code, we must do it just once. So that we may utilize a number and maintain it much more easily. Remember that professional experience and expertise are only one aspect of recruitment. Previous experience and personal skills are both vital in landing (or finding the ideal applicant for the job.
Remember that many JavaScript structured interviews are free and have no one proper answer. Interviewers would like to know why you answered the way you did, not if you remembered the answer. Explain your answer process and be prepared to address it. If you're looking to further enhance your JavaScript skills, consider enrolling in this free JavaScript course by Scaler Topics to gain hands-on experience and improve your problem-solving abilities.
Recommended Resources
- JavaScript Cheat Sheet: Basics to Advanced(2023)
- Online Javascript Compiler
- Top JavaScript Features You Must Know
- 50 JavaScript MCQ With Answers
- Top 15+ JavaScript Projects for Beginners to Advanced [With Source Code]
- 9 Best JavaScript IDE & Source Code Editors [2023]
- Top ES6 Interview Questions (2023)
- 10 Best JavaScript Books for Beginners to Advanced [2023]
- 30+ Top Node.js Interview Questions (2023)
- Typescript vs Javascript: What’s The Difference?
- Top 5 JavaScript Libraries You Must Know in 2023
- Difference Between HTML and JavaScript
- Javascript Vs jQuery: What’s the Difference? [2023]
- Javascript Vs Python: What’s The Difference? [2023]
- Difference Between Java and Javascript
- Difference between Typescript and Javascript
Interview Guides
- The Ultimate Guide to Acing Your Technical Interview
- 300+ Must Do Coding Questions from Interviews
- Mock Interview
- InterviewBit - No.1 Resource for Tech Interview Preparation
Coding Problems
Javascript mcq.
Which of the following statements regarding JavaScript is true?
Which of the following JavaScript code snippets will provide the desired result?
In JavaScript, which of the following is not a bug?
JavaScript was created by which company?
What do you mean by block statement in JavaScript?
Argument class is
Which of these String object functions gives the calling data type transformed to upper case?
The JSON() method's property is
Which of the below do not belong in the JavaScript Data Types category?
The interpreter for Javascript is
- Privacy Policy
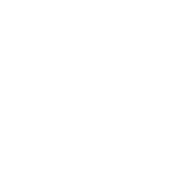
- Practice Questions
- Programming
- System Design
- Fast Track Courses
- Online Interviewbit Compilers
- Online C Compiler
- Online C++ Compiler
- Online Java Compiler
- Online Python Compiler
- Interview Preparation
- Java Interview Questions
- Sql Interview Questions
- Python Interview Questions
- Javascript Interview Questions
- Angular Interview Questions
- Networking Interview Questions
- Selenium Interview Questions
- Data Structure Interview Questions
- Data Science Interview Questions
- System Design Interview Questions
- Hr Interview Questions
- Html Interview Questions
- C Interview Questions
- Amazon Interview Questions
- Facebook Interview Questions
- Google Interview Questions
- Tcs Interview Questions
- Accenture Interview Questions
- Infosys Interview Questions
- Capgemini Interview Questions
- Wipro Interview Questions
- Cognizant Interview Questions
- Deloitte Interview Questions
- Zoho Interview Questions
- Hcl Interview Questions
- Highest Paying Jobs In India
- Exciting C Projects Ideas With Source Code
- Top Java 8 Features
- Angular Vs React
- 10 Best Data Structures And Algorithms Books
- Best Full Stack Developer Courses
- Best Data Science Courses
- Python Commands List
- Data Scientist Salary
- Maximum Subarray Sum Kadane’s Algorithm
- Python Cheat Sheet
- C++ Cheat Sheet
- Javascript Cheat Sheet
- Git Cheat Sheet
- Java Cheat Sheet
- Data Structure Mcq
- C Programming Mcq
- Javascript Mcq
1 Million +
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Tutorial Playlist
Javascript tutorial: learn javascript from scratch, an introduction to javascript: here is all you need to know, javascript hello world: here's what you need to know, all you need to know about javascript arrays, using array filter in javascript to filter array elements, everything you need to know about array reduce javascript, introduction to javascript loops: do-while, for, for-in loops, all you need to learn about javascript functions, the best guide on how to implement javascript closures, javascript “this” keyword and how to implement it, how to implement javascript form validation, an introduction guide to javascript regex, how to validate an email address in javascript, all you need to know about javascript promises, how to implement javascript async/await, javascript dom tutorial: 2020 edition, javascript objects: properties, methods, and accessors, an introduction to javascript games: the best guide, an easy guide to build a calculator app in javascript, javascript projects: the best guide, what is oop in javascript how is it implemented, the best guide to understanding javascript learning path, java vs .net: which is the best technology to choose, top 80+ javascript interview questions and answers for 2024, typeof in javascript: checking data types using the typeof operator, tips and tricks that you should know before going to a coding interview, callback functions in javascript, javascript events.
Lesson 23 of 27 By Aryan Gupta

Table of Contents
Reviewed and fact-checked by Sayantoni Das
JavaScript is an open-source programming language . It is designed for creating web-centric applications. It is lightweight and interpreted, which makes it much faster than other languages. JavaScript is integrated with HTML, which makes it easier to implement JavaScript in web applications.
Learn more: JavaScript Tutorial: Learn JavaScript from Scratch
This article provides you with a comprehensive list of common JavaScript interview questions and answers that often come up in interviews. It will also help you understand the fundamental concepts of JavaScript .
Want a Top Software Development Job? Start Here!
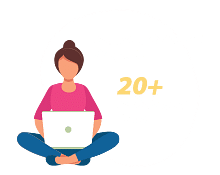
JavaScript Interview Questions for Freshers
Here are some basic JavaScript interview questions and answers for you to prepare during your interviews.
1. What do you understand about JavaScript?
Fig: JavaScript Logo
JavaScript is a popular web scripting language and is used for client-side and server-side development. The JavaScript code can be inserted into HTML pages that can be understood and executed by web browsers while also supporting object-oriented programming abilities.
2. What’s the difference between JavaScript and Java?
3. what are the various data types that exist in javascript.
These are the different types of data that JavaScript supports:
- Boolean - For true and false values
- Null - For empty or unknown values
- Undefined - For variables that are only declared and not defined or initialized
- Number - For integer and floating-point numbers
- String - For characters and alphanumeric values
- Object - For collections or complex values
- Symbols - For unique identifiers for objects
4. What are the features of JavaScript?
These are the features of JavaScript:
- Lightweight, interpreted programming language
- Cross-platform compatible
- Open-source
- Object-oriented
- Integration with other backend and frontend technologies
- Used especially for the development of network-based applications
5. What are the advantages of JavaScript over other web technologies?
These are the advantages of JavaScript:
Enhanced Interaction
JavaScript adds interaction to otherwise static web pages and makes them react to users’ inputs.
Quick Feedback
There is no need for a web page to reload when running JavaScript. For example, form input validation.
Rich User Interface
JavaScript helps in making the UI of web applications look and feel much better.
JavaScript has countless frameworks and libraries that are extensively used for developing web applications and games of all kinds.
6. How do you create an object in JavaScript?
Since JavaScript is essentially an object-oriented scripting language, it supports and encourages the usage of objects while developing web applications.
const student = {
name: 'John',
age: 17
7. How do you create an array in JavaScript?
Here is a very simple way of creating arrays in JavaScript using the array literal:
var a = [];
var b = [‘a’, ‘b’, ‘c’, ‘d’, ‘e’];
Preparing Your Blockchain Career for 2024
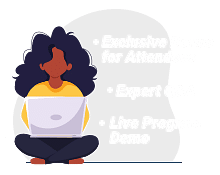
8. What are some of the built-in methods in JavaScript?
9. what are the scopes of a variable in javascript.
The scope of a variable implies where the variable has been declared or defined in a JavaScript program. There are two scopes of a variable:
Global Scope
Global variables, having global scope are available everywhere in a JavaScript code.
Local Scope
Local variables are accessible only within a function in which they are defined.
10. What is the ‘this’ keyword in JavaScript?
The ‘this’ keyword in JavaScript refers to the currently calling object. It is commonly used in constructors to assign values to object properties.
11. What are the conventions of naming a variable in JavaScript?
Following are the naming conventions for a variable in JavaScript:
- Variable names cannot be similar to that of reserved keywords. For example, var, let, const, etc.
- Variable names cannot begin with a numeric value. They must only begin with a letter or an underscore character.
- Variable names are case-sensitive.
12. What is Callback in JavaScript?
In JavaScript, functions are objects and therefore, functions can take other functions as arguments and can also be returned by other functions.
Fig: Callback function
A callback is a JavaScript function that is passed to another function as an argument or a parameter. This function is to be executed whenever the function that it is passed to gets executed.
13. How do you debug a JavaScript code?
All modern web browsers like Chrome, Firefox, etc. have an inbuilt debugger that can be accessed anytime by pressing the relevant key, usually the F12 key. There are several features available to users in the debugging tools.
We can also debug a JavaScript code inside a code editor that we use to develop a JavaScript application—for example, Visual Studio Code, Atom, Sublime Text, etc.
14. What is the difference between Function declaration and Function expression?
15. what are the ways of adding javascript code in an html file.
There are primarily two ways of embedding JavaScript code:
- We can write JavaScript code within the script tag in the same HTML file; this is suitable when we need just a few lines of scripting within a web page.
- We can import a JavaScript source file into an HTML document; this adds all scripting capabilities to a web page without cluttering the code.
Intermediate JavaScript Interview Questions and Answers
Here are some intermediate level JavaScript interview questions and answers for you to prepare during your interviews.
16. What do you understand about cookies?
Fig: Browser cookies
A cookie is generally a small data that is sent from a website and stored on the user’s machine by a web browser that was used to access the website. Cookies are used to remember information for later use and also to record the browsing activity on a website.
17. How would you create a cookie?
The simplest way of creating a cookie using JavaScript is as below:
document.cookie = "key1 = value1; key2 = value2; expires = date";
18. How would you read a cookie?
Reading a cookie using JavaScript is also very simple. We can use the document.cookie string that contains the cookies that we just created using that string.
The document.cookie string keeps a list of name-value pairs separated by semicolons, where ‘name’ is the name of the cookie, and ‘value’ is its value. We can also use the split() method to break the cookie value into keys and values.
19. How would you delete a cookie?
To delete a cookie, we can just set an expiration date and time. Specifying the correct path of the cookie that we want to delete is a good practice since some browsers won’t allow the deletion of cookies unless there is a clear path that tells which cookie to delete from the user’s machine.
function delete_cookie(name) {
document.cookie = name + "=; Path=/; Expires=Thu, 01 Jan 1970 00:00:01 GMT;";
20. What’s the difference between let and var?
Both let and var are used for variable and method declarations in JavaScript. So there isn’t much of a difference between these two besides that while var keyword is scoped by function, the let keyword is scoped by a block.
21. What are Closures in JavaScript?
Closures provide a better, and concise way of writing JavaScript code for the developers and programmers. Closures are created whenever a variable that is defined outside the current scope is accessed within the current scope.
function hello(name) {
var message = "hello " + name;
return function hello() {
console.log(message);
//generate closure
var helloWorld = hello("World");
//use closure
helloWorld();
22. What are the arrow functions in JavaScript?
Arrow functions are a short and concise way of writing functions in JavaScript. The general syntax of an arrow function is as below:
const helloWorld = () => {
console.log("hello world!");
23. What are the different ways an HTML element can be accessed in a JavaScript code?
Here are the ways an HTML element can be accessed in a JavaScript code:
- getElementByClass(‘classname’): Gets all the HTML elements that have the specified classname.
- getElementById(‘idname’): Gets an HTML element by its ID name.
- getElementbyTagName(‘tagname’): Gets all the HTML elements that have the specified tagname.
- querySelector(): Takes CSS style selector and returns the first selected HTML element.
24. What are the ways of defining a variable in JavaScript?
There are three ways of defining a variable in JavaScript:
This is used to declare a variable and the value can be changed at a later time within the JavaScript code.
We can also use this to declare/define a variable but the value, as the name implies, is constant throughout the JavaScript program and cannot be modified at a later time.
This mostly implies that the values can be changed at a later time within the JavaScript code.
25. What are Imports and Exports in JavaScript?
Imports and exports help in writing modular code for our JavaScript applications . With the help of imports and exports, we can split a JavaScript code into multiple files in a project. This greatly simplifies the application source code and encourages code readability.
export const sqrt = Math.sqrt;
export function square(x) {
return x * x;
export function diag(x, y) {
return sqrt(square(x) + square(y));
This file exports two functions that calculate the squares and diagonal of the input respectively.
import { square, diag } from "calc";
console.log(square(4)); // 16
console.log(diag(4, 3)); // 5
Therefore, here we import those functions and pass input to those functions to calculate square and diagonal.
26. What is the difference between Document and Window in JavaScript?
27. what are some of the javascript frameworks and their uses.
JavaScript has a collection of many frameworks that aim towards fulfilling the different aspects of the web application development process. Some of the prominent frameworks are:
- React - Frontend development of a web application
- Angular - Frontend development of a web application
- Node - Backend or server-side development of a web application
28. What is the difference between Undefined and Undeclared in JavaScript?
29. what is the difference between undefined and null in javascript, 30. what is the difference between session storage and local storage, 31. what are the various data types that exist in javascript.
Javascript consists of two data types, primitive data types, and non-primitive data types.
- Primitive Data types: These data types are used to store a single value. Following are the sub-data types in the Primitive data type.
- Boolean Data Types: It stores true and false values.
var b = 4;
(a == b) // returns false
(a == c) //returns true
- Null data Types: It stores either empty or unknown values.
var z = null;
- Undefined data Types: It stores variables that are only declared, but not defined or initialized.
var a; // a is undefined
var b = undefined; // we can also set the value of b variable as undefined
- Number Data Types: It stores integer as well as floating-point numbers.
var x = 4; //without decimal
var y = 5.6; //with decimal
- String data Types: It stores characters and alphanumeric values.
var str = "Raja Ram Mohan"; //using double quotes
var str2 = 'Raja Rani'; //using single quotes
- Symbols Data Types: It stores unique identifiers for objects.
var symbol1 = Symbol('symbol');
- BigInt Data Types: It stores the Number data types that are large integers and are above the limitations of number data types.
var bigInteger = 234567890123456789012345678901234567890;
- Non-Primitive Data Types
Non-Primitive data types are used to store multiple as well as complex values.
// Collection of data in key-value pairs
var obj1 = {
y: "Hello world!",
z: function(){
return this.x;
// Data collection with an ordered list
var array1 = [5, "Hello", true, 4.1];
32. What is the ‘this’ keyword in JavaScript?
The Keyword ‘this’ in JavaScript is used to call the current object as a constructor to assign values to object properties.
33. What is the difference between Call and Apply? (explain in detail with examples)
Call uses arguments separately.
function sayHello()
return "Hello " + this.name;
var obj = {name: "Sandy"};
sayHello.call(obj);
// Returns "Hello Sandy"
Apply uses an argument as an array.
function saySomething(message)
return this.name + " is " + message;
var person4 = {name: "John"};
saySomething.apply(person4, ["awesome"]);
34. What are the scopes of a variable in JavaScript?
The scope of variables in JavaScript is used to determine the accessibility of variables and functions at various parts of one’s code. There are three types of scopes of a variable, global scope, function scope, block scope
- Global Scope: It is used to access the variables and functions from anywhere inside the code.
var globalVariable = "Hello world";
function sendMessage(){
return globalVariable; // globalVariable is accessible as it's written in global space
function sendMessage2(){
return sendMessage(); // sendMessage function is accessible as it's written in global space
sendMessage2(); // Returns “Hello world”
- Function scope: It is used to declare the function and variables inside the function itself and not outside.
function awesomeFunction()
var a = 3;
var multiplyBy3 = function()
console.log(a*3); // Can access variable "a" since a and multiplyBy3 both are written inside the same function
console.log(a); // a is written in local scope and can't be accessed outside and throws a reference error
multiplyBy3(); // MultiplyBy3 is written in local scope thus it throws a reference error
- Block Scope: It uses let and const to declare the variables.
let x = 45;
console.log(x); // Gives reference error since x cannot be accessed outside of the block
for(let i=0; i<2; i++){
// do something
console.log(i); // Gives reference error since i cannot be accessed outside of the for loop block
35. What are the arrow functions in JavaScript?
Arrow functions are used to write functions with a short and concise syntax. Also, it does not require a function keyword for declaration. An arrow function can be omitted with curly braces { } when we have one line of code.
syntax of an arrow function:
// Traditional Function Expression
var add = function(a,b)
return a + b;
// Arrow Function Expression
var arrowAdd = (a,b) => a + b;
36. Explain Hoisting in javascript. (with examples)
Hoisting in javascript is the default process behavior of moving declaration of all the variables and functions on top of the scope where scope can be either local or global.
Example 1:
hoistedFunction(); // " Hi There! " is an output that is declared as function even after it is called
function hoistedFunction(){
console.log(" Hi There! ");
hoistedVariable = 5;
console.log(hoistedVariable); // outputs 5 though the variable is declared after it is initialized
var hoistedVariable;
37. Difference between “ == “ and “ === “ operators (with examples)
- “==” operator is a comparison operator that used to compare the values
- “===” operator is also a comparison operator that is used to compare the values as well as types.
var y = "3";
(x == y) // it returns true as the value of both x and y is the same
(x === y) // it returns false as the typeof x is "number" and typeof y is "string"
38. Difference between var and let keyword
- Keyword “var”
- In JavaScript programming, the “var” keyword has been used from the very initial stages of JavaScript.
- We can perform functions with the help of the keyword “var’ by accessing various variables.
- Keyword “let”
- The Keyword “let” was added later in ECMAScript 2015 in JavaScript Programming.
- Variable declaration is very limited with the help of the “let” keyword that is declared in Block. Also, it might result in a ReferenceError as the variable was declared in the “temporal dead zone” at the beginning of the block.
39. Implicit Type Coercion in javascript (in detail with examples)
When the value of one data type is automatically converted into another data type, it is called Implicit type coercion in javascript.
- String coercion
var y = "4";
x + y // Returns "44"
- Boolean coercion
var b = 32;
if(a) { console.log(a) } // This code will run inside the block as the value of x is 0(Falsy)
if(b) { console.log(b) } // This code will run inside the block as the value of y is 32 (Truthy)
40. Is javascript a statically typed or a dynamically typed language?
Yes, JavaScript is a dynamically typed language and not statically.
41. NaN property in JavaScript
NaN property in JavaScript is the “Not-a-Number” value that is not a legal number.
42. Passed by value and passed by reference
- Passed By Values Are Primitive Data Types.
Consider the following example:
Here, the a=432 is a primitive data type i.e. a number type that has an assigned value by the operator. When the var b=a code gets executed, the value of ‘var a’ returns a new address for ‘var b’ by allocating a new space in the memory, so that ‘var b’ will be operated at a new location.
var a = 432;
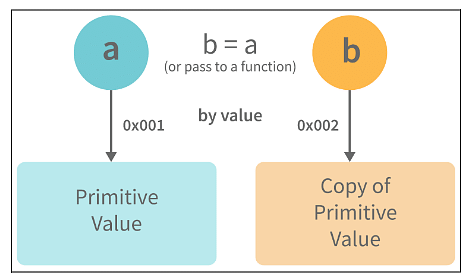
- Passed by References Are Non-primitive Data Types.
The reference of the 1st variable object i.e. ‘var obj’ is passed through the location of another variable i.e. ‘var obj2’ with the help of an assigned operator.
var obj = { name: "Raj", surname: "Sharma" };
var obj2 = obj;
43. Immediately Invoked Function in JavaScript
An Immediately Invoked Function also abbreviated as IIFE or IIFY runs as soon as it is defined. To run the function, it needs to be invoked otherwise the declaration of the function is returned.
(function()
// Do something;
44. Characteristics of javascript strict-mode
- Strict mode does not allow duplicate arguments and global variables.
- One cannot use JavaScript keywords as a parameter or function name in strict mode.
- All browsers support strict mode.
- Strict mode can be defined at the start of the script with the help of the keyword ‘use strict’.
45. Higher Order Functions (with examples)
Higher-order functions are the functions that take functions as arguments and return them by operating on other functions
function higherOrder(fn)
higherOrder(function() { console.log("Hello world") });
46. Self Invoking Functions
Self Invoking Functions is an automatically invoked function expression followed by (), where it does not need to be requested. Nevertheless, the declaration of the function is not able to be invoked by itself.
47. difference between exec () and test () methods
- It is an expression method in JavaScript that is used to search a string with a specific pattern.
- Once it has been found, the pattern will be returned directly, otherwise, it returns an “empty” result.
- It is an expression method in JavaScript that is also used to search a string with a specific pattern or text.
- Once it has been found, the pattern will return the Boolean value 'true', else it returns ‘false’.
48. currying in JavaScript (with examples)
In JavaScript, when a function of an argument is transformed into functions of one or more arguments is called Currying.
function add (a) {
return function(b){
return a + b;
49. Advantages of using External JavaScript
- External Javascript allows web designers and developers to collaborate on HTML and javascript files.
- It also enables you to reuse the code.
- External javascript makes Code readability simple.
50. What are object prototypes?
Following are the different object prototypes in javascript that are used to inherit particular properties and methods from the Object.prototype.
- Date objects are used to inherit properties from the Date prototype
- Math objects are used to inherit properties from the Math prototype
- Array objects are used to inherit properties from the Array prototype.
51. Types of errors in javascript
Javascript has two types of errors, Syntax error, and Logical error.
52. What is memoization?
In JavaScript, when we want to cache the return value of a function concerning its parameters, it is called memoization. It is used to speed up the application especially in case of complex, time consuming functions.
53. Recursion in a programming language
Recursion is a technique in a programming language that is used to iterate over an operation whereas a function calls itself repeatedly until we get the result.
54. Use of a constructor function (with examples)
Constructor functions are used to create single objects or multiple objects with similar properties and methods.
function Person(name,age,gender)
this.name = name;
this.age = age;
this.gender = gender;
var person1 = new Person("Vivek", 76, "male");
console.log(person1);
var person2 = new Person("Courtney", 34, "female");
console.log(person2);
55. Which method is used to retrieve a character from a certain index?
We can retrieve a character from a certain index with the help of charAt() function method.
56. What is BOM?
BOM is the Browser Object Model where users can interact with browsers that is a window, an initial object of the browser. The window object consists of a document, history, screen, navigator, location, and other attributes. Nevertheless, the window’s function can be called directly as well as by referencing the window.
57. Difference between client-side and server-side
- Client-side JavaScript
- Client-side JavaScript is made up of fundamental language and predefined objects that perform JavaScript in a browser.
- Also, it is automatically included in the HTML pages where the browser understands the script.
- Server-side Javascript
- Server-side JavaScript is quite similar to Client-side javascript.
- Server-side JavaScript can be executed on a server.
- The server-side JavaScript is deployed once the server processing is done.
58. What is the prototype design pattern?
The Prototype design Pattern is also known as a property or prototype pattern that is used to produce different objects as well as prototypes that are replicated from a template with a specific value.
59. Differences between declaring variables using var, let and const.
var variable1 = 31;
let variable2 = 89;
function catchValues()
console.log(variable1);
console.log(variable2);
// Both the variables are accessible from anywhere as their declaration is in the global scope
window.variable1; // Returns the value 31
window.variable2; // Returns undefined
Example 2: Using ‘const’ variable
const x = {name:"Vijay"};
x = {address: "Mumbai"}; // Throws an error
x.name = "Radha"; // No error is thrown
const y = 31;
y = 44; // Throws an error
60. Rest parameter and spread operator
- Rest Parameter(...)
- Rest parameter is used to declare the function with improved handling of parameters.
- Rest parameter syntax can be used to create functions to perform functions on the variable number of arguments.
- It also helps to convert any number of arguments into an array as well as helps in extracting some or all parts of the arguments.
- Spread Operator(...)
- In a function call, we use the spread operator.
- It's also to spread one or more arguments that are expected in a function call.
- The spread operator is used to take an array or an object and spread them.
61. Promises in JavaScript
Promises in JavaScript have four different states. They are as follows:
function sumOfThreeElements(...elements)
return new Promise((resolve,reject)=>{
if(elements.length > 3 )
reject("Just 3 elements or less are allowed");
let sum = 0;
let i = 0;
while(i < elements.length)
sum += elements[i];
i++;
resolve("Sum has been calculated: "+sum);
62. Classes in JavaScript
classes are syntactic sugars for constructor functions mentioned in the ES6 version of JavaScript. Classes are not hoisted-like Functions and can’t be used before it is declared. Also, it can inherit properties and methods from other classes with the help of extended keywords. If the strict mode (‘use strict’) is not followed, an error will be shown.
63. What are generator functions?
Generator functions are declared with a special class of functions and keywords using function*. It does not execute the code, however, it returns a generator object and handles the execution.
64. What is WeakSet?
WeakSet is a collection of unique and ordered elements that contain only objects which are referenced weakly.
65. What is the use of callbacks?
- A callback function is used to send input into another function and is performed inside another function.
- It also ensures that a particular code does not run until another code has completed its execution.
66. What is a WeakMap?
Weakmap is referred to as an object having keys and values, if the object is without reference, it is collected as garbage.
67. What is Object Destructuring? (with examples)
Object destructuring is a method to extract elements from an array or an object.
Example 1: Array Destructuring
const arr = [1, 2, 3];
const first = arr[0];
const second = arr[1];
const third = arr[2];
Example 2: Object Destructuring
const [first,second,third,fourth] = arr;
console.log(first); // Outputs 1
console.log(second); // Outputs 2
console.log(third); // Outputs 3
68. Prototypal vs Classical Inheritance
- Prototypal Inheritance
- Prototypal inheritance allows any object to be cloned via an object linking method and it serves as a template for those other objects, whether they extend the parent object or not.
- Classical Inheritance
- Classical inheritance is a class that inherits from the other remaining classes.
69. What is a Temporal Dead Zone?
Temporal Dead Zone is a behavior that occurs with variables declared using let and const keywords before they are initialized.
70. JavaScript Design Patterns
When we build JavaScript browser applications, there might be chances to occur errors where JavaScript approaches it in a repetitive manner. This repetitive approach pattern is called JavaScript design patterns. JavaScript design patterns consist of Creational Design Pattern, Structural Design Pattern, and Behavioral Design patterns.
71. Difference between Async/Await and Generators
- Async/Await
- Async-await functions are executed sequentially one after another in an easier way.
- Async/Await function might throw an error when the value is returned.
- Generator functions are executed with one output at a time by the generator’s yield by yield.
- The ‘value: X, done: Boolean’ is the output result of the Generator function.
72. Primitive data types
The primitive data types are capable of displaying one value at a time. It consists of Boolean, Undefined, Null, Number, and String data types.
73. Role of deferred scripts
The Deferred scripts are used for the HTML parser to finish before executing it.
74. What is Lexical Scoping?
Lexical Scoping in JavaScript can be performed when the internal state of the JavaScript function object consists of the function’s code as well as references concerning the current scope chain.
75. What is this [[[]]]?
This ‘[[[]]]’ is a three-dimensional array.
76. Are Java and JavaScript the same?
Yes, Java and JavaScript are the same.
77. How to detect the OS of the client machine using JavaScript?
The OS on the client machine can be detected with the help of navigator.appVersion string
78. Requirement of debugging in JavaScript
- To debug the code, we can use web browsers such as Google Chrome, and Mozilla Firefox.
- We can debug in JavaScript with the help of two methods, console.log() and debugger keyword.
79. What are the pop-up boxes available in JavaScript?
Pop-up boxes available in JavaScript are Alert Box, Confirm Box, and Prompt Box.
Advanced JS Interview Questions and Answers
Here are some advanced level JavaScript interview questions and answers for you to prepare during your interviews.
80. How do you empty an array in JavaScript?
There are a few ways in which we can empty an array in JavaScript:
- By assigning array length to 0:
var arr = [1, 2, 3, 4];
arr.length = 0;
- By assigning an empty array:
- By popping the elements of the array:
while (arr.length > 0) {
arr.pop();
- By using the splice array function:
arr.splice(0, arr.length);
81. What is the difference between Event Capturing and Event Bubbling?
82. what is the strict mode in javascript.
Strict mode in JavaScript introduces more stringent error-checking in a JavaScript code.
- While in Strict mode, all variables have to be declared explicitly, values cannot be assigned to a read-only property, etc.
- We can enable strict mode by adding ‘use strict’ at the beginning of a JavaScript code, or within a certain segment of code.
83. What would be the output of the below JavaScript code?
var a = 10;
if (function abc(){})
a += typeof abc;
console.log(a);
The output of this JavaScript code will be 10undefined. The if condition statement in the code evaluates using eval. Hence, eval(function abc(){}) will return function abc(){}.
Inside the if statement, executing typeof abc returns undefined because the if statement code executes at run time while the statement inside the if the condition is being evaluated.
84. Can you write a JavaScript code for adding new elements in a dynamic manner?
<script type="text/javascript">
function addNode() {
var newP = document.createElement("p");
var textNode = document.createTextNode(" This is a new text node");
newP.appendChild(textNode); document.getElementById("firstP").appendChild(newP);
</script>
85. What is the difference between Call and Apply?
86. what will be the output of the following code.
var Bar = Function Foo()
typeof Foo();
The output would be a reference error since a function definition can only have a single reference variable as its name.
87. What will be the output of the following code?
var Student = {
college: "abc",
var stud1 = Object.create(Student);
delete stud1.college;
console.log(stud1.company);
This is essentially a simple example of object-oriented programming. Therefore, the output will be ‘abc’ as we are accessing the property of the student object.
88. How do you remove duplicates from a JavaScript array?
There are two ways in which we can remove duplicates from a JavaScript array:
By Using the Filter Method
To call the filter() method , three arguments are required. These are namely array, current element, and index of the current element.
By Using the For Loop
An empty array is used for storing all the repeating elements.
81. Can you draw a simple JavaScript DOM (Document Object Model)?
As you prepare for your upcoming job interview, we hope that these JavaScript Interview Questions and answers have provided more insight into what types of questions you are likely to be asked.
Choose The Right Software Development Program
This table compares various courses offered by Simplilearn, based on several key features and details. The table provides an overview of the courses' duration, skills you will learn, additional benefits, among other important factors, to help learners make an informed decision about which course best suits their needs.
Program Name Full Stack Java Developer Career Bootcamp Automation Testing Masters Program Post Graduate Program in Full Stack Web Development Geo IN All Non-US University Simplilearn Simplilearn Caltech Course Duration 11 Months 11 Months 9 Months Coding Experience Required Basic Knowledge Basic Knowledge Basic Knowledge Skills You Will Learn 15+ Skills Including Core Java, SQL, AWS, ReactJS, etc. Java, AWS, API Testing, TDD, etc. Java, DevOps, AWS, HTML5, CSS3, etc. Additional Benefits Interview Preparation Exclusive Job Portal 200+ Hiring Partners Structured Guidance Learn From Experts Hands-on Training Caltech CTME Circle Membership Learn 30+ Tools and Skills 25 CEUs from Caltech CTME Cost $$ $$ $$$ Explore Program Explore Program Explore Program
Get Ahead of the Curve and Master JavaScript Today
Are you wondering how you can gain the skills necessary to take advantage of JavaScript’s immense popularity now that you are familiar with JS Interview Questions and Answers? We have got your back! We offer a comprehensive Post Graduate Program in Full Stack Web Development , which will help you get a job as a software engineer upon completion.
To learn more, check out our Youtube video that provides a quick introduction to JavaScript Interview Questions and answers and helps in clearing doubts for your next JavaScript interview. If you’re an aspiring web and mobile developer , JavaScript training will broaden your skills and career horizons.
Want to ace the upcoming JavaScript interview? There's nowhere else to look! We guarantee you're ready to wow prospective employers with our extensive list of the Top 80+ JavaScript Interview Questions and Answers, which covers everything from fundamental ideas to complex subjects. Our Java Course will help you brush up on your JavaScript knowledge and approach any interview with confidence, regardless of experience level.
Do you have any questions for us? Please mention it in the comments section below and we'll have our experts answer it for you at the earliest.
Find our Full Stack Java Developer Online Bootcamp in top cities:
About the author.
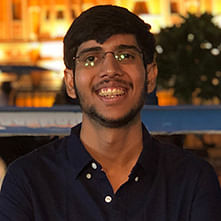
Aryan is a tech enthusiast who likes to stay updated about trending technologies of today. He is passionate about all things technology, a keen researcher, and writes to inspire. Aside from technology, he is an active football player and a keen enthusiast of the game.
Recommended Resources
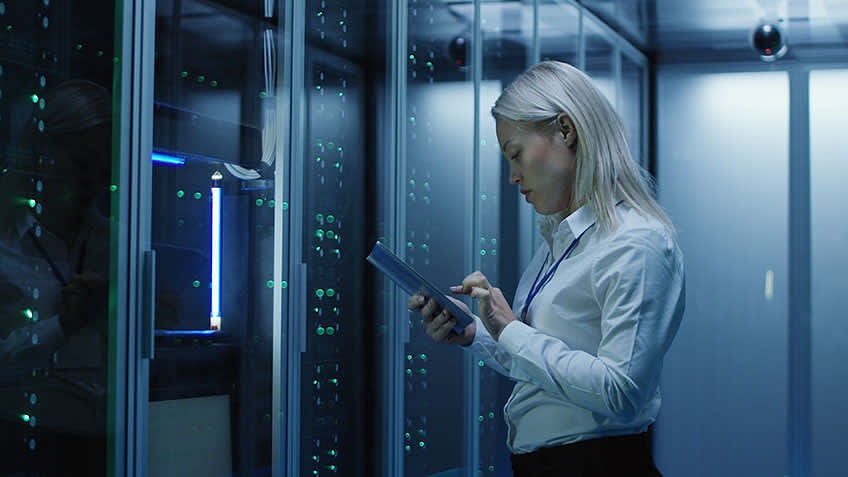
60+ Top Angular Interview Questions With Answers for 2024
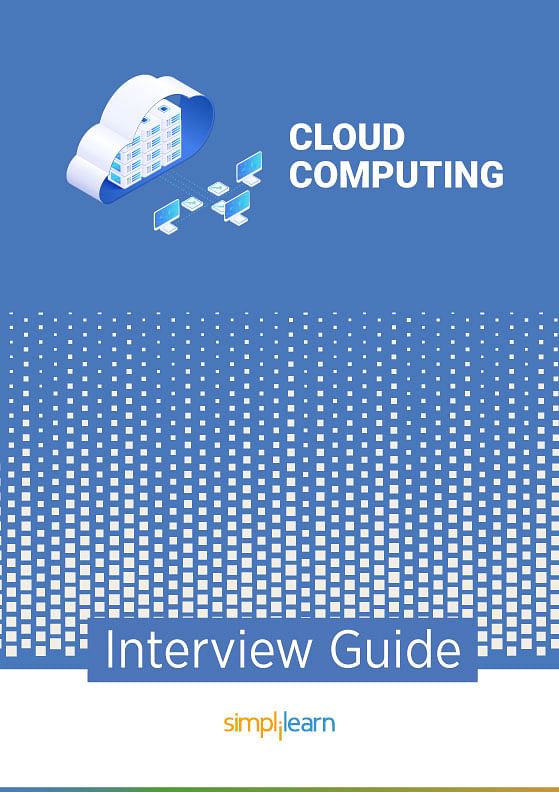
Cloud Computing Interview Guide
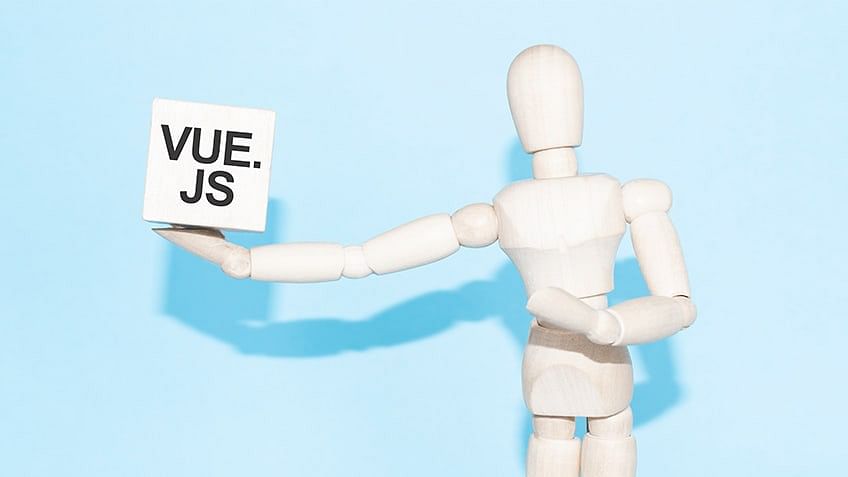
Top 50 Vue.js Interview Questions for 2024
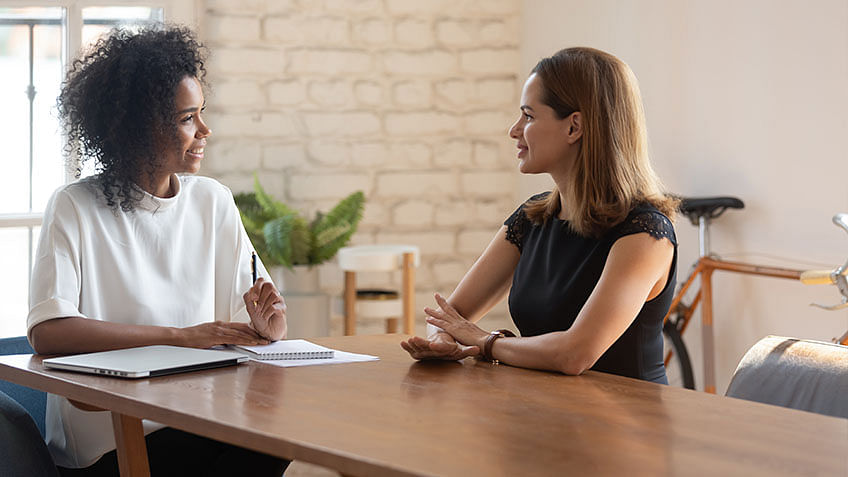
180+ Core Java Interview Questions and Answers for 2024

Top 60 C++ Interview Questions and Answers for 2024
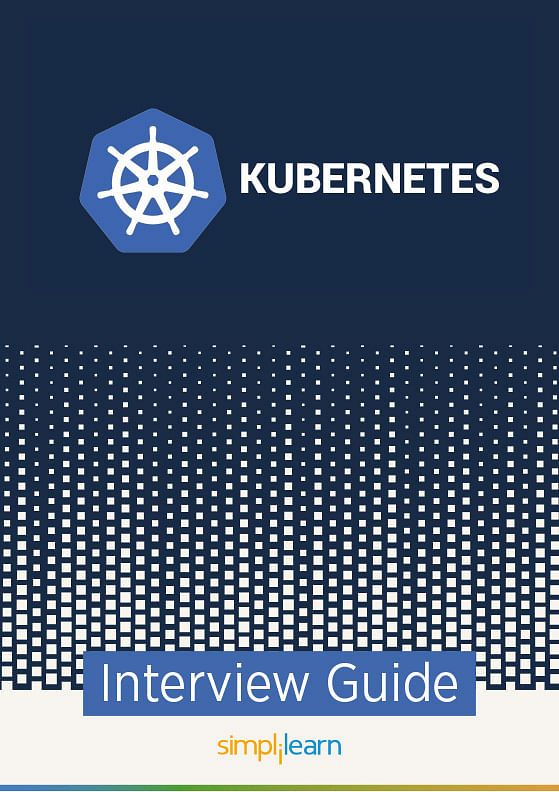
Kubernetes Interview Guide
- PMP, PMI, PMBOK, CAPM, PgMP, PfMP, ACP, PBA, RMP, SP, and OPM3 are registered marks of the Project Management Institute, Inc.

Precision in Payroll - Acing Taxes and Compliance
Webinar HR Metrics Magic: Transforming Insights into Actions Register Now
- Interview Questions
Top 50 JavaScript coding Interview Questions and Answers
JavaScript is one of the widely used programming languages and used to create engaging and dynamic websites. Due to its vast scope, JavaScript interviews can be challenging . However, thorough preparation can help overcome these challenges. An interviewee should familiarize themselves with the most basic concepts to the most complex libraries and frameworks.
These top 50 JavaScript interview questions and answers will help an intervie wee to thoroughly practice and prepare for their interview.
Top 50 JavaScript Coding Interview Questions
Basic javascript coding questions.
Basic JavaScript questions cover concepts like data types, variables and scoping, array, string manipulation, OOP (Object Oriented Programming), control flow, error handling, DOM manipulation, and asynchronous programming. The basic JavaScript coding interview questions are:
1. Write a JavaScript function to calculate the sum of two numbers.
When managers ask this question, they are looking for the candidate’s basic understanding of JavaScript. They assess their understanding of basic syntax along with problem-solving skills. This also helps evaluate the candidate’s coding style and attention to detail.
Sample answer: I would take two parameters and the following function can be used to calculate the sum of any 2 numbers that are passed as arguments.
function sumOfTwoNumbers(a, b) {
return a + b;
2. Write a JavaScript program to find the maximum number in an array.
A hiring manager asks this question to analyze the candidate’s ability to write clear and efficient code. It’s crucial for candidates to explain the code step-by-step while demonstrating bug-free code.
Sample answer:
function findMaxNumber(arr) {
return Math.max(…arr);
3. Write a JavaScript function to check if a given string is a palindrome (reads the same forwards and backwards).
The interviewer is looking for the candidate’s familiarity with loop constructs, JavaScript string methods, and other basic JavaScript syntax. They will evaluate the candidate’s skills based on the approach used to solve the palindrome problem.
function isPalindrome(str) {
return str === str.split(”).reverse().join(”);
4. Write a JavaScript program to reverse a given string.
Hiring managers are expecting an accurate solution that demonstrates the interviewee’s proficiency in JavaScript programming.
const reverseString = (str) => str.split(”).reverse().join(”);
5. Write a JavaScript function that takes an array of numbers and returns a new array with only the even numbers.
Interviewers are looking for candidates who can not only clearly explain the solution along with the code, but also show the ability to think logically and articulate their thought processes.
Sample answer: By using the filter method on the array, I can check if each element is even or not by using the modulus operator (%) with 2. The element is even if the result is 0. This can be included in the new array.
function filterEvenNumbers(numbers) {
return numbers.filter(num => num % 2 === 0);
6. Write a JavaScript program to calculate the factorial of a given number.
By asking this question, managers aim to assess the candidate’s algorithmic thinking and understanding of JavaScript programming. The interviewer expects the candidate to demonstrate their knowledge of the factorial concept.
Sample answer: A factorial number is the product of all positive integers, which are equal to or less than the given number.
function factorial(number) {
if (number === 0 || number === 1) {
return 1;
} else {
return number * factorial(number – 1);
7. Write a JavaScript function to check if a given number is prime.
Interviewers can analyze the candidate’s knowledge of JavaScript algorithms and mathematical concepts. They expect the candidate to translate a mathematical concept into functional code.
Sample answer: To check if a given number is prime, loop from 2 to the square root of the number. If any integer evenly divides it, the number is not prime.
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
return true;
8. Write a JavaScript program to find the largest element in a nested array.
When asking this question, interviewers are looking for the candidate’s ability to handle nested data structures and apply their knowledge of conditional statements, arrays, and loops. Candidates must apply their knowledge to real-world scenarios.
function findLargestElement(nestedArray) {
let largest = nestedArray[0][0];
for (let arr of nestedArray) {
for (let num of arr) {
if (num > largest) {
largest = num;
return largest;
9. Write a JavaScript function that returns the Fibonacci sequence up to a given number of terms.
This question helps hiring managers assess the interviewee’s understanding of fundamental algorithms in JavaScript. They expect the candidate to consider edge cases and handle errors.
function fibonacciSequence(numTerms) {
if (numTerms <= 0) return [];
if (numTerms === 1) return [0];
let sequence = [0, 1];
while (sequence.length < numTerms) {
let nextNumber = sequence[sequence.length – 1] + sequence[sequence.length – 2];
sequence.push(nextNumber);
return sequence;
10. Write a JavaScript program to convert a string to title case (capitalize the first letter of each word).
Interviewers analyze the candidate’s ability to break down a problem into manageable steps and demonstrate knowledge of string manipulation, looping, and basic JavaScript functions.
function toTitleCase(str) {
return str.replace(/\b\w/g, l => l.toUpperCase());
A dvanced JavaScript coding interview questions
Advanced JavaScript coding includes various complex concepts and techniques. Such key concepts are often tested in JavaScript interviews. Some of the concepts are – closure and scope, prototypal inheritance, functional programming , design patterns, memory management, ES6+ features, and many more.
1. Implement a debounce function in JavaScript that limits the frequency of a function’s execution when it’s called repeatedly within a specified time frame.
Interviewers expect the candidate to showcase their ability to clearly explain the purpose of the debounce function and its usage in scenarios where function calls need to be controlled. They are looking for the person’s ability to articulate technical concepts clearly.
Sample answer: By delaying the execution of the debounce function until the specified time frame has passed, the frequency can be limited.
function debounce(func, delay) {
let timer;
return function() {
clearTimeout(timer);
timer = setTimeout(func, delay);
2. Write a function that takes an array of objects and a key, and returns a new array sorted based on the values of that key in ascending order.
By asking this question, hiring managers analyze how well the candidate can discuss the sorting algorithm and its time complexity. It’s also crucial for candidates to demonstrate their code’s robustness.
Sample answer: The following function takes an array of objects and a key to sort the array based on the values in ascending order.
function sortByKey(arr, key) {
return arr.sort((a, b) => a[key] – b[key]);
3. Implement a deep clone function in JavaScript that creates a copy of a nested object or array without any reference to the original.
Hiring managers want to assess the interviewee’s skill to handle complex coding tasks and understand the concept of avoiding reference issues while cloning.
Sample answer: By using two methods together and creating a deep clone, I can serialize the object to a JSON string. I would then parse it back into a new object, thereby removing any reference to the original object.
function deepClone(obj) {
return JSON.parse(JSON.stringify(obj));
4. Write a recursive function to calculate the factorial of a given number.
Interviewers expect the candidate to write a concise recursive function that handles edge cases. Candidates must show their understanding of how recursion works to avoid infinite loops or stack overflow errors.
function factorial(num) {
if (num <= 1) return 1;
return num * factorial(num – 1);
5. Implement a function that takes two sorted arrays and merges them into a single sorted array without using any built-in sorting functions.
When interviewers ask this question, they seek to assess the knowledge of algorithms and efficiency in handling sorted data. They also look for the ability to think of and execute a correct solution.
Sample answer: I can implement a function that can efficiently merge two sorted arrays.
function mergeSortedArrays(arr1, arr2) {
return […arr1, …arr2].sort((a, b) => a – b);
6. Write a function that checks if a given string is a palindrome, considering only alphanumeric characters and ignoring case.
Interviewers analyze the interviewee’s approach to execute code and demonstrate familiarity with handling case-sensitive and alphanumeric checks, regular expressions, and JavaScript string methods.
const cleanStr = str.replace(/[^a-zA-Z0-9]/g, ”).toLowerCase();
const reversedStr = cleanStr.split(”).reverse().join(”);
return cleanStr === reversedStr;
7. Create a JavaScript class for a linked list with methods to insert a node at the beginning, end, or at a specific position, and to delete a node from a given position.
By asking this question, interviewers can evaluate how well a candidate can design and implement a class for a linked list while also presenting their problem-solving skills .
Sample answer: I would implement a linked list with methods to insert a node at the beginning, end, and at specific positions. Then, I would delete a node from a given position.
8. Implement a function that flattens a nested array in JavaScript, converting it into a single-level array.
Managers can gauge the candidate’s logical thinking skills and capability to handle complex data structures. Interviewees should demonstrate their knowledge of loops, recursion, and arrays.
const flattenArray = (nestedArray) => {
return nestedArray.flat(Infinity);
9. Write a function that determines if two strings are anagrams of each other
When interviewers present this question, they aim to measure how well the candidate can use appropriate string-related methods and identify anagrams accurately.
function areAnagrams(str1, str2) {
return str1.split(“”).sort().join(“”) === str2.split(“”).sort().join(“”);
10. Create a JavaScript function that returns the Fibonacci sequence up to a given number, utilizing memoization for optimized performance.
Interviewees are expected to show their proficiency in OOP and familiarity with recursion and memoization. They can also determine the candidate’s attention to detail in class design and organizing code.
Sample answer: By creating a function that uses an array to store the computed values, a Fibonacci sequence can be generated.
function fibonacciWithMemoization(n) {
let memo = [0, 1];
for (let i = 2; i <= n; i++) {
memo[i] = memo[i – 1] + memo[i – 2];
return memo;
Common JavaScript coding interview questions
Some of the common JavaScript coding interview questions typically cover these topics: checking for palindrome, finding missing/largest numbers, object manipulation, removing duplicates, merging, etc.
1. Write a function to check if a given string is a palindrome.
Hiring managers review how well a candidate can handle edge cases while handling case sensitivity, punctuation, and whitespace.
Sample answer: This function takes a string as input to convert it into lowercase and then compares it with its reverse. The string can be deemed a palindrome if the two match.
return str.toLowerCase() === str.toLowerCase().split(”).reverse().join(”);
2. Implement a function to reverse a string without using the built-in reverse() method.
Hiring managers aim to analyze the candidate’s knowledge of string manipulation in JavaScript while also measuring their ability to think of alternative solutions.
Sample answer: I would use a for lopp to iterate through the characters from the end to the beginning. By appending the character to a new string, it results in the reversed output.
function reverseString(str) {
let reversed = ”;
for (let i = str.length – 1; i >= 0; i–) {
reversed += str[i];
return reversed;
3. Given an array of numbers, write a function to find the largest and smallest numbers in the array.
By presenting the candidates with this question, managers can gauge how well a candidate is familiar with basic JavaScript functions and array manipulation.
Sample answer: I would use the following functions to find the smallest and largest numbers in the array.
function findMinMax(arr) {
let min = Math.min(…arr);
let max = Math.max(…arr);
return [min, max];
4. Write a function that takes an array of integers as input and returns a new array with only the unique elements.
Hiring managers can evaluate the candidate’s knowledge of JavaScript functions, array handling capabilities, and communicating technical concepts.
function getUniqueElements(arr) {
return Array.from(new Set(arr));
5. Implement a function to find the factorial of a given number.
Interviewers can determine the candidate’s capability to execute functional code and ability to handle input validation and edge cases. Interviewers also assess the ability to use concise and effective code and provide efficient code implementation.
if (number === 0 || number === 1) return 1;
return number * factorial(number – 1);
6. Write a function that determines if a given number is prime or not.
By asking this question, interviewers can understand how good the candidate is proficient in math operations and JavaScript logic. The interviewee should excute a clean and optimized solution that is efficient.
7. Implement a function to find the sum of all the numbers in an array.
Such a question helps understand if the interviewee can manipulate arrays and handle numeric values. This also helps managers assess problem-solving capabilities and ability to pay attention to code efficiency.
Sample answer: I would use the reduce method to implement the following function:
function findSum(arr) {
return arr.reduce((sum, num) => sum + num, 0);
8. Given a string, write a function to count the occurrences of each character in the string.
Hiring managers expect the candidate to be familiar with string manipulation and loop constructs. When they ask this question, they can evaluate whether the candidate knows data structures.
function countCharacterOccurrences(str) {
const charCount = {};
for (let char of str) {
charCount[char] = (charCount[char] || 0) + 1;
return charCount;
9. Implement a function to remove duplicates from an array.
When interviewers present the candidate with this question, they can gauge the level of understanding a candidate has regarding array methods and different approaches to solve the problem.
Sample answer: The following function duplicates from an array by converting it into a Set. This automatically removes duplicates. Next, the function converts the Set back into an array.
function removeDuplicates(arr) {
10. Write a function that sorts an array of numbers in ascending order.
Interviewees must show their knowledge of bubble sort, merge sort, sorting algorithms, and other approaches. The HR manager aims to measure the capability to execute strong algorithms and handle edge cases.
Sample answer: I can solve this by using JavaScript’s built-in sort method.
function ascendingSort(numbers) {
return numbers.sort((a, b) => a – b);
Tricky JavaScript coding questions
By asking tricky JavaScript coding questions, managers can assess problem—solving skills, JavaScript concepts, and critical thinking . These go beyond syntax knowledge and require the candidate to think creatively and logically to solve problems.
1. Write a function that reverses the order of words in a sentence without using the built-in reverse() method.
This question not only assesses the creativity of the candidates but also helps hiring managers understand how well a candidate can come up with a clean and understandable solution.
function reverseSentence(sentence) {
const words = sentence.split(‘ ‘);
const reversedWords = words.reverse();
return reversedWords.join(‘ ‘);
2. Implement a function that checks if a given string is a palindrome (reads the same forwards and backwards) while ignoring whitespace and punctuation.
Interviewers can gauge the interviewee’s capability to handle whitespace and punctuation gracefully while also maintaining the palindrome-checking logic. Candidates must express their knowledge of regular expressions or any other efficient approach.
const cleanedStr = str.replace(/[^\w]/g, ”).toLowerCase();
const reversedStr = cleanedStr.split(”).reverse().join(”);
return cleanedStr === reversedStr;
3. Write a function that takes an array of integers and returns the largest difference between any two numbers in the array.
Candidates should demonstrate their approach to finding the maximum difference between the array elements to handle edge cases and invalid inputs.
function largestDifference(arr) {
let min = arr[0];
let maxDiff = 0;
for (let i = 1; i < arr.length; i++) {
if (arr[i] < min) {
min = arr[i];
const diff = arr[i] – min;
if (diff > maxDiff) {
maxDiff = diff;
return maxDiff;
4. Implement a function that removes duplicates from an array, keeping only the unique elements.
Interviewers can analyze how well a candidate can effectively communicate code explanations and their familiarity with algorithmic efficiency.
return arr.filter((item, index) => arr.indexOf(item) === index);
5. Write a function that accepts a number and returns its factorial (e.g., factorial of 5 is 5 x 4 x 3 x 2 x 1).
By presenting this question in the interview, hiring managers can assess the capability of the candidate to handle numeric calculations. They can also determine how well the interviewee can pay attention to handling edge cases, if applicable.
if (num === 0 || num === 1) {
return num * factorial(num – 1);
6. Implement a function that flattens a nested array into a single-dimensional array.
Interviewers expect the candidates to demonstrate their ability to work with complex data structures and use appropriate techniques to accomplish tasks.
function flattenArray(arr) {
return arr.flat();
7. Write a function that checks if a given string is an anagram of another string (contains the same characters in a different order).
Candidates should showcase how well they can handle complex algorithms and logic. Interviewers are specifically looking for knowledge in string methods, data structures, and loop constructs.
function isAnagram(str1, str2) {
const sortedStr1 = str1.split(”).sort().join(”);
const sortedStr2 = str2.split(”).sort().join(”);
return sortedStr1 === sortedStr2;
8. Implement a function that finds the second smallest element in an array of integers.
Interviewers can measure the candidate’s problem-solving skills and their understanding of conditional statements, loops, and arrays.
function secondSmallest(arr) {
const sortedArr = arr.sort((a, b) => a – b);
return sortedArr[1];
9. Write a function that generates a random alphanumeric string of a given length.
By asking this question, interviewers can understand how well a candidate can ensure the function produces a reliable and consistent random output.
function generateRandomString(length) {
const characters = ‘ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789’;
let result = ”;
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * characters.length);
result += characters.charAt(randomIndex);
return result;
10. Implement a function that converts a number to its Roman numeral representation.
Hiring managers can gauge a candidate’s capability to implement coding solutions and create an efficient algorithm.
Sample answers:
function toRomanNumeral(number) {
// Implement your code here
JavaScript array coding questions
JavaScript array coding interview questions are technical questions asked to gauge candidates’ ability to work with arrays along with their familiarity with fundamental data structures.
1. Write a function that returns the sum of all numbers in an array.
By asking such a question, hiring managers can evaluate whether the candidate would be able to perform common tasks and solve basic coding challenges.
Sample answer:
function sumArray(arr) {
return arr.reduce((total, num) => total + num, 0);
2. Implement a function that finds the maximum number in an array.
Depending on the candidate’s answer, the manager can determine how effectively the interviewee can work with arrays. The managers can also understand the capability to communicate technical solutions.
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
return max;
3. Write a function that returns a new array containing only the unique elements from an input array.
The hiring manager is specifically looking for candidates who can demonstrate an understanding in data manipulation and JavaScript arrays. Additionally, interviewers evaluate how well the candidate strives for an optimized solution without duplicate elements.
function getUniqueElements(inputArray) {
return […new Set(inputArray)];
4. Implement a function that returns the average value of numbers in an array.
By asking this question, hiring managers can assess the candidate’s knowledge of arithmetic operations, array manipulation, and looping.
function calculateAverage(numbers) {
let sum = 0;
for (let number of numbers) {
sum += number;
return sum / numbers.length;
5. Write a function that sorts an array of strings in alphabetical order.
When interviewers present this question in the interview, they expect the candidate to be familiar with sorting algorithms and JavaScript array manipulation.
Sample answer:
function sortStrings(arr) {
return arr.slice().sort();
6. Implement a function that finds the index of a specific element in an array. If the element is not found, the function should return -1.
Interviewers aim to gauge the candidate’s proficiency in use of array methods, handling edge cases, and in JavaScript syntax. Candidates must implement the function proper error handling.
function findElementIndex(arr, element) {
const index = arr.indexOf(element);
return index !== -1 ? index : -1;
7. Write a function that removes all falsy values (false, null, 0, “”, undefined, and NaN) from an array.
Candidates must showcase communication skills and explain their solutions logically. Interviewers analyze the interviewee’s ability to write a function that filters false values from an array.
function removeFalsyValues(arr) {
return arr.filter(Boolean);
8. Implement a function that merges two arrays into a single array, alternating elements from each array.
Hiring managers determine a candidate’s ability to craft efficient algorithms and knowledge of array manipulation.
function mergeArrays(array1, array2) {
const mergedArray = [];
const maxLength = Math.max(array1.length, array2.length);
for (let i = 0; i < maxLength; i++) {
if (i < array1.length) mergedArray.push(array1[i]);
if (i < array2.length) mergedArray.push(array2[i]);
return mergedArray;
9. Write a function that finds the second largest number in an array.
Such a question reveals to the interviewers how well the candidate can use loops and array methods, work with them, and utilize logic to find solutions.
function findSecondLargest(arr) {
arr.sort((a, b) => b – a);
return arr[1];
10. Implement a function that groups elements in an array based on a given condition. For example, grouping even and odd numbers into separate arrays.
When interviews ask this question, they aim to evaluate a candidate’s understanding of concepts like array methods, conditional statements, and other technical concepts. Candidate should demonstrate good coding style.
function groupByCondition(arr, condition) {
return [
arr.filter(element => condition(element)),
arr.filter(element => !condition(element))
Tips to prepare for a JavaScript coding interview
There are 5 tips a candidate should keep in mind when attending a JavaScript coding interview:
- Master JavaScript basics: Candidates must have a solid understanding of core JavaScript fundamentals, including variables, loops, conditionals, objects, and data types. Additionally, practicing coding skills is important.
- Explore popular libraries and frameworks: Familiarizing oneself with the most common JavaScript libraries and frameworks like Vue.js, React, etc., helps understand key concepts.
- Practice whiteboard Java coding: Java coding skills can further be sharpened by practicing whiteboard coding. Candidates should solve coding challenges and explain their thought processes loudly. They should mainly focus on simplicity, clarity, and efficiency.
- Test code : After writing Java solutions, test it with various inputs and ensure it works correctly and can handle edge cases. Consider the time complexity of the solutions and aim for efficiency.
- Review JavaScript projects : Discussing JavaScript projects in the interview is essential. Interviewees should explain their approach clearly and how they overcame the challenges.
There are some red flags hiring managers should watch out for in a JavaScript coding interview:
- Lack of basic JavaScript knowledge
- Providing inefficient solutions
- No practical problem-solving abilities
- Limited knowledge of asynchronous programming
- Copy-pasting code
- Inability to articulate thought processes or provide a clear explanation of their approach to the problem
- Unfamiliarity with Modern JavaScript
- Failure to provide error handling and consider edge cases
Candidates are evaluated majorly on their understanding of core JavaScript concepts and skills. By mastering the fundamentals and practicing, candidates can crack their interview. Also, it’s important to focus on being clear, accurate , and honest in the interview.
Related Interview Questions
- Java programming interview questions and Answers
- Top 40 Java Interview Questions and Answers 2023
Related Job Descriptions
- Java Developer Job Description
- Full Stack Developer Job Description Template
By clicking “Accept", you consent to our website's use of cookies to give you the most relevant experience by remembering your preferences and repeat visits. You may visit "cookie policy” to know more about cookies we use.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Tutorial
JavaScript Basics
- Introduction to JavaScript
- JavaScript Versions
- How to Add JavaScript in HTML Document?
- JavaScript Statements
- JavaScript Syntax
- JavaScript Output
- JavaScript Comments
JS Variables & Datatypes
- Variables and Datatypes in JavaScript
- Global and Local variables in JavaScript
- JavaScript Let
- JavaScript Const
- JavaScript var
JS Operators
- JavaScript Operators
- Operator precedence in JavaScript
- JavaScript Arithmetic Operators
JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
- JavaScript Bitwise Operators
- JavaScript Ternary Operator
- JavaScript Comma Operator
- JavaScript Unary Operators
- JavaScript Relational operators
- JavaScript String Operators
- JavaScript Loops
- 7 Loops of JavaScript
- JavaScript For Loop
- JavaScript While Loop
- JavaScript for-in Loop
- JavaScript for...of Loop
- JavaScript do...while Loop
JS Perfomance & Debugging
- JavaScript | Performance
- Debugging in JavaScript
- JavaScript Errors Throw and Try to Catch
- Objects in Javascript
- Introduction to Object Oriented Programming in JavaScript
- JavaScript Objects
- Creating objects in JavaScript (4 Different Ways)
- JavaScript JSON Objects
- JavaScript Object Reference
JS Function
- Functions in JavaScript
- How to write a function in JavaScript ?
- JavaScript Function Call
- Different ways of writing functions in JavaScript
- Difference between Methods and Functions in JavaScript
- Explain the Different Function States in JavaScript
- JavaScript Function Complete Reference
- JavaScript Arrays
- JavaScript Array Methods
- Best-Known JavaScript Array Methods
- What are the Important Array Methods of JavaScript ?
- JavaScript Array Reference
- JavaScript Strings
- JavaScript String Methods
- JavaScript String Reference
- JavaScript Numbers
- How numbers are stored in JavaScript ?
- How to create a Number object using JavaScript ?
- JavaScript Number Reference
- JavaScript Math Object
- What is the use of Math object in JavaScript ?
- JavaScript Math Reference
- JavaScript Map
- What is JavaScript Map and how to use it ?
- JavaScript Map Reference
- Sets in JavaScript
- How are elements ordered in a Set in JavaScript ?
- How to iterate over Set elements in JavaScript ?
- How to sort a set in JavaScript ?
- JavaScript Set Reference
- JavaScript Date
- JavaScript Promise
- JavaScript BigInt
- JavaScript Boolean
- JavaScript Proxy/Handler
- JavaScript WeakMap
- JavaScript WeakSet
- JavaScript Function Generator
- JavaScript JSON
- Arrow functions in JavaScript
- JavaScript this Keyword
- Strict mode in JavaScript
- Introduction to ES6
- JavaScript Hoisting
- Async and Await in JavaScript
JavaScript Exercises
- JavaScript Exercises, Practice Questions and Solutions
A ssignment Operators
Assignment operators are used to assign values to variables in JavaScript.
Assignment Operators List
There are so many assignment operators as shown in the table with the description.
Below we have described each operator with an example code:
Addition assignment operator(+=).
The Addition assignment operator adds the value to the right operand to a variable and assigns the result to the variable. Addition or concatenation is possible. In case of concatenation then we use the string as an operand.
Subtraction Assignment Operator(-=)
The Substraction Assignment Operator subtracts the value of the right operand from a variable and assigns the result to the variable.
Multiplication Assignment Operator(*=)
The Multiplication Assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable.
Division Assignment Operator(/=)
The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable.
Remainder Assignment Operator(%=)
The Remainder Assignment Operator divides a variable by the value of the right operand and assigns the remainder to the variable.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator raises the value of a variable to the power of the right operand.
Left Shift Assignment Operator(<<=)
This Left Shift Assignment O perator moves the specified amount of bits to the left and assigns the result to the variable.
Right Shift Assignment O perator(>>=)
The Right Shift Assignment Operator moves the specified amount of bits to the right and assigns the result to the variable.
Bitwise AND Assignment Operator(&=)
The Bitwise AND Assignment Operator uses the binary representation of both operands, does a bitwise AND operation on them, and assigns the result to the variable.
Btwise OR Assignment Operator(|=)
The Btwise OR Assignment Operator uses the binary representation of both operands, does a bitwise OR operation on them, and assigns the result to the variable.
Bitwise XOR Assignment Operator(^=)
The Bitwise XOR Assignment Operator uses the binary representation of both operands, does a bitwise XOR operation on them, and assigns the result to the variable.
Logical AND Assignment Operator(&&=)
The Logical AND Assignment assigns the value of y into x only if x is a truthy value.
Logical OR Assignment Operator( ||= )
The Logical OR Assignment Operator is used to assign the value of y to x if the value of x is falsy.
Nullish coalescing Assignment Operator(??=)
The Nullish coalescing Assignment Operator assigns the value of y to x if the value of x is null.
Supported Browsers: The browsers supported by all JavaScript Assignment operators are listed below:
- Google Chrome
- Microsoft Edge
- Internet Explorer
Please Login to comment...
Similar reads.
- javascript-operators
- Web Technologies
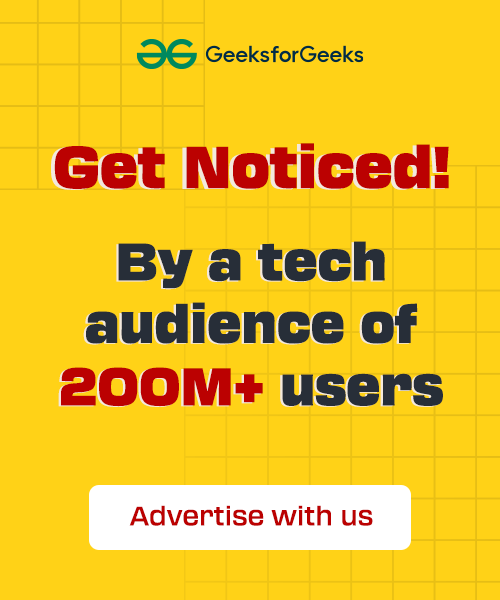
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO
COMMENTS
JavaScript Exercises, Practice, Solution: JavaScript is a cross-platform, object-oriented scripting language. Inside a host environment, JavaScript can be connected to the objects of its environment to provide programmatic control over them. ... The best way we learn anything is by practice and exercise questions. We have started this section ...
Embark on your JavaScript learning journey with our online practice portal. Start by selecting quizzes tailored to your skill level. Engage in hands-on coding exercises, receive real-time feedback, and monitor your progress. With our user-friendly platform, mastering JavaScript becomes an enjoyable and personalized experience.
We have gathered a variety of JavaScript exercises (with answers) for each JavaScript Chapter. Try to solve an exercise by editing some code, or show the answer to see what you've done wrong. Count Your Score. You will get 1 point for each correct answer. Your score and total score will always be displayed.
There is a single operator in JavaScript, capable of providing the remainder of a division operation. Two numbers are passed as parameters. The first parameter divided by the second parameter will have a remainder, possibly zero. Return that value. Examples remainder(1, 3) 1 remainder(3, 4) 3 remainder(-9, 45) -9 r …
JavaScript interview questions range from the basics like explaining the different data types in JavaScript to more complicated concepts like generator functions and async and await. Each question will have answers and examples you can use to prepare for your own interview. ... null is an assignment value that represents no value or an empty ...
JSchallenger recognizes what skill level you're on and adjusts the difficulty of the next challenges automatically. Making you continuously improve your Javascript skills in a short amount of time. JSchallenger. Free Javascript challenges. Learn Javascript online by solving coding exercises. Javascript for all levels.
Code practice and mentorship for everyone. Develop fluency in 70 programming languages with our unique blend of learning, practice and mentoring. Exercism is fun, effective and 100% free, forever. Explore the 146 JavaScript exercises on Exercism.
JavaScript is also flexible in how it handles data. Unlike some other programming languages, you don't need to specify what kind of data a variable will hold. For example, you could create a variable called "x" and set it equal to a number, a string of text, or even an entire object. #2.
Toptal Connects the Top 3% of Freelance Talent All Over The World. Comprehensive, community-driven list of essential JavaScript interview questions. Whether you're a candidate or interviewer, these interview questions will help prepare you for your next JavaScript interview ahead of time.
Advanced JavaScript Concepts to Know - Async/defer, Polyfills, Debouncing, and Throttling. Storage in JavaScript. Caveat: The focus here will largely be to cover concepts relevant to the interview and not to create a comprehensive booklet for learning the language. Treat this more like a cheatsheet.
The average salary for a JavaScript developer in India is ₹7,51,785 per year. The average additional cash compensation is ₹1,46,359, with a range from ₹40,000 - ₹3,67,618. The salary of a JavaScript developer can vary based on several factors, including experience, location, and specific domain expertise.
I meticulously searched the JavaScript docs to create these questions. I hope they push the limits of your JS knowledge. Let me know in the comments if you enjoyed this list! Related: Test your TypeScript knowledge against these 50 difficult TypeScript questions. 1.
5. freeCodeCamp.org. freeCodeCamp is one of the best websites to learn to code from scratch, and they offer a ton of free JavaScript practice material ranging from beginner to intermediate level skills. The freeCodeCamp curriculum starts with HTML and CSS, after which you will start learning and practising JavaScript.
Animal Fun Facts. HTML & CSS • JavaScript • Web Development Using a variety of JSX concepts, build an interactive interface that will display a selection of animal images and allow users to click an image for a fun fact. More guidance, 30 min. Practice Project.
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time. Check 25+ JavaScript Coding Interview Questions (SOLVED with CODE) and Land Your Next Six-Figure Job Offer! 100% Tech Interview Success!
Expected Output : mm-dd-yyyy, mm/dd/yyyy or dd-mm-yyyy, dd/mm/yyyy Click me to see the solution. 4. Write a JavaScript program to find the area of a triangle where three sides are 5, 6, 7. Click me to see the solution. 5. Write a JavaScript program to rotate the string 'w3resource' in the right direction.
JavaScript, created by Brendan Eich in 1995, is one of the most widely used web development languages. It was designed to build dynamic web pages at first. A script is a JS program that may be added to the HTML of any web page. When the page loads, these scripts execute automatically.
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
JavaScript applications are meant to run inside a web browser. Java applications are generally made for use in operating systems and virtual machines. JavaScript does not need compilation before running the application code. Java source code needs a compiler before it can be ready to run in realtime. 3.
The basic JavaScript coding interview questions are: 1. Write a JavaScript function to calculate the sum of two numbers. When managers ask this question, they are looking for the candidate's basic understanding of JavaScript. They assess their understanding of basic syntax along with problem-solving skills.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
This question assesses your knowledge of variable declaration in JavaScript. Explain that "let" and "const" are block-scoped variables, while "var" is function-scoped. Emphasize that ...
You need to test for that first, and when it's clear that data.area is a proper object that has (or can have) a "width" property, you can do a simple assignment to data.area.width.
Division Assignment Operator (/=) The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable. Example: Javascript. let yoo = 10; const moo = 2; // Expected output 5 console.log(yoo = yoo / moo); // Expected output Infinity console.log(yoo /= 0); Output: